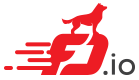 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
25 #include <vnet/pg/pg.api_enum.h>
26 #include <vnet/pg/pg.api_types.h>
28 #define REPLY_MSG_ID_BASE pg->msg_id_base
71 vl_api_pg_interface_enable_disable_coalesce_reply_t *rmp;
91 rv = VNET_API_ERROR_CANNOT_ENABLE_DISABLE_FEATURE;
95 rv = VNET_API_ERROR_NO_MATCHING_INTERFACE;
99 REPLY_MACRO (VL_API_PG_INTERFACE_ENABLE_DISABLE_COALESCE_REPLY);
106 vl_api_pg_capture_reply_t *rmp;
115 u32 hw_if_index = ~0;
121 if (hw_if_index != ~0)
124 char *pcap_file_name =
128 a->hw_if_index = hw_if_index;
129 a->dev_instance =
hi->dev_instance;
131 a->pcap_file_name = pcap_file_name;
132 a->count = ntohl (mp->
count);
138 rv = VNET_API_ERROR_CANNOT_CREATE_PCAP_FILE;
149 vl_api_pg_enable_disable_reply_t *rmp;
153 u32 stream_index = ~0;
171 #include <vnet/pg/pg.api.c>
void pg_interface_enable_disable_coalesce(pg_interface_t *pi, u8 enable, u32 tx_node_index)
vnet_interface_main_t * im
#define VALIDATE_SW_IF_INDEX(mp)
VLIB_API_INIT_FUNCTION(pg_api_hookup)
bool is_enabled[default=true]
#define REPLY_MACRO2(t, body)
u32 pg_interface_add_or_get(pg_main_t *pg, uword stream_index, u8 gso_enabled, u32 gso_size, u8 coalesce_enabled, pg_interface_mode_t mode)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
static void vl_api_pg_enable_disable_t_handler(vl_api_pg_enable_disable_t *mp)
static void vl_api_pg_create_interface_v2_t_handler(vl_api_pg_create_interface_v2_t *mp)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
clib_error_t * pg_capture(pg_capture_args_t *a)
vl_api_pg_interface_mode_t mode
#define clib_error_report(e)
vl_api_interface_index_t sw_if_index
Enable / disable packet generator request.
PacketGenerator interface enable/disable packet coalesce.
bool is_enabled[default=true]
vnet_main_t * vnet_get_main(void)
vl_api_interface_index_t interface_id
static void setup_message_id_table(api_main_t *am)
vl_api_interface_index_t interface_id
static vnet_hw_interface_t * vnet_get_sup_hw_interface_api_visible_or_null(vnet_main_t *vnm, u32 sw_if_index)
PacketGenerator capture packets on given interface request.
PacketGenerator create interface request.
static clib_error_t * pg_api_hookup(vlib_main_t *vm)
u32 vl_api_string_len(vl_api_string_t *astr)
#define BAD_SW_IF_INDEX_LABEL
#define hash_get_mem(h, key)
#define vec_free(V)
Free vector's memory (no header).
pg_interface_t * interfaces
uword * stream_index_by_name
description fragment has unexpected format
PacketGenerator create interface response.
void pg_enable_disable(u32 stream_index, int is_enable)
static vnet_hw_interface_t * vnet_get_sup_hw_interface(vnet_main_t *vnm, u32 sw_if_index)
vl_api_interface_index_t interface_id
vl_api_interface_index_t sw_if_index
static void vl_api_pg_create_interface_t_handler(vl_api_pg_create_interface_t *mp)
uword * hw_interface_by_name
u8 * vl_api_from_api_to_new_vec(void *mp, vl_api_string_t *astr)
#define vec_terminate_c_string(V)
(If necessary) NULL terminate a vector containing a c-string.
static void vl_api_pg_capture_t_handler(vl_api_pg_capture_t *mp)
static void vl_api_pg_interface_enable_disable_coalesce_t_handler(vl_api_pg_interface_enable_disable_coalesce_t *mp)
vl_api_interface_index_t sw_if_index
char * vl_api_from_api_to_new_c_string(vl_api_string_t *astr)
vnet_interface_main_t interface_main
vl_api_interface_index_t sw_if_index