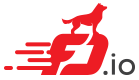 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
52 .path =
"packet-generator",
53 .short_help =
"Packet generator commands",
63 if (stream_index == ~0)
86 if (
a->is_enabled == 1)
89 if (stat (
a->pcap_file_name, &sb) != -1)
101 if (
a->is_enabled == 0)
119 u32 stream_index = ~0;
143 .path =
"packet-generator enable-stream",
144 .short_help =
"Enable packet generator streams",
152 .path =
"packet-generator disable-stream",
153 .short_help =
"Disable packet generator streams",
174 while (*junk_after_name && *junk_after_name !=
' ')
176 *junk_after_name = 0;
188 int verbose = va_arg (*va,
int);
191 return format (s,
"%-16s%=12s%=16s%s",
192 "Name",
"Enabled",
"Count",
"Parameters");
194 s =
format (s,
"%-16v%=12s%=16Ld",
203 s =
format (s,
"size %d%c%d, ",
259 .path =
"show packet-generator ",
260 .short_help =
"show packet-generator [verbose]",
295 if (
unformat (input,
"limit %f", &x))
298 else if (
unformat (input,
"rate %f", &x))
342 return (
"ethernet-input");
344 return (
"ip4-input");
346 return (
"ip6-input");
350 return (
"ethernet-input");
359 u32 maxframe, hw_if_index;
361 int sub_input_given = 0;
365 char *pcap_file_name;
384 else if (
unformat (input,
"node %U",
396 else if (
unformat (input,
"buffer-flags %U",
399 else if (
unformat (input,
"buffer-offload-flags %U",
402 else if (
unformat (input,
"node %U",
405 else if (
unformat (input,
"maxframe %u", &maxframe))
410 else if (
unformat (input,
"interface %U",
414 else if (
unformat (input,
"tx-interface %U",
419 else if (
unformat (input,
"pcap %s", &pcap_file_name))
422 else if (!sub_input_given
437 if (!sub_input_given && !pcap_file_name)
445 if (pcap_file_name != 0)
476 if (pcap_file_name != 0)
491 (
"failed to parse packet data from `%U'",
512 .path =
"packet-generator new",
514 .short_help =
"Create packet generator stream",
516 "Create packet generator stream\n"
520 "name STRING sets stream name\n"
521 "interface STRING interface for stream output \n"
522 "node NODE-NAME node for stream output\n"
523 "data STRING specifies packet data\n"
524 "pcap FILENAME read packet data from pcap file\n"
525 "rate PPS rate to transfer packet data\n"
526 "maxframe NPKTS maximum number of packets per frame\n",
548 .path =
"packet-generator delete",
550 .short_help =
"Delete stream with given name",
560 u32 stream_index = ~0;
595 .path =
"packet-generator configure",
596 .short_help =
"Change packet generator stream parameters",
609 u8 *pcap_file_name = 0;
625 else if (
unformat (line_input,
"pcap %s", &pcap_file_name))
629 else if (
unformat (line_input,
"disable"))
653 if (!pcap_file_name && is_disable == 0)
662 a->hw_if_index = hw_if_index;
663 a->dev_instance =
hi->dev_instance;
664 a->is_enabled = !is_disable;
665 a->pcap_file_name = (
char *) pcap_file_name;
678 .path =
"packet-generator capture",
679 .short_help =
"packet-generator capture <interface name> pcap <filename> [count <n>]",
690 u32 if_id, gso_enabled = 0, gso_size = 0, coalesce_enabled = 0;
698 if (
unformat (line_input,
"interface pg%u", &if_id))
700 else if (
unformat (line_input,
"coalesce-enabled"))
701 coalesce_enabled = 1;
702 else if (
unformat (line_input,
"gso-enabled"))
705 if (
unformat (line_input,
"gso-size %u", &gso_size))
732 .path =
"create packet-generator",
733 .short_help =
"create packet-generator interface <interface name>"
734 " [gso-enabled gso-size <size> [coalesce-enabled]]",
static clib_error_t * new_stream(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
pg_edit_group_t * edit_groups
vnet_device_class_t pg_dev_class
pg_edit_type_t packet_size_edit_type
static u32 vlib_num_workers()
__clib_export clib_error_t * pcap_read(pcap_main_t *pm)
Read PCAP file.
static uword pg_edit_group_n_bytes(pg_stream_t *s, u32 group_index)
static vlib_cli_command_t change_stream_parameters_cli
(constructor) VLIB_CLI_COMMAND (change_stream_parameters_cli)
u32 pg_interface_add_or_get(pg_main_t *pg, uword stream_index, u8 gso_enabled, u32 gso_size, u8 coalesce_enabled, pg_interface_mode_t mode)
void pg_stream_del(pg_main_t *pg, uword index)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
u32 min_packet_bytes
Min/Max Packet bytes.
static vlib_cli_command_t del_stream_cli
(constructor) VLIB_CLI_COMMAND (del_stream_cli)
__clib_export clib_error_t * pcap_close(pcap_main_t *pm)
Close PCAP file.
#define clib_error_return(e, args...)
u64 * replay_packet_timestamps
static vlib_cli_command_t enable_streams_cli
(constructor) VLIB_CLI_COMMAND (enable_streams_cli)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
clib_error_t * pg_capture(pg_capture_args_t *a)
static clib_error_t * pg_cli_init(vlib_main_t *vm)
static vlib_cli_command_t pg_capture_cmd
(constructor) VLIB_CLI_COMMAND (pg_capture_cmd)
pcap_packet_type_t packet_type
Packet type.
void pg_stream_change(pg_main_t *pg, pg_stream_t *s)
static vlib_cli_command_t disable_streams_cli
(constructor) VLIB_CLI_COMMAND (disable_streams_cli)
PCAP main state data structure.
static u8 * format_pg_edit_group(u8 *s, va_list *va)
u64 * timestamps
Timestamps.
void pg_stream_enable_disable(pg_main_t *pg, pg_stream_t *s, int is_enable)
int file_descriptor
File descriptor for reading/writing.
#define pool_foreach(VAR, POOL)
Iterate through pool.
#define clib_error_create(args...)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
unformat_function_t * unformat_edit
static clib_error_t * show_streams(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
u32 sw_if_index[VLIB_N_RX_TX]
vnet_main_t * vnet_get_main(void)
static void pg_stream_free(pg_stream_t *s)
unformat_function_t unformat_vlib_node
static clib_error_t * validate_stream(pg_stream_t *s)
f64 rate_packets_per_second
PCAP utility definitions.
void pg_stream_add(pg_main_t *pg, pg_stream_t *s_init)
#define VLIB_CLI_COMMAND(x,...)
format_function_t format_clib_elf_symbol_with_address
unformat_function_t unformat_vnet_hw_interface
u8 ** packets_read
Packets read from file.
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static clib_error_t * change_stream_parameters(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static clib_error_t * pg_pcap_read(pg_stream_t *s, char *file_name)
static vlib_cli_command_t show_streams_cli
(constructor) VLIB_CLI_COMMAND (show_streams_cli)
unformat_function_t unformat_vnet_buffer_offload_flags
uword unformat_pg_payload(unformat_input_t *input, va_list *args)
unformat_function_t unformat_hash_vec_string
#define vec_free(V)
Free vector's memory (no header).
pg_interface_t * interfaces
vlib_node_t * vlib_get_node_by_name(vlib_main_t *vm, u8 *name)
uword * stream_index_by_name
unformat_function_t unformat_vnet_sw_interface
description fragment has unexpected format
void pg_enable_disable(u32 stream_index, int is_enable)
static_always_inline u32 vlib_buffer_get_default_data_size(vlib_main_t *vm)
#define VLIB_INIT_FUNCTION(x)
#define vec_foreach(var, vec)
Vector iterator.
const char * pg_interface_get_input_node(pg_interface_t *pi)
static uword pool_elts(void *v)
Number of active elements in a pool.
u32 n_packets_to_capture
Number of packets to capture.
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
static clib_error_t * del_stream(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
#define PCAP_MAIN_INIT_DONE
u8 ** replay_packet_templates
char * file_name
File name of pcap output.
static vlib_cli_command_t vlib_cli_pg_command
(constructor) VLIB_CLI_COMMAND (vlib_cli_pg_command)
static clib_error_t * create_pg_if_cmd_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
unformat_function_t unformat_vnet_buffer_flags
static clib_error_t * pg_capture_cmd_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
void(* edit_function)(struct pg_main_t *pg, struct pg_stream_t *s, struct pg_edit_group_t *g, u32 *buffers, u32 n_buffers)
static clib_error_t * enable_disable_stream(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static vlib_cli_command_t new_stream_cli
(constructor) VLIB_CLI_COMMAND (new_stream_cli)
unsigned char function_name[]
static int pg_stream_is_enabled(pg_stream_t *s)
static vlib_cli_command_t create_pg_if_cmd
(constructor) VLIB_CLI_COMMAND (create_pg_if_cmd)
static uword unformat_pg_stream_parameter(unformat_input_t *input, va_list *args)
static u8 * format_pg_stream(u8 *s, va_list *va)