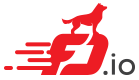 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
35 #define MAX_LUKPS_PER_PACKET 4
47 #define LOOKUP_SUB_TYPE_NUM (LOOKUP_SUB_TYPE_DST_TABLE_FROM_INTERFACE+1)
49 #define FOR_EACH_LOOKUP_SUB_TYPE(_st) \
50 for (_st = LOOKUP_SUB_TYPE_IP4_SRC; _st < LOOKUP_SUB_TYPE_NUM; _st++)
111 switch (table_config)
204 s =
format(s,
"%s,%s lookup in interface's %U table",
213 s =
format(s,
"%s,%s lookup in %U",
221 s =
format(s,
"%s,%s lookup in %U",
290 int table_from_interface)
309 u32 bi0, lkdi0, lbi0, fib_index0, next0, hash_c0;
317 u32 bi1, lkdi1, lbi1, fib_index1, next1, hash_c1;
364 if (table_from_interface)
395 input_addr1, &lbi0, &lbi1);
439 if (!(b0->
flags & VNET_BUFFER_F_LOOP_COUNTER_VALID)) {
441 b0->
flags |= VNET_BUFFER_F_LOOP_COUNTER_VALID;
443 if (!(b1->
flags & VNET_BUFFER_F_LOOP_COUNTER_VALID)) {
445 b1->
flags |= VNET_BUFFER_F_LOOP_COUNTER_VALID;
462 tr->
addr.ip4 = *input_addr0;
470 tr->
addr.ip4 = *input_addr1;
474 to_next, n_left_to_next,
475 bi0, bi1, next0, next1);
480 u32 bi0, lkdi0, lbi0, fib_index0, next0, hash_c0;
507 if (table_from_interface)
557 if (!(b0->
flags & VNET_BUFFER_F_LOOP_COUNTER_VALID)) {
559 b0->
flags |= VNET_BUFFER_F_LOOP_COUNTER_VALID;
573 tr->
addr.ip4 = *input_addr;
577 n_left_to_next, bi0, next0);
591 s =
format (s,
"%U fib-index:%d addr:%U load-balance:%d",
607 .name =
"lookup-ip4-dst",
608 .vector_size =
sizeof (
u32),
609 .sibling_of =
"ip4-lookup",
621 .name =
"lookup-ip4-dst-itf",
622 .vector_size =
sizeof (
u32),
623 .sibling_of =
"ip4-lookup",
635 .name =
"lookup-ip4-src",
636 .vector_size =
sizeof (
u32),
638 .sibling_of =
"ip4-lookup",
646 int table_from_interface)
665 u32 bi0, lkdi0, lbi0, fib_index0, next0, hash_c0;
667 const ip6_address_t *input_addr0;
673 u32 bi1, lkdi1, lbi1, fib_index1, next1, hash_c1;
675 const ip6_address_t *input_addr1;
720 if (table_from_interface)
766 if (!(b0->
flags & VNET_BUFFER_F_LOOP_COUNTER_VALID)) {
768 b0->
flags |= VNET_BUFFER_F_LOOP_COUNTER_VALID;
770 if (!(b1->
flags & VNET_BUFFER_F_LOOP_COUNTER_VALID)) {
772 b1->
flags |= VNET_BUFFER_F_LOOP_COUNTER_VALID;
822 tr->
addr.ip6 = *input_addr0;
830 tr->
addr.ip6 = *input_addr1;
833 n_left_to_next, bi0, bi1,
838 u32 bi0, lkdi0, lbi0, fib_index0, next0, hash_c0;
840 const ip6_address_t *input_addr0;
865 if (table_from_interface)
913 if (!(b0->
flags & VNET_BUFFER_F_LOOP_COUNTER_VALID)) {
915 b0->
flags |= VNET_BUFFER_F_LOOP_COUNTER_VALID;
933 tr->
addr.ip6 = *input_addr0;
936 n_left_to_next, bi0, next0);
951 .name =
"lookup-ip6-dst",
952 .vector_size =
sizeof (
u32),
954 .sibling_of =
"ip6-lookup",
965 .name =
"lookup-ip6-dst-itf",
966 .vector_size =
sizeof (
u32),
968 .sibling_of =
"ip6-lookup",
979 .name =
"lookup-ip6-src",
980 .vector_size =
sizeof (
u32),
982 .sibling_of =
"ip6-lookup",
989 int table_from_interface)
1011 u32 bi0, lkdi0, lbi0, fib_index0, next0, hash0;
1023 n_left_to_next -= 1;
1036 if (table_from_interface)
1090 vnet_buffer (b0)->mpls.exp = (((
char*)hdr0)[2] & 0xe) >> 1;
1094 if (!(b0->
flags & VNET_BUFFER_F_LOOP_COUNTER_VALID)) {
1096 b0->
flags |= VNET_BUFFER_F_LOOP_COUNTER_VALID;
1114 n_left_to_next, bi0, next0);
1132 s =
format (s,
"%U fib-index:%d hdr:%U load-balance:%d",
1148 .name =
"lookup-mpls-dst",
1149 .vector_size =
sizeof (
u32),
1150 .sibling_of =
"mpls-lookup",
1163 .name =
"lookup-mpls-dst-itf",
1164 .vector_size =
sizeof (
u32),
1165 .sibling_of =
"mpls-lookup",
1200 u32 bi0, lkdi0, fib_index0, next0;
1210 n_left_to_next -= 1;
1258 if (!(b0->
flags & VNET_BUFFER_F_LOOP_COUNTER_VALID)) {
1260 b0->
flags |= VNET_BUFFER_F_LOOP_COUNTER_VALID;
1269 n_left_to_next, bi0, next0);
1284 .name =
"lookup-ip4-dst-mcast",
1285 .vector_size =
sizeof (
u32),
1303 .name =
"lookup-ip6-dst-mcast",
1304 .vector_size =
sizeof (
u32),
1376 "lookup-ip4-dst-mcast",
1381 "lookup-ip6-dst-mcast",
1392 "lookup-ip4-dst-itf",
1397 "lookup-ip6-dst-itf",
1402 "lookup-mpls-dst-itf",
1451 .path =
"show lookup-dpo",
1452 .short_help =
"show lookup-dpo [<index>]",
const static char *const *const lookup_dst_nodes[DPO_PROTO_NUM]
#define LOOKUP_SUB_TYPE_NUM
static uword lookup_dpo_ip4_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *from_frame, int input_src_addr, int table_from_interface)
vlib_node_registration_t lookup_mpls_dst_itf_node
(constructor) VLIB_REGISTER_NODE (lookup_mpls_dst_itf_node)
u16 dpoi_next_node
The next VLIB node to follow.
dpo_type_t dpo_register_new_type(const dpo_vft_t *vft, const char *const *const *nodes)
Create and register a new DPO type.
u16 lb_n_buckets
number of buckets in the load-balance.
index_t dpoi_index
the index of objects of that type
vlib_node_registration_t lookup_mpls_dst_node
(constructor) VLIB_REGISTER_NODE (lookup_mpls_dst_node)
#define vlib_prefetch_buffer_header(b, type)
Prefetch buffer metadata.
static ip6_mfib_t * ip6_mfib_get(u32 index)
Get the FIB at the given index.
const static char *const *const lookup_src_nodes[DPO_PROTO_NUM]
mpls_unicast_header_t hdr
vlib_node_registration_t lookup_ip4_src_node
(constructor) VLIB_REGISTER_NODE (lookup_ip4_src_node)
static vlib_cli_command_t replicate_show_command
(constructor) VLIB_CLI_COMMAND (replicate_show_command)
nat44_ei_hairpin_src_next_t next_index
enum dpo_proto_t_ dpo_proto_t
Data path protocol.
static const char *const lookup_cast_names[]
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
static index_t lookup_dpo_get_index(lookup_dpo_t *lkd)
static lookup_dpo_t * lookup_dpo_get(index_t index)
static void lookup_dpo_unlock(dpo_id_t *dpo)
#define pool_get_aligned(P, E, A)
Allocate an object E from a pool P with alignment A.
@ LOOKUP_SUB_TYPE_DST_MCAST
vlib_main_t vlib_node_runtime_t * node
flow_hash_config_t lb_hash_config
the hash config to use when selecting a bucket.
vlib_node_registration_t lookup_ip4_dst_mcast_node
(constructor) VLIB_REGISTER_NODE (lookup_ip4_dst_mcast_node)
lookup_dpo_t * lookup_dpo_pool
pool of all MPLS Label DPOs
u8 * format_fib_table_name(u8 *s, va_list *ap)
Format the description/name of the table.
static uword lookup_dpo_ip6_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *from_frame, int input_src_addr, int table_from_interface)
u32 mfib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, mfib_source_t src)
Get the index of the FIB for a Table-ID.
static u8 * format_lookup_trace(u8 *s, va_list *args)
A representation of an MPLS label for imposition in the data-path.
#define FIB_NODE_INDEX_INVALID
enum lookup_table_t_ lookup_table_t
Switch to use the packet's source or destination address for lookup.
const static dpo_vft_t lkd_vft
u32 ip4_fib_table_get_index_for_sw_if_index(u32 sw_if_index)
#define pool_put(P, E)
Free an object E in pool P.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
fib_node_index_t fib_index
vlib_main_t vlib_node_runtime_t vlib_frame_t * from_frame
vlib_node_registration_t lookup_ip6_dst_itf_node
(constructor) VLIB_REGISTER_NODE (lookup_ip6_dst_itf_node)
#define dpo_pool_barrier_sync(VM, P, YESNO)
Barrier sync if a dpo pool is about to expand.
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
static uword lookup_dpo_ip_dst_mcast_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *from_frame, int is_v4)
u16 lkd_locks
Number of locks.
const static char *const lookup_dst_from_interface_ip6_nodes[]
@ LOOKUP_IP_DST_MCAST_N_NEXT
lookup_ip_dst_mcast_next_t_
format_function_t format_mpls_header
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
const static char *const lookup_dst_mcast_ip6_nodes[]
void mfib_table_lock(u32 fib_index, fib_protocol_t proto, mfib_source_t source)
Release a reference counting lock on the table.
static clib_error_t * lookup_dpo_show(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define pool_foreach(VAR, POOL)
Iterate through pool.
load_balance_main_t load_balance_main
The one instance of load-balance main.
u8 * format_lookup_dpo(u8 *s, va_list *args)
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
static const dpo_id_t * load_balance_get_fwd_bucket(const load_balance_t *lb, u16 bucket)
static u32 mpls_fib_table_get_index_for_sw_if_index(u32 sw_if_index)
@ IP_LOOKUP_NEXT_DROP
Adjacency to drop this packet.
#define MPLS_IS_REPLICATE
The top bit of the index, which is the result of the MPLS lookup is used to determine if the DPO is a...
#define VLIB_NODE_FN(node)
const static char *const lookup_dst_from_interface_ip4_nodes[]
static u32 ip6_compute_flow_hash(const ip6_header_t *ip, flow_hash_config_t flow_hash_config)
vlib_node_registration_t lookup_ip6_src_node
(constructor) VLIB_REGISTER_NODE (lookup_ip6_src_node)
const static char *const lookup_src_ip4_nodes[]
u32 fib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, fib_source_t src)
Get the index of the FIB for a Table-ID.
const static char *const *const lookup_dst_mcast_nodes[DPO_PROTO_NUM]
static_always_inline uword vlib_get_thread_index(void)
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
enum flow_hash_config_t_ flow_hash_config_t
A flow hash configuration is a mask of the flow hash options.
const static char *const lookup_dst_ip6_nodes[]
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
static lookup_dpo_t * lookup_dpo_alloc(void)
static ip4_mfib_t * ip4_mfib_get(u32 index)
Get the FIB at the given index.
u32 fib_node_index_t
A typedef of a node index.
void fib_show_memory_usage(const char *name, u32 in_use_elts, u32 allocd_elts, size_t size_elt)
Show the memory usage for a type.
u32 mpls_lookup_to_replicate_edge
The arc/edge from the MPLS lookup node to the MPLS replicate node.
static void lookup_dpo_mem_show(void)
const static char *const lookup_dst_mcast_ip4_nodes[]
#define MAX_LUKPS_PER_PACKET
If a packet encounters a lookup DPO more than the many times then we assume there is a loop in the fo...
@ LOOKUP_SUB_TYPE_DST_TABLE_FROM_INTERFACE
enum dpo_type_t_ dpo_type_t
Common types of data-path objects New types can be dynamically added using dpo_register_new_type()
const static char *const lookup_dst_from_interface_mpls_nodes[]
static const dpo_id_t * load_balance_get_bucket_i(const load_balance_t *lb, u32 bucket)
static void lookup_dpo_add_or_lock_i(fib_node_index_t fib_index, dpo_proto_t proto, lookup_cast_t cast, lookup_input_t input, lookup_table_t table_config, dpo_id_t *dpo)
void lookup_dpo_module_init(void)
vnet_feature_config_main_t * cm
static u32 mpls_compute_flow_hash(const mpls_unicast_header_t *hdr, flow_hash_config_t flow_hash_config)
const static char *const lookup_src_ip6_nodes[]
vlib_node_registration_t lookup_ip6_dst_node
(constructor) VLIB_REGISTER_NODE (lookup_ip6_dst_node)
#define VLIB_CLI_COMMAND(x,...)
u32 ip6_fib_table_get_index_for_sw_if_index(u32 sw_if_index)
dpo_lock_fn_t dv_lock
A reference counting lock function.
@ LOOKUP_TABLE_FROM_CONFIG
struct lookup_trace_t_ lookup_trace_t
Lookup trace data.
static_always_inline void ip4_fib_forwarding_lookup_x2(u32 fib_index0, u32 fib_index1, const ip4_address_t *addr0, const ip4_address_t *addr1, index_t *lb0, index_t *lb1)
static u8 * format_lookup_mpls_trace(u8 *s, va_list *args)
#define CLIB_CACHE_LINE_BYTES
enum lookup_ip_dst_mcast_next_t_ mfib_forward_lookup_next_t
struct _vlib_node_registration vlib_node_registration_t
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static u32 ip6_fib_table_fwding_lookup(u32 fib_index, const ip6_address_t *dst)
void mfib_table_unlock(u32 fib_index, fib_protocol_t proto, mfib_source_t source)
Take a reference counting lock on the table.
lookup_table_t lkd_table
Switch to use the table index passed, or the table of the input interface.
lookup_cast_t lkd_cast
Unicast of rmulticast FIB lookup.
static const char *const lookup_input_names[]
vlib_node_registration_t lookup_ip4_dst_node
(constructor) VLIB_REGISTER_NODE (lookup_ip4_dst_node)
enum lookup_sub_type_t_ lookup_sub_type_t
Enumeration of the lookup subtypes.
#define pool_len(p)
Number of elements in pool vector.
#define vlib_validate_buffer_enqueue_x1(vm, node, next_index, to_next, n_left_to_next, bi0, next0)
Finish enqueueing one buffer forward in the graph.
vlib_combined_counter_main_t lbm_to_counters
@ LOOKUP_TABLE_FROM_INPUT_INTERFACE
fib_protocol_t dpo_proto_to_fib(dpo_proto_t dpo_proto)
description fragment has unexpected format
A collection of combined counters.
const static char *const lookup_dst_ip4_nodes[]
vlib_put_next_frame(vm, node, next_index, 0)
format_function_t format_ip46_address
void fib_table_lock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Release a reference counting lock on the table.
static dpo_type_t lookup_dpo_sub_types[LOOKUP_SUB_TYPE_NUM]
An array of registered DPO type values for the sub-types.
vlib_node_registration_t lookup_ip4_dst_itf_node
(constructor) VLIB_REGISTER_NODE (lookup_ip4_dst_itf_node)
static load_balance_t * load_balance_get(index_t lbi)
static uword lookup_dpo_mpls_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *from_frame, int table_from_interface)
static void lookup_dpo_lock(dpo_id_t *dpo)
enum lookup_input_t_ lookup_input_t
Switch to use the packet's source or destination address for lookup.
fib_node_index_t ip4_mfib_table_lookup(const ip4_mfib_t *mfib, const ip4_address_t *src, const ip4_address_t *grp, u32 len)
The IPv4 Multicast-FIB.
static uword pool_elts(void *v)
Number of active elements in a pool.
lookup_sub_type_t_
Enumeration of the lookup subtypes.
@ LOOKUP_IP_DST_MCAST_NEXT_DROP
enum lookup_cast_t_ lookup_cast_t
Switch to use the packet's source or destination address for lookup.
static u32 ip4_compute_flow_hash(const ip4_header_t *ip, flow_hash_config_t flow_hash_config)
@ LOOKUP_IP_DST_MCAST_NEXT_RPF
fib_node_index_t ip6_mfib_table_fwd_lookup(const ip6_mfib_t *mfib, const ip6_address_t *src, const ip6_address_t *grp)
const static char *const *const lookup_dst_from_interface_nodes[DPO_PROTO_NUM]
A virtual function table regisitered for a DPO type.
u8 * format_dpo_proto(u8 *s, va_list *args)
format a DPO protocol
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
static index_t mpls_fib_table_forwarding_lookup(u32 mpls_fib_index, const mpls_unicast_header_t *hdr)
Lookup a label and EOS bit in the MPLS_FIB table to retrieve the load-balance index to be used for pa...
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
void lookup_dpo_add_or_lock_w_table_id(u32 table_id, dpo_proto_t proto, lookup_cast_t cast, lookup_input_t input, lookup_table_t table_config, dpo_id_t *dpo)
void fib_table_unlock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Take a reference counting lock on the table.
@ FIB_SOURCE_RR
Recursive resolution source.
const static dpo_vft_t lkd_vft_w_mem_show
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
dpo_proto_t lkd_proto
The protocol of the FIB for the lookup, and hence the protocol of the packet.
vlib_node_registration_t lookup_ip6_dst_mcast_node
(constructor) VLIB_REGISTER_NODE (lookup_ip6_dst_mcast_node)
u16 lb_n_buckets_minus_1
number of buckets in the load-balance - 1.
#define vlib_validate_buffer_enqueue_x2(vm, node, next_index, to_next, n_left_to_next, bi0, bi1, next0, next1)
Finish enqueueing two buffers forward in the graph.
static index_t ip4_fib_forwarding_lookup(u32 fib_index, const ip4_address_t *addr)
fib_node_index_t lkd_fib_index
The FIB, or interface from which to get a FIB, in which to perform the next lookup;.
static_always_inline void clib_prefetch_store(void *p)
void dpo_set(dpo_id_t *dpo, dpo_type_t type, dpo_proto_t proto, index_t index)
Set/create a DPO ID The DPO will be locked.
lookup_input_t lkd_input
Switch to use src or dst address.
u8 * format_mfib_table_name(u8 *s, va_list *ap)
Format the description/name of the table.
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
static uword is_pow2(uword x)
vl_api_interface_index_t sw_if_index
#define vlib_get_next_frame(vm, node, next_index, vectors, n_vectors_left)
Get pointer to next frame vector data by (vlib_node_runtime_t, next_index).
void lookup_dpo_add_or_lock_w_fib_index(fib_node_index_t fib_index, dpo_proto_t proto, lookup_cast_t cast, lookup_input_t input, lookup_table_t table_config, dpo_id_t *dpo)
void dpo_reset(dpo_id_t *dpo)
reset a DPO ID The DPO will be unlocked.
vl_api_fib_path_type_t type
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
const static char *const lookup_dst_mpls_nodes[]
void dpo_register(dpo_type_t type, const dpo_vft_t *vft, const char *const *const *nodes)
For a given DPO type Register:
#define dpo_pool_barrier_release(VM, YESNO)
Release barrier sync after dpo pool expansion.
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)