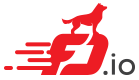 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
22 #include <vpp/app/version.h>
23 #include <linux/limits.h>
24 #include <sys/ioctl.h>
26 #include <perfmon/perfmon.h>
45 seq = mmap_page->lock;
48 idx = mmap_page->index;
49 count = mmap_page->offset;
51 count += _rdpmc (idx - 1);
55 while (mmap_page->lock != seq);
62 struct perf_event_mmap_page **mmap_pages,
96 u8 n_events =
rt->n_events;
114 for (
int i = 0;
i < n_events;
i++)
115 s->
value[
i] += after[
i] - before[
i];
127 counters[6] = _rdpmc (pmc_index[6]);
129 counters[5] = _rdpmc (pmc_index[5]);
131 counters[4] = _rdpmc (pmc_index[4]);
133 counters[3] = _rdpmc (pmc_index[3]);
135 counters[2] = _rdpmc (pmc_index[2]);
137 counters[1] = _rdpmc (pmc_index[1]);
139 counters[0] = _rdpmc (pmc_index[0]);
147 return (
int) (
b->metrics[
i]);
160 u8 n_events =
rt->n_events;
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
perfmon_main_t perfmon_main
vlib_main_t vlib_node_runtime_t * node
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
perfmon_thread_runtime_t * thread_runtimes
struct perfmon_node_stats_t::@769::@771 t[2]
#define CLIB_COMPILER_BARRIER()
static_always_inline int perfmon_metric_index(perfmon_bundle_t *b, u8 i)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
#define static_always_inline
uword perfmon_dispatch_wrapper_metrics(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
static_always_inline void clib_prefetch_load(void *p)
static_always_inline void perfmon_mmap_read_pmcs(u64 *counters, struct perf_event_mmap_page **mmap_pages, u8 n_counters)
uword perfmon_dispatch_wrapper_mmap(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
vnet_interface_output_runtime_t * rt
static_always_inline void perfmon_metric_read_pmcs(u64 *counters, int *pmc_index, u8 n_counters)
u64 value[PERF_MAX_EVENTS]
static_always_inline u64 perfmon_mmap_read_pmc1(const struct perf_event_mmap_page *mmap_page)