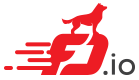 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
21 #include <vpp/app/version.h>
22 #include <linux/limits.h>
23 #include <sys/ioctl.h>
25 #include <perfmon/perfmon.h>
30 .version = VPP_BUILD_VER,
31 .description =
"Performance Monitor",
35 .class_name =
"perfmon",
38 #define log_debug(fmt, ...) \
39 vlib_log_debug (if_default_log.class, fmt, __VA_ARGS__)
40 #define log_warn(fmt, ...) \
41 vlib_log_warn (if_default_log.class, fmt, __VA_ARGS__)
42 #define log_err(fmt, ...) vlib_log_err (if_default_log.class, fmt, __VA_ARGS__)
91 u32 instance_type = 0;
106 it->name = is_node ?
"Thread/Node" :
"Thread";
126 for (
int i = 1;
i <
b->n_events;
i++)
145 for (
int j = 0; j <
b->n_events; j++)
149 struct perf_event_attr
pe = {
150 .size =
sizeof (
struct perf_event_attr),
155 (PERF_FORMAT_GROUP | PERF_FORMAT_TOTAL_TIME_ENABLED |
156 PERF_FORMAT_TOTAL_TIME_RUNNING),
160 log_debug (
"perf_event_open pe.type=%u pe.config=0x%x pid=%d "
161 "cpu=%d group_fd=%d",
163 fd = syscall (__NR_perf_event_open, &
pe, in->
pid, in->
cpu,
182 mmap (0, page_size, PROT_READ, MAP_SHARED, fd, 0);
197 rt->n_events =
b->n_events;
198 rt->n_nodes = n_nodes;
230 for (
int i = 0;
i < n_groups;
i++)
232 if (ioctl (pm->
group_fds[
i], PERF_EVENT_IOC_ENABLE,
233 PERF_IOC_FLAG_GROUP) == -1)
243 #define _(type, pfunc) funcs[type] = pfunc;
248 ASSERT (funcs[
b->offset_type]);
252 funcs[
b->offset_type]);
276 for (
int i = 0;
i < n_groups;
i++)
278 if (ioctl (pm->
group_fds[
i], PERF_EVENT_IOC_DISABLE,
279 PERF_IOC_FLAG_GROUP) == -1)
324 clib_panic (
"duplicate bundle name '%s'",
b->name);
328 log_debug (
"missing source '%s', skipping bundle '%s'",
b->source,
335 if (
b->init_fn && ((err = (
b->init_fn) (
vm,
b))))
345 log_debug (
"bundle '%s' regisrtered",
b->name);
@ PERFMON_BUNDLE_TYPE_NODE
perfmon_bundle_type_t type
void perfmon_reset(vlib_main_t *vm)
perfmon_bundle_t * active_bundle
perfmon_main_t perfmon_main
#define hash_create_string(elts, value_bytes)
#define clib_error_return(e, args...)
perfmon_bundle_t * bundles
vlib_node_main_t node_main
#define hash_set_mem(h, key, value)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
perfmon_instance_type_t * instances_by_type
uword() vlib_node_function_t(struct vlib_main_t *vm, struct vlib_node_runtime_t *node, struct vlib_frame_t *frame)
perfmon_instance_type_t * active_instance_type
vlib_worker_thread_t * vlib_worker_threads
perfmon_thread_runtime_t * thread_runtimes
VLIB_REGISTER_LOG_CLASS(if_default_log, static)
perfmon_source_init_fn_t * init_fn
__clib_export u8 * format_clib_error(u8 *s, va_list *va)
struct perfmon_source * next
perfmon_source_t * sources
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
#define vec_validate_aligned(V, I, A)
Make sure vector is long enough for given index (no header, specified alignment)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
save_rewrite_length must be aligned so that reass doesn t overwrite it
#define CLIB_CACHE_LINE_BYTES
uword pe(void *v)
GDB callable function: pe - call pool_elts - number of elements in a pool.
static clib_error_t * perfmon_init(vlib_main_t *vm)
struct perf_event_mmap_page * mmap_pages[PERF_MAX_EVENTS]
@ PERFMON_OFFSET_TYPE_MAX
static int vlib_node_set_dispatch_wrapper(vlib_main_t *vm, vlib_node_function_t *fn)
#define hash_get_mem(h, key)
#define vec_free(V)
Free vector's memory (no header).
description fragment has unexpected format
#define VLIB_INIT_FUNCTION(x)
perfmon_instance_type_t * default_instance_type
#define foreach_permon_offset_type
static_always_inline uword clib_mem_get_page_size(void)
static vlib_main_t * vlib_get_main_by_index(u32 thread_index)
#define clib_error_return_unix(e, args...)
static u32 vlib_get_n_threads()
static clib_error_t * perfmon_set(vlib_main_t *vm, perfmon_bundle_t *b)
vnet_interface_output_runtime_t * rt
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
perfmon_node_stats_t * node_stats
#define log_warn(fmt,...)
clib_error_t * perfmon_stop(vlib_main_t *vm)
#define clib_error_free(e)
clib_error_t * perfmon_start(vlib_main_t *vm, perfmon_bundle_t *b)
static f64 vlib_time_now(vlib_main_t *vm)
#define clib_panic(format, args...)
vl_api_fib_path_type_t type
#define log_debug(fmt,...)