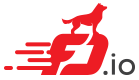 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
15 #ifndef __IPSEC_SPD_SA_H__
16 #define __IPSEC_SPD_SA_H__
24 #define foreach_ipsec_crypto_alg \
26 _ (1, AES_CBC_128, "aes-cbc-128") \
27 _ (2, AES_CBC_192, "aes-cbc-192") \
28 _ (3, AES_CBC_256, "aes-cbc-256") \
29 _ (4, AES_CTR_128, "aes-ctr-128") \
30 _ (5, AES_CTR_192, "aes-ctr-192") \
31 _ (6, AES_CTR_256, "aes-ctr-256") \
32 _ (7, AES_GCM_128, "aes-gcm-128") \
33 _ (8, AES_GCM_192, "aes-gcm-192") \
34 _ (9, AES_GCM_256, "aes-gcm-256") \
35 _ (10, DES_CBC, "des-cbc") \
36 _ (11, 3DES_CBC, "3des-cbc")
40 #define _(v, f, s) IPSEC_CRYPTO_ALG_##f = v,
44 } __clib_packed ipsec_crypto_alg_t;
46 #define IPSEC_CRYPTO_ALG_IS_GCM(_alg) \
47 (((_alg == IPSEC_CRYPTO_ALG_AES_GCM_128) || \
48 (_alg == IPSEC_CRYPTO_ALG_AES_GCM_192) || \
49 (_alg == IPSEC_CRYPTO_ALG_AES_GCM_256)))
51 #define IPSEC_CRYPTO_ALG_IS_CTR(_alg) \
52 (((_alg == IPSEC_CRYPTO_ALG_AES_CTR_128) || \
53 (_alg == IPSEC_CRYPTO_ALG_AES_CTR_192) || \
54 (_alg == IPSEC_CRYPTO_ALG_AES_CTR_256)))
56 #define foreach_ipsec_integ_alg \
58 _ (1, MD5_96, "md5-96") \
59 _ (2, SHA1_96, "sha1-96") \
60 _ (3, SHA_256_96, "sha-256-96") \
61 _ (4, SHA_256_128, "sha-256-128") \
62 _ (5, SHA_384_192, "sha-384-192") \
63 _ (6, SHA_512_256, "sha-512-256")
67 #define _(v, f, s) IPSEC_INTEG_ALG_##f = v,
71 } __clib_packed ipsec_integ_alg_t;
77 } __clib_packed ipsec_protocol_t;
79 #define IPSEC_KEY_MAX_LEN 128
94 #define foreach_ipsec_sa_flags \
96 _ (1, USE_ESN, "esn") \
97 _ (2, USE_ANTI_REPLAY, "anti-replay") \
98 _ (4, IS_TUNNEL, "tunnel") \
99 _ (8, IS_TUNNEL_V6, "tunnel-v6") \
100 _ (16, UDP_ENCAP, "udp-encap") \
101 _ (32, IS_PROTECT, "Protect") \
102 _ (64, IS_INBOUND, "inbound") \
103 _ (128, IS_AEAD, "aead") \
104 _ (256, IS_CTR, "ctr") \
105 _ (512, IS_ASYNC, "async")
109 #define _(v, f, s) IPSEC_SA_FLAG_##f = v,
236 "IPSec data is overlapping with IP data");
240 ipsec_sa_is_set_##v (const ipsec_sa_t *sa) { \
241 return (sa->flags & IPSEC_SA_FLAG_##v); \
247 ipsec_sa_set_##v (ipsec_sa_t *sa) { \
248 return (sa->flags |= IPSEC_SA_FLAG_##v); \
254 ipsec_sa_unset_##v (ipsec_sa_t *sa) { \
255 return (sa->flags &= ~IPSEC_SA_FLAG_##v); \
269 ipsec_crypto_alg_t crypto_alg,
const ipsec_key_t *ck,
279 ipsec_crypto_alg_t crypto_alg);
297 #define IPSEC_UDP_PORT_NONE ((u16)~0)
303 #define IPSEC_SA_ANTI_REPLAY_WINDOW_SIZE (64)
304 #define IPSEC_SA_ANTI_REPLAY_WINDOW_MAX_INDEX (IPSEC_SA_ANTI_REPLAY_WINDOW_SIZE-1)
311 #define IPSEC_SA_ANTI_REPLAY_WINDOW_LOWER_BOUND(_tl) (_tl - IPSEC_SA_ANTI_REPLAY_WINDOW_SIZE + 1)
316 if (ipsec_sa_is_set_USE_ANTI_REPLAY (sa) &&
339 u32 hi_seq_used,
bool post_decrypt,
342 ASSERT ((post_decrypt ==
false) == (hi_seq_req != 0));
344 if (!ipsec_sa_is_set_USE_ESN (sa))
350 if (!ipsec_sa_is_set_USE_ANTI_REPLAY (sa))
366 if (!ipsec_sa_is_set_USE_ANTI_REPLAY (sa))
390 if (sa->
seq - seq > (1 << 30))
392 *hi_seq_req = sa->
seq_hi + 1;
420 if (hi_seq_used == sa->
seq_hi)
437 *hi_seq_req = sa->
seq_hi + 1;
515 *hi_seq_req = sa->
seq_hi - 1;
539 if (ipsec_sa_is_set_USE_ESN (sa))
541 int wrap = hi_seq - sa->
seq_hi;
543 if (wrap == 0 && seq > sa->
seq)
554 pos = ~seq + sa->
seq + 1;
564 pos = ~seq + sa->
seq + 1;
600 return ((thread_id) ? thread_id
ipsec_sa_t * ipsec_sa_pool
Pool of IPSec SAs.
void ipsec_sa_set_integ_alg(ipsec_sa_t *sa, ipsec_integ_alg_t integ_alg)
static u32 vlib_num_workers()
vnet_crypto_key_index_t integ_key_index
ipsec_protocol_t protocol
enum tunnel_encap_decap_flags_t_ tunnel_encap_decap_flags_t
vl_api_ip_port_and_mask_t dst_port
static int ipsec_sa_anti_replay_and_sn_advance(const ipsec_sa_t *sa, u32 seq, u32 hi_seq_used, bool post_decrypt, u32 *hi_seq_req)
index_t ipsec_sa_find_and_lock(u32 id)
int ipsec_sa_add_and_lock(u32 id, u32 spi, ipsec_protocol_t proto, ipsec_crypto_alg_t crypto_alg, const ipsec_key_t *ck, ipsec_integ_alg_t integ_alg, const ipsec_key_t *ik, ipsec_sa_flags_t flags, u32 salt, u16 src_port, u16 dst_port, const tunnel_t *tun, u32 *sa_out_index)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
A representation of an IP tunnel config.
#define CLIB_CACHE_LINE_ALIGN_MARK(mark)
uword unformat_ipsec_key(unformat_input_t *input, va_list *args)
u32 vnet_crypto_key_index_t
#define IPSEC_SA_ANTI_REPLAY_WINDOW_MAX_INDEX
vnet_crypto_op_id_t crypto_dec_op_id
static int ipsec_sa_anti_replay_check(const ipsec_sa_t *sa, u32 seq)
u8 data[IPSEC_KEY_MAX_LEN]
STATIC_ASSERT(sizeof(ipsec_sa_flags_t)==2, "IPSEC SA flags != 2 byte")
void ipsec_sa_walk(ipsec_sa_walk_cb_t cd, void *ctx)
#define STRUCT_OFFSET_OF(t, f)
int ipsec_sa_unlock_id(u32 id)
enum ipsec_sad_flags_t_ ipsec_sa_flags_t
vnet_crypto_async_op_id_t crypto_async_dec_op_id
void ipsec_sa_clear(index_t sai)
u8 * format_ipsec_crypto_alg(u8 *s, va_list *args)
#define foreach_ipsec_integ_alg
u8 * format_ipsec_integ_alg(u8 *s, va_list *args)
static ipsec_sa_t * ipsec_sa_get(u32 sa_index)
#define IPSEC_SA_ANTI_REPLAY_WINDOW_SIZE
void ipsec_sa_lock(index_t sai)
walk_rc_t(* ipsec_sa_walk_cb_t)(ipsec_sa_t *sa, void *ctx)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
tunnel_encap_decap_flags_t tunnel_flags
static u64 unix_time_now_nsec(void)
vnet_crypto_alg_t crypto_calg
vl_api_ip_port_and_mask_t src_port
#define foreach_ipsec_sa_flags
uword unformat_ipsec_integ_alg(unformat_input_t *input, va_list *args)
static u32 ipsec_sa_assign_thread(u32 thread_id)
#define CLIB_CACHE_LINE_BYTES
ipsec_crypto_alg_t crypto_alg
vnet_crypto_async_op_id_t crypto_async_enc_op_id
#define IPSEC_KEY_MAX_LEN
void ipsec_sa_unlock(index_t sai)
uword unformat_ipsec_crypto_alg(unformat_input_t *input, va_list *args)
void ipsec_sa_set_crypto_alg(ipsec_sa_t *sa, ipsec_crypto_alg_t crypto_alg)
A collection of combined counters.
vnet_crypto_op_id_t integ_op_id
vnet_crypto_key_index_t linked_key_index
void ipsec_mk_key(ipsec_key_t *key, const u8 *data, u8 len)
An node in the FIB graph.
foreach_ipsec_sa_flags vlib_combined_counter_main_t ipsec_sa_counters
SA packet & bytes counters.
vnet_crypto_async_op_id_t
ipsec_integ_alg_t integ_alg
u8 * format_ipsec_replay_window(u8 *s, va_list *args)
#define IPSEC_SA_ANTI_REPLAY_WINDOW_LOWER_BOUND(_tl)
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
vnet_crypto_alg_t integ_calg
u8 * format_ipsec_sa(u8 *s, va_list *args)
STATIC_ASSERT_OFFSET_OF(ipsec_sa_t, cacheline1, CLIB_CACHE_LINE_BYTES)
#define foreach_ipsec_crypto_alg
u8 * format_ipsec_key(u8 *s, va_list *args)
vl_api_gbp_endpoint_tun_t tun
enum walk_rc_t_ walk_rc_t
Walk return code.
vnet_crypto_op_id_t crypto_enc_op_id
vnet_crypto_key_index_t crypto_key_index
struct ipsec_key_t_ ipsec_key_t
static void ipsec_sa_anti_replay_advance(ipsec_sa_t *sa, u32 seq, u32 hi_seq)
vl_api_wireguard_peer_flags_t flags