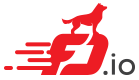 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
29 .stat_segment_name =
"/net/ipsec/sa",
36 u32 sa_index,
int is_add)
59 memset (
key, 0,
sizeof (*
key));
61 if (
len >
sizeof (
key->data))
62 key->len =
sizeof (
key->data);
82 im->ah6_encrypt_node_index :
83 im->ah4_encrypt_node_index), &sa->
dpo, &
tmp);
86 im->esp6_encrypt_node_index :
87 im->esp4_encrypt_node_index), &sa->
dpo, &
tmp);
106 ipsec_sa_set_IS_CTR (sa);
107 ipsec_sa_set_IS_AEAD (sa);
111 ipsec_sa_set_IS_CTR (sa);
130 if (ipsec_sa_is_set_USE_ESN (sa))
133 if( sa->sync_op_data.crypto_enc_op_id == VNET_CRYPTO_OP_##n##_ENC ) \
134 sa->async_op_data.crypto_async_enc_op_id = \
135 VNET_CRYPTO_OP_##n##_TAG16_AAD12_ENC; \
136 if( sa->sync_op_data.crypto_dec_op_id == VNET_CRYPTO_OP_##n##_DEC ) \
137 sa->async_op_data.crypto_async_dec_op_id = \
138 VNET_CRYPTO_OP_##n##_TAG16_AAD12_DEC;
145 if( sa->sync_op_data.crypto_enc_op_id == VNET_CRYPTO_OP_##n##_ENC ) \
146 sa->async_op_data.crypto_async_enc_op_id = \
147 VNET_CRYPTO_OP_##n##_TAG16_AAD8_ENC; \
148 if( sa->sync_op_data.crypto_dec_op_id == VNET_CRYPTO_OP_##n##_DEC ) \
149 sa->async_op_data.crypto_async_dec_op_id = \
150 VNET_CRYPTO_OP_##n##_TAG16_AAD8_DEC;
155 #define _(c, h, s, k ,d) \
156 if( sa->sync_op_data.crypto_enc_op_id == VNET_CRYPTO_OP_##c##_ENC && \
157 sa->sync_op_data.integ_op_id == VNET_CRYPTO_OP_##h##_HMAC) \
158 sa->async_op_data.crypto_async_enc_op_id = \
159 VNET_CRYPTO_OP_##c##_##h##_TAG##d##_ENC; \
160 if( sa->sync_op_data.crypto_dec_op_id == VNET_CRYPTO_OP_##c##_DEC && \
161 sa->sync_op_data.integ_op_id == VNET_CRYPTO_OP_##h##_HMAC) \
162 sa->async_op_data.crypto_async_dec_op_id = \
163 VNET_CRYPTO_OP_##c##_##h##_TAG##d##_DEC;
171 ipsec_crypto_alg_t crypto_alg,
const ipsec_key_t *ck,
186 return VNET_API_ERROR_ENTRY_ALREADY_EXISTS;
216 im->crypto_algs[crypto_alg].alg,
221 return VNET_API_ERROR_KEY_LENGTH;
233 return VNET_API_ERROR_KEY_LENGTH;
238 !ipsec_sa_is_set_IS_AEAD (sa))
249 if (ipsec_sa_is_set_IS_ASYNC (sa))
263 return VNET_API_ERROR_UNIMPLEMENTED;
270 return VNET_API_ERROR_SYSCALL_ERROR_1;
273 if (ipsec_sa_is_set_IS_TUNNEL (sa) &&
275 ipsec_sa_set_IS_TUNNEL_V6 (sa);
277 if (ipsec_sa_is_set_IS_TUNNEL (sa) && !ipsec_sa_is_set_IS_INBOUND (sa))
291 if (ipsec_sa_is_set_IS_TUNNEL_V6 (sa))
294 (ipsec_sa_is_set_UDP_ENCAP (sa) ?
296 IP_PROTOCOL_IPSEC_ESP),
302 (ipsec_sa_is_set_UDP_ENCAP (sa) ?
304 IP_PROTOCOL_IPSEC_ESP),
309 if (ipsec_sa_is_set_UDP_ENCAP (sa))
321 if (ipsec_sa_is_set_IS_INBOUND (sa))
328 *sa_out_index = sa_index;
347 if (ipsec_sa_is_set_IS_ASYNC (sa))
349 if (ipsec_sa_is_set_UDP_ENCAP (sa) && ipsec_sa_is_set_IS_INBOUND (sa))
352 if (ipsec_sa_is_set_IS_TUNNEL (sa) && !ipsec_sa_is_set_IS_INBOUND (sa))
355 if (sa->
integ_alg != IPSEC_INTEG_ALG_NONE)
414 return VNET_API_ERROR_NO_SUCH_ENTRY;
vnet_interface_main_t * im
static u32 vlib_num_workers()
vnet_crypto_key_index_t integ_key_index
ipsec_protocol_t protocol
#define DPO_INVALID
An initialiser for DPOs declared on the stack.
#define hash_set(h, key, value)
vl_api_ip_port_and_mask_t dst_port
enum fib_node_back_walk_rc_t_ fib_node_back_walk_rc_t
Return code from a back walk function.
#define clib_memcpy(d, s, n)
void vnet_crypto_key_del(vlib_main_t *vm, vnet_crypto_key_index_t index)
#define pool_get_aligned_zero(P, E, A)
Allocate an object E from a pool P with alignment A and zero it.
#define foreach_crypto_link_async_alg
clib_error_t * ipsec_sa_interface_init(vlib_main_t *vm)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
A representation of an IP tunnel config.
vlib_main_t vlib_node_runtime_t * node
A FIB graph nodes virtual function table.
void vlib_validate_combined_counter(vlib_combined_counter_main_t *cm, u32 index)
validate a combined counter
#define pool_put(P, E)
Free an object E in pool P.
index_t ipsec_sa_find_and_lock(u32 id)
void tunnel_contribute_forwarding(const tunnel_t *t, dpo_id_t *dpo)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
char * name
The counter collection's name.
static void ipsec_sa_last_lock_gone(fib_node_t *node)
Function definition to inform the FIB node that its last lock has gone.
void ipsec_sa_walk(ipsec_sa_walk_cb_t cb, void *ctx)
void tunnel_copy(const tunnel_t *src, tunnel_t *dst)
vnet_crypto_op_id_t crypto_dec_op_id
static ipsec_sa_t * ipsec_sa_from_fib_node(fib_node_t *node)
void ipsec_sa_set_crypto_alg(ipsec_sa_t *sa, ipsec_crypto_alg_t crypto_alg)
#define hash_unset(h, key)
void ipsec_unregister_udp_port(u16 port)
clib_error_t * ipsec_check_support_cb(ipsec_main_t *im, ipsec_sa_t *sa)
u8 data[IPSEC_KEY_MAX_LEN]
static fib_node_t * ipsec_sa_fib_node_get(fib_node_index_t index)
Function definition to get a FIB node from its index.
#define STRUCT_OFFSET_OF(t, f)
#define IPSEC_UDP_PORT_NONE
void tunnel_build_v4_hdr(const tunnel_t *t, ip_protocol_t next_proto, ip4_header_t *ip)
#define ip_addr_version(_a)
#define pool_foreach(VAR, POOL)
Iterate through pool.
enum ipsec_sad_flags_t_ ipsec_sa_flags_t
static fib_node_back_walk_rc_t ipsec_sa_back_walk(fib_node_t *node, fib_node_back_walk_ctx_t *ctx)
Function definition to backwalk a FIB node.
static void ipsec_sa_stack(ipsec_sa_t *sa)
'stack' (resolve the recursion for) the SA tunnel destination
static ipsec_sa_t * ipsec_sa_get(u32 sa_index)
void ipsec_sa_clear(index_t sai)
u32 vnet_crypto_key_add_linked(vlib_main_t *vm, vnet_crypto_key_index_t index_crypto, vnet_crypto_key_index_t index_integ)
Use 2 created keys to generate new key for linked algs (cipher + integ) The returned key index is to ...
vlib_combined_counter_main_t ipsec_sa_counters
SA packet & bytes counters.
walk_rc_t(* ipsec_sa_walk_cb_t)(ipsec_sa_t *sa, void *ctx)
#define foreach_crypto_aead_alg
static clib_error_t * ipsec_call_add_del_callbacks(ipsec_main_t *im, ipsec_sa_t *sa, u32 sa_index, int is_add)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
tunnel_encap_decap_flags_t tunnel_flags
void ipsec_sa_set_async_op_ids(ipsec_sa_t *sa)
#define IPSEC_CRYPTO_ALG_IS_CTR(_alg)
const static fib_node_vft_t ipsec_sa_vft
u32 fib_node_index_t
A typedef of a node index.
static void vlib_zero_combined_counter(vlib_combined_counter_main_t *cm, u32 index)
Clear a combined counter Clears the set of per-thread counters.
vnet_crypto_alg_t crypto_calg
vl_api_ip_port_and_mask_t src_port
void ipsec_sa_unlock(index_t sai)
void vnet_crypto_request_async_mode(int is_enable)
void ipsec_register_udp_port(u16 port)
void dpo_stack_from_node(u32 child_node_index, dpo_id_t *dpo, const dpo_id_t *parent)
Stack one DPO object on another, and thus establish a child parent relationship.
add_del_sa_sess_cb_t add_del_sa_sess_cb
void fib_node_register_type(fib_node_type_t type, const fib_node_vft_t *vft)
fib_node_register_type
void ipsec_sa_lock(index_t sai)
#define CLIB_CACHE_LINE_BYTES
@ FIB_NODE_BACK_WALK_CONTINUE
ipsec_crypto_alg_t crypto_alg
vnet_crypto_async_op_id_t crypto_async_enc_op_id
#define ESP_MAX_BLOCK_SIZE
u32 vnet_crypto_key_add(vlib_main_t *vm, vnet_crypto_alg_t alg, u8 *data, u16 length)
void ipsec_mk_key(ipsec_key_t *key, const u8 *data, u8 len)
A collection of combined counters.
void tunnel_build_v6_hdr(const tunnel_t *t, ip_protocol_t next_proto, ip6_header_t *ip)
add_del_sa_sess_cb_t add_del_sa_sess_cb
#define VLIB_INIT_FUNCTION(x)
int tunnel_resolve(tunnel_t *t, fib_node_type_t child_type, index_t child_index)
vnet_crypto_op_id_t integ_op_id
void fib_node_lock(fib_node_t *node)
vnet_crypto_key_index_t linked_key_index
An node in the FIB graph.
void fib_node_init(fib_node_t *node, fib_node_type_t type)
static vlib_main_t * vlib_get_main(void)
void fib_node_unlock(fib_node_t *node)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
tunnel_encap_decap_flags_t t_encap_decap_flags
Context passed between object during a back walk.
ipsec_integ_alg_t integ_alg
#define clib_warning(format, args...)
union ipsec_sa_t::@451 sync_op_data
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
vnet_crypto_alg_t integ_calg
union ipsec_sa_t::@452 async_op_data
int ipsec_sa_add_and_lock(u32 id, u32 spi, ipsec_protocol_t proto, ipsec_crypto_alg_t crypto_alg, const ipsec_key_t *ck, ipsec_integ_alg_t integ_alg, const ipsec_key_t *ik, ipsec_sa_flags_t flags, u32 salt, u16 src_port, u16 dst_port, const tunnel_t *tun, u32 *sa_out_index)
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
vl_api_gbp_endpoint_tun_t tun
void dpo_reset(dpo_id_t *dpo)
reset a DPO ID The DPO will be unlocked.
int ipsec_sa_unlock_id(u32 id)
vnet_crypto_op_id_t crypto_enc_op_id
ipsec_sa_t * ipsec_sa_pool
Pool of IPSec SAs.
void tunnel_unresolve(tunnel_t *t)
#define IPSEC_CRYPTO_ALG_IS_GCM(_alg)
void ipsec_sa_set_integ_alg(ipsec_sa_t *sa, ipsec_integ_alg_t integ_alg)
vnet_crypto_key_index_t crypto_key_index
static void ipsec_sa_del(ipsec_sa_t *sa)
vl_api_wireguard_peer_flags_t flags