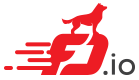 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
52 #define FOREACH_IP6_LINK_DELEGATE(_ild, _il, body) \
55 vec_foreach (_ild, _il->il_delegates) { \
56 if (ip6_link_delegate_is_init(_ild)) \
62 #define FOREACH_IP6_LINK_DELEGATE_ID(_id) \
63 for (_id = 0; _id < il_delegate_id; _id++)
80 #define IP6_LINK_DBG(...) \
81 vlib_log_debug (ip6_link_logger, __VA_ARGS__);
83 #define IP6_LINK_INFO(...) \
84 vlib_log_notice (ip6_link_logger, __VA_ARGS__);
101 ip->as_u64[0] = clib_host_to_net_u64 (0xFE80000000000000ULL);
103 ip->as_u8[8] =
mac[0] ^ (1 << 1);
104 ip->as_u8[9] =
mac[1];
105 ip->as_u8[10] =
mac[2];
106 ip->as_u8[11] = 0xFF;
107 ip->as_u8[12] = 0xFE;
108 ip->as_u8[13] =
mac[3];
109 ip->as_u8[14] =
mac[4];
110 ip->as_u8[15] =
mac[5];
117 mac[0] =
ip->as_u8[8] ^ (1 << 1);
118 mac[1] =
ip->as_u8[9];
119 mac[2] =
ip->as_u8[10];
120 mac[3] =
ip->as_u8[13];
121 mac[4] =
ip->as_u8[14];
122 mac[5] =
ip->as_u8[15];
180 if (NULL != link_local_addr)
192 clib_host_to_net_u64 (0xFE80000000000000ULL);
232 rv = VNET_API_ERROR_VALUE_EXIST;
299 return (VNET_API_ERROR_IP6_NOT_ENABLED);
309 const ip6_address_t *
449 u32 if_address_index,
u32 is_delete)
459 (is_delete ?
"del" :
"add"),
533 ip6_address_t _a, *
a = &_a;
561 .path =
"test ip6 link",
563 .short_help =
"test ip6 link <mac-address>",
579 s =
format (s,
"%U%U/%d\n",
597 s =
format (s,
"%U is admin %s\n",
604 u32 *link_scope = 0, *global_scope = 0;
605 u32 *local_scope = 0, *unknown_scope = 0;
613 while (ai != (
u32) ~ 0)
627 ai =
a->next_this_sw_interface;
646 s =
format (s,
"%UGlobal unicast address(es):\n",
745 .path =
"show ip6 interface",
747 .short_help =
"show ip6 interface <interface>",
785 .path =
"enable ip6 interface",
787 .short_help =
"enable ip6 interface <interface>",
825 .path =
"disable ip6 interface",
827 .short_help =
"disable ip6 interface <interface>",
static bool ip6_link_delegate_is_init(const ip6_link_delegate_t *ild)
static uword ip6_address_is_link_local_unicast(const ip6_address_t *a)
static clib_error_t * test_ip6_link_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
vnet_interface_main_t * im
uword unformat_ethernet_address(unformat_input_t *input, va_list *args)
static void * ip_interface_address_get_address(ip_lookup_main_t *lm, ip_interface_address_t *a)
index_t ip6_link_delegate_get(u32 sw_if_index, ip6_link_delegate_id_t id)
vnet_sw_interface_type_t type
static void ip6_mac_address_from_link_local(u8 *mac, const ip6_address_t *ip)
static uword ip6_address_is_global_unicast(const ip6_address_t *a)
static vlib_cli_command_t ip6_link_show_command
(constructor) VLIB_CLI_COMMAND (ip6_link_show_command)
static clib_error_t * ip6_link_show(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define clib_memcpy(d, s, n)
static vlib_cli_command_t enable_ip6_interface_command
(constructor) VLIB_CLI_COMMAND (enable_ip6_interface_command)
#define ADJ_INDEX_INVALID
Invalid ADJ index - used when no adj is known likewise blazoned capitals INVALID speak volumes where ...
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
u8 * format_ethernet_address(u8 *s, va_list *args)
static uword ip6_address_is_local_unicast(const ip6_address_t *a)
vlib_log_class_t vlib_log_register_class(char *class, char *subclass)
static void ip6_link_delegate_flush(ip6_link_t *il)
adj_index_t adj_mcast_add_or_lock(fib_protocol_t proto, vnet_link_t link_type, u32 sw_if_index)
Mcast Adjacency.
#define clib_error_return(e, args...)
adj_index_t il_mcast_adj
multicast adjacency for this link
void adj_unlock(adj_index_t adj_index)
Release a reference counting lock on the adjacency.
ip6_link_disable_fn_t ildv_disable
bool ip6_link_delegate_update(u32 sw_if_index, ip6_link_delegate_id_t id, index_t ii)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static vnet_sw_interface_t * vnet_get_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
static void ip6_link_last_lock_gone(ip6_link_t *il)
void ip6_ll_table_entry_delete(const ip6_ll_prefix_t *ilp)
Delete a IP6 link-local entry.
static ip6_link_delegate_id_t il_delegate_id
last used delegate ID
fib_node_index_t ip6_ll_table_entry_update(const ip6_ll_prefix_t *ilp, fib_route_path_flags_t flags)
Update an entry in the table.
ip6_link_delegate_id_t ip6_link_delegate_register(const ip6_link_delegate_vft_t *vft)
static clib_error_t * ip6_link_init(vlib_main_t *vm)
static vlib_log_class_t ip6_link_logger
Logging.
static void ip6_link_local_address_from_mac(ip6_address_t *ip, const u8 *mac)
struct ip6_link_t_ ip6_link_t
u32 il_sw_if_index
interface ip6 is enabled on
static ip6_link_delegate_vft_t * il_delegate_vfts
VFT registered per-delegate type.
u32 * if_address_pool_index_by_sw_if_index
Head of doubly linked list of interface addresses for each software interface.
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
ip6_link_address_change_fn_t ildv_addr_add
void ip6_mfib_interface_enable_disable(u32 sw_if_index, int is_enable)
Add/remove the interface from the accepting list of the special MFIB entries.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
const ip6_address_t * ip6_get_link_local_address(u32 sw_if_index)
vnet_main_t * vnet_get_main(void)
ip6_link_ll_change_fn_t ildv_ll_change
u32 ip6_link_delegate_id_t
ip6_link_delegate_id_t ild_type
ip6_add_del_interface_address_callback_t * add_del_interface_address_callbacks
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
ip6_link_enable_fn_t ildv_enable
format_function_t * ildv_format
static clib_error_t * ip6_link_interface_add_del(vnet_main_t *vnm, u32 sw_if_index, u32 is_add)
#define IP6_LINK_INFO(...)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
manual_print typedef address
#define VLIB_CLI_COMMAND(x,...)
const static ip6_link_delegate_t ip6_link_delegate_uninit
Aggregate type for a prefix in the IPv6 Link-local table.
static void ip6_address_copy(ip6_address_t *dst, const ip6_address_t *src)
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static uword vnet_sw_interface_is_admin_up(vnet_main_t *vnm, u32 sw_if_index)
ethernet_interface_t * ethernet_get_interface(ethernet_main_t *em, u32 hw_if_index)
static bool ip6_link_is_enabled_i(const ip6_link_t *il)
ip6_link_address_change_fn_t ildv_addr_del
bool ip6_link_is_enabled(u32 sw_if_index)
#define vec_free(V)
Free vector's memory (no header).
ip6_add_del_interface_address_function_t * function
static ip6_link_t * ip6_link_get(u32 sw_if_index)
struct ip6_link_delegate_t_ ip6_link_delegate_t
ethernet_main_t ethernet_main
ip6_address_t il_ll_addr
link-local address - if unset that IP6 is disabled
format_function_t format_vnet_sw_if_index_name
unformat_function_t unformat_vnet_sw_interface
static u8 * format_ip6_link(u8 *s, va_list *arg)
static vlib_cli_command_t test_link_command
(constructor) VLIB_CLI_COMMAND (test_link_command)
description fragment has unexpected format
static void ip6_link_add_del_address(ip6_main_t *im, uword opaque, u32 sw_if_index, ip6_address_t *address, u32 address_length, u32 if_address_index, u32 is_delete)
#define vec_validate_init_empty(V, I, INIT)
Make sure vector is long enough for given index and initialize empty space (no header,...
void ip6_link_delegate_remove(u32 sw_if_index, ip6_link_delegate_id_t id, index_t ii)
#define VLIB_INIT_FUNCTION(x)
ip6_link_delegate_t * il_delegates
list of delegates
static u8 * ip6_print_addrs(u8 *s, u32 *addrs)
static void ip6_address_set_zero(ip6_address_t *a)
#define vec_foreach(var, vec)
Vector iterator.
void ip6_sw_interface_enable_disable(u32 sw_if_index, u32 is_enable)
ip_lookup_main_t lookup_main
static u64 random_u64(u64 *seed)
64-bit random number generator Again, constants courtesy of Donald Knuth.
static clib_error_t * disable_ip6_interface_cmd(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
ip_interface_address_t * if_address_pool
Pool of addresses that are assigned to interfaces.
u32 il_locks
number of references to IP6 enabled on this link
u32 adj_index_t
An index for adjacencies.
static ip6_link_t * ip6_links
Per interface configs.
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
adj_index_t ip6_link_get_mcast_adj(u32 sw_if_index)
static vlib_cli_command_t disable_ip6_interface_command
(constructor) VLIB_CLI_COMMAND (disable_ip6_interface_command)
ip6_address_t ilp_addr
the IP6 address
static uword ip6_address_is_zero(const ip6_address_t *a)
#define FOREACH_IP6_LINK_DELEGATE(_ild, _il, body)
@ VNET_SW_INTERFACE_TYPE_HARDWARE
int ip6_link_set_local_address(u32 sw_if_index, const ip6_address_t *address)
static clib_error_t * enable_ip6_interface_cmd(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
VNET_SW_INTERFACE_ADD_DEL_FUNCTION(ip6_link_interface_add_del)
#define IP6_LINK_DBG(...)
static vnet_sw_interface_t * vnet_get_sup_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
int ip6_link_enable(u32 sw_if_index, const ip6_address_t *link_local_addr)
IPv6 Configuration on an interface.
static u64 clib_cpu_time_now(void)
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
static void ip6_link_unlock(ip6_link_t *il)
vl_api_interface_index_t sw_if_index
static u64 il_randomizer
Randomizer.
ethernet_interface_address_t address
@ FIB_ROUTE_PATH_LOCAL
A for-us/local path.
int ip6_link_disable(u32 sw_if_index)
format_function_t format_vnet_sw_interface_name
#define FOREACH_IP6_LINK_DELEGATE_ID(_id)