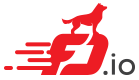 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
40 #ifndef included_snap_h
41 #define included_snap_h
45 #define foreach_ieee_oui \
46 _ (0x000000, ethernet) \
51 #define _(n,f) IEEE_OUI_##f = n,
56 #define foreach_snap_cisco_protocol \
58 _ (0x0104, port_aggregation_protocol) \
59 _ (0x0105, mls_hello) \
60 _ (0x010b, per_vlan_spanning_tree) \
61 _ (0x010c, vlan_bridge) \
62 _ (0x0111, unidirectional_link_detection) \
67 _ (0x200a, stp_uplink_fast)
71 #define _(n,f) SNAP_cisco_##f = n,
116 h->protocol = clib_host_to_net_u16 (
protocol);
117 h->oui[0] = (oui >> 16) & 0xff;
118 h->oui[1] = (oui >> 8) & 0xff;
119 h->oui[2] = (oui >> 0) & 0xff;
122 #define foreach_snap_error \
123 _ (NONE, "no error") \
124 _ (UNKNOWN_PROTOCOL, "unknown oui/snap protocol")
128 #define _(f,s) SNAP_ERROR_##f,
151 return (
h->oui[0] << 16) | (
h->oui[1] << 8) |
h->oui[2];
161 key.protocol =
h->protocol;
static u32 snap_header_get_oui(snap_header_t *h)
format_function_t format_snap_protocol
static uword * mhash_get(mhash_t *h, const void *key)
unformat_function_t unformat_snap_protocol
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
unformat_function_t unformat_pg_snap_header
snap_oui_and_protocol_t oui_and_protocol
format_function_t format_snap_header
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
unformat_function_t unformat_snap_header
void snap_register_input_protocol(vlib_main_t *vm, char *name, u32 ieee_oui, u16 protocol, u32 node_index)
uword * protocol_info_by_name
static void snap_header_set_protocol(snap_header_t *h, snap_oui_and_protocol_t *p)
vl_api_ip_proto_t protocol
#define foreach_snap_cisco_protocol
#define foreach_snap_error
format_function_t format_snap_header_with_length
static snap_protocol_info_t * snap_get_protocol_info(snap_main_t *sm, snap_header_t *h)
snap_protocol_info_t * protocols