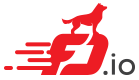 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
40 s =
format (s,
"IPv%s mtu: %u fragments: %u next: %d",
68 to->flags |= VNET_BUFFER_F_QOS_DATA_VALID;
98 u16 len, max, rem, ip_frag_id, ip_frag_offset;
99 u8 *org_from_packet, more;
113 return IP_FRAG_ERROR_MALFORMED;
118 return IP_FRAG_ERROR_CANT_FRAGMENT_HEADER;
121 if (
ip4->flags_and_fragment_offset &
124 return IP_FRAG_ERROR_DONT_FRAGMENT_SET;
129 ip_frag_id =
ip4->fragment_id;
132 !(!(
ip4->flags_and_fragment_offset &
142 u8 *from_data = (
void *) (
ip4 + 1);
145 u16 left_in_from_buffer =
157 len = (rem > max ? max : rem);
162 return IP_FRAG_ERROR_MEMORY;
171 to_ip4 = (
ip4_header_t *) (to_data + l2unfragmentablesize);
172 to_data = (
void *) (to_ip4 + 1);
175 to_b->
flags |= VNET_BUFFER_F_L3_HDR_OFFSET_VALID;
177 if (from_b->
flags & VNET_BUFFER_F_L4_HDR_OFFSET_VALID)
183 to_b->
flags |= VNET_BUFFER_F_L4_HDR_OFFSET_VALID;
187 u16 left_in_to_buffer =
len, to_ptr = 0;
193 bytes_to_copy = left_in_to_buffer <= left_in_from_buffer ?
194 left_in_to_buffer : left_in_from_buffer;
196 left_in_to_buffer -= bytes_to_copy;
197 ptr += bytes_to_copy;
198 left_in_from_buffer -= bytes_to_copy;
199 if (left_in_to_buffer == 0)
202 ASSERT (left_in_from_buffer <= 0);
204 if (!(from_b->
flags & VLIB_BUFFER_NEXT_PRESENT))
206 return IP_FRAG_ERROR_MALFORMED;
212 to_ptr += bytes_to_copy;
215 to_b->
flags |= VNET_BUFFER_F_IS_IP4;
221 clib_host_to_net_u16 ((fo >> 3) + ip_frag_offset);
223 clib_host_to_net_u16 (((
len != rem) || more) << 13);
234 return IP_FRAG_ERROR_NONE;
255 u32 frag_sent = 0, small_packets = 0;
264 u32 pi0, *frag_from, frag_left;
294 if (!
is_ip6 && error0 == IP_FRAG_ERROR_DONT_FRAGMENT_SET)
297 ICMP4_destination_unreachable_fragmentation_needed_and_dont_fragment_set,
303 next0 = (error0 == IP_FRAG_ERROR_NONE ?
308 if (error0 == IP_FRAG_ERROR_NONE)
325 while (frag_left > 0)
327 while (frag_left > 0 && n_left_to_next > 0)
330 i = to_next[0] = frag_from[0];
338 to_next, n_left_to_next,
i,
352 IP_FRAG_ERROR_FRAGMENT_SENT, frag_sent);
354 IP_FRAG_ERROR_SMALL_PACKET, small_packets);
356 return frame->n_vectors;
386 u16 len, max, rem, ip_frag_id;
393 rem = clib_net_to_host_u16 (
ip6->payload_length);
394 max = (mtu -
sizeof (
ip6_header_t) -
sizeof (ip6_frag_hdr_t)) & ~0x7;
399 return IP_FRAG_ERROR_MALFORMED;
403 if (
ip6->protocol == IP_PROTOCOL_IPV6_FRAGMENTATION)
405 return IP_FRAG_ERROR_MALFORMED;
408 u8 *from_data = (
void *) (
ip6 + 1);
411 u16 left_in_from_buffer =
423 ip6_frag_hdr_t *to_frag_hdr;
428 (mtu -
sizeof (
ip6_header_t) -
sizeof (ip6_frag_hdr_t)) ? max : rem);
433 return IP_FRAG_ERROR_MEMORY;
442 to_frag_hdr = (ip6_frag_hdr_t *) (to_ip6 + 1);
443 to_data = (
void *) (to_frag_hdr + 1);
446 to_b->
flags |= VNET_BUFFER_F_L3_HDR_OFFSET_VALID;
448 if (from_b->
flags & VNET_BUFFER_F_L4_HDR_OFFSET_VALID)
454 to_b->
flags |= VNET_BUFFER_F_L4_HDR_OFFSET_VALID;
456 to_b->
flags |= VNET_BUFFER_F_IS_IP6;
459 u16 left_in_to_buffer =
len, to_ptr = 0;
465 bytes_to_copy = left_in_to_buffer <= left_in_from_buffer ?
466 left_in_to_buffer : left_in_from_buffer;
468 left_in_to_buffer -= bytes_to_copy;
469 ptr += bytes_to_copy;
470 left_in_from_buffer -= bytes_to_copy;
471 if (left_in_to_buffer == 0)
474 ASSERT (left_in_from_buffer <= 0);
476 if (!(from_b->
flags & VLIB_BUFFER_NEXT_PRESENT))
478 return IP_FRAG_ERROR_MALFORMED;
484 to_ptr += bytes_to_copy;
490 clib_host_to_net_u16 (
len +
sizeof (ip6_frag_hdr_t));
491 to_ip6->
protocol = IP_PROTOCOL_IPV6_FRAGMENTATION;
492 to_frag_hdr->fragment_offset_and_more =
494 to_frag_hdr->identification = ip_frag_id;
495 to_frag_hdr->next_hdr =
ip6->protocol;
496 to_frag_hdr->rsv = 0;
502 return IP_FRAG_ERROR_NONE;
506 #define _(sym,string) string,
515 .vector_size =
sizeof (
u32),
538 .vector_size =
sizeof (
u32),
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
u32 next_buffer
Next buffer for this linked-list of buffers.
#define IP4_HEADER_FLAG_DONT_FRAGMENT
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
nat44_ei_hairpin_src_next_t next_index
vlib_node_registration_t ip4_frag_node
(constructor) VLIB_REGISTER_NODE (ip4_frag_node)
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
@ VLIB_NODE_TYPE_INTERNAL
vlib_main_t vlib_node_runtime_t * node
#define ip6_frag_hdr_offset_and_more(offset, more)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static void vlib_error_count(vlib_main_t *vm, uword node_index, uword counter, uword increment)
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
static void vlib_buffer_copy_trace_flag(vlib_main_t *vm, vlib_buffer_t *b, u32 bi_target)
void ip_frag_set_vnet_buffer(vlib_buffer_t *b, u16 mtu, u8 next_index, u8 flags)
i16 current_data
signed offset in data[], pre_data[] that we are currently processing.
vlib_error_t * errors
Vector of errors for this node.
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
vlib_error_t error
Error code for buffers to be enqueued to error handler.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
static vlib_buffer_t * frag_buffer_alloc(vlib_buffer_t *org_b, u32 *bi)
static __clib_warn_unused_result u32 vlib_buffer_alloc(vlib_main_t *vm, u32 *buffers, u32 n_buffers)
Allocate buffers into supplied array.
static int ip4_is_fragment(const ip4_header_t *i)
static_always_inline void vnet_buffer_offload_flags_clear(vlib_buffer_t *b, vnet_buffer_oflags_t oflags)
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
static u16 ip4_get_fragment_offset(const ip4_header_t *i)
static uword frag_node_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, u32 node_index, bool is_ip6)
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
@ IP_FRAG_NEXT_IP_REWRITE
#define IP4_FRAG_NODE_NAME
ip_frag_error_t ip4_frag_do_fragment(vlib_main_t *vm, u32 from_bi, u16 mtu, u16 l2unfragmentablesize, u32 **buffer)
struct _vlib_node_registration vlib_node_registration_t
#define foreach_ip_frag_error
#define IP6_FRAG_NODE_NAME
u16 current_length
Nbytes between current data and the end of this buffer.
static uword ip6_frag(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
static_always_inline void icmp4_error_set_vnet_buffer(vlib_buffer_t *b, u8 type, u8 code, u32 data)
#define vec_free(V)
Free vector's memory (no header).
#define vlib_validate_buffer_enqueue_x1(vm, node, next_index, to_next, n_left_to_next, bi0, next0)
Finish enqueueing one buffer forward in the graph.
static uword ip4_frag(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
@ IP_FRAG_NEXT_IP6_LOOKUP
static void frag_set_sw_if_index(vlib_buffer_t *to, vlib_buffer_t *from)
vlib_node_registration_t ip6_frag_node
(constructor) VLIB_REGISTER_NODE (ip6_frag_node)
description fragment has unexpected format
vlib_put_next_frame(vm, node, next_index, 0)
static_always_inline u32 vlib_buffer_get_default_data_size(vlib_main_t *vm)
static u32 running_fragment_id
static vlib_node_runtime_t * vlib_node_get_runtime(vlib_main_t *vm, u32 node_index)
Get node runtime by node index.
ip_frag_error_t ip6_frag_do_fragment(vlib_main_t *vm, u32 from_bi, u16 mtu, u16 l2unfragmentablesize, u32 **buffer)
static char * ip4_frag_error_strings[]
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
static vlib_main_t * vlib_get_main(void)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
static u16 ip4_header_checksum(ip4_header_t *i)
@ IP_FRAG_NEXT_ICMP_ERROR
static u8 * format_ip_frag_trace(u8 *s, va_list *args)
static void vlib_buffer_free_one(vlib_main_t *vm, u32 buffer_index)
Free one buffer Shorthand to free a single buffer chain.
@ IP_FRAG_NEXT_IP4_LOOKUP
#define vlib_get_next_frame(vm, node, next_index, vectors, n_vectors_left)
Get pointer to next frame vector data by (vlib_node_runtime_t, next_index).
#define IP4_HEADER_FLAG_MORE_FRAGMENTS
vl_api_fib_path_type_t type
@ IP_FRAG_NEXT_IP_REWRITE_MIDCHAIN
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)
vl_api_wireguard_peer_flags_t flags