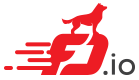 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
23 #define NAT66_EXPECTED_ARGUMENT "expected required argument(s)"
24 #define NAT66_PLUGIN_DISABLED "error plugin disabled"
26 #define CHECK_ENABLED() \
29 if (PREDICT_FALSE (!nat66_main.enabled)) \
31 return clib_error_return (0, NAT66_PLUGIN_DISABLED); \
45 u8 enable_set = 0, enable = 0;
52 if (
unformat (line_input,
"outside-vrf %u", &outside_vrf))
57 if (
unformat (line_input,
"disable"))
59 else if (
unformat (line_input,
"enable"))
113 u32 *inside_sw_if_indices = 0;
114 u32 *outside_sw_if_indices = 0;
131 else if (
unformat (line_input,
"del"))
141 if (
vec_len (inside_sw_if_indices))
143 for (
i = 0;
i <
vec_len (inside_sw_if_indices);
i++)
149 case VNET_API_ERROR_NO_SUCH_ENTRY:
155 case VNET_API_ERROR_VALUE_EXIST:
161 case VNET_API_ERROR_INVALID_VALUE:
162 case VNET_API_ERROR_INVALID_VALUE_2:
165 "%U NAT66 feature enable/disable failed.",
176 if (
vec_len (outside_sw_if_indices))
178 for (
i = 0;
i <
vec_len (outside_sw_if_indices);
i++)
184 case VNET_API_ERROR_NO_SUCH_ENTRY:
190 case VNET_API_ERROR_VALUE_EXIST:
196 case VNET_API_ERROR_INVALID_VALUE:
197 case VNET_API_ERROR_INVALID_VALUE_2:
200 "%U NAT66 feature enable/disable failed.",
247 ip6_address_t l_addr, e_addr;
259 if (
unformat (line_input,
"local %U external %U",
265 else if (
unformat (line_input,
"del"))
279 case VNET_API_ERROR_NO_SUCH_ENTRY:
282 case VNET_API_ERROR_VALUE_EXIST:
342 .short_help =
"nat66 <enable [outside-vrf <vrf-id>]>|disable",
357 .path =
"set interface nat66",
358 .short_help =
"set interface nat66 in|out <intfc> [del]",
374 .path =
"show nat66 interfaces",
375 .short_help =
"show nat66 interfaces",
389 .path =
"nat66 add static mapping",
390 .short_help =
"nat66 add static mapping local <ip6-addr> external <ip6-addr>"
391 " [vfr <table-id>] [del]",
407 .path =
"show nat66 static mappings",
408 .short_help =
"show nat66 static mappings",
int nat66_interface_add_del(u32 sw_if_index, u8 is_inside, u8 is_add)
static vlib_cli_command_t set_interface_nat66_command
(constructor) VLIB_CLI_COMMAND (set_interface_nat66_command)
static vlib_cli_command_t nat66_enable_disable_command
(constructor) VLIB_CLI_COMMAND (nat66_enable_disable_command)
void nat66_interfaces_walk(nat66_interface_walk_fn_t fn, void *ctx)
static int nat66_cli_interface_walk(nat66_interface_t *i, void *ctx)
static vlib_cli_command_t show_nat66_static_mappings_command
(constructor) VLIB_CLI_COMMAND (show_nat66_static_mappings_command)
int nat66_plugin_enable(u32 outside_vrf)
#define clib_error_return(e, args...)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static vlib_cli_command_t show_nat66_interfaces_command
(constructor) VLIB_CLI_COMMAND (show_nat66_interfaces_command)
static vnet_sw_interface_t * vnet_get_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
static clib_error_t * nat66_enable_disable_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
void nat66_static_mappings_walk(nat66_static_mapping_walk_fn_t fn, void *ctx)
int nat66_static_mapping_add_del(ip6_address_t *l_addr, ip6_address_t *e_addr, u32 vrf_id, u8 is_add)
static clib_error_t * nat66_add_del_static_mapping_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
A protocol Independent FIB table.
static int nat66_cli_static_mapping_walk(nat66_static_mapping_t *sm, void *ctx)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
vnet_main_t * vnet_get_main(void)
u32 ft_table_id
Table ID (hash key) for this FIB.
Combined counter to hold both packets and byte differences.
static clib_error_t * nat66_interface_feature_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define VLIB_CLI_COMMAND(x,...)
counter_t packets
packet counter
#define NAT66_EXPECTED_ARGUMENT
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static void vlib_get_combined_counter(const vlib_combined_counter_main_t *cm, u32 index, vlib_counter_t *result)
Get the value of a combined counter, never called in the speed path Scrapes the entire set of per-thr...
#define vec_free(V)
Free vector's memory (no header).
static clib_error_t * nat66_show_interfaces_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static vlib_cli_command_t show_nat66_add_del_static_mapping_command
(constructor) VLIB_CLI_COMMAND (show_nat66_add_del_static_mapping_command)
int nat66_plugin_disable()
unformat_function_t unformat_vnet_sw_interface
fib_table_t * fib_table_get(fib_node_index_t index, fib_protocol_t proto)
Get a pointer to a FIB table.
counter_t bytes
byte counter
#define nat66_interface_is_inside(i)
NAT66 global declarations.
vl_api_interface_index_t sw_if_index
static clib_error_t * nat66_show_static_mappings_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
format_function_t format_vnet_sw_interface_name