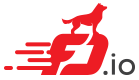 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
87 memset (ct, 0xfc,
sizeof (*ct));
134 sep->port = ct->c_lcl_port;
135 sep->is_ip4 = ct->c_is_ip4;
136 ip_copy (&sep->ip, &ct->c_lcl_ip, ct->c_is_ip4);
158 seg_index = rx_fifo->segment_index;
174 if (ct->
flags & CT_CONN_F_CLIENT)
202 if (ct->
flags & CT_CONN_F_CLIENT)
230 table_handle = (
u64) seg_ctx->
sm_index << 32 | table_handle;
246 if (ct->
flags & CT_CONN_F_CLIENT)
304 cct->
flags &= ~CT_CONN_F_HALF_OPEN;
329 err = SESSION_E_ALLOC;
343 cs =
session_get (cct->c_s_index, cct->c_thread_index);
364 uword free_bytes, max_free_bytes;
382 if (free_bytes > max_free_bytes &&
385 max_free_bytes = free_bytes;
397 u32 seg_ctx_index = ~0, sm_index, pair_bytes;
399 const u32 margin = 16 << 10;
402 u64 seg_size, seg_handle;
411 pair_bytes = props->rx_fifo_size + props->tx_fifo_size + margin;
417 spp =
hash_get (
cm->app_segs_ctxs_table, table_handle);
420 seg_ctx_index = *spp;
430 seg_size =
clib_max (props->segment_size, 128 << 20);
436 if (seg_ctx_index == ~0)
439 seg_ctx_index = seg_ctx -
cm->app_seg_ctxs;
440 hash_set (
cm->app_segs_ctxs_table, table_handle, seg_ctx_index);
484 u64 seg_handle, table_handle;
485 u32 sm_index, fs_index = ~0;
501 table_handle = (
u64) sm_index << 32 | table_handle;
509 spp =
hash_get (
cm->app_segs_ctxs_table, table_handle);
554 fs, ls->
thread_index, props->rx_fifo_size, props->tx_fifo_size,
576 ls->
rx_fifo->segment_manager = sm_index;
577 ls->
tx_fifo->segment_manager = sm_index;
578 ls->
rx_fifo->segment_index = fs_index;
579 ls->
tx_fifo->segment_index = fs_index;
603 cct_index = cct->c_c_index;
616 cct->c_c_index = cct_index;
618 cct->
flags |= CT_CONN_F_HALF_OPEN;
641 sct->c_lcl_port = ll_ct->lcl_port;
642 sct->c_is_ip4 = cct->c_is_ip4;
643 clib_memcpy (&sct->c_lcl_ip, &ll_ct->lcl_ip, sizeof (ll_ct->lcl_ip));
645 sct->c_proto = TRANSPORT_PROTO_NONE;
716 ho_index = ho->c_c_index;
717 ho->c_rmt_port = sep->port;
719 ho->c_is_ip4 = sep->is_ip4;
723 ho->c_proto = TRANSPORT_PROTO_NONE;
725 clib_memcpy (&ho->c_rmt_ip, &sep->ip, sizeof (sep->ip));
726 ho->
flags |= CT_CONN_F_CLIENT;
751 ct->c_is_ip4 = sep->is_ip4;
752 clib_memcpy (&ct->c_lcl_ip, &sep->ip, sizeof (sep->ip));
753 ct->c_lcl_port = sep->port;
754 ct->c_s_index = app_listener_index;
756 return ct->c_c_index;
820 sep->transport_proto = sep_ext->original_tp;
824 return SESSION_E_FILTERED;
849 return SESSION_E_NOROUTE;
852 return SESSION_E_SCOPE;
863 return SESSION_E_LOCAL_CONNECT;
874 s =
session_get (ct->c_s_index, ct->c_thread_index);
880 if (peer_ct->
flags & CT_CONN_F_HALF_OPEN)
884 else if (peer_ct->c_s_index != ~0)
890 if (ct->
flags & CT_CONN_F_CLIENT)
928 s =
format (s,
"[%d:%d][CT:%U] %U:%d->%U:%d", ct->c_thread_index,
932 &ct->c_rmt_ip4, clib_net_to_host_u16 (ct->c_rmt_port));
936 s =
format (s,
"[%d:%d][CT:%U] %U:%d->%U:%d", ct->c_thread_index,
940 &ct->c_rmt_ip6, clib_net_to_host_u16 (ct->c_rmt_port));
974 ps =
session_get (peer_ct->c_s_index, peer_ct->c_thread_index);
981 u32 tc_index = va_arg (*args,
u32);
983 u32 __clib_unused verbose = va_arg (*args,
u32);
994 u32 ho_index = va_arg (*args,
u32);
995 u32 verbose = va_arg (*args,
u32);
1007 u32 verbose = va_arg (*args,
u32);
1026 u32 ct_index = va_arg (*args,
u32);
1028 u32 verbose = va_arg (*args,
u32);
1034 s =
format (s,
"empty\n");
1071 .transport_options = {
1090 peer_s =
session_get (peer_ct->c_s_index, peer_ct->c_thread_index);
1091 if (peer_s->
session_state >= SESSION_STATE_TRANSPORT_CLOSING)
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
fifo_segment_t * segment_manager_get_segment_w_lock(segment_manager_t *sm, u32 segment_index)
Reads a segment from the segment manager's pool and acquires reader lock.
static void clib_spinlock_init(clib_spinlock_t *p)
#define SESSION_CLI_STATE_LEN
struct ct_segments_ ct_segments_ctx_t
static u8 * format_ct_listener(u8 *s, va_list *args)
static u32 vlib_num_workers()
u32 wrk_index
Worker index in global worker pool.
static ct_connection_t * ct_half_open_alloc(void)
enum session_error_ session_error_t
#define hash_set(h, key, value)
int session_enqueue_notify(session_t *s)
@ CT_SEGMENT_F_SERVER_DETACHED
segment_manager_t * segment_manager_get(u32 index)
int session_dequeue_notify(session_t *s)
ct_segments_ctx_t * app_seg_ctxs
Pool of ct segment contexts.
u32 session_index
Index in thread pool where session was allocated.
static void svm_fifo_unset_event(svm_fifo_t *f)
Unset fifo event flag.
#define clib_memcpy(d, s, n)
#define SESSION_CLI_ID_LEN
void session_close(session_t *s)
Initialize session closing procedure.
static u32 ct_start_listen(u32 app_listener_index, transport_endpoint_t *tep)
void session_transport_closing_notify(transport_connection_t *tc)
Notification from transport that connection is being closed.
static ct_connection_t * ct_connection_alloc(u32 thread_index)
static uword pointer_to_uword(const void *p)
void session_half_open_delete_notify(transport_connection_t *tc)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
static int ct_custom_tx(void *session, transport_send_params_t *sp)
static u64 listen_session_get_handle(session_t *s)
session_t * ct_session_get_peer(session_t *s)
static void ct_session_dealloc_fifos(ct_connection_t *ct, svm_fifo_t *rx_fifo, svm_fifo_t *tx_fifo)
void ct_half_open_add_reusable(u32 ho_index)
segment_manager_t * app_worker_get_listen_segment_manager(app_worker_t *, session_t *)
struct _segment_manager segment_manager_t
struct _session_endpoint_cfg session_endpoint_cfg_t
segment_manager_props_t * application_segment_manager_properties(application_t *app)
struct _transport_proto_vft transport_proto_vft_t
int ct_session_connect_notify(session_t *ss, session_error_t err)
static session_t * listen_session_get(u32 ls_index)
u32 app_index
App index in app pool.
int session_half_open_migrated_notify(transport_connection_t *tc)
u8 thread_index
Index of the thread that allocated the session.
#define pool_put(P, E)
Free an object E in pool P.
session_type_t session_type
Type built from transport and network protocol types.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
u32 * ho_reusable
Vector of reusable ho indices.
static u8 session_endpoint_is_local(session_endpoint_t *sep)
ct_connection_flags_t flags
struct _transport_connection transport_connection_t
int app_worker_del_segment_notify(app_worker_t *app_wrk, u64 segment_handle)
static void clib_rwlock_reader_lock(clib_rwlock_t *p)
static void clib_rwlock_reader_unlock(clib_rwlock_t *p)
void session_free(session_t *s)
#define pool_put_index(p, i)
Free pool element with given index.
static void clib_rwlock_writer_unlock(clib_rwlock_t *p)
#define hash_unset(h, key)
u8 segment_manager_app_detached(segment_manager_t *sm)
struct _svm_fifo svm_fifo_t
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
int app_worker_cleanup_notify(app_worker_t *app_wrk, session_t *s, session_cleanup_ntf_t ntf)
application_t * application_get(u32 app_index)
u32 app_wrk_index
Index of the app worker that owns the session.
u8 application_has_global_scope(application_t *app)
#define pool_foreach(VAR, POOL)
Iterate through pool.
int app_worker_init_connected(app_worker_t *app_wrk, session_t *s)
@ TRANSPORT_SND_F_DESCHED
static ct_segment_t * ct_lookup_free_segment(ct_main_t *cm, segment_manager_t *sm, u32 seg_ctx_index)
svm_fifo_t * rx_fifo
Pointers to rx/tx buffers.
static void ct_session_close(u32 ct_index, u32 thread_index)
u32 app_index
owning app index
u32 app_index
Index of application that owns the listener.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
void segment_manager_dealloc_fifos(svm_fifo_t *rx_fifo, svm_fifo_t *tx_fifo)
transport_connection_t * session_get_transport(session_t *s)
static transport_connection_t * ct_listener_get(u32 ct_index)
session_t * session_lookup_listener_wildcard(u32 table_index, session_endpoint_t *sep)
Lookup listener wildcard match.
struct _segment_manager_props segment_manager_props_t
static_always_inline uword vlib_get_thread_index(void)
app_worker_t * app_worker_get_if_valid(u32 wrk_index)
app_worker_t * application_listener_select_worker(session_t *ls)
static_always_inline void clib_spinlock_lock(clib_spinlock_t *p)
static u8 session_endpoint_fib_proto(session_endpoint_t *sep)
static void clib_rwlock_writer_lock(clib_rwlock_t *p)
svm_fifo_t * client_rx_fifo
void segment_manager_segment_reader_unlock(segment_manager_t *sm)
fifo_segment_t * segment_manager_get_segment(segment_manager_t *sm, u32 segment_index)
Reads a segment from the segment manager's pool without lock.
void session_half_open_migrate_notify(transport_connection_t *tc)
void transport_register_protocol(transport_proto_t transport_proto, const transport_proto_vft_t *vft, fib_protocol_t fib_proto, u32 output_node)
Register transport virtual function table.
u64 segment_manager_segment_handle(segment_manager_t *sm, fifo_segment_t *segment)
vnet_feature_config_main_t * cm
void segment_manager_lock_and_del_segment(segment_manager_t *sm, u32 fs_index)
u64 session_lookup_local_endpoint(u32 table_index, session_endpoint_t *sep)
Look up endpoint in local session table.
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
static ct_connection_t * ct_connection_get(u32 ct_index, u32 thread_index)
static u8 * format_ct_session(u8 *s, va_list *args)
#define SESSION_DROP_HANDLE
static u8 * format_ct_connection_id(u8 *s, va_list *args)
u32 segment_manager_index(segment_manager_t *sm)
static session_t * session_get(u32 si, u32 thread_index)
void session_transport_delete_notify(transport_connection_t *tc)
Notification from transport that connection is being deleted.
int app_worker_connect_notify(app_worker_t *app_wrk, session_t *s, session_error_t err, u32 opaque)
static void clib_rwlock_init(clib_rwlock_t *p)
u32 n_workers
Number of vpp workers.
transport_proto_t actual_tp
@ TRANSPORT_TX_INTERNAL
apps acting as transports
session_handle_t listener_handle
Parent listener session index if the result of an accept.
static session_type_t session_type_from_proto_and_ip(transport_proto_t proto, u8 is_ip4)
@ TRANSPORT_SERVICE_VC
virtual circuit service
u32 application_local_session_table(application_t *app)
transport_connection_t connection
u32 fifo_segment_num_fifos(fifo_segment_t *fs)
Get number of active fifos.
static u32 ct_stop_listen(u32 ct_index)
void fifo_segment_free_fifo(fifo_segment_t *fs, svm_fifo_t *f)
Free fifo allocated in fifo segment.
static int ct_init_accepted_session(app_worker_t *server_wrk, ct_connection_t *ct, session_t *ls, session_t *ll)
@ TRANSPORT_CONNECTION_F_NO_LOOKUP
Don't register connection in lookup.
description fragment has unexpected format
static transport_connection_t * ct_half_open_get(u32 ct_index)
clib_rwlock_t app_segs_lock
RW lock for seg contexts.
struct ct_main_ ct_main_t
int app_worker_add_segment_notify(app_worker_t *app_wrk, u64 segment_handle)
Send an API message to the external app, to map new segment.
@ SESSION_CLEANUP_SESSION
#define VLIB_INIT_FUNCTION(x)
transport_connection_t * listen_session_get_transport(session_t *s)
void ip_copy(ip46_address_t *dst, ip46_address_t *src, u8 is_ip4)
u32 n_sessions
Cumulative sessions counter.
app_worker_t * app_worker_get(u32 wrk_index)
enum ct_segment_flags_ ct_segment_flags_t
ct_connection_t ** connections
Per-worker connection pools.
#define vec_foreach(var, vec)
Vector iterator.
uword * app_segs_ctxs_table
App handle to segment pool map.
static void ct_cleanup_ho(u32 ho_index)
struct _session_endpoint session_endpoint_t
static uword pool_elts(void *v)
Number of active elements in a pool.
static void ct_connection_free(ct_connection_t *ct)
svm_fifo_t * client_tx_fifo
static_always_inline void clib_spinlock_unlock(clib_spinlock_t *p)
#define SESSION_INVALID_HANDLE
static clib_error_t * ct_transport_init(vlib_main_t *vm)
uword fifo_segment_available_bytes(fifo_segment_t *fs)
u8 segment_manager_has_fifos(segment_manager_t *sm)
segment_manager_t * app_worker_get_connect_segment_manager(app_worker_t *)
int ct_session_tx(session_t *s)
@ FIFO_SEGMENT_F_CUSTOM_USE
@ CT_SEGMENT_F_CLIENT_DETACHED
uword fifo_segment_size(fifo_segment_t *fs)
Fifo segment allocated size.
#define pool_get_zero(P, E)
Allocate an object E from a pool P and zero it.
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
void ct_session_endpoint(session_t *ll, session_endpoint_t *sep)
#define uword_to_pointer(u, type)
#define pool_free(p)
Free a pool.
static const transport_proto_vft_t cut_thru_proto
transport_snd_flags_t flags
struct ct_segment_ ct_segment_t
static int ct_app_rx_evt(transport_connection_t *tc)
static u8 * format_ct_connection(u8 *s, va_list *args)
void segment_manager_free_safe(segment_manager_t *sm)
static ct_segment_t * ct_alloc_segment(ct_main_t *cm, app_worker_t *server_wrk, u64 table_handle, segment_manager_t *sm, u32 client_wrk_index)
void session_free_w_fifos(session_t *s)
int segment_manager_add_segment2(segment_manager_t *sm, uword segment_size, u8 flags)
app_listener_t * app_listener_get_w_session(session_t *ls)
static transport_connection_t * ct_session_get(u32 ct_index, u32 thread_index)
static u8 * format_ct_half_open(u8 *s, va_list *args)
clib_spinlock_t ho_reuseable_lock
Lock for reusable ho indices.
u32 app_index
Index of owning app.
static u8 session_has_transport(session_t *s)
static void ct_session_cleanup(u32 conn_index, u32 thread_index)
static int ct_connect(app_worker_t *client_wrk, session_t *ll, session_endpoint_cfg_t *sep)
u8 * format_transport_proto_short(u8 *s, va_list *args)
u32 session_lookup_get_index_for_fib(u32 fib_proto, u32 fib_index)
clib_error_t * ct_enable_disable(vlib_main_t *vm, u8 is_en)
int app_worker_accept_notify(app_worker_t *app_wrk, session_t *s)
session_t * session_alloc(u32 thread_index)
static int ct_session_connect(transport_endpoint_cfg_t *tep)
volatile u8 session_state
State in session layer state machine.
u32 connection_index
Index of the transport connection associated to the session.
static void ct_accept_rpc_wrk_handler(void *accept_args)
int segment_manager_try_alloc_fifos(fifo_segment_t *fifo_segment, u32 thread_index, u32 rx_fifo_size, u32 tx_fifo_size, svm_fifo_t **rx_fifo, svm_fifo_t **tx_fifo)
static session_t * listen_session_get_from_handle(session_handle_t handle)
void session_send_rpc_evt_to_thread_force(u32 thread_index, void *fp, void *rpc_args)
u64 segment_manager_make_segment_handle(u32 segment_manager_index, u32 segment_index)