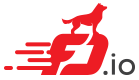 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
53 iproto =
ip4->protocol;
59 iproto =
ip6->protocol;
74 if (!session_not_found)
102 clib_host_to_net_u16 (udp0->
dst_port), iproto);
113 u32 rsession_flags = 0;
163 CNAT_ERROR_EXHAUSTED_PORTS, 1);
223 .name =
"ip4-cnat-tx",
224 .vector_size =
sizeof (
u32),
237 .name =
"ip6-cnat-tx",
238 .vector_size =
sizeof (
u32),
u16 dpoi_next_node
The next VLIB node to follow.
cnat_src_policy_main_t cnat_src_policy_main
static vlib_cli_command_t trace
(constructor) VLIB_CLI_COMMAND (trace)
static_always_inline void cnat_add_trace(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_buffer_t *b, cnat_session_t *session, const cnat_translation_t *ct, u8 flags)
index_t dpoi_index
the index of objects of that type
static_always_inline cnat_ep_trk_t * cnat_load_balance(const cnat_translation_t *ct, ip_address_family_t af, ip4_header_t *ip4, ip6_header_t *ip6, u32 *dpoi_index)
ip46_address_t cs_ip[VLIB_N_DIR]
IP 4/6 address in the rx/tx direction.
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
cnat_endpoint_t ct_ep[VLIB_N_DIR]
The EP being tracked.
static void ip46_address_set_ip4(ip46_address_t *ip46, const ip4_address_t *ip)
index_t cs_lbi
The load balance object to use to forward.
static_always_inline u32 cnat_client_cnt_session(cnat_client_t *cc)
Add a session refcnt to this client.
@ VLIB_NODE_TYPE_INTERNAL
static_always_inline void ip46_address_set_ip6(ip46_address_t *dst, const ip6_address_t *src)
vlib_main_t vlib_node_runtime_t * node
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static_always_inline void cnat_session_create(cnat_session_t *session, cnat_node_ctx_t *ctx, cnat_session_location_t rsession_location, u8 rsession_flags)
Create NAT sessions rsession_location is the location the (return) session will be matched at.
u32 dpoi_next_node
Persist translation->ct_lb.dpoi_next_node.
A session represents the memory of a translation.
struct cnat_session_t_::@645 key
this key sits in the same memory location a 'key' in the bihash kvp
@ CNAT_SOURCE_ERROR_USE_DEFAULT
@ CNAT_SOURCE_ERROR_EXHAUSTED_PORTS
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
@ CNAT_TRANSLATION_N_NEXT
dpo_id_t cc_parent
How to send packets to this client post translation.
@ CNAT_TRACE_SESSION_FOUND
#define VLIB_NODE_FN(node)
index_t parent_cci
Parent cnat_client index if cloned via interpose or own index if vanilla client.
#define VLIB_NODE_FLAG_TRACE
static u8 * format_cnat_trace(u8 *s, va_list *args)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
@ CNAT_SESSION_FLAG_HAS_SNAT
Indicates a return path session that was source NATed on the way in.
static_always_inline void cnat_translation_ip6(const cnat_session_t *session, ip6_header_t *ip6, udp_header_t *udp)
static void cnat_timestamp_update(u32 index, f64 t)
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
vlib_node_registration_t cnat_vip_ip4_node
(constructor) VLIB_REGISTER_NODE (cnat_vip_ip4_node)
static_always_inline void cnat_translation_ip4(const cnat_session_t *session, ip4_header_t *ip4, udp_header_t *udp)
cnat_vip_source_policy_t vip_policy
struct _vlib_node_registration vlib_node_registration_t
static_always_inline void ip46_address_copy(ip46_address_t *dst, const ip46_address_t *src)
u32 cs_ts_index
Timestamp index this session was last used.
ip_protocol_t cs_proto
The IP protocol TCP or UDP only supported.
bool ip_address_is_zero(const ip_address_t *ip)
A collection of combined counters.
vlib_node_registration_t cnat_vip_ip6_node
(constructor) VLIB_REGISTER_NODE (cnat_vip_ip6_node)
static uword cnat_node_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, cnat_node_sub_t cnat_sub, ip_address_family_t af, cnat_session_location_t cs_loc, u8 do_trace)
cnat_vip_source_policy_t default_policy
static_always_inline cnat_client_t * cnat_client_get(index_t i)
enum ip_protocol ip_protocol_t
vlib_combined_counter_main_t cnat_translation_counters
Counters for each translation.
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
dpo_id_t ct_lb
The LB used to forward to the backends.
cnat_translation_t * cnat_translation_pool
struct cnat_session_t_::@646 value
this value sits in the same memory location a 'value' in the bihash kvp
static uword cnat_vip_node_fn(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_buffer_t *b, cnat_node_ctx_t *ctx, int session_not_found, cnat_session_t *session)
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
A client is a representation of an IP address behind the NAT.
@ CNAT_TRANSLATION_NEXT_DROP
@ CNAT_TRANSLATION_NEXT_LOOKUP
enum cnat_translation_next_t_ cnat_translation_next_t
vl_api_fib_path_type_t type
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
@ CNAT_TRACE_SESSION_CREATED
A Translation represents the translation of a VEP to one of a set of real server addresses.
Data used to track an EP in the FIB.
VLIB buffer representation.
u16 cs_port[VLIB_N_DIR]
ports in rx/tx
static_always_inline cnat_translation_t * cnat_find_translation(index_t cti, u16 port, ip_protocol_t proto)
#define VLIB_REGISTER_NODE(x,...)