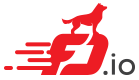 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
34 .
name =
"cnat-translation",
35 .stat_segment_name =
"/net/cnat-translation",
118 s =
format (s,
"default");
122 s =
format (s,
"unknown");
132 else if (
unformat (input,
"maglev"))
271 bk.
skip = h2 % (
cm->maglev_len - 1) + 1;
291 u32 c = (bk->offset +
next * bk->skip) %
cm->maglev_len;
295 c = (bk->offset +
next * bk->skip) %
cm->maglev_len;
299 if (done ==
cm->maglev_len)
348 return (VNET_API_ERROR_NO_SUCH_ENTRY);
466 u32 indent = va_arg (*args,
u32);
495 s =
format (s,
"\n via:");
503 s =
format (s,
"\nmaglev backends map");
504 uword *bitmap = NULL;
560 index_t tri, *trp, *trs = NULL;
577 .path =
"show cnat translation",
579 .short_help =
"show cnat translation <VIP>",
657 else if (
unformat (line_input,
"del %d", &del_index))
694 .path =
"cnat translation",
695 .short_help =
"cnat translation [add|del] proto [TCP|UDP] [vip|real] [ip|sw_if_index [v6]] [port] [to [ip|sw_if_index [v6]] [port]->[ip|sw_if_index [v6]] [port]]",
793 u32 address_length,
u32 if_address_index,
805 u32 address_length,
u32 if_address_index,
831 cm->translation_hash_buckets,
832 cm->translation_hash_memory);
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
vnet_interface_main_t * im
@ CNAT_RESOLV_ADDR_BACKEND
int cnat_translation_delete(u32 id)
Delete a translation.
index_t dpoi_index
the index of objects of that type
static vlib_cli_command_t cnat_translation_cli_add_del_command
(constructor) VLIB_CLI_COMMAND (cnat_translation_cli_add_del_command)
static fib_node_t * cnat_translation_get_node(fib_node_index_t index)
u8 cnat_resolve_ep(cnat_endpoint_t *ep)
Resolve endpoint address.
u32 sw_if_index
The interface index to resolve.
enum fib_node_back_walk_rc_t_ fib_node_back_walk_rc_t
Return code from a back walk function.
ip_protocol_t ct_proto
The ip protocol for the translation.
#define clib_memcpy(d, s, n)
cnat_endpoint_t ct_ep[VLIB_N_DIR]
The EP being tracked.
ip4_main_t ip4_main
Global ip4 main structure.
enum dpo_proto_t_ dpo_proto_t
Data path protocol.
load_balance_t * load_balance_pool
Pool of all DPOs.
@ CNAT_TRANSLATION_STACKED
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
void fib_entry_untrack(fib_node_index_t fei, u32 sibling)
Stop tracking a FIB entry.
vlib_main_t vlib_node_runtime_t * node
void cnat_translation_register_addr_add_cb(cnat_addr_resol_type_t typ, cnat_if_addr_add_cb_t fn)
static void cnat_tracker_release(cnat_ep_trk_t *trk)
A FIB graph nodes virtual function table.
void cnat_translation_watch_addr(index_t cti, u64 opaque, cnat_endpoint_t *ep, cnat_addr_resol_type_t type)
Add an address resolution request.
#define clib_error_return(e, args...)
index_t load_balance_create(u32 n_buckets, dpo_proto_t lb_proto, flow_hash_config_t fhc)
void cnat_translation_unwatch_addr(u32 cti, cnat_addr_resol_type_t type)
Cleanup matching addr resolution requests.
void ip_address_to_fib_prefix(const ip_address_t *addr, fib_prefix_t *prefix)
convert from a IP address to a FIB prefix
void vlib_validate_combined_counter(vlib_combined_counter_main_t *cm, u32 index)
validate a combined counter
#define pool_put(P, E)
Free an object E in pool P.
int cnat_translation_purge(void)
Purge all the trahslations.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
char * name
The counter collection's name.
static clib_error_t * cnat_translation_show(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static void cnat_tracker_track(index_t cti, cnat_ep_trk_t *trk)
#define clib_bitmap_alloc(v, n_bits)
Allocate a bitmap with the supplied number of bits.
enum fib_node_type_t_ fib_node_type_t
The types of nodes in a FIB graph.
#define pool_put_index(p, i)
Free pool element with given index.
#define hash_v3_finalize32(a, b, c)
void fib_entry_contribute_forwarding(fib_node_index_t fib_entry_index, fib_forward_chain_type_t fct, dpo_id_t *dpo)
static void cnat_translation_init_maglev(cnat_translation_t *ct)
void cnat_client_translation_added(index_t cci)
A translation that references this VIP was added.
import vnet interface_types api
fib_node_index_t ct_fei
The FIB entry for the EP.
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
static fib_node_back_walk_rc_t cnat_translation_back_walk_notify(fib_node_t *node, fib_node_back_walk_ctx_t *ctx)
static void cnat_translation_last_lock_gone(fib_node_t *node)
u32 cnat_translation_update(cnat_endpoint_t *vip, ip_protocol_t proto, cnat_endpoint_tuple_t *paths, u8 flags, cnat_lb_type_t lb_type)
create or update a translation
fib_node_index_t fib_entry_track(u32 fib_index, const fib_prefix_t *prefix, fib_node_type_t child_type, index_t child_index, u32 *sibling)
Trackers are used on FIB entries by objects that which to track the changing state of the entry.
static void cnat_ip6_if_addr_add_del_callback(struct ip6_main_t *im, uword opaque, u32 sw_if_index, ip6_address_t *address, u32 address_length, u32 if_address_index, u32 is_del)
#define STRUCT_OFFSET_OF(t, f)
#define ip_addr_version(_a)
#define pool_foreach(VAR, POOL)
Iterate through pool.
uword unformat_cnat_ep_tuple(unformat_input_t *input, va_list *args)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
cnat_endpoint_t ct_vip
The Virtual end point.
cnat_ep_trk_t * ct_paths
The vector of tracked back-ends.
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
void dpo_stack(dpo_type_t child_type, dpo_proto_t child_proto, dpo_id_t *dpo, const dpo_id_t *parent)
Stack one DPO object on another, and thus establish a child-parent relationship.
cnat_lb_type_t lb_type
Type of load balancing.
u8 * format_cnat_translation(u8 *s, va_list *args)
ip_address_family_t version
u8 ct_flags
Allows to disable if not resolved yet.
static void cnat_ip4_if_addr_add_del_callback(struct ip4_main_t *im, uword opaque, u32 sw_if_index, ip4_address_t *address, u32 address_length, u32 if_address_index, u32 is_del)
index_t parent_cci
Parent cnat_client index if cloned via interpose or own index if vanilla client.
vl_api_cnat_lb_type_t lb_type
ip6_add_del_interface_address_callback_t * add_del_interface_address_callbacks
static u8 * format_cnat_ep_trk(u8 *s, va_list *args)
u8 * format_cnat_lb_type(u8 *s, va_list *args)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
#define IP_FLOW_HASH_DEFAULT
Default: 5-tuple + flowlabel without the "reverse" bit.
u32 fib_node_index_t
A typedef of a node index.
#define clib_bitmap_free(v)
Free a bitmap.
u32 ct_sibling
The sibling on the entry's child list.
static void vlib_zero_combined_counter(vlib_combined_counter_main_t *cm, u32 index)
Clear a combined counter Clears the set of per-thread counters.
index_t ct_cci
The client object this translation belongs on INDEX_INVALID if vip is unresolved.
static void cnat_add_translation_to_db(index_t cci, cnat_endpoint_t *vip, ip_protocol_t proto, index_t cti)
Add a translation to the bihash.
vnet_feature_config_main_t * cm
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
static fib_node_type_t cnat_translation_fib_node_type
manual_print typedef address
#define VLIB_CLI_COMMAND(x,...)
enum fib_protocol_t_ fib_protocol_t
Protocol Type.
void ip_address_set(ip_address_t *dst, const void *src, ip_address_family_t af)
cnat_if_addr_add_cb_t * cnat_if_addr_add_cbs
static uword * clib_bitmap_set(uword *ai, uword i, uword value)
Sets the ith bit of a bitmap to new_value Removes trailing zeros from the bitmap.
@ CNAT_ADDR_N_RESOLUTIONS
@ FIB_NODE_BACK_WALK_CONTINUE
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
dpo_proto_t fib_proto_to_dpo(fib_protocol_t fib_proto)
static clib_error_t * cnat_translation_init(vlib_main_t *vm)
void(* cnat_if_addr_add_cb_t)(addr_resolution_t *ar, ip_address_t *address, u8 is_del)
Register a call back for endpoint->address resolution.
u8 * format_dpo_id(u8 *s, va_list *args)
Format a DPO_id_t oject.
fib_protocol_t ip_address_family_to_fib_proto(ip_address_family_t af)
walk_rc_t(* cnat_translation_walk_cb_t)(index_t index, void *ctx)
Callback function invoked during a walk of all translations.
index_t cnat_client_add(const ip_address_t *ip, u8 flags)
void load_balance_set_bucket(index_t lbi, u32 bucket, const dpo_id_t *next)
@ DPO_LOAD_BALANCE
load-balancing over a choice of [un]equal cost paths
ip4_add_del_interface_address_callback_t * add_del_interface_address_callbacks
Functions to call when interface address changes.
ip4_add_del_interface_address_function_t * function
#define vec_free(V)
Free vector's memory (no header).
cnat_addr_resol_type_t type
The cnat_addr_resolution_t.
static void cnat_if_addr_add_del_translation_cb(addr_resolution_t *ar, ip_address_t *address, u8 is_del)
static int cnat_maglev_entry_compare(void *_a, void *_b)
ip6_add_del_interface_address_function_t * function
#define pool_foreach_index(i, v)
fib_forward_chain_type_t fib_forw_chain_type_from_fib_proto(fib_protocol_t proto)
Convert from a fib-protocol to a chain type.
description fragment has unexpected format
A collection of combined counters.
fib_node_t ct_node
Linkage into the FIB graph.
static clib_error_t * cnat_translation_cli_add_del(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define VLIB_INIT_FUNCTION(x)
static cnat_translation_t * cnat_translation_get_from_node(fib_node_t *node)
void cnat_lazy_init()
Lazy initialization when first adding a translation or using snat.
static void cnat_resolve_ep_tuple(cnat_endpoint_tuple_t *path)
static void cnat_remove_translation_from_db(index_t cci, cnat_endpoint_t *vip, ip_protocol_t proto)
Remove a translation from the bihash.
#define vec_foreach(var, vec)
Vector iterator.
cnat_ep_trk_t * ct_active_paths
The vector of active tracked back-ends.
8 octet key, 8 octet key value pair
static uword pool_elts(void *v)
Number of active elements in a pool.
ip_address_family_t af
ip4 or ip6 resolution
fib_protocol_t fp_proto
protocol type
dpo_type_t cnat_client_dpo
addr_resolution_t * tr_resolutions
An node in the FIB graph.
static void cnat_translation_stack(cnat_translation_t *ct)
#define vec_sort_with_function(vec, f)
Sort a vector using the supplied element comparison function.
static_always_inline cnat_client_t * cnat_client_get(index_t i)
static void cnat_if_addr_add_del_callback(u32 sw_if_index, ip_address_t *address, u8 is_del)
enum ip_protocol ip_protocol_t
index_t index
Own index (if copied for trace)
void cnat_translation_walk(cnat_translation_walk_cb_t cb, void *ctx)
Walk/visit each of the translations.
u8 * format_cnat_endpoint(u8 *s, va_list *args)
vlib_combined_counter_main_t cnat_translation_counters
Counters for each translation.
#define vec_set(v, val)
Set all vector elements to given value.
#define pool_get_zero(P, E)
Allocate an object E from a pool P and zero it.
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
static vlib_cli_command_t cnat_translation_show_cmd_node
(constructor) VLIB_CLI_COMMAND (cnat_translation_show_cmd_node)
@ CNAT_RESOLV_ADDR_TRANSLATION
uword unformat_cnat_lb_type(unformat_input_t *input, va_list *args)
dpo_id_t ct_lb
The LB used to forward to the backends.
Context passed between object during a back walk.
static const fib_node_vft_t cnat_translation_vft
u8 cnat_resolve_addr(u32 sw_if_index, ip_address_family_t af, ip_address_t *addr)
cnat_translation_t * cnat_translation_pool
__clib_export u8 * format_bitmap_hex(u8 *s, va_list *args)
Format a bitmap as a string of hex bytes.
int ip_address_cmp(const ip_address_t *ip1, const ip_address_t *ip2)
static void cnat_if_addr_add_del_backend_cb(addr_resolution_t *ar, ip_address_t *address, u8 is_del)
index_t cti
Translation index.
#define clib_bitmap_zero(v)
Clear a bitmap.
void dpo_set(dpo_id_t *dpo, dpo_type_t type, dpo_proto_t proto, index_t index)
Set/create a DPO ID The DPO will be locked.
#define hash_mix64(a0, b0, c0)
u8 flags
Translation flags.
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
void ip_address_copy(ip_address_t *dst, const ip_address_t *src)
vl_api_interface_index_t sw_if_index
A client is a representation of an IP address behind the NAT.
dpo_id_t ct_dpo
The forwarding contributed by the entry.
void dpo_reset(dpo_id_t *dpo)
reset a DPO ID The DPO will be unlocked.
fib_node_type_t fib_node_register_new_type(const fib_node_vft_t *vft)
Create a new FIB node type and Register the function table for it.
clib_bihash_8_8_t cnat_translation_db
Aggregate type for a prefix.
vl_api_fib_path_type_t type
void cnat_client_translation_deleted(index_t cci)
A translation that references this VIP was deleted.
static_always_inline cnat_translation_t * cnat_translation_get(index_t cti)
Entry used to account for a translation's backend waiting for address resolution.
uword unformat_cnat_ep(unformat_input_t *input, va_list *args)
A Translation represents the translation of a VEP to one of a set of real server addresses.
Data used to track an EP in the FIB.
#define hash_v3_mix32(a, b, c)
static_always_inline cnat_translation_t * cnat_find_translation(index_t cti, u16 port, ip_protocol_t proto)
vl_api_wireguard_peer_flags_t flags