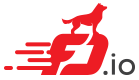 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
97 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
111 return VNET_API_ERROR_INVALID_VALUE;
129 app_ns->sock_name =
vec_dup (
a->sock_name);
143 app_ns->ns_secret =
a->secret;
144 app_ns->sw_if_index =
a->sw_if_index;
145 app_ns->ip4_fib_index =
147 app_ns->ip6_fib_index =
156 return VNET_API_ERROR_INVALID_VALUE;
160 return VNET_API_ERROR_INVALID_VALUE;
172 if (app_ns->sock_name)
184 return app_ns->ns_id;
216 app_ns->ip4_fib_index : app_ns->ip6_fib_index;
269 u8 is_add = 0, *ns_id = 0, secret_set = 0, sw_if_index_set = 0;
270 u8 *netns = 0, *sock_name = 0;
287 else if (
unformat (line_input,
"del"))
289 else if (
unformat (line_input,
"id %_%v%_", &ns_id))
291 else if (
unformat (line_input,
"secret %lu", &secret))
298 else if (
unformat (line_input,
"fib_id", &fib_id))
300 else if (
unformat (line_input,
"netns %_%v%_", &netns))
302 else if (
unformat (line_input,
"sock-name %_%v%_", &sock_name))
318 if (is_add && (!secret_set || !sw_if_index_set))
330 .sock_name = sock_name,
331 .ip4_fib_id = fib_id,
352 .short_help =
"app ns [add|del] id <namespace-id> secret <secret> "
353 "sw_if_index <sw_if_index> if <interface> [netns <ns>]",
364 s =
format (s,
"Application namespace [%u]\nid: %s\nsecret: %lu",
366 if (app_ns->sw_if_index != (
u32) ~0)
368 app_ns->sw_if_index);
370 s =
format (s,
"\nNetns: %s", app_ns->netns);
371 if (app_ns->sock_name)
372 s =
format (s,
"\nSocket: %s", app_ns->sock_name);
420 u8 *ns_id = 0, do_table = 0, had_input = 1, do_api = 0;
424 table_t table = {}, *t = &table;
436 if (
unformat (line_input,
"id %_%v%_", &ns_id))
438 else if (
unformat (line_input,
"api-clients"))
489 app_ns->sw_if_index);
510 .path =
"show app ns",
511 .short_help =
"show app ns [id <id> [api-clients]]",
#define FIB_PROTOCOL_MAX
Definition outside of enum so it does not need to be included in non-defaulted switch statements.
clib_file_main_t file_main
void app_namespace_free(app_namespace_t *app_ns)
const u8 * app_namespace_id_from_index(u32 index)
struct _vnet_app_namespace_add_del_args vnet_app_namespace_add_del_args_t
#define APP_NAMESPACE_INVALID_INDEX
u8 * format_app_namespace(u8 *s, va_list *args)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
static vnet_sw_interface_t * vnet_get_sw_interface_or_null(vnet_main_t *vnm, u32 sw_if_index)
void session_table_free(session_table_t *slt, u8 fib_proto)
struct _app_namespace app_namespace_t
#define clib_error_return(e, args...)
static clib_error_t * app_ns_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
int appns_sapi_add_ns_socket(app_namespace_t *app_ns)
#define hash_set_mem(h, key, value)
#define APP_INVALID_INDEX
#define pool_put(P, E)
Free an object E in pool P.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
void app_namespaces_init(void)
app_namespace_t * app_namespace_get(u32 index)
void application_namespace_cleanup(app_namespace_t *app_ns)
void session_lookup_show_table_entries(vlib_main_t *vm, session_table_t *table, u8 type, u8 is_local)
void session_table_init(session_table_t *slt, u8 fib_proto)
Initialize session table hash tables.
uword * app_namespace_lookup_table
Hash table of application namespaces by app ns ids.
#define pool_foreach(VAR, POOL)
Iterate through pool.
u32 app_namespace_get_fib_index(app_namespace_t *app_ns, u8 fib_proto)
u32 fib_table_get_table_id_for_sw_if_index(fib_protocol_t proto, u32 sw_if_index)
Get the Table-ID of the FIB bound to the interface.
static vlib_cli_command_t app_ns_command
(constructor) VLIB_CLI_COMMAND (app_ns_command)
#define hash_unset_mem(h, key)
#define vec_dup(V)
Return copy of vector (no header, no alignment)
vnet_main_t * vnet_get_main(void)
static app_namespace_t * app_namespace_pool
Pool of application namespaces.
u32 session_table_index(session_table_t *slt)
void appns_sapi_del_ns_socket(app_namespace_t *app_ns)
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
#define VLIB_CLI_COMMAND(x,...)
static clib_error_t * show_app_ns_fn(vlib_main_t *vm, unformat_input_t *main_input, vlib_cli_command_t *cmd)
app_namespace_t * app_namespace_alloc(const u8 *ns_id)
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static clib_file_t * clib_file_get(clib_file_main_t *fm, u32 file_index)
#define hash_create_vec(elts, key_bytes, value_bytes)
#define hash_get_mem(h, key)
#define vec_free(V)
Free vector's memory (no header).
static void app_namespace_show_api(vlib_main_t *vm, app_namespace_t *app_ns)
format_function_t format_vnet_sw_if_index_name
unformat_function_t unformat_vnet_sw_interface
description fragment has unexpected format
u32 app_namespace_index_from_id(const u8 *ns_id)
app_worker_t * app_worker_get(u32 wrk_index)
static vlib_cli_command_t show_app_ns_command
(constructor) VLIB_CLI_COMMAND (show_app_ns_command)
static uword pool_elts(void *v)
Number of active elements in a pool.
u32 app_namespace_index(app_namespace_t *app_ns)
void appns_sapi_enable(void)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
u32 wrk_map_index
Worker index in app's map pool.
#define vec_terminate_c_string(V)
(If necessary) NULL terminate a vector containing a c-string.
session_table_t * session_table_alloc(void)
u32 app_index
Index of owning app.
struct _socket_t clib_socket_t
void session_lookup_set_tables_appns(app_namespace_t *app_ns)
Mark (global) tables as pertaining to app ns.
int vnet_app_namespace_add_del(vnet_app_namespace_add_del_args_t *a)
#define session_cli_return_if_not_enabled()
u32 fib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
vl_api_interface_index_t sw_if_index
session_table_t * app_namespace_get_local_table(app_namespace_t *app_ns)
u8 appns_sapi_enabled(void)
struct _session_lookup_table session_table_t
app_namespace_t * app_namespace_get_from_id(const u8 *ns_id)
session_table_t * session_table_get(u32 table_index)
static u8 app_sapi_enabled
const u8 * app_namespace_id(app_namespace_t *app_ns)