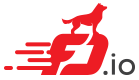 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
19 #ifndef __included_nat44_ed_h__
20 #define __included_nat44_ed_h__
40 #define NAT_FQ_NELTS_DEFAULT 64
43 #define SNAT_FLAG_HAIRPINNING (1 << 0)
46 #define foreach_nat44_config_flag \
47 _ (0x00, IS_ENDPOINT_INDEPENDENT) \
48 _ (0x01, IS_ENDPOINT_DEPENDENT) \
49 _ (0x02, IS_STATIC_MAPPING_ONLY) \
50 _ (0x04, IS_CONNECTION_TRACKING)
54 #define _(n,f) NAT44_API_##f = n,
95 #define foreach_nat_addr_and_port_alloc_alg \
96 _(0, DEFAULT, "default") \
98 _(2, RANGE, "port-range")
102 #define _(v, N, s) NAT_ADDR_AND_PORT_ALLOC_ALG_##N = v,
108 #define foreach_snat_session_state \
109 _(0, UNKNOWN, "unknown") \
110 _(1, UDP_ACTIVE, "udp-active") \
111 _(2, TCP_SYN_SENT, "tcp-syn-sent") \
112 _(3, TCP_ESTABLISHED, "tcp-established") \
113 _(4, TCP_FIN_WAIT, "tcp-fin-wait") \
114 _(5, TCP_CLOSE_WAIT, "tcp-close-wait") \
115 _(6, TCP_CLOSING, "tcp-closing") \
116 _(7, TCP_LAST_ACK, "tcp-last-ack") \
117 _(8, TCP_CLOSED, "tcp-closed") \
118 _(9, ICMP_ACTIVE, "icmp-active")
122 #define _(v, N, s) SNAT_SESSION_##N = v,
127 #define foreach_nat_in2out_ed_error \
128 _ (UNSUPPORTED_PROTOCOL, "unsupported protocol") \
129 _ (OUT_OF_PORTS, "out of ports") \
130 _ (BAD_ICMP_TYPE, "unsupported ICMP type") \
131 _ (MAX_SESSIONS_EXCEEDED, "maximum sessions exceeded") \
132 _ (NON_SYN, "non-SYN packet try to create session") \
133 _ (TCP_CLOSED, "drops due to TCP in transitory timeout") \
134 _ (TRNSL_FAILED, "couldn't translate packet")
138 #define _(sym,str) NAT_IN2OUT_ED_ERROR_##sym,
144 #define foreach_nat_out2in_ed_error \
145 _ (UNSUPPORTED_PROTOCOL, "unsupported protocol") \
146 _ (OUT_OF_PORTS, "out of ports") \
147 _ (BAD_ICMP_TYPE, "unsupported ICMP type") \
148 _ (NO_TRANSLATION, "no translation") \
149 _ (MAX_SESSIONS_EXCEEDED, "maximum sessions exceeded") \
150 _ (NON_SYN, "non-SYN packet try to create session") \
151 _ (TCP_CLOSED, "drops due to TCP in transitory timeout") \
152 _ (HASH_ADD_FAILED, "hash table add failed") \
153 _ (TRNSL_FAILED, "couldn't translate packet")
157 #define _(sym,str) NAT_OUT2IN_ED_ERROR_##sym,
165 #define NAT44_SES_I2O_FIN 1
166 #define NAT44_SES_O2I_FIN 2
167 #define NAT44_SES_I2O_FIN_ACK 4
168 #define NAT44_SES_O2I_FIN_ACK 8
169 #define NAT44_SES_I2O_SYN 16
170 #define NAT44_SES_O2I_SYN 32
171 #define NAT44_SES_RST 64
174 #define SNAT_SESSION_FLAG_STATIC_MAPPING (1 << 0)
175 #define SNAT_SESSION_FLAG_UNKNOWN_PROTO (1 << 1)
176 #define SNAT_SESSION_FLAG_LOAD_BALANCING (1 << 2)
177 #define SNAT_SESSION_FLAG_TWICE_NAT (1 << 3)
178 #define SNAT_SESSION_FLAG_ENDPOINT_DEPENDENT (1 << 4)
179 #define SNAT_SESSION_FLAG_FWD_BYPASS (1 << 5)
180 #define SNAT_SESSION_FLAG_AFFINITY (1 << 6)
181 #define SNAT_SESSION_FLAG_EXACT_ADDRESS (1 << 7)
182 #define SNAT_SESSION_FLAG_HAIRPINNING (1 << 8)
185 #define NAT_INTERFACE_FLAG_IS_INSIDE 1
186 #define NAT_INTERFACE_FLAG_IS_OUTSIDE 2
189 #define NAT_SM_FLAG_SELF_TWICE_NAT (1 << 1)
190 #define NAT_SM_FLAG_TWICE_NAT (1 << 2)
191 #define NAT_SM_FLAG_IDENTITY_NAT (1 << 3)
192 #define NAT_SM_FLAG_ADDR_ONLY (1 << 4)
193 #define NAT_SM_FLAG_EXACT_ADDRESS (1 << 5)
194 #define NAT_SM_FLAG_OUT2IN_ONLY (1 << 6)
195 #define NAT_SM_FLAG_LB (1 << 7)
196 #define NAT_SM_FLAG_SWITCH_ADDRESS (1 << 8)
208 }) per_vrf_sessions_t;
228 #define NAT_FLOW_OP_SADDR_REWRITE (1 << 1)
229 #define NAT_FLOW_OP_SPORT_REWRITE (1 << 2)
230 #define NAT_FLOW_OP_DADDR_REWRITE (1 << 3)
231 #define NAT_FLOW_OP_DPORT_REWRITE (1 << 4)
232 #define NAT_FLOW_OP_ICMP_ID_REWRITE (1 << 5)
233 #define NAT_FLOW_OP_TXFIB_REWRITE (1 << 6)
255 f->rewrite.saddr.as_u32 = saddr;
262 f->rewrite.daddr.as_u32 = daddr;
283 f->rewrite.fib_index = tx_fib_index;
290 f->rewrite.icmp_id =
id;
329 f64 ha_last_refreshed;
341 u16 ext_host_nat_port;
347 u64 tcp_closed_timestamp;
350 u32 per_vrf_sessions_index;
359 #define _(N, i, n, s) \
360 u32 busy_##n##_ports; \
361 u32 * busy_##n##_ports_per_thread; \
362 u32 busy_##n##_port_refcounts[65535];
497 u32 rx_fib_index,
u8 is_output);
499 u32 rx_fib_index,
u8 is_output);
511 u32 snat_thread_index);
618 #define _(x) vlib_simple_counter_main_t x;
820 return s->state == 0xf;
882 #define nat_log_err(...) \
883 vlib_log(VLIB_LOG_LEVEL_ERR, snat_main.log_class, __VA_ARGS__)
884 #define nat_log_warn(...) \
885 vlib_log(VLIB_LOG_LEVEL_WARNING, snat_main.log_class, __VA_ARGS__)
886 #define nat_log_notice(...) \
887 vlib_log(VLIB_LOG_LEVEL_NOTICE, snat_main.log_class, __VA_ARGS__)
888 #define nat_log_info(...) \
889 vlib_log(VLIB_LOG_LEVEL_INFO, snat_main.log_class, __VA_ARGS__)
890 #define nat_log_debug(...)\
891 vlib_log(VLIB_LOG_LEVEL_DEBUG, snat_main.log_class, __VA_ARGS__)
922 u8 *tag,
u32 affinity);
static bool nat44_ed_is_interface_outside(snat_interface_t *i)
Check if NAT interface is outside.
snat_static_map_resolve_t * to_resolve
static bool is_sm_lb(u32 f)
int nat44_ed_del_session(snat_main_t *sm, ip4_address_t *addr, u16 port, ip4_address_t *eh_addr, u16 eh_port, u8 proto, u32 vrf_id, int is_in)
Delete NAT44 endpoint-dependent session.
NAT port/address allocation lib.
static bool snat_is_unk_proto_session(snat_session_t *s)
Check if SNAT session for unknown protocol.
ip_lookup_main_t * ip4_lookup_main
unformat_function_t unformat_nat_protocol
static bool is_sm_switch_address(u32 f)
u32 unk_proto_lru_head_index
struct snat_main_s::@746::@747 fastpath
static bool tcp_flags_is_init(u8 f)
Check if client initiating TCP connection (received SYN from client)
#define SNAT_SESSION_FLAG_AFFINITY
static void nat_6t_flow_saddr_rewrite_set(nat_6t_flow_t *f, u32 saddr)
vlib_node_registration_t snat_in2out_output_worker_handoff_node
(constructor) VLIB_REGISTER_NODE (snat_in2out_output_worker_handoff_node)
enum nat44_config_flags_t_ nat44_config_flags_t
nat44_lb_addr_port_t * locals
u32 in2out_output_node_index
snat_static_mapping_t * static_mappings
snat_address_t * twice_nat_addresses
vl_api_ip_port_and_mask_t dst_port
format_function_t format_nat_protocol
vlib_simple_counter_main_t total_sessions
int nat44_plugin_disable()
void nat_free_session_data(snat_main_t *sm, snat_session_t *s, u32 thread_index, u8 is_ha)
Free NAT44 session data (lookup keys, external address port)
format_function_t format_snat_key
u32 nat_calc_bihash_buckets(u32 n_elts)
int nat44_ed_del_address(ip4_address_t addr, u8 delete_sm, u8 twice_nat)
#define SNAT_SESSION_FLAG_UNKNOWN_PROTO
struct snat_main_s::@746 counters
fib_source_t nat_fib_src_low
int snat_static_mapping_match(vlib_main_t *vm, snat_main_t *sm, ip4_address_t match_addr, u16 match_port, u32 match_fib_index, nat_protocol_t match_protocol, ip4_address_t *mapping_addr, u16 *mapping_port, u32 *mapping_fib_index, u8 by_external, u8 *is_addr_only, twice_nat_type_t *twice_nat, lb_nat_type_t *lb, ip4_address_t *ext_host_addr, u8 *is_identity_nat, snat_static_mapping_t **out)
Match NAT44 static mapping.
int nat44_ed_add_lb_static_mapping(ip4_address_t e_addr, u16 e_port, nat_protocol_t proto, nat44_lb_addr_port_t *locals, u32 flags, u8 *tag, u32 affinity)
u8 translation_memory_size_set
format_function_t format_nat_addr_and_port_alloc_alg
@ NAT_NEXT_IN2OUT_CLASSIFY
static bool nat44_ed_is_affinity_session(snat_session_t *s)
Check if NAT session has affinity record.
struct snat_main_s::@746::@748 slowpath
clib_error_t * nat44_api_hookup(vlib_main_t *vm)
#define foreach_nat44_config_flag
#define NAT_FLOW_OP_TXFIB_REWRITE
int snat_set_workers(uword *bitmap)
int nat44_ed_add_address(ip4_address_t *addr, u32 vrf_id, u8 twice_nat)
vlib_node_registration_t snat_out2in_node
int nat44_ed_add_static_mapping(ip4_address_t l_addr, ip4_address_t e_addr, u16 l_port, u16 e_port, nat_protocol_t proto, u32 vrf_id, u32 sw_if_index, u32 flags, ip4_address_t pool_addr, u8 *tag)
snat_interface_t * interfaces
vlib_node_registration_t snat_in2out_node
int nat44_ed_del_interface_address(u32 sw_if_index, u8 twice_nat)
int nat44_ed_add_output_interface(u32 sw_if_index)
#define NAT_SM_FLAG_ADDR_ONLY
vlib_log_class_t log_class
#define SNAT_SESSION_FLAG_STATIC_MAPPING
int nat44_ed_del_lb_static_mapping(ip4_address_t e_addr, u16 e_port, nat_protocol_t proto, u32 flags)
struct snat_main_s snat_main_t
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static bool is_sm_out2in_only(u32 f)
static bool nat44_ed_is_exact_address_session(snat_session_t *s)
Check if exact pool address should be used.
vl_api_dhcp_client_state_t state
@ NAT_NEXT_IN2OUT_ED_SLOW_PATH
static void nat_6t_flow_daddr_rewrite_set(nat_6t_flow_t *f, u32 daddr)
snat_address_t * addresses
typedef CLIB_PACKED(struct { u32 ses_count;u32 rx_fib_index;u32 tx_fib_index;u8 expired;}) per_vrf_sessions_t
clib_bihash_8_8_t static_mapping_by_external
void nat_6t_l3_l4_csum_calc(nat_6t_flow_t *f)
void snat_free_outside_address_and_port(snat_address_t *addresses, u32 thread_index, ip4_address_t *addr, u16 port, nat_protocol_t protocol)
Free outside address and port pair.
format_function_t format_snat_static_mapping
#define NAT_SM_FLAG_OUT2IN_ONLY
format_function_t format_static_mapping_key
#define SNAT_SESSION_FLAG_EXACT_ADDRESS
vlib_node_registration_t nat_pre_in2out_node
(constructor) VLIB_REGISTER_NODE (nat_pre_in2out_node)
#define NAT_SM_FLAG_IDENTITY_NAT
u8 * format_session_kvp(u8 *s, va_list *args)
u32 get_thread_idx_by_port(u16 e_port)
nat_translation_error_e nat_6t_flow_buf_translate_i2o(vlib_main_t *vm, snat_main_t *sm, vlib_buffer_t *b, ip4_header_t *ip, nat_6t_flow_t *f, nat_protocol_t proto, int is_output_feature)
u32 nat44_ed_get_out2in_worker_index(vlib_buffer_t *b, ip4_header_t *ip, u32 rx_fib_index, u8 is_output)
vlib_node_registration_t nat44_ed_in2out_output_node
(constructor) VLIB_REGISTER_NODE (nat44_ed_in2out_output_node)
nat_addr_and_port_alloc_alg_t addr_and_port_alloc_alg
int nat44_ed_del_interface(u32 sw_if_index, u8 is_inside)
int nat44_ed_del_output_interface(u32 sw_if_index)
static bool nat44_is_ses_closed(snat_session_t *s)
Check if NAT44 endpoint-dependent TCP session is closed.
static bool is_sm_twice_nat(u32 f)
vlib_node_registration_t snat_out2in_worker_handoff_node
(constructor) VLIB_REGISTER_NODE (snat_out2in_worker_handoff_node)
@ NAT_NEXT_IN2OUT_ED_OUTPUT_SLOW_PATH
nat_addr_and_port_alloc_alg_t
snat_main_per_thread_data_t * per_thread_data
void nat44_ed_forwarding_enable_disable(u8 is_enable)
@ NAT_ED_TRNSL_ERR_INVALID_CSUM
clib_bihash_16_8_t flow_hash
@ NAT_NEXT_IN2OUT_ED_FAST_PATH
static bool nat44_ed_is_lb_session(snat_session_t *s)
Check if NAT session is load-balancing.
per_vrf_sessions_t * per_vrf_sessions_vec
#define NAT_FLOW_OP_DADDR_REWRITE
nat_translation_error_e nat_6t_flow_buf_translate_o2i(vlib_main_t *vm, snat_main_t *sm, vlib_buffer_t *b, ip4_header_t *ip, nat_6t_flow_t *f, nat_protocol_t proto, int is_output_feature)
@ NAT_NEXT_OUT2IN_ED_FAST_PATH
#define NAT_INTERFACE_FLAG_IS_OUTSIDE
nat_outside_fib_t * outside_fibs
void nat44_ed_sessions_clear()
u32 affinity_per_service_list_head_index
int nat44_update_session_limit(u32 session_limit, u32 vrf_id)
Update NAT44 session limit flushing all data (session limit, vrf id)
#define foreach_snat_session_state
static bool is_sm_addr_only(u32 f)
vlib_node_registration_t snat_in2out_worker_handoff_node
(constructor) VLIB_REGISTER_NODE (snat_in2out_worker_handoff_node)
void expire_per_vrf_sessions(u32 fib_index)
u8 * format_static_mapping_kvp(u8 *s, va_list *args)
#define NAT_FLOW_OP_ICMP_ID_REWRITE
u32 fq_in2out_output_index
#define NAT_SM_FLAG_TWICE_NAT
#define NAT_SM_FLAG_EXACT_ADDRESS
static bool nat44_ed_is_session_static(snat_session_t *s)
Check if SNAT session is created from static mapping.
vl_api_ip_port_and_mask_t src_port
int nat44_ed_add_del_lb_static_mapping_local(ip4_address_t e_addr, u16 e_port, ip4_address_t l_addr, u16 l_port, nat_protocol_t proto, u32 vrf_id, u8 probability, u8 is_add)
static void nat_6t_flow_icmp_id_rewrite_set(nat_6t_flow_t *f, u16 id)
u32 nat44_ed_get_in2out_worker_index(vlib_buffer_t *b, ip4_header_t *ip, u32 rx_fib_index, u8 is_output)
format_function_t format_snat_static_map_to_resolve
#define NAT_INTERFACE_FLAG_IS_INSIDE
snat_session_t * sessions
@ NAT_ED_TRNSL_ERR_FLOW_MISMATCH
struct _vlib_node_registration vlib_node_registration_t
vlib_node_registration_t nat44_ed_out2in_node
(constructor) VLIB_REGISTER_NODE (nat44_ed_out2in_node)
STATIC_ASSERT_SIZEOF(nat_6t_t, 2 *sizeof(u64))
#define foreach_nat_in2out_ed_error
API main structure, used by both vpp and binary API clients.
static bool nat44_ed_is_twice_nat_session(snat_session_t *s)
Check if NAT session is twice NAT.
format_function_t format_nat_ed_translation_error
#define SNAT_SESSION_FLAG_TWICE_NAT
u32 tcp_trans_lru_head_index
vlib_node_registration_t nat_default_node
(constructor) VLIB_REGISTER_NODE (nat_default_node)
int nat44_ed_del_static_mapping(ip4_address_t l_addr, ip4_address_t e_addr, u16 l_port, u16 e_port, nat_protocol_t proto, u32 vrf_id, u32 sw_if_index, u32 flags)
#define SNAT_SESSION_FLAG_LOAD_BALANCING
#define NAT_FLOW_OP_DPORT_REWRITE
u32 * max_translations_per_fib
@ NAT_NEXT_IN2OUT_ED_OUTPUT_FAST_PATH
int nat44_set_session_limit(u32 session_limit, u32 vrf_id)
Set NAT44 session limit (session limit, vrf id)
static void nat_6t_flow_sport_rewrite_set(nat_6t_flow_t *f, u32 sport)
static bool is_sm_self_twice_nat(u32 f)
static bool nat44_ed_is_interface_inside(snat_interface_t *i)
Check if NAT interface is inside.
static bool is_sm_identity_nat(u32 f)
int nat44_ed_set_frame_queue_nelts(u32 frame_queue_nelts)
vl_api_ip_proto_t protocol
u32 max_cfg_sessions_gauge
@ NAT_ED_TRNSL_ERR_PACKET_TRUNCATED
u32 * auto_add_sw_if_indices
#define NAT_FLOW_OP_SADDR_REWRITE
clib_bihash_8_8_t static_mapping_by_local
format_function_t format_ed_session_kvp
u32 max_translations_per_thread
@ NAT_NEXT_OUT2IN_ED_SLOW_PATH
format_function_t format_nat_6t_flow
#define NAT_SM_FLAG_SWITCH_ADDRESS
@ NAT_ED_TRNSL_ERR_INNER_IP_CORRUPT
fib_source_t nat_fib_src_hi
@ NAT_NEXT_OUT2IN_CLASSIFY
int nat44_plugin_enable(nat44_config_t c)
A collection of simple counters.
#define NAT_SM_FLAG_SELF_TWICE_NAT
vlib_node_registration_t nat_pre_out2in_node
(constructor) VLIB_REGISTER_NODE (nat_pre_out2in_node)
vlib_simple_counter_main_t hairpinning
#define foreach_nat_addr_and_port_alloc_alg
ip4_address_t external_addr
#define NAT_FLOW_OP_SPORT_REWRITE
#define foreach_nat_out2in_ed_error
enum fib_source_t_ fib_source_t
The different sources that can create a route.
nat_alloc_out_addr_and_port_function_t * alloc_addr_and_port
int nat44_ed_add_interface(u32 sw_if_index, u8 is_inside)
vlib_node_registration_t nat44_ed_in2out_node
(constructor) VLIB_REGISTER_NODE (nat44_ed_in2out_node)
static bool is_sm_exact_address(u32 f)
vlib_node_registration_t snat_in2out_output_node
#define SNAT_SESSION_FLAG_FWD_BYPASS
uword * cached_presence_by_ip4_address
u32 tcp_estab_lru_head_index
vl_api_interface_index_t sw_if_index
void nat44_addresses_free(snat_address_t **addresses)
@ NAT_ED_TRNSL_ERR_SUCCESS
static void nat_6t_flow_dport_rewrite_set(nat_6t_flow_t *f, u32 dport)
@ NAT_ED_TRNSL_ERR_TRANSLATION_FAILED
int() nat_alloc_out_addr_and_port_function_t(snat_address_t *addresses, u32 fib_index, u32 thread_index, nat_protocol_t proto, ip4_address_t *addr, u16 *port, u16 port_per_thread, u32 snat_thread_index)
u8 static_mapping_connection_tracking
u32 * auto_add_sw_if_indices_twice_nat
format_function_t format_snat_session
static bool na44_ed_is_fwd_bypass_session(snat_session_t *s)
Check if NAT session is forwarding bypass.
static void nat_6t_flow_txfib_rewrite_set(nat_6t_flow_t *f, u32 tx_fib_index)
VLIB buffer representation.
snat_interface_t * output_feature_interfaces
int nat44_ed_add_interface_address(u32 sw_if_index, u8 twice_nat)
vl_api_wireguard_peer_flags_t flags