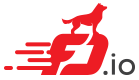 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
18 #include <vpp/app/version.h>
46 #define skip_if_disabled() \
49 snat_main_t *sm = &snat_main; \
50 if (PREDICT_FALSE (!sm->enabled)) \
55 #define fail_if_enabled() \
58 snat_main_t *sm = &snat_main; \
59 if (PREDICT_FALSE (sm->enabled)) \
61 nat_log_err ("plugin enabled"); \
67 #define fail_if_disabled() \
70 snat_main_t *sm = &snat_main; \
71 if (PREDICT_FALSE (!sm->enabled)) \
73 nat_log_err ("plugin disabled"); \
81 .arc_name =
"ip4-unicast",
82 .node_name =
"nat-pre-in2out",
84 "ip4-sv-reassembly-feature"),
87 .arc_name =
"ip4-unicast",
88 .node_name =
"nat-pre-out2in",
90 "ip4-dhcp-client-detect",
91 "ip4-sv-reassembly-feature"),
94 .arc_name =
"ip4-unicast",
95 .node_name =
"nat44-in2out-worker-handoff",
99 .arc_name =
"ip4-unicast",
100 .node_name =
"nat44-out2in-worker-handoff",
102 "ip4-dhcp-client-detect"),
105 .arc_name =
"ip4-unicast",
106 .node_name =
"nat44-in2out",
107 .runs_after =
VNET_FEATURES (
"acl-plugin-in-ip4-fa",
"ip4-sv-reassembly-feature"),
110 .arc_name =
"ip4-unicast",
111 .node_name =
"nat44-out2in",
112 .runs_after =
VNET_FEATURES (
"acl-plugin-in-ip4-fa",
"ip4-sv-reassembly-feature",
113 "ip4-dhcp-client-detect"),
116 .arc_name =
"ip4-unicast",
117 .node_name =
"nat44-ed-in2out",
118 .runs_after =
VNET_FEATURES (
"acl-plugin-in-ip4-fa",
"ip4-sv-reassembly-feature"),
121 .arc_name =
"ip4-unicast",
122 .node_name =
"nat44-ed-out2in",
123 .runs_after =
VNET_FEATURES (
"acl-plugin-in-ip4-fa",
"ip4-sv-reassembly-feature",
124 "ip4-dhcp-client-detect"),
127 .arc_name =
"ip4-unicast",
128 .node_name =
"nat44-ed-classify",
129 .runs_after =
VNET_FEATURES (
"acl-plugin-in-ip4-fa",
"ip4-sv-reassembly-feature"),
132 .arc_name =
"ip4-unicast",
133 .node_name =
"nat44-handoff-classify",
134 .runs_after =
VNET_FEATURES (
"acl-plugin-in-ip4-fa",
"ip4-sv-reassembly-feature"),
137 .arc_name =
"ip4-unicast",
138 .node_name =
"nat44-in2out-fast",
139 .runs_after =
VNET_FEATURES (
"acl-plugin-in-ip4-fa",
"ip4-sv-reassembly-feature"),
142 .arc_name =
"ip4-unicast",
143 .node_name =
"nat44-out2in-fast",
144 .runs_after =
VNET_FEATURES (
"acl-plugin-in-ip4-fa",
"ip4-sv-reassembly-feature",
145 "ip4-dhcp-client-detect"),
150 .arc_name =
"ip4-output",
151 .node_name =
"nat44-in2out-output",
152 .runs_after =
VNET_FEATURES (
"ip4-sv-reassembly-output-feature"),
156 .arc_name =
"ip4-output",
157 .node_name =
"nat44-in2out-output-worker-handoff",
158 .runs_after =
VNET_FEATURES (
"ip4-sv-reassembly-output-feature"),
162 .arc_name =
"ip4-output",
163 .node_name =
"nat-pre-in2out-output",
164 .runs_after =
VNET_FEATURES (
"ip4-sv-reassembly-output-feature"),
168 .arc_name =
"ip4-output",
169 .node_name =
"nat44-ed-in2out-output",
170 .runs_after =
VNET_FEATURES (
"ip4-sv-reassembly-output-feature"),
175 .version = VPP_BUILD_VER,
176 .description =
"Network Address Translation (NAT)",
202 s =
format (s,
"%U static-mapping-index %llu",
220 "local %U:%d remote %U:%d proto %U fib %d thread-index %u "
253 0, s->in2out.fib_index, &s->in2out.addr, s->in2out.port,
254 &s->ext_host_nat_addr, s->ext_host_nat_port, &s->out2in.addr,
255 s->out2in.port, &s->ext_host_addr, s->ext_host_port, s->nat_proto,
265 thread_index, s->in2out.addr.as_u32, s->out2in.addr.as_u32,
266 s->nat_proto, s->in2out.port, s->out2in.port, s->in2out.fib_index);
274 s->ext_host_nat_port, s->nat_proto);
281 &s->out2in.addr, s->out2in.port,
317 .ip4.as_u32 =
addr->as_u32,
398 return VNET_API_ERROR_UNSUPPORTED;
407 return VNET_API_ERROR_VALUE_EXIST;
429 #define _(N, i, n, s) \
430 clib_memset (ap->busy_##n##_port_refcounts, 0, \
431 sizeof (ap->busy_##n##_port_refcounts)); \
432 ap->busy_##n##_ports = 0; \
433 ap->busy_##n##_ports_per_thread = 0; \
434 vec_validate_init_empty (ap->busy_##n##_ports_per_thread, \
435 tm->n_vlib_mains - 1, 0);
454 u32 *ses_to_be_removed = 0, *ses_index;
461 for (j = 0; j <
vec_len (addresses); j++)
463 if (addresses[j].
addr.as_u32 ==
addr.as_u32)
472 return VNET_API_ERROR_NO_SUCH_ENTRY;
494 return VNET_API_ERROR_UNSPECIFIED;
498 if (
a->fib_index != ~0)
504 if (
a->busy_tcp_ports ||
a->busy_udp_ports ||
a->busy_icmp_ports)
510 if (ses->out2in.addr.as_u32 ==
addr.as_u32)
526 #define _(N, i, n, s) vec_free (a->busy_##n##_ports_per_thread);
559 u32 fib_index,
int addr_only,
563 u32 *indexes_to_free = NULL;
565 if (s->in2out.fib_index != fib_index ||
566 s->in2out.addr.as_u32 != l_addr.
as_u32)
572 if ((s->out2in.addr.as_u32 != e_addr.
as_u32) ||
573 s->out2in.port != e_port ||
574 s->in2out.port != l_port ||
609 if (
a->addr.as_u32 !=
addr.as_u32)
614 #define _(N, j, n, s) \
615 case NAT_PROTOCOL_##N: \
616 if (a->busy_##n##_port_refcounts[port]) \
618 ++a->busy_##n##_port_refcounts[port]; \
621 a->busy_##n##_ports++; \
622 a->busy_##n##_ports_per_thread[ti]++; \
650 if (
a->addr.as_u32 !=
addr.as_u32)
655 #define _(N, j, n, s) \
656 case NAT_PROTOCOL_##N: \
657 --a->busy_##n##_port_refcounts[port]; \
660 a->busy_##n##_ports--; \
661 a->busy_##n##_ports_per_thread[ti]--; \
769 return VNET_API_ERROR_UNSUPPORTED;
776 return VNET_API_ERROR_UNSUPPORTED;
797 return VNET_API_ERROR_UNSUPPORTED;
808 e_port = l_port =
proto = 0;
820 return VNET_API_ERROR_VALUE_EXIST;
851 return VNET_API_ERROR_VALUE_EXIST;
860 return VNET_API_ERROR_VALUE_EXIST;
897 return VNET_API_ERROR_VALUE_EXIST;
912 return VNET_API_ERROR_NO_SUCH_ENTRY;
998 return VNET_API_ERROR_UNSUPPORTED;
1009 e_port = l_port =
proto = 0;
1021 return VNET_API_ERROR_NO_SUCH_ENTRY;
1026 if (!first_int_addr)
1050 return VNET_API_ERROR_NO_SUCH_ENTRY;
1077 return VNET_API_ERROR_NO_SUCH_ENTRY;
1090 return VNET_API_ERROR_INVALID_VALUE;
1139 u8 *tag,
u32 affinity)
1153 return VNET_API_ERROR_UNSUPPORTED;
1168 return VNET_API_ERROR_VALUE_EXIST;
1173 return VNET_API_ERROR_INVALID_VALUE;
1188 #define _(N, j, n, s) \
1189 case NAT_PROTOCOL_##N: \
1190 if (a->busy_##n##_port_refcounts[e_port]) \
1191 return VNET_API_ERROR_INVALID_VALUE; \
1192 ++a->busy_##n##_port_refcounts[e_port]; \
1193 if (e_port > 1024) \
1195 a->busy_##n##_ports++; \
1196 a->busy_##n##_ports_per_thread[get_thread_idx_by_port (e_port)]++; \
1202 return VNET_API_ERROR_INVALID_VALUE_2;
1210 return VNET_API_ERROR_NO_SUCH_ENTRY;
1235 nat_elog_err (sm,
"static_mapping_by_external key add failed");
1236 return VNET_API_ERROR_UNSPECIFIED;
1246 locals[
i].fib_index, m->
proto, 0,
1258 .src_address = locals[
i].
addr,
1294 return VNET_API_ERROR_UNSUPPORTED;
1304 return VNET_API_ERROR_NO_SUCH_ENTRY;
1307 return VNET_API_ERROR_INVALID_VALUE;
1318 #define _(N, j, n, s) \
1319 case NAT_PROTOCOL_##N: \
1320 --a->busy_##n##_port_refcounts[e_port]; \
1321 if (e_port > 1024) \
1323 a->busy_##n##_ports--; \
1324 a->busy_##n##_ports_per_thread[get_thread_idx_by_port (e_port)]--; \
1330 return VNET_API_ERROR_INVALID_VALUE_2;
1340 nat_elog_err (sm,
"static_mapping_by_external key del failed");
1341 return VNET_API_ERROR_UNSPECIFIED;
1353 nat_elog_err (sm,
"static_mapping_by_local key del failed");
1354 return VNET_API_ERROR_UNSPECIFIED;
1361 .src_address = local->
addr,
1376 if ((s->in2out.addr.as_u32 != local->
addr.
as_u32) ||
1377 s->in2out.port != local->
port)
1416 return VNET_API_ERROR_UNSUPPORTED;
1425 return VNET_API_ERROR_NO_SUCH_ENTRY;
1430 return VNET_API_ERROR_INVALID_VALUE;
1438 match_local = local;
1447 return VNET_API_ERROR_VALUE_EXIST;
1453 local->
port = l_port;
1465 nat_elog_err (sm,
"static_mapping_by_local key add failed");
1471 return VNET_API_ERROR_NO_SUCH_ENTRY;
1474 return VNET_API_ERROR_UNSPECIFIED;
1483 nat_elog_err (sm,
"static_mapping_by_local key del failed");
1489 .src_address = local->
addr,
1503 if ((s->in2out.addr.as_u32 != match_local->
addr.
as_u32) ||
1504 s->in2out.port != match_local->
port)
1552 per_vrf_sessions_t *per_vrf_sessions;
1560 if ((per_vrf_sessions->rx_fib_index == fib_index) ||
1561 (per_vrf_sessions->tx_fib_index == fib_index))
1563 per_vrf_sessions->expired = 1;
1612 if (
f->fib_index == fib_index)
1637 const char *del_feature_name, *feature_name;
1648 return VNET_API_ERROR_UNSUPPORTED;
1653 nat_log_err (
"error interface already configured");
1654 return VNET_API_ERROR_VALUE_EXIST;
1667 del_feature_name = !is_inside ?
"nat44-in2out-worker-handoff" :
1668 "nat44-out2in-worker-handoff";
1669 feature_name =
"nat44-handoff-classify";
1673 del_feature_name = !is_inside ?
"nat-pre-in2out" :
"nat-pre-out2in";
1675 feature_name =
"nat44-ed-classify";
1690 feature_name = is_inside ?
"nat44-in2out-worker-handoff" :
1691 "nat44-out2in-worker-handoff";
1695 feature_name = is_inside ?
"nat-pre-in2out" :
"nat-pre-out2in";
1745 const char *del_feature_name, *feature_name;
1756 return VNET_API_ERROR_UNSUPPORTED;
1762 nat_log_err (
"error interface couldn't be found");
1763 return VNET_API_ERROR_NO_SUCH_ENTRY;
1770 del_feature_name =
"nat44-handoff-classify";
1771 feature_name = !is_inside ?
"nat44-in2out-worker-handoff" :
1772 "nat44-out2in-worker-handoff";
1776 del_feature_name =
"nat44-ed-classify";
1777 feature_name = !is_inside ?
"nat-pre-in2out" :
"nat-pre-out2in";
1803 feature_name = is_inside ?
"nat44-in2out-worker-handoff" :
1804 "nat44-out2in-worker-handoff";
1808 feature_name = is_inside ?
"nat-pre-in2out" :
"nat-pre-out2in";
1860 return VNET_API_ERROR_UNSUPPORTED;
1865 nat_log_err (
"error interface already configured");
1866 return VNET_API_ERROR_VALUE_EXIST;
1871 nat_log_err (
"error interface already configured");
1872 return VNET_API_ERROR_VALUE_EXIST;
1890 "ip4-unicast",
"nat44-out2in-worker-handoff",
sw_if_index, 1, 0, 0);
1892 "nat44-in2out-output-worker-handoff",
1958 return VNET_API_ERROR_UNSUPPORTED;
1964 nat_log_err (
"error interface couldn't be found");
1965 return VNET_API_ERROR_NO_SUCH_ENTRY;
1983 "ip4-unicast",
"nat44-out2in-worker-handoff",
sw_if_index, 0, 0, 0);
1985 "nat44-in2out-output-worker-handoff",
2038 return VNET_API_ERROR_FEATURE_DISABLED;
2041 return VNET_API_ERROR_INVALID_WORKER;
2068 u32 new_fib_index,
u32 old_fib_index)
2076 if (!sm->
enabled || (new_fib_index == old_fib_index)
2107 if (outside_fib->
fib_index == old_fib_index)
2118 if (outside_fib->
fib_index == new_fib_index)
2140 u32 address_length,
u32 if_address_index,
u32 is_delete);
2144 u32 address_length,
u32 if_address_index,
u32 is_delete);
2150 l_addr.
as_u8[0] = 1;
2151 l_addr.
as_u8[1] = 1;
2152 l_addr.
as_u8[2] = 1;
2153 l_addr.
as_u8[3] = 1;
2155 r_addr.
as_u8[0] = 2;
2156 r_addr.
as_u8[1] = 2;
2157 r_addr.
as_u8[2] = 2;
2158 r_addr.
as_u8[3] = 2;
2162 u32 fib_index = 9000001;
2164 u32 session_index = 3000000221;
2176 split_ed_kv (&kv, &l_addr2, &r_addr2, &proto2, &fib_index2, &l_port2,
2180 ASSERT (l_port == l_port2);
2181 ASSERT (r_port == r_port2);
2183 ASSERT (fib_index == fib_index2);
2193 ASSERT (l_port == l_port2);
2195 ASSERT (fib_index == fib_index2);
2205 if (fib_index != ~0)
2230 #define nat_validate_simple_counter(c, i) \
2233 vlib_validate_simple_counter (&c, i); \
2234 vlib_zero_simple_counter (&c, i); \
2238 #define nat_init_simple_counter(c, n, sn) \
2242 c.stat_segment_name = sn; \
2243 nat_validate_simple_counter (c, 0); \
2251 nat_validate_simple_counter (sm->counters.fastpath.in2out.x, sw_if_index); \
2252 nat_validate_simple_counter (sm->counters.fastpath.out2in.x, sw_if_index); \
2253 nat_validate_simple_counter (sm->counters.slowpath.in2out.x, sw_if_index); \
2254 nat_validate_simple_counter (sm->counters.slowpath.out2in.x, sw_if_index);
2268 u32 i, num_threads = 0;
2269 uword *p, *bitmap = 0;
2293 "/nat44-ed/total-sessions");
2298 nat_init_simple_counter (sm->counters.fastpath.in2out.x, #x, \
2299 "/nat44-ed/in2out/fastpath/" #x); \
2300 nat_init_simple_counter (sm->counters.fastpath.out2in.x, #x, \
2301 "/nat44-ed/out2in/fastpath/" #x); \
2302 nat_init_simple_counter (sm->counters.slowpath.in2out.x, #x, \
2303 "/nat44-ed/in2out/slowpath/" #x); \
2304 nat_init_simple_counter (sm->counters.slowpath.out2in.x, #x, \
2305 "/nat44-ed/out2in/slowpath/" #x);
2309 "/nat44-ed/hairpinning");
2370 if (
c.static_mapping_only && !
c.connection_tracking)
2372 nat_log_err (
"unsupported combination of configuration");
2381 sm->
pat = (!
c.static_mapping_only ||
2382 (
c.static_mapping_only &&
c.connection_tracking));
2385 c.sessions = 63 * 1024;
2447 #define _(N, i, n, s) \
2448 vec_free (ap->busy_##n##_ports_per_thread);
2478 nat_log_err (
"error occurred while removing interface %u",
2492 nat_log_err (
"error occurred while removing interface %u",
2530 u32 *ses_to_be_removed = 0, *ses_index;
2534 if (!sm->
enabled || is_enable)
2567 u16 port_host_byte_order = clib_net_to_host_u16 (
port);
2569 for (address_index = 0; address_index <
vec_len (addresses);
2572 if (addresses[address_index].
addr.as_u32 ==
addr->as_u32)
2578 a = addresses + address_index;
2582 #define _(N, i, n, s) \
2583 case NAT_PROTOCOL_##N: \
2584 ASSERT (a->busy_##n##_port_refcounts[port_host_byte_order] >= 1); \
2585 --a->busy_##n##_port_refcounts[port_host_byte_order]; \
2586 a->busy_##n##_ports--; \
2587 a->busy_##n##_ports_per_thread[thread_index]--; \
2601 u32 *mapping_fib_index,
u8 by_external,
2607 clib_bihash_8_8_t *mapping_hash;
2616 init_nat_k (&kv, match_addr, match_port, match_fib_index,
2618 if (clib_bihash_search_8_8 (mapping_hash, &kv, &
value))
2621 init_nat_k (&kv, match_addr, 0, match_fib_index, 0);
2622 if (clib_bihash_search_8_8 (mapping_hash, &kv, &
value))
2629 init_nat_k (&kv, match_addr, match_port, 0, match_protocol);
2630 if (clib_bihash_search_8_8 (mapping_hash, &kv, &
value))
2634 if (clib_bihash_search_8_8 (mapping_hash, &kv, &
value))
2648 vm, ext_host_addr[0], match_addr,
2649 match_protocol, match_port, &backend_index))
2652 *mapping_addr = local->
addr;
2653 *mapping_port = local->
port;
2666 .src_address = local->
addr,
2689 mid = ((
hi -
lo) >> 1) +
lo;
2691 (rand > local->
prefix) ? (
lo = mid + 1) : (
hi = mid);
2694 if (!(local->
prefix >= rand))
2696 *mapping_addr = local->
addr;
2697 *mapping_port = local->
port;
2702 match_protocol, match_port,
2758 u32 rx_fib_index,
u8 is_output)
2766 u32 fib_index = rx_fib_index;
2778 .ip4.as_u32 =
ip->dst_address.as_u32,
2810 u16 lookup_sport, lookup_dport;
2814 b,
ip, &lookup_saddr, &lookup_sport, &lookup_daddr,
2815 &lookup_dport, &lookup_protocol))
2817 init_ed_k (&kv16, lookup_saddr, lookup_sport, lookup_daddr,
2818 lookup_dport, rx_fib_index, lookup_protocol);
2819 if (!clib_bihash_search_16_8 (&sm->
flow_hash, &kv16, &value16))
2831 fib_index,
ip->protocol);
2833 if (!clib_bihash_search_16_8 (&sm->
flow_hash, &kv16, &value16))
2844 rx_fib_index,
ip->protocol);
2845 if (!clib_bihash_search_16_8 (&sm->
flow_hash, &kv16, &value16))
2854 hash =
ip->src_address.as_u32 + (
ip->src_address.as_u32 >> 8) +
2855 (
ip->src_address.as_u32 >> 16) + (
ip->src_address.as_u32 >> 24);
2858 next_worker_index += sm->
workers[hash & (_vec_len (sm->
workers) - 1)];
2867 clib_net_to_host_u32 (
ip->src_address.as_u32),
2868 clib_net_to_host_u32 (
ip->dst_address.as_u32));
2873 next_worker_index, rx_fib_index,
2874 clib_net_to_host_u32 (
ip->src_address.as_u32),
2875 clib_net_to_host_u32 (
ip->dst_address.as_u32));
2878 return next_worker_index;
2883 u32 rx_fib_index,
u8 is_output)
2899 u16 lookup_sport, lookup_dport;
2902 b,
ip, &lookup_saddr, &lookup_sport, &lookup_daddr, &lookup_dport,
2905 init_ed_k (&kv16, lookup_saddr, lookup_sport, lookup_daddr,
2906 lookup_dport, rx_fib_index, lookup_protocol);
2908 !clib_bihash_search_16_8 (&sm->
flow_hash, &kv16, &value16)))
2912 sm,
"HANDOFF OUT2IN (session)", next_worker_index,
2913 rx_fib_index, clib_net_to_host_u32 (
ip->src_address.as_u32),
2914 clib_net_to_host_u32 (
ip->dst_address.as_u32));
2915 return next_worker_index;
2922 rx_fib_index,
ip->protocol);
2925 !clib_bihash_search_16_8 (&sm->
flow_hash, &kv16, &value16)))
2931 next_worker_index, rx_fib_index,
2932 clib_net_to_host_u32 (
ip->src_address.as_u32),
2933 clib_net_to_host_u32 (
ip->dst_address.as_u32));
2934 return next_worker_index;
2941 if (!clib_bihash_search_8_8
2945 next_worker_index = m->
workers[0];
2963 icmp46_header_t *
icmp = (icmp46_header_t *) udp;
2976 case NAT_PROTOCOL_ICMP:
2977 icmp = (icmp46_header_t *) l4_header;
2981 case NAT_PROTOCOL_UDP:
2982 case NAT_PROTOCOL_TCP:
2996 if (!clib_bihash_search_8_8
3002 next_worker_index = m->
workers[0];
3006 hash =
ip->src_address.as_u32 + (
ip->src_address.as_u32 >> 8) +
3007 (
ip->src_address.as_u32 >> 16) + (
ip->src_address.as_u32 >> 24);
3020 next_worker_index +=
3026 clib_net_to_host_u32 (
ip->src_address.as_u32),
3027 clib_net_to_host_u32 (
ip->dst_address.as_u32));
3028 return next_worker_index;
3035 u32 max_limit = 0,
len = 0;
3039 if (max_limit < sm->max_translations_per_fib[
len])
3052 if (
len <= fib_index)
3085 u32 translation_buckets)
3118 clib_bihash_init_16_8 (
3129 u32 static_mapping_buckets = 1024;
3130 u32 static_mapping_memory_size = 64 << 20;
3135 "static_mapping_by_local", static_mapping_buckets,
3136 static_mapping_memory_size);
3141 "static_mapping_by_external", static_mapping_buckets,
3142 static_mapping_memory_size);
3208 u32 address_length,
u32 if_address_index,
u32 is_delete)
3214 int i,
rv = 0, match = 0;
3292 u32 if_address_index,
u32 is_delete)
3367 u32 *auto_add_sw_if_indices = twice_nat ?
3372 for (
i = 0;
i <
vec_len (auto_add_sw_if_indices);
i++)
3376 return VNET_API_ERROR_VALUE_EXIST;
3407 u32 *indices_to_delete = 0;
3409 u32 *auto_add_sw_if_indices;
3413 return VNET_API_ERROR_UNSUPPORTED;
3419 for (
i = 0;
i <
vec_len (auto_add_sw_if_indices);
i++)
3440 if (
vec_len (indices_to_delete))
3442 for (j =
vec_len (indices_to_delete) - 1; j >= 0; j--)
3461 return VNET_API_ERROR_NO_SUCH_ENTRY;
3477 return VNET_API_ERROR_UNSUPPORTED;
3481 ip.dst_address.as_u32 =
ip.src_address.as_u32 =
addr->as_u32;
3492 return VNET_API_ERROR_NO_SUCH_ENTRY;
3496 return VNET_API_ERROR_UNSPECIFIED;
3511 .name =
"nat-default",
3512 .vector_size =
sizeof (
u32),
3534 f->l3_csum_delta = 0;
3535 f->l4_csum_delta = 0;
3537 f->rewrite.saddr.as_u32 !=
f->match.saddr.as_u32)
3546 f->rewrite.saddr.as_u32 =
f->match.saddr.as_u32;
3549 f->rewrite.daddr.as_u32 !=
f->match.daddr.as_u32)
3558 f->rewrite.daddr.as_u32 =
f->match.daddr.as_u32;
3567 f->rewrite.sport =
f->match.sport;
3576 f->rewrite.dport =
f->match.dport;
3579 f->rewrite.icmp_id !=
f->match.sport)
3587 f->rewrite.icmp_id =
f->match.sport;
3594 f->rewrite.fib_index =
f->match.fib_index;
3605 int is_icmp_inner_ip4,
int skip_saddr_rewrite)
3610 if ((NAT_PROTOCOL_TCP ==
proto || NAT_PROTOCOL_UDP ==
proto) &&
3613 if (!is_icmp_inner_ip4)
3615 ip->src_address =
f->rewrite.saddr;
3616 ip->dst_address =
f->rewrite.daddr;
3622 ip->src_address =
f->rewrite.daddr;
3623 ip->dst_address =
f->rewrite.saddr;
3628 if (NAT_PROTOCOL_TCP ==
proto)
3646 if (!is_icmp_inner_ip4)
3648 if (!skip_saddr_rewrite)
3650 ip->src_address =
f->rewrite.saddr;
3652 ip->dst_address =
f->rewrite.daddr;
3656 ip->src_address =
f->rewrite.daddr;
3657 ip->dst_address =
f->rewrite.saddr;
3661 if (skip_saddr_rewrite)
3671 if (0xffff ==
ip->checksum)
3679 int result = ((
u8 *)
object +
size <=
3689 if (IP_PROTOCOL_ICMP !=
ip->protocol)
3745 switch (inner_proto)
3747 case NAT_PROTOCOL_UDP:
3766 icmp->checksum = new_icmp_sum;
3768 case NAT_PROTOCOL_TCP:
3774 old_tcp_sum = tcp->checksum;
3787 icmp->checksum = new_icmp_sum;
3789 case NAT_PROTOCOL_ICMP:
3793 if (!
it_fits (
vm,
b, inner_icmp,
sizeof (*inner_icmp)))
3806 ip_csum_t inner_sum = inner_icmp->checksum;
3816 clib_warning (
"unexpected NAT protocol value `%d'", inner_proto);
3836 if (NAT_PROTOCOL_ICMP ==
proto)
3838 if (
ip->src_address.as_u32 !=
f->rewrite.saddr.as_u32)
3865 int is_output_feature)
3875 int is_output_feature)
3886 s =
format (s,
"saddr %U sport %u daddr %U dport %u proto %U fib_idx %u",
3902 s =
format (s,
"success");
3905 s =
format (s,
"translation-failed");
3908 s =
format (s,
"flow-mismatch");
3911 s =
format (s,
"packet-truncated");
3914 s =
format (s,
"inner-ip-corrupted");
3917 s =
format (s,
"invalid-checksum");
3933 f->rewrite.saddr.as_u8);
3940 s =
format (s,
"rewrite: ");
3943 s =
format (s,
"sport %u ", clib_net_to_host_u16 (
f->rewrite.sport));
3949 s =
format (s,
"rewrite: ");
3958 s =
format (s,
"rewrite: ");
3961 s =
format (s,
"dport %u ", clib_net_to_host_u16 (
f->rewrite.dport));
3967 s =
format (s,
"rewrite: ");
3970 s =
format (s,
"icmp-id %u ", clib_net_to_host_u16 (
f->rewrite.icmp_id));
3976 s =
format (s,
"rewrite: ");
3979 s =
format (s,
"txfib %u ",
f->rewrite.fib_index);
static bool nat44_ed_is_interface_outside(snat_interface_t *i)
Check if NAT interface is outside.
snat_static_map_resolve_t * to_resolve
static bool is_sm_lb(u32 f)
static void nat44_ed_worker_db_free(snat_main_per_thread_data_t *tsm)
static u32 ed_value_get_thread_index(clib_bihash_kv_16_8_t *value)
static bool snat_is_unk_proto_session(snat_session_t *s)
Check if SNAT session for unknown protocol.
ip_lookup_main_t * ip4_lookup_main
void nat44_ed_sessions_clear()
static void clib_dlist_init(dlist_elt_t *pool, u32 index)
static u32 nat_value_get_thread_index(clib_bihash_kv_8_8_t *value)
static bool is_sm_switch_address(u32 f)
int snat_set_workers(uword *bitmap)
int nat44_update_session_limit(u32 session_limit, u32 vrf_id)
Update NAT44 session limit flushing all data (session limit, vrf id)
vnet_interface_main_t * im
u32 unk_proto_lru_head_index
#define nat_elog_debug_handoff(_pm, _str, _tid, _fib, _src, _dst)
int nat44_ed_del_static_mapping(ip4_address_t l_addr, ip4_address_t e_addr, u16 l_port, u16 e_port, nat_protocol_t proto, u32 vrf_id, u32 sw_if_index, u32 flags)
nat44_lb_addr_port_t * locals
u32 in2out_output_node_index
u32 nat_affinity_get_per_service_list_head_index(void)
Get new affinity per service list head index.
int nat44_ed_add_output_interface(u32 sw_if_index)
static u32 ed_value_get_session_index(clib_bihash_kv_16_8_t *value)
snat_static_mapping_t * static_mappings
snat_address_t * twice_nat_addresses
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
static void split_ed_kv(clib_bihash_kv_16_8_t *kv, ip4_address_t *l_addr, ip4_address_t *r_addr, u8 *proto, u32 *fib_index, u16 *l_port, u16 *r_port)
vlib_simple_counter_main_t total_sessions
ip_lookup_main_t lookup_main
format_function_t format_snat_key
#define nat_elog_warn(_pm, nat_elog_str)
void fib_table_entry_delete(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source)
Delete a FIB entry.
struct snat_main_s::@746 counters
static clib_error_t * nat_ip_table_add_del(vnet_main_t *vnm, u32 table_id, u32 is_add)
u8 * format_nat_6t_flow(u8 *s, va_list *args)
ip4_table_bind_callback_t * table_bind_callbacks
Functions to call when interface to table biding changes.
ip4_main_t ip4_main
Global ip4 main structure.
fib_node_index_t fib_table_entry_update_one_path(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, dpo_proto_t next_hop_proto, const ip46_address_t *next_hop, u32 next_hop_sw_if_index, u32 next_hop_fib_index, u32 next_hop_weight, fib_mpls_label_t *next_hop_labels, fib_route_path_flags_t path_flags)
Update the entry to have just one path.
static_always_inline nat_outside_fib_t * nat44_ed_get_outside_fib(nat_outside_fib_t *outside_fibs, u32 fib_index)
int nat44_ed_add_static_mapping(ip4_address_t l_addr, ip4_address_t e_addr, u16 l_port, u16 e_port, nat_protocol_t proto, u32 vrf_id, u32 sw_if_index, u32 flags, ip4_address_t pool_addr, u8 *tag)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
#define nat_validate_simple_counter(c, i)
@ NAT_NEXT_IN2OUT_CLASSIFY
void nat_free_session_data(snat_main_t *sm, snat_session_t *s, u32 thread_index, u8 is_ha)
Free NAT44 session data (lookup keys, external address port)
static_always_inline int nat_get_icmp_session_lookup_values(vlib_buffer_t *b, ip4_header_t *ip0, ip4_address_t *lookup_saddr, u16 *lookup_sport, ip4_address_t *lookup_daddr, u16 *lookup_dport, u8 *lookup_protocol)
static bool nat44_ed_is_affinity_session(snat_session_t *s)
Check if NAT session has affinity record.
void snat_free_outside_address_and_port(snat_address_t *addresses, u32 thread_index, ip4_address_t *addr, u16 port, nat_protocol_t protocol)
Free outside address and port pair.
int nat44_ed_del_session(snat_main_t *sm, ip4_address_t *addr, u16 port, ip4_address_t *eh_addr, u16 eh_port, u8 proto, u32 vrf_id, int is_in)
Delete NAT44 endpoint-dependent session.
struct _tcp_header tcp_header_t
static uword ip4_header_checksum_is_valid(ip4_header_t *i)
static nat_protocol_t ip_proto_to_nat_proto(u8 ip_proto)
Common NAT inline functions.
clib_error_t * nat44_api_hookup(vlib_main_t *vm)
#define NAT_FLOW_OP_TXFIB_REWRITE
@ VLIB_NODE_TYPE_INTERNAL
vlib_log_class_t vlib_log_register_class(char *class, char *subclass)
vlib_main_t vlib_node_runtime_t * node
snat_interface_t * interfaces
static void nat44_ed_add_del_addr_to_fib(ip4_address_t *addr, u8 p_len, u32 sw_if_index, int is_add)
static void reinit_ed_flow_hash()
u32 nat44_ed_get_out2in_worker_index(vlib_buffer_t *b, ip4_header_t *ip, u32 rx_fib_index, u8 is_output)
#define FIB_NODE_INDEX_INVALID
vlib_log_class_t log_class
nat_translation_error_e nat_6t_flow_buf_translate_o2i(vlib_main_t *vm, snat_main_t *sm, vlib_buffer_t *b, ip4_header_t *ip, nat_6t_flow_t *f, nat_protocol_t proto, int is_output_feature)
u32 ip4_fib_table_get_index_for_sw_if_index(u32 sw_if_index)
static_always_inline void per_vrf_sessions_unregister_session(snat_session_t *s, u32 thread_index)
#define nat_elog_err(_pm, nat_elog_str)
#define pool_put(P, E)
Free an object E in pool P.
#define NAT_FQ_NELTS_DEFAULT
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static_always_inline void nat44_ed_add_del_addr_to_fib_foreach_addr(u32 sw_if_index, u8 is_add)
static bool is_sm_out2in_only(u32 f)
fib_node_index_t fib_table_lookup(u32 fib_index, const fib_prefix_t *prefix)
Perfom a longest prefix match in the non-forwarding table.
@ NAT_NEXT_IN2OUT_ED_SLOW_PATH
NAT port/address allocation lib.
void nat44_ed_forwarding_enable_disable(u8 is_enable)
int snat_static_mapping_match(vlib_main_t *vm, snat_main_t *sm, ip4_address_t match_addr, u16 match_port, u32 match_fib_index, nat_protocol_t match_protocol, ip4_address_t *mapping_addr, u16 *mapping_port, u32 *mapping_fib_index, u8 by_external, u8 *is_addr_only, twice_nat_type_t *twice_nat, lb_nat_type_t *lb, ip4_address_t *ext_host_addr, u8 *is_identity_nat, snat_static_mapping_t **out)
Match NAT44 static mapping.
static void nat44_ed_add_del_addr_to_fib_foreach_out_if(ip4_address_t *addr, u8 is_add)
int nat44_ed_add_lb_static_mapping(ip4_address_t e_addr, u16 e_port, nat_protocol_t proto, nat44_lb_addr_port_t *locals, u32 flags, u8 *tag, u32 affinity)
snat_address_t * addresses
@ FIB_ENTRY_FLAG_EXCLUSIVE
u32 get_thread_idx_by_port(u16 e_port)
vnet_hw_if_output_node_runtime_t * r
static_always_inline u8 icmp_type_is_error_message(u8 icmp_type)
clib_bihash_8_8_t static_mapping_by_external
int nat44_ed_get_resolve_record(ip4_address_t l_addr, u16 l_port, u16 e_port, nat_protocol_t proto, u32 vrf_id, u32 sw_if_index, u32 flags, int *out)
int ip4_sv_reass_enable_disable_with_refcnt(u32 sw_if_index, int is_enable)
static void nat44_ed_add_del_static_mapping_addr_only_cb(ip4_main_t *im, uword opaque, u32 sw_if_index, ip4_address_t *address, u32 address_length, u32 if_address_index, u32 is_delete)
int nat44_ed_add_interface(u32 sw_if_index, u8 is_inside)
int nat44_ed_del_resolve_record(ip4_address_t l_addr, u16 l_port, u16 e_port, nat_protocol_t proto, u32 vrf_id, u32 sw_if_index, u32 flags)
void nat_ipfix_logging_init(vlib_main_t *vm)
Initialize NAT plugin IPFIX logging.
static void nat44_ed_add_del_interface_address_cb(ip4_main_t *im, uword opaque, u32 sw_if_index, ip4_address_t *address, u32 address_length, u32 if_address_index, u32 is_delete)
static u32 random_u32(u32 *seed)
32-bit random number generator
u32 vlib_frame_queue_main_init(u32 node_index, u32 frame_queue_nelts)
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
int nat44_ed_reserve_port(ip4_address_t addr, u16 port, nat_protocol_t proto)
static clib_error_t * nat_init(vlib_main_t *vm)
static bool is_sm_twice_nat(u32 f)
#define pool_foreach(VAR, POOL)
Iterate through pool.
@ NAT_NEXT_IN2OUT_ED_OUTPUT_SLOW_PATH
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
snat_main_per_thread_data_t * per_thread_data
static_always_inline void nat44_ed_add_del_addr_to_fib_foreach_addr_only_sm(u32 sw_if_index, u8 is_add)
IPv4 shallow virtual reassembly.
@ NAT_ED_TRNSL_ERR_INVALID_CSUM
#define VLIB_NODE_FN(node)
int nat44_ed_del_output_interface(u32 sw_if_index)
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
clib_bihash_16_8_t flow_hash
static u32 nat_value_get_session_index(clib_bihash_kv_8_8_t *value)
int nat44_ed_del_interface_address(u32 sw_if_index, u8 twice_nat)
#define vec_dup(V)
Return copy of vector (no header, no alignment)
@ NAT_NEXT_IN2OUT_ED_FAST_PATH
void nat_6t_l3_l4_csum_calc(nat_6t_flow_t *f)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
vnet_main_t * vnet_get_main(void)
static void init_nat_kv(clib_bihash_kv_8_8_t *kv, ip4_address_t addr, u16 port, u32 fib_index, nat_protocol_t proto, u32 thread_index, u32 session_index)
void expire_per_vrf_sessions(u32 fib_index)
static bool nat44_ed_is_lb_session(snat_session_t *s)
Check if NAT session is load-balancing.
per_vrf_sessions_t * per_vrf_sessions_vec
u32 fib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, fib_source_t src)
Get the index of the FIB for a Table-ID.
static ip_csum_t ip_incremental_checksum(ip_csum_t sum, void *_data, uword n_bytes)
#define NAT_FLOW_OP_DADDR_REWRITE
static_always_inline uword vlib_get_thread_index(void)
@ NAT_NEXT_OUT2IN_ED_FAST_PATH
#define NAT_INTERFACE_FLAG_IS_OUTSIDE
nat_outside_fib_t * outside_fibs
u8 * format_session_kvp(u8 *s, va_list *args)
u32 affinity_per_service_list_head_index
f64 end
end of the time range
static bool is_sm_addr_only(u32 f)
static int vlib_object_within_buffer_data(vlib_main_t *vm, vlib_buffer_t *b, void *obj, size_t len)
#define static_always_inline
u32 fib_node_index_t
A typedef of a node index.
#define clib_bitmap_free(v)
Free a bitmap.
#define NAT_FLOW_OP_ICMP_ID_REWRITE
#define fail_if_enabled()
u32 fq_in2out_output_index
uword * thread_registrations_by_name
static void init_ed_k(clib_bihash_kv_16_8_t *kv, ip4_address_t l_addr, u16 l_port, ip4_address_t r_addr, u16 r_port, u32 fib_index, u8 proto)
static_always_inline int nat_6t_flow_icmp_translate(vlib_main_t *vm, snat_main_t *sm, vlib_buffer_t *b, ip4_header_t *ip, nat_6t_flow_t *f)
static bool nat44_ed_is_session_static(snat_session_t *s)
Check if SNAT session is created from static mapping.
static api_main_t * vlibapi_get_main(void)
static_always_inline int is_sw_if_index_reg_for_auto_resolve(u32 *sw_if_indices, u32 sw_if_index)
u8 * format_static_mapping_kvp(u8 *s, va_list *args)
static void nat44_ed_update_outside_fib_cb(ip4_main_t *im, uword opaque, u32 sw_if_index, u32 new_fib_index, u32 old_fib_index)
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
int ip4_sv_reass_output_enable_disable_with_refcnt(u32 sw_if_index, int is_enable)
int nat44_set_session_limit(u32 session_limit, u32 vrf_id)
Set NAT44 session limit (session limit, vrf id)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
manual_print typedef address
int nat_affinity_create_and_lock(ip4_address_t client_addr, ip4_address_t service_addr, u8 proto, u16 service_port, u8 backend_index, u32 sticky_time, u32 affinity_per_service_list_head_index)
Create affinity record and take reference counting lock.
void test_key_calc_split()
static uword * clib_bitmap_set(uword *ai, uword i, uword value)
Sets the ith bit of a bitmap to new_value Removes trailing zeros from the bitmap.
#define NAT_INTERFACE_FLAG_IS_INSIDE
u32 fib_entry_get_resolving_interface(fib_node_index_t entry_index)
int nat44_ed_add_del_lb_static_mapping_local(ip4_address_t e_addr, u16 e_port, ip4_address_t l_addr, u16 l_port, nat_protocol_t proto, u32 vrf_id, u8 probability, u8 is_add)
snat_session_t * sessions
static_always_inline nat_translation_error_e nat_6t_flow_buf_translate(vlib_main_t *vm, snat_main_t *sm, vlib_buffer_t *b, ip4_header_t *ip, nat_6t_flow_t *f, nat_protocol_t proto, int is_output_feature, int is_i2o)
@ NAT_ED_TRNSL_ERR_FLOW_MISMATCH
int nat44_plugin_enable(nat44_config_t c)
int nat44_ed_add_address(ip4_address_t *addr, u32 vrf_id, u8 twice_nat)
struct _vlib_node_registration vlib_node_registration_t
int nat44_ed_set_frame_queue_nelts(u32 frame_queue_nelts)
fib_source_t fib_source_allocate(const char *name, fib_source_priority_t prio, fib_source_behaviour_t bh)
u16 current_length
Nbytes between current data and the end of this buffer.
static void nat_ed_session_delete(snat_main_t *sm, snat_session_t *ses, u32 thread_index, int lru_delete)
void update_per_vrf_sessions_vec(u32 fib_index, int is_del)
static_always_inline snat_interface_t * nat44_ed_get_interface(snat_interface_t *interfaces, u32 sw_if_index)
#define FIB_SOURCE_PRIORITY_HI
Some priority values that plugins might use when they are not to concerned where in the list they'll ...
#define nat_elog_notice_X1(_pm, nat_elog_fmt_str, nat_elog_fmt_arg, nat_elog_val1)
static bool nat44_ed_is_twice_nat_session(snat_session_t *s)
Check if NAT session is twice NAT.
ip4_add_del_interface_address_callback_t * add_del_interface_address_callbacks
Functions to call when interface address changes.
int nat44_ed_del_lb_static_mapping(ip4_address_t e_addr, u16 e_port, nat_protocol_t proto, u32 flags)
#define hash_get_mem(h, key)
static int is_snat_address_used_in_static_mapping(snat_main_t *sm, ip4_address_t addr)
static ip_csum_t ip_csum_sub_even(ip_csum_t c, ip_csum_t x)
ip4_add_del_interface_address_function_t * function
u32 tcp_trans_lru_head_index
void nat44_set_node_indexes(snat_main_t *sm, vlib_main_t *vm)
#define vec_free(V)
Free vector's memory (no header).
NAT plugin client-IP based session affinity for load-balancing.
vlib_node_t * vlib_get_node_by_name(vlib_main_t *vm, u8 *name)
#define fail_if_disabled()
void nat44_ed_add_resolve_record(ip4_address_t l_addr, u16 l_port, u16 e_port, nat_protocol_t proto, u32 vrf_id, u32 sw_if_index, u32 flags, ip4_address_t pool_addr, u8 *tag)
#define NAT_FLOW_OP_DPORT_REWRITE
clib_error_t * nat_affinity_init(vlib_main_t *vm)
Initialize NAT client-IP based affinity.
static void mss_clamping(u16 mss_clamping, tcp_header_t *tcp, ip_csum_t *sum)
u32 * max_translations_per_fib
@ NAT_NEXT_IN2OUT_ED_OUTPUT_FAST_PATH
#define pool_foreach_index(i, v)
@ FIB_ENTRY_FLAG_CONNECTED
int nat44_ed_free_port(ip4_address_t addr, u16 port, nat_protocol_t proto)
#define nat_elog_info(_pm, nat_elog_str)
description fragment has unexpected format
static void vlib_zero_simple_counter(vlib_simple_counter_main_t *cm, u32 index)
Clear a simple counter Clears the set of per-thread u16 counters, and the u64 counter.
static void nat44_ed_db_free()
static_always_inline void nat_reset_timeouts(nat_timeouts_t *timeouts)
static bool is_sm_self_twice_nat(u32 f)
static u64 calc_nat_key(ip4_address_t addr, u16 port, u32 fib_index, u8 proto)
static bool nat44_ed_is_interface_inside(snat_interface_t *i)
Check if NAT interface is inside.
u32 fib_table_get_index_for_sw_if_index(fib_protocol_t proto, u32 sw_if_index)
Get the index of the FIB bound to the interface.
@ FIB_ROUTE_PATH_FLAG_NONE
void fib_table_lock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Release a reference counting lock on the table.
static bool is_sm_identity_nat(u32 f)
#define VLIB_INIT_FUNCTION(x)
u32 nat44_get_max_session_limit()
@ STAT_DIR_TYPE_SCALAR_INDEX
vl_api_ip_proto_t protocol
u32 max_cfg_sessions_gauge
@ NAT_ED_TRNSL_ERR_PACKET_TRUNCATED
u32 * auto_add_sw_if_indices
static void init_ed_kv(clib_bihash_kv_16_8_t *kv, ip4_address_t l_addr, u16 l_port, ip4_address_t r_addr, u16 r_port, u32 fib_index, u8 proto, u32 thread_index, u32 session_index)
nat_translation_error_e nat_6t_flow_buf_translate_i2o(vlib_main_t *vm, snat_main_t *sm, vlib_buffer_t *b, ip4_header_t *ip, nat_6t_flow_t *f, nat_protocol_t proto, int is_output_feature)
#define NAT_FLOW_OP_SADDR_REWRITE
#define vec_foreach(var, vec)
Vector iterator.
clib_bihash_8_8_t static_mapping_by_local
u8 * format_nat_6t(u8 *s, va_list *args)
8 octet key, 8 octet key value pair
static void nat44_ed_worker_db_init(snat_main_per_thread_data_t *tsm, u32 translations, u32 translation_buckets)
u32 max_translations_per_thread
VNET_IP_TABLE_ADD_DEL_FUNCTION(nat_ip_table_add_del)
static void nat44_ed_db_init(u32 translations, u32 translation_buckets)
static uword pool_elts(void *v)
Number of active elements in a pool.
ip4_table_bind_function_t * function
u32 nat44_ed_get_in2out_worker_index(vlib_buffer_t *b, ip4_header_t *ip, u32 rx_fib_index, u8 is_output)
#define foreach_nat_counter
fib_protocol_t fp_proto
protocol type
@ NAT_NEXT_OUT2IN_ED_SLOW_PATH
int vnet_feature_enable_disable(const char *arc_name, const char *node_name, u32 sw_if_index, int enable_disable, void *feature_config, u32 n_feature_config_bytes)
vlib_node_registration_t nat_default_node
(constructor) VLIB_REGISTER_NODE (nat_default_node)
static_always_inline int nat_ed_ses_i2o_flow_hash_add_del(snat_main_t *sm, u32 thread_idx, snat_session_t *s, int is_add)
VNET_FEATURE_INIT(nat_pre_in2out, static)
u8 * format_ed_session_kvp(u8 *s, va_list *args)
static uword clib_bitmap_last_set(uword *ai)
Return the higest numbered set bit in a bitmap.
static u32 ip4_fib_index_from_table_id(u32 table_id)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
@ NAT_ED_TRNSL_ERR_INNER_IP_CORRUPT
#define ip_csum_update(sum, old, new, type, field)
static_always_inline int nat44_ed_validate_sm_input(u32 flags)
void nat44_addresses_free(snat_address_t **addresses)
@ NAT_NEXT_OUT2IN_CLASSIFY
#define VNET_FEATURES(...)
static_always_inline void nat_6t_flow_ip4_translate(snat_main_t *sm, vlib_buffer_t *b, ip4_header_t *ip, nat_6t_flow_t *f, nat_protocol_t proto, int is_icmp_inner_ip4, int skip_saddr_rewrite)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
static u16 ip4_header_checksum(ip4_header_t *i)
#define pool_dup(P)
Return copy of pool without alignment.
vlib_simple_counter_main_t hairpinning
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
static void split_nat_key(u64 key, ip4_address_t *addr, u16 *port, u32 *fib_index, nat_protocol_t *proto)
void fib_table_unlock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Take a reference counting lock on the table.
@ FIB_SOURCE_BH_SIMPLE
add paths without path extensions
#define pool_free(p)
Free a pool.
ip4_address_t external_addr
#define FIB_SOURCE_PRIORITY_LOW
#define NAT_FLOW_OP_SPORT_REWRITE
#define clib_warning(format, args...)
#define pool_alloc(P, N)
Allocate N more free elements to pool (unspecified alignment).
#define nat_init_simple_counter(c, n, sn)
void nat_affinity_flush_service(u32 affinity_per_service_list_head_index)
Flush all service affinity data.
int nat44_plugin_disable()
void nat_syslog_nat44_sdel(u32 ssubix, u32 sfibix, ip4_address_t *isaddr, u16 isport, ip4_address_t *idaddr, u16 idport, ip4_address_t *xsaddr, u16 xsport, ip4_address_t *xdaddr, u16 xdport, nat_protocol_t proto, u8 is_twicenat)
static int ip4_header_bytes(const ip4_header_t *i)
u32 nat_calc_bihash_buckets(u32 n_elts)
static_always_inline int it_fits(vlib_main_t *vm, vlib_buffer_t *b, void *object, size_t size)
void nat_ed_static_mapping_del_sessions(snat_main_t *sm, snat_main_per_thread_data_t *tsm, ip4_address_t l_addr, u16 l_port, u8 protocol, u32 fib_index, int addr_only, ip4_address_t e_addr, u16 e_port)
void nat_ipfix_logging_nat44_ses_delete(u32 thread_index, u32 src_ip, u32 nat_src_ip, nat_protocol_t nat_proto, u16 src_port, u16 nat_src_port, u32 fib_index)
Generate NAT44 session delete event.
static bool is_sm_exact_address(u32 f)
ip4_address_t * ip4_interface_first_address(ip4_main_t *im, u32 sw_if_index, ip_interface_address_t **result_ia)
#define clib_bitmap_foreach(i, ai)
Macro to iterate across set bits in a bitmap.
static_always_inline void nat_validate_interface_counters(snat_main_t *sm, u32 sw_if_index)
static vlib_thread_main_t * vlib_get_thread_main()
static uword is_pow2(uword x)
u32 fib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
void stat_segment_set_state_counter(u32 index, u64 value)
static ip_csum_t ip_csum_add_even(ip_csum_t c, ip_csum_t x)
u32 tcp_estab_lru_head_index
vl_api_interface_index_t sw_if_index
u32 stat_segment_new_entry(u8 *name, stat_directory_type_t t)
u32 sw_if_indices[VLIB_FRAME_SIZE]
static u16 ip_csum_fold(ip_csum_t c)
int nat44_ed_del_interface(u32 sw_if_index, u8 is_inside)
@ NAT_ED_TRNSL_ERR_SUCCESS
u8 * format_nat_ed_translation_error(u8 *s, va_list *args)
static void init_nat_k(clib_bihash_kv_8_8_t *kv, ip4_address_t addr, u16 port, u32 fib_index, nat_protocol_t proto)
@ NAT_ED_TRNSL_ERR_TRANSLATION_FAILED
int nat44_ed_add_interface_address(u32 sw_if_index, u8 twice_nat)
u8 static_mapping_connection_tracking
Aggregate type for a prefix.
vl_api_fib_path_type_t type
int nat44_ed_del_address(ip4_address_t addr, u8 delete_sm, u8 twice_nat)
u32 * auto_add_sw_if_indices_twice_nat
void nat_affinity_unlock(ip4_address_t client_addr, ip4_address_t service_addr, u8 proto, u16 service_port)
Release a reference counting lock for affinity.
#define vec_del1(v, i)
Delete the element at index I.
static_always_inline int nat_ed_ses_o2i_flow_hash_add_del(snat_main_t *sm, u32 thread_idx, snat_session_t *s, int is_add)
static void * ip4_next_header(ip4_header_t *i)
static bool na44_ed_is_fwd_bypass_session(snat_session_t *s)
Check if NAT session is forwarding bypass.
VLIB buffer representation.
void nat_affinity_enable()
NAT affinity enable.
snat_interface_t * output_feature_interfaces
#define VLIB_REGISTER_NODE(x,...)
int nat_affinity_find_and_lock(vlib_main_t *vm, ip4_address_t client_addr, ip4_address_t service_addr, u8 proto, u16 service_port, u8 *backend_index)
Find service backend index for client-IP and take a reference counting lock.
vl_api_wireguard_peer_flags_t flags