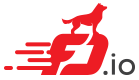 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
28 #define foreach_snort_deq_error \
29 _ (BAD_DESC, "bad descriptor") \
30 _ (BAD_DESC_INDEX, "bad descriptor index")
34 #define _(sym, str) SNORT_DEQ_ERROR_##sym,
41 #define _(sym, string) string,
56 head = __atomic_load_n (qp->
deq_head, __ATOMIC_ACQUIRE);
80 SNORT_DEQ_ERROR_BAD_DESC_INDEX, 1);
89 SNORT_DEQ_ERROR_BAD_DESC, 1);
96 buffer_indices++[0] = bi;
118 u32 max_recv,
u8 drop_on_disconnect)
120 u32 *bi, n_processed = 0;
125 if (n_processed >= max_recv)
134 if (drop_on_disconnect)
151 u8 drop_on_disconnect)
159 qp, buffer_indices,
nexts, max_recv, drop_on_disconnect);
161 if (n_processed == max_recv)
170 __atomic_store_n (&qp->
ready, 1, __ATOMIC_RELEASE);
195 u32 ready = __atomic_load_n (&qp->
ready, __ATOMIC_ACQUIRE);
198 si->drop_on_disconnect);
226 head = __atomic_load_n (qp->
deq_head, __ATOMIC_ACQUIRE);
246 SNORT_DEQ_ERROR_BAD_DESC_INDEX, 1);
255 SNORT_DEQ_ERROR_BAD_DESC, 1);
262 buffer_indices++[0] = bi;
285 u8 drop_on_disconnect)
288 qp, buffer_indices,
nexts, max_recv, drop_on_disconnect);
289 if (n_processed < max_recv)
293 __atomic_store_n (&qp->
ready, 1, __ATOMIC_RELEASE);
313 u32 ready = __atomic_load_n (&qp->
ready, __ATOMIC_ACQUIRE);
316 si->drop_on_disconnect);
331 nexts = next_indices;
349 if (sm->
input_mode == VLIB_NODE_STATE_POLLING)
356 .vector_size =
sizeof (
u32),
359 .
state = VLIB_NODE_STATE_DISABLED,
360 .sibling_of =
"snort-enq",
static_always_inline uword snort_deq_instance_all_poll(vlib_main_t *vm, snort_qpair_t *qp, u32 *buffer_indices, u16 *nexts, u32 max_recv, u8 drop_on_disconnect)
daq_vpp_desc_t * descriptors
static uword pow2_mask(uword x)
static_always_inline int clib_interrupt_get_next(void *in, int last)
static_always_inline uword snort_deq_instance_all_interrupt(vlib_main_t *vm, u32 instance_index, snort_qpair_t *qp, u32 *buffer_indices, u16 *nexts, u32 max_recv, u8 drop_on_disconnect)
vl_api_dhcp_client_state_t state
vlib_node_registration_t snort_deq_node
(constructor) VLIB_REGISTER_NODE (snort_deq_node)
vlib_buffer_enqueue_to_next(vm, node, from,(u16 *) nexts, frame->n_vectors)
static u32 snort_deq_node_polling(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
#define VLIB_NODE_FN(node)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
static_always_inline void clib_interrupt_clear(void *in, int int_num)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
vlib_main_t vlib_node_runtime_t * node
#define foreach_snort_deq_error
snort_instance_t * instances
#define static_always_inline
static_always_inline void clib_interrupt_set(void *in, int int_num)
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
static void snort_freelist_init(u32 *fl)
snort_per_thread_data_t * per_thread_data
struct _vlib_node_registration vlib_node_registration_t
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
description fragment has unexpected format
static void vlib_node_set_interrupt_pending(vlib_main_t *vm, u32 node_index)
#define vec_foreach(var, vec)
Vector iterator.
static char * snort_deq_error_strings[]
static u32 snort_deq_node_interrupt(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
static_always_inline uword snort_deq_instance(vlib_main_t *vm, u32 instance_index, snort_qpair_t *qp, u32 *buffer_indices, u16 *nexts, u32 max_recv)
static u8 * format_snort_deq_trace(u8 *s, va_list *args)
static_always_inline uword snort_deq_instance_poll(vlib_main_t *vm, snort_qpair_t *qp, u32 *buffer_indices, u16 *nexts, u32 max_recv)
static_always_inline u32 snort_process_all_buffer_indices(snort_qpair_t *qp, u32 *b, u16 *nexts, u32 max_recv, u8 drop_on_disconnect)
u16 nexts[VLIB_FRAME_SIZE]
vl_api_fib_path_type_t type
#define VLIB_REGISTER_NODE(x,...)