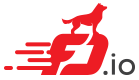 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
23 #include <vpp/app/version.h>
30 #include <plugins/ikev2/ikev2.api_enum.h>
31 #include <plugins/ikev2/ikev2.api_types.h>
35 #include <plugins/ikev2/ikev2.api.h>
36 #include <plugins/ikev2/ikev2_types.api.h>
41 #define IKEV2_PLUGIN_VERSION_MAJOR 1
42 #define IKEV2_PLUGIN_VERSION_MINOR 0
43 #define REPLY_MSG_ID_BASE ikev2_main.msg_id_base
46 #define IKEV2_MAX_DATA_LEN (1 << 10)
51 return (
ti << 16) | sai;
57 *sai = api_sai & 0xffff;
67 vl_api_ts->dh_group = ts->
dh_type;
87 vl_api_id->type =
id->type;
88 size_data =
sizeof (vl_api_id->data) - 1;
92 vl_api_id->data_len = size_data;
110 vl_api_auth->method =
auth->method;
112 vl_api_auth->hex =
auth->hex;
120 vl_api_responder->sw_if_index =
responder->sw_if_index;
128 vl_tr->transform_type = tr->
type;
145 rmp->_vl_msg_id =
ntohs (VL_API_IKEV2_PROFILE_DETAILS +
im->msg_id_base);
148 int size_data =
sizeof (rmp->
profile.name) - 1;
176 vl_api_ikev2_profile_t_endian (&rmp->
profile);
202 dst->n_rekey_req =
src->n_rekey_req;
203 dst->n_keepalives =
src->n_keepalives;
204 dst->n_retransmit =
src->n_retransmit;
205 dst->n_init_sa_retransmit =
src->n_init_retransmit;
206 dst->n_sa_init_req =
src->n_sa_init_req;
207 dst->n_sa_auth_req =
src->n_sa_auth_req;
220 vl_api_ikev2_sa_t *rsa = &rmp->
sa;
221 vl_api_ikev2_keys_t* k = &rsa->keys;
222 rsa->profile_index = rsa->profile_index;
223 rsa->sa_index = api_sa_index;
226 rsa->ispi = sa->
ispi;
227 rsa->rspi = sa->
rspi;
240 IKEV2_TRANSFORM_TYPE_INTEG);
271 vl_api_ikev2_sa_t_endian(rsa);
290 send_sa (sa, mp, api_sa_index);
309 vl_api_ikev2_keys_t *k = &rmp->
child_sa.keys;
318 IKEV2_TRANSFORM_TYPE_ENCR);
323 IKEV2_TRANSFORM_TYPE_INTEG);
328 IKEV2_TRANSFORM_TYPE_ESN);
349 vl_api_ikev2_child_sa_t_endian (&rmp->
child_sa);
361 u32 sai = ~0,
ti = ~0;
391 u32 sai = ~0,
ti = ~0;
393 u32 api_sa_index = clib_net_to_host_u32 (mp->
sa_index);
420 rmp->
ts.sa_index = api_sa_index;
423 vl_api_ikev2_ts_t_endian (&rmp->
ts);
435 u32 sai = ~0,
ti = ~0;
469 int msg_size =
sizeof (*rmp);
479 ntohs (VL_API_IKEV2_PLUGIN_GET_VERSION_REPLY +
im->msg_id_base);
491 vl_api_ikev2_profile_set_liveness_reply_t *rmp;
502 rv = VNET_API_ERROR_UNSPECIFIED;
505 rv = VNET_API_ERROR_UNIMPLEMENTED;
508 REPLY_MACRO (VL_API_IKEV2_PROFILE_SET_LIVENESS_REPLY);
514 vl_api_ikev2_profile_add_del_reply_t *rmp;
527 rv = VNET_API_ERROR_UNSPECIFIED;
530 rv = VNET_API_ERROR_UNIMPLEMENTED;
540 vl_api_ikev2_profile_set_auth_reply_t *rmp;
560 rv = VNET_API_ERROR_UNSPECIFIED;
564 rv = VNET_API_ERROR_INVALID_VALUE;
566 rv = VNET_API_ERROR_UNIMPLEMENTED;
575 vl_api_ikev2_profile_set_id_reply_t *rmp;
594 rv = VNET_API_ERROR_UNSPECIFIED;
598 rv = VNET_API_ERROR_INVALID_VALUE;
600 rv = VNET_API_ERROR_UNIMPLEMENTED;
610 vl_api_ikev2_profile_set_udp_encap_reply_t *rmp;
623 rv = VNET_API_ERROR_UNSPECIFIED;
626 rv = VNET_API_ERROR_UNIMPLEMENTED;
629 REPLY_MACRO (VL_API_IKEV2_PROFILE_SET_UDP_ENCAP_REPLY);
635 vl_api_ikev2_profile_set_ts_reply_t *rmp;
647 clib_net_to_host_u16 (mp->
ts.start_port),
648 clib_net_to_host_u16 (mp->
ts.end_port),
655 rv = VNET_API_ERROR_UNSPECIFIED;
658 rv = VNET_API_ERROR_UNIMPLEMENTED;
667 vl_api_ikev2_set_local_key_reply_t *rmp;
679 rv = VNET_API_ERROR_UNSPECIFIED;
682 rv = VNET_API_ERROR_UNIMPLEMENTED;
692 vl_api_ikev2_set_responder_hostname_reply_t *rmp;
711 rv = VNET_API_ERROR_UNSPECIFIED;
714 rv = VNET_API_ERROR_UNIMPLEMENTED;
717 REPLY_MACRO (VL_API_IKEV2_SET_RESPONDER_HOSTNAME_REPLY);
723 vl_api_ikev2_set_responder_reply_t *rmp;
741 rv = VNET_API_ERROR_UNSPECIFIED;
744 rv = VNET_API_ERROR_UNIMPLEMENTED;
754 vl_api_ikev2_set_ike_transforms_reply_t *rmp;
767 ntohl (mp->
tr.crypto_key_size));
773 rv = VNET_API_ERROR_UNSPECIFIED;
776 rv = VNET_API_ERROR_UNIMPLEMENTED;
779 REPLY_MACRO (VL_API_IKEV2_SET_IKE_TRANSFORMS_REPLY);
786 vl_api_ikev2_set_esp_transforms_reply_t *rmp;
798 ntohl (mp->
tr.crypto_key_size));
804 rv = VNET_API_ERROR_UNSPECIFIED;
807 rv = VNET_API_ERROR_UNIMPLEMENTED;
810 REPLY_MACRO (VL_API_IKEV2_SET_ESP_TRANSFORMS_REPLY);
816 vl_api_ikev2_set_sa_lifetime_reply_t *rmp;
827 clib_net_to_host_u64 (mp->
lifetime),
837 rv = VNET_API_ERROR_UNSPECIFIED;
840 rv = VNET_API_ERROR_UNIMPLEMENTED;
850 vl_api_ikev2_profile_set_ipsec_udp_port_reply_t *rmp;
860 clib_net_to_host_u16 (mp->
port),
864 rv = VNET_API_ERROR_UNIMPLEMENTED;
867 REPLY_MACRO (VL_API_IKEV2_PROFILE_SET_IPSEC_UDP_PORT_REPLY);
874 vl_api_ikev2_set_tunnel_interface_reply_t *rmp;
890 rv = VNET_API_ERROR_UNSPECIFIED;
894 rv = VNET_API_ERROR_UNIMPLEMENTED;
898 REPLY_MACRO (VL_API_IKEV2_SET_TUNNEL_INTERFACE_REPLY);
904 vl_api_ikev2_initiate_sa_init_reply_t *rmp;
919 rv = VNET_API_ERROR_UNSPECIFIED;
922 rv = VNET_API_ERROR_UNIMPLEMENTED;
932 vl_api_ikev2_initiate_del_ike_sa_reply_t *rmp;
944 rv = VNET_API_ERROR_UNSPECIFIED;
947 rv = VNET_API_ERROR_UNIMPLEMENTED;
950 REPLY_MACRO (VL_API_IKEV2_INITIATE_DEL_IKE_SA_REPLY);
957 vl_api_ikev2_initiate_del_child_sa_reply_t *rmp;
969 rv = VNET_API_ERROR_UNSPECIFIED;
972 rv = VNET_API_ERROR_UNIMPLEMENTED;
975 REPLY_MACRO (VL_API_IKEV2_INITIATE_DEL_CHILD_SA_REPLY);
982 vl_api_ikev2_profile_disable_natt_reply_t *rmp;
995 rv = VNET_API_ERROR_UNSPECIFIED;
998 rv = VNET_API_ERROR_UNIMPLEMENTED;
1001 REPLY_MACRO (VL_API_IKEV2_PROFILE_DISABLE_NATT_REPLY);
1008 vl_api_ikev2_initiate_rekey_child_sa_reply_t *rmp;
1020 rv = VNET_API_ERROR_UNSPECIFIED;
1023 rv = VNET_API_ERROR_UNIMPLEMENTED;
1026 REPLY_MACRO (VL_API_IKEV2_INITIATE_REKEY_CHILD_SA_REPLY);
1029 #include <ikev2/ikev2.api.c>
IKEv2: Initiate the rekey Child SA exchange.
vnet_interface_main_t * im
ikev2_sa_proposal_t * i_proposals
#define ikev2_log_error(...)
#define VALIDATE_SW_IF_INDEX(mp)
static void vl_api_ikev2_set_sa_lifetime_t_handler(vl_api_ikev2_set_sa_lifetime_t *mp)
clib_error_t * ikev2_set_profile_sa_lifetime(vlib_main_t *vm, u8 *name, u64 lifetime, u32 jitter, u32 handover, u64 maxdata)
static vl_api_registration_t * vl_api_client_index_to_registration(u32 index)
IKEv2: Set IKEv2 local RSA private key.
static void vl_api_ikev2_profile_add_del_t_handler(vl_api_ikev2_profile_add_del_t *mp)
clib_error_t * ikev2_set_profile_tunnel_interface(vlib_main_t *vm, u8 *name, u32 sw_if_index)
clib_error_t * ikev2_initiate_rekey_child_sa(vlib_main_t *vm, u32 ispi)
vl_api_address_t end_addr
static void vl_api_ikev2_initiate_del_ike_sa_t_handler(vl_api_ikev2_initiate_del_ike_sa_t *mp)
#define vec_new(T, N)
Create new vector of given type and length (unspecified alignment, no header).
#define clib_memcpy(d, s, n)
static void vl_api_send_msg(vl_api_registration_t *rp, u8 *elem)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
IKEv2: Set Child SA lifetime, limited by time and/or data.
#define REPLY_MACRO2_ZERO(t, body)
static void vl_api_ikev2_profile_set_id_t_handler(vl_api_ikev2_profile_set_id_t *mp)
IKEv2: Set IKEv2 profile local/remote identification.
Dump child SA of specific SA.
static void vl_api_ikev2_initiate_sa_init_t_handler(vl_api_ikev2_initiate_sa_init_t *mp)
static u32 ikev2_encode_sa_index(u32 sai, u32 ti)
ikev2_transforms_set ike_ts
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
IKEv2: Initiate the delete IKE SA exchange.
static void vl_api_ikev2_nonce_get_t_handler(vl_api_ikev2_nonce_get_t *mp)
ikev2_child_sa_t * childs
clib_error_t * ikev2_set_profile_esp_transforms(vlib_main_t *vm, u8 *name, ikev2_transform_encr_type_t crypto_alg, ikev2_transform_integ_type_t integ_alg, u32 crypto_key_size)
static void cp_ike_transforms(vl_api_ikev2_ike_transforms_t *vl_api_ts, ikev2_transforms_set *ts)
static clib_error_t * ikev2_api_init(vlib_main_t *vm)
static void vl_api_ikev2_child_sa_dump_t_handler(vl_api_ikev2_child_sa_dump_t *mp)
vl_api_address_t start_addr
clib_error_t * ikev2_set_local_key(vlib_main_t *vm, u8 *file)
clib_error_t * ikev2_set_profile_id(vlib_main_t *vm, u8 *name, u8 id_type, u8 *data, int is_local)
static void cp_ts(vl_api_ikev2_ts_t *vl_api_ts, ikev2_ts_t *ts, u8 is_local)
static void vl_api_ikev2_set_tunnel_interface_t_handler(vl_api_ikev2_set_tunnel_interface_t *mp)
IKEv2: Initiate the delete Child SA exchange.
vnet_api_error_t ikev2_set_profile_ipsec_udp_port(vlib_main_t *vm, u8 *name, u16 port, u8 is_set)
static void vl_api_ikev2_initiate_del_child_sa_t_handler(vl_api_ikev2_initiate_del_child_sa_t *mp)
__clib_export u8 * format_clib_error(u8 *s, va_list *va)
ikev2_main_per_thread_data_t * per_thread_data
static void vl_api_ikev2_traffic_selector_dump_t_handler(vl_api_ikev2_traffic_selector_dump_t *mp)
void ip_address_encode2(const ip_address_t *in, vl_api_address_t *out)
static void cp_id(vl_api_ikev2_id_t *vl_api_id, ikev2_id_t *id)
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
vl_api_ikev2_responder_t responder
static void cp_responder(vl_api_ikev2_responder_t *vl_api_responder, ikev2_responder_t *responder)
#define pool_foreach(VAR, POOL)
Iterate through pool.
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
static void cp_esp_transforms(vl_api_ikev2_esp_transforms_t *vl_api_ts, ikev2_transforms_set *ts)
clib_error_t * ikev2_initiate_delete_child_sa(vlib_main_t *vm, u32 ispi)
static void ikev2_decode_sa_index(u32 api_sai, u32 *sai, u32 *ti)
static void vl_api_ikev2_profile_set_ts_t_handler(vl_api_ikev2_profile_set_ts_t *mp)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
#define IKEV2_MAX_DATA_LEN
An API client registration, only in vpp/vlib.
static void setup_message_id_table(api_main_t *am)
vl_api_ikev2_profile_t profile
vl_api_ikev2_child_sa_t child_sa
static void send_child_sa(ikev2_child_sa_t *child, vl_api_ikev2_child_sa_dump_t *mp, u32 child_sa_index, u32 sa_index)
static void send_profile(ikev2_profile_t *profile, vl_api_registration_t *reg, u32 context)
static void vl_api_ikev2_set_local_key_t_handler(vl_api_ikev2_set_local_key_t *mp)
vl_api_ikev2_responder_t responder
IKEv2: Disable NAT traversal.
Details about all profiles.
clib_error_t * ikev2_set_profile_responder(vlib_main_t *vm, u8 *name, u32 sw_if_index, ip_address_t addr)
static void vl_api_ikev2_profile_set_udp_encap_t_handler(vl_api_ikev2_profile_set_udp_encap_t *mp)
ikev2_sa_proposal_t * r_proposals
static void vl_api_ikev2_set_responder_t_handler(vl_api_ikev2_set_responder_t *mp)
static void vl_api_ikev2_profile_disable_natt_t_handler(vl_api_ikev2_profile_disable_natt_t *mp)
vl_api_interface_index_t sw_if_index
static void vl_api_ikev2_profile_set_liveness_t_handler(vl_api_ikev2_profile_set_liveness_t *mp)
static void vl_api_ikev2_plugin_get_version_t_handler(vl_api_ikev2_plugin_get_version_t *mp)
#define IKEV2_PLUGIN_VERSION_MINOR
clib_error_t * ikev2_initiate_sa_init(vlib_main_t *vm, u8 *name)
clib_error_t * ikev2_set_profile_auth(vlib_main_t *vm, u8 *name, u8 auth_method, u8 *auth_data, u8 data_hex_format)
void ip_address_decode2(const vl_api_address_t *in, ip_address_t *out)
#define BAD_SW_IF_INDEX_LABEL
IKEv2: Set liveness parameters.
IKEv2: Set the tunnel interface which will be protected by IKE If this API is not called,...
static void vl_api_ikev2_profile_set_ipsec_udp_port_t_handler(vl_api_ikev2_profile_set_ipsec_udp_port_t *mp)
IKEv2: Add/delete profile.
#define vec_free(V)
Free vector's memory (no header).
#define pool_len(p)
Number of elements in pool vector.
static void ikev2_copy_stats(vl_api_ikev2_sa_stats_t *dst, const ikev2_stats_t *src)
details on specific traffic selector
clib_error_t * ikev2_profile_natt_disable(u8 *name)
clib_error_t * ikev2_set_profile_ike_transforms(vlib_main_t *vm, u8 *name, ikev2_transform_encr_type_t crypto_alg, ikev2_transform_integ_type_t integ_alg, ikev2_transform_dh_type_t dh_type, u32 crypto_key_size)
static void vl_api_ikev2_profile_set_auth_t_handler(vl_api_ikev2_profile_set_auth_t *mp)
description fragment has unexpected format
ikev2_responder_t responder
ikev2_sa_proposal_t * r_proposals
IKEv2: Set IKEv2 profile authentication method.
void cp_sa_transform(vl_api_ikev2_sa_transform_t *vl_tr, ikev2_sa_transform_t *tr)
#define VLIB_INIT_FUNCTION(x)
vnet_interface_per_thread_data_t * per_thread_data
#define vec_foreach(var, vec)
Vector iterator.
clib_error_t * ikev2_set_profile_udp_encap(vlib_main_t *vm, u8 *name)
static void vl_api_ikev2_set_responder_hostname_t_handler(vl_api_ikev2_set_responder_hostname_t *mp)
IKEv2: Set UDP encapsulation.
ikev2_transforms_set esp_ts
static void vl_api_ikev2_initiate_rekey_child_sa_t_handler(vl_api_ikev2_initiate_rekey_child_sa_t *mp)
static void vl_api_ikev2_sa_dump_t_handler(vl_api_ikev2_sa_dump_t *mp)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
IKEv2: Set IKEv2 responder interface and IP address.
static vlib_main_t * vlib_get_main(void)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
clib_error_t * ikev2_initiate_delete_ike_sa(vlib_main_t *vm, u64 ispi)
clib_error_t * ikev2_add_del_profile(vlib_main_t *vm, u8 *name, int is_add)
clib_error_t * ikev2_set_profile_ts(vlib_main_t *vm, u8 *name, u8 protocol_id, u16 start_port, u16 end_port, ip_address_t start_addr, ip_address_t end_addr, int is_local)
static void cp_auth(vl_api_ikev2_auth_t *vl_api_auth, ikev2_auth_t *auth)
IKEv2: Set/unset custom ipsec-over-udp port.
static void vl_api_ikev2_set_esp_transforms_t_handler(vl_api_ikev2_set_esp_transforms_t *mp)
static void send_sa(ikev2_sa_t *sa, vl_api_ikev2_sa_dump_t *mp, u32 api_sa_index)
#define clib_error_free(e)
IKEv2: Set IKEv2 profile traffic selector parameters.
clib_error_t * ikev2_set_liveness_params(u32 period, u32 max_retries)
clib_error_t * ikev2_set_profile_responder_hostname(vlib_main_t *vm, u8 *name, u8 *hostname, u32 sw_if_index)
vl_api_interface_index_t sw_if_index
ikev2_sa_transform_t * ikev2_sa_get_td_for_type(ikev2_sa_proposal_t *p, ikev2_transform_type_t type)
vl_api_interface_index_t sw_if_index
static void vl_api_ikev2_set_ike_transforms_t_handler(vl_api_ikev2_set_ike_transforms_t *mp)
Reply to get the plugin version.
#define REPLY_MACRO3_ZERO(t, n, body)
static void vl_api_ikev2_profile_dump_t_handler(vl_api_ikev2_profile_dump_t *mp)
IKEv2: Initiate the SA_INIT exchange.
void * vl_msg_api_alloc(int nbytes)
#define IKEV2_PLUGIN_VERSION_MAJOR