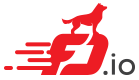 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
19 #include <vpp/app/version.h>
30 #include <openssl/sha.h>
32 #include <plugins/ikev2/ikev2.api_enum.h>
34 #define IKEV2_LIVENESS_RETRIES 3
35 #define IKEV2_LIVENESS_PERIOD_CHECK 30
43 #define ikev2_set_state(sa, v, ...) do { \
45 ikev2_elog_sa_state("ispi %lx SA state changed to " #v __VA_ARGS__, sa->ispi); \
61 s =
format (s,
"ikev2: sw_if_index %d, next index %d",
66 #define IKEV2_GENERATE_SA_INIT_OK_str ""
67 #define IKEV2_GENERATE_SA_INIT_OK_ERR_NO_DH_STR \
68 "no DH group configured for IKE proposals!"
69 #define IKEV2_GENERATE_SA_INIT_OK_ERR_UNSUPP_STR \
70 "DH group not supported!"
141 if (td->
type == IKEV2_TRANSFORM_TYPE_ENCR)
144 || t->
attrs[1] != 14)
162 u8 mandatory_bitmap, optional_bitmap;
166 mandatory_bitmap = (1 << IKEV2_TRANSFORM_TYPE_ENCR) |
167 (1 << IKEV2_TRANSFORM_TYPE_PRF) | (1 << IKEV2_TRANSFORM_TYPE_DH);
168 optional_bitmap = mandatory_bitmap | (1 << IKEV2_TRANSFORM_TYPE_INTEG);
172 mandatory_bitmap = (1 << IKEV2_TRANSFORM_TYPE_ENCR) |
173 (1 << IKEV2_TRANSFORM_TYPE_ESN);
174 optional_bitmap = mandatory_bitmap |
175 (1 << IKEV2_TRANSFORM_TYPE_INTEG) | (1 << IKEV2_TRANSFORM_TYPE_DH);
179 mandatory_bitmap = (1 << IKEV2_TRANSFORM_TYPE_INTEG) |
180 (1 << IKEV2_TRANSFORM_TYPE_ESN);
181 optional_bitmap = mandatory_bitmap | (1 << IKEV2_TRANSFORM_TYPE_DH);
196 if ((1 << transform->
type) & bitmap)
201 bitmap |= 1 << transform->
type;
208 if ((bitmap & mandatory_bitmap) == mandatory_bitmap &&
209 (bitmap & ~optional_bitmap) == 0)
213 RAND_bytes ((
u8 *) &
rv->spi, sizeof (
rv->spi));
252 by_initiator ? &
c->i_proposals[0] : &
c->r_proposals[0];
374 if (sa->
dh_group == IKEV2_TRANSFORM_DH_TYPE_NONE)
380 if (t2->type == IKEV2_TRANSFORM_TYPE_DH && sa->
dh_group == t2->dh_type)
389 sa->
dh_group = IKEV2_TRANSFORM_DH_TYPE_NONE;
396 RAND_bytes ((
u8 *) & sa->
ispi, 8);
405 RAND_bytes ((
u8 *) & sa->
rspi, 8);
425 #define _(A) ({void* __tmp__ = (A); (A) = 0; __tmp__;})
455 if (sa->
dh_group == IKEV2_TRANSFORM_DH_TYPE_NONE)
463 if (t2->type == IKEV2_TRANSFORM_TYPE_DH && sa->
dh_group == t2->dh_type)
472 sa->
dh_group = IKEV2_TRANSFORM_DH_TYPE_NONE;
489 u16 integ_key_len = 0, salt_len = 0;
499 integ_key_len = tr_integ->
key_len;
501 salt_len =
sizeof (
u32);
511 spi[0] = clib_host_to_net_u64 (sa->
ispi);
512 spi[1] = clib_host_to_net_u64 (sa->
rspi);
538 pos += integ_key_len;
543 pos += integ_key_len;
549 pos += tr_encr->
key_len + salt_len;
554 pos += tr_encr->
key_len + salt_len;
574 u16 integ_key_len = 0;
586 integ_key_len = ctr_integ->
key_len;
588 salt_len =
sizeof (
u32);
594 int len = ctr_encr->
key_len * 2 + integ_key_len * 2 + salt_len * 2;
628 pos += integ_key_len;
644 const u32 max_buf_size =
645 sizeof (
ispi) +
sizeof (
rspi) +
sizeof (ip6_address_t) +
sizeof (
u16);
646 u8 buf[max_buf_size];
661 const ike_ke_payload_header_t *ke = p;
662 u16 plen = clib_net_to_host_u16 (ke->length);
663 ASSERT (plen >=
sizeof (*ke) && plen <= rlen);
664 if (
sizeof (*ke) > rlen)
667 sa->
dh_group = clib_net_to_host_u16 (ke->dh_group);
669 vec_add (ke_data[0], ke->payload, plen - sizeof (*ke));
676 const ike_payload_header_t *ikep = p;
677 u16 plen = clib_net_to_host_u16 (ikep->length);
678 ASSERT (plen >=
sizeof (*ikep) && plen <= rlen);
687 if (
sizeof (*ikep) > rlen)
689 *plen = clib_net_to_host_u16 (ikep->length);
690 if (*plen <
sizeof (*ikep) || *plen > rlen)
701 u8 payload =
ike->nextpayload;
702 ike_payload_header_t *ikep;
706 "from ", clib_net_to_host_u64 (
ike->ispi),
707 clib_net_to_host_u64 (
ike->rspi),
711 sa->
ispi = clib_net_to_host_u64 (
ike->ispi);
721 len -=
sizeof (*ike);
724 ikep = (ike_payload_header_t *) &
ike->payload[p];
725 int current_length =
len - p;
750 if (n->
msg_type == IKEV2_NOTIFY_MSG_NAT_DETECTION_SOURCE_IP)
759 " behind NAT", sa->
ispi);
764 IKEV2_NOTIFY_MSG_NAT_DETECTION_DESTINATION_IP)
773 " (self) behind NAT", sa->
ispi);
795 payload = ikep->nextpayload;
810 u8 payload =
ike->nextpayload;
811 ike_payload_header_t *ikep;
814 sa->
ispi = clib_net_to_host_u64 (
ike->ispi);
815 sa->
rspi = clib_net_to_host_u64 (
ike->rspi);
829 len -=
sizeof (*ike);
832 int current_length =
len - p;
833 ikep = (ike_payload_header_t *) &
ike->payload[p];
845 clib_host_to_net_u32 (clib_net_to_host_u32 (
ike->msgid) + 1);
864 if (n->
msg_type == IKEV2_NOTIFY_MSG_NAT_DETECTION_SOURCE_IP)
872 " behind NAT, unsupported", sa->
ispi);
877 IKEV2_NOTIFY_MSG_NAT_DETECTION_DESTINATION_IP)
887 " (self) behind NAT", sa->
ispi);
909 payload = ikep->nextpayload;
916 u8 * payload,
u32 rlen,
u32 * out_len)
921 ike_payload_header_t *ikep = 0;
930 int is_aead = tr_encr->
encr_type == IKEV2_TRANSFORM_ENCR_TYPE_AES_GCM_16;
935 if (rlen <=
sizeof (*
ike))
938 int len = rlen -
sizeof (*ike);
942 ikep = (ike_payload_header_t *) &
ike->payload[p];
943 int current_length =
len - p;
949 last_payload = *payload;
962 *payload = ikep->nextpayload;
979 u32 aad_len = ikep->payload - aad;
980 u8 *tag = ikep->payload + plen;
983 plen, aad, aad_len, tag, &dlen);
999 if (plen <
sizeof (*ikep) + tr_integ->
key_trunc)
1002 plen = plen -
sizeof (*ikep) - tr_integ->
key_trunc;
1098 const ike_id_payload_header_t *
id = p;
1099 u16 plen = clib_net_to_host_u16 (
id->length);
1100 if (plen <
sizeof (*
id) || plen > rlen)
1103 sa_id->type =
id->id_type;
1113 const ike_auth_payload_header_t *ah = p;
1114 u16 plen = clib_net_to_host_u16 (ah->length);
1116 a->method = ah->auth_method;
1118 vec_add (
a->data, ah->payload, plen - sizeof (*ah));
1128 u8 payload =
ike->nextpayload;
1130 ike_payload_header_t *ikep;
1135 "from ", clib_host_to_net_u64 (
ike->ispi),
1136 clib_host_to_net_u64 (
ike->rspi),
1159 first_child_sa = &sa->
childs[0];
1171 ikep = (ike_payload_header_t *) &
plaintext[p];
1172 int current_length = dlen - p;
1220 if (n->
msg_type == IKEV2_NOTIFY_MSG_INITIAL_CONTACT)
1253 payload = ikep->nextpayload;
1269 u8 payload =
ike->nextpayload;
1271 ike_payload_header_t *ikep;
1277 "from ", clib_host_to_net_u64 (
ike->ispi),
1278 clib_host_to_net_u64 (
ike->rspi),
1291 u32 current_length = dlen - p;
1292 if (p +
sizeof (*ikep) > dlen)
1295 ikep = (ike_payload_header_t *) &
plaintext[p];
1296 u16 plen = clib_net_to_host_u16 (ikep->length);
1298 if (plen <
sizeof (*ikep) || plen > current_length)
1306 if (n->
msg_type == IKEV2_NOTIFY_MSG_AUTHENTICATION_FAILED)
1328 payload = ikep->nextpayload;
1340 u8 payload =
ike->nextpayload;
1345 ike_payload_header_t *ikep;
1360 clib_host_to_net_u64 (
ike->ispi),
1361 clib_host_to_net_u64 (
ike->rspi),
src,
1367 goto cleanup_and_exit;
1373 ikep = (ike_payload_header_t *) &
plaintext[p];
1374 int current_length = dlen - p;
1376 goto cleanup_and_exit;
1385 if (n->
msg_type == IKEV2_NOTIFY_MSG_REKEY_SA)
1417 goto cleanup_and_exit;
1420 payload = ikep->nextpayload;
1425 goto cleanup_and_exit;
1431 goto cleanup_and_exit;
1457 goto cleanup_and_exit;
1560 ikev2_ts_t *ts, *p_tsi, *p_tsr, *tsi = 0, *tsr = 0;
1632 u8 *authmsg, *psk = 0, *
auth = 0;
1656 if (sa_auth->
method == IKEV2_AUTH_METHOD_SHARED_KEY_MIC)
1658 if (!p->auth.data ||
1659 p->auth.method != IKEV2_AUTH_METHOD_SHARED_KEY_MIC)
1675 "shared key mismatch! ispi %lx", sa->
ispi);
1678 else if (sa_auth->
method == IKEV2_AUTH_METHOD_RSA_SIG)
1680 if (p->auth.method != IKEV2_AUTH_METHOD_RSA_SIG)
1692 "cert verification failed! ispi %lx", sa->
ispi);
1706 u8 *psk, *authmsg, *key_pad;
1712 if (!(sa->
i_auth.
method == IKEV2_AUTH_METHOD_SHARED_KEY_MIC ||
1715 ikev2_elog_uint (IKEV2_LOG_ERROR,
"unsupported authentication method %u",
1740 if (sel_p->
auth.
method == IKEV2_AUTH_METHOD_SHARED_KEY_MIC)
1748 else if (sel_p->
auth.
method == IKEV2_AUTH_METHOD_RSA_SIG)
1771 ikev2_elog_uint (IKEV2_LOG_ERROR,
"authentication failed, no matching "
1772 "profile found! ispi %lx", sa->
ispi);
1782 u8 *authmsg, *key_pad, *psk = 0;
1789 if (!(sa->
i_auth.
method == IKEV2_AUTH_METHOD_SHARED_KEY_MIC ||
1793 "unsupported authentication method %u",
1801 if (sa->
i_auth.
method == IKEV2_AUTH_METHOD_SHARED_KEY_MIC)
1811 else if (sa->
i_auth.
method == IKEV2_AUTH_METHOD_RSA_SIG)
1823 return (0x80000000 | (
ti << 24) | (sai << 12) | ci);
1829 return (0xc0000000 | (
ti << 24) | (sai << 12) | ci);
1862 .t_encap_decap_flags = TUNNEL_ENCAP_DECAP_FLAG_NONE,
1864 .t_mode = TUNNEL_MODE_P2P,
1867 .t_src =
a->local_ip,
1868 .t_dst =
a->remote_ip,
1872 .t_encap_decap_flags = TUNNEL_ENCAP_DECAP_FLAG_NONE,
1874 .t_mode = TUNNEL_MODE_P2P,
1877 .t_src =
a->remote_ip,
1878 .t_dst =
a->local_ip,
1881 if (~0 ==
a->sw_if_index)
1886 TUNNEL_ENCAP_DECAP_FLAG_NONE, IP_DSCP_CS0,
1889 if (
rv == VNET_API_ERROR_IF_ALREADY_EXISTS)
1906 "installing ipip tunnel failed! local spi: %x",
1925 a->encr_type, &
a->loc_ckey,
a->integ_type,
1926 &
a->loc_ikey,
a->flags,
a->salt_local,
1927 a->src_port,
a->dst_port, &tun_out, NULL);
1933 &
a->rem_ckey,
a->integ_type, &
a->rem_ikey,
1934 (
a->flags | IPSEC_SA_FLAG_IS_INBOUND),
a->salt_remote,
1935 a->ipsec_over_udp_port,
a->ipsec_over_udp_port, &tun_in, NULL);
1957 u32 child_index,
u8 is_rekey)
1961 ipsec_crypto_alg_t encr_type;
1962 ipsec_integ_alg_t integ_type;
1994 a.flags = IPSEC_SA_FLAG_USE_ANTI_REPLAY;
1997 a.flags |= IPSEC_SA_FLAG_IS_TUNNEL;
1998 a.flags |= IPSEC_SA_FLAG_UDP_ENCAP;
2001 a.flags |= IPSEC_SA_FLAG_UDP_ENCAP;
2002 a.is_rekey = is_rekey;
2006 a.flags |= IPSEC_SA_FLAG_USE_ESN;
2016 encr_type = IPSEC_CRYPTO_ALG_AES_CBC_128;
2019 encr_type = IPSEC_CRYPTO_ALG_AES_CBC_192;
2022 encr_type = IPSEC_CRYPTO_ALG_AES_CBC_256;
2030 else if (tr->
encr_type == IKEV2_TRANSFORM_ENCR_TYPE_AES_GCM_16
2036 encr_type = IPSEC_CRYPTO_ALG_AES_GCM_128;
2039 encr_type = IPSEC_CRYPTO_ALG_AES_GCM_192;
2042 encr_type = IPSEC_CRYPTO_ALG_AES_GCM_256;
2062 a.encr_type = encr_type;
2071 case IKEV2_TRANSFORM_INTEG_TYPE_AUTH_HMAC_SHA2_256_128:
2072 integ_type = IPSEC_INTEG_ALG_SHA_256_128;
2074 case IKEV2_TRANSFORM_INTEG_TYPE_AUTH_HMAC_SHA2_384_192:
2075 integ_type = IPSEC_INTEG_ALG_SHA_384_192;
2077 case IKEV2_TRANSFORM_INTEG_TYPE_AUTH_HMAC_SHA2_512_256:
2078 integ_type = IPSEC_INTEG_ALG_SHA_512_256;
2080 case IKEV2_TRANSFORM_INTEG_TYPE_AUTH_HMAC_SHA1_96:
2081 integ_type = IPSEC_INTEG_ALG_SHA1_96;
2096 integ_type = IPSEC_INTEG_ALG_NONE;
2099 a.integ_type = integ_type;
2154 if (child_index & 0xfffff000 || sa_index & 0xfffff000)
2180 remote_sa_id |=
mask;
2192 (
u8 *) &
a,
sizeof (
a));
2221 if (~0 ==
a->sw_if_index)
2224 ipip_tunnel_key_t
key = {
2226 .dst =
a->remote_ip,
2294 ike_payload_header_t *
ph;
2312 IKEV2_NOTIFY_MSG_NO_PROPOSAL_CHOSEN, 0);
2315 else if (sa->
dh_group == IKEV2_TRANSFORM_DH_TYPE_NONE)
2321 IKEV2_TRANSFORM_TYPE_DH);
2328 IKEV2_NOTIFY_MSG_INVALID_KE_PAYLOAD,
2339 IKEV2_NOTIFY_MSG_UNSUPPORTED_CRITICAL_PAYLOAD,
2347 ike->rspi = clib_host_to_net_u64 (sa->
rspi);
2352 u8 *nat_detection_sha1 =
2354 clib_host_to_net_u64 (sa->
rspi),
2357 IKEV2_NOTIFY_MSG_NAT_DETECTION_SOURCE_IP,
2358 nat_detection_sha1);
2360 nat_detection_sha1 =
2362 clib_host_to_net_u64 (sa->
rspi),
2365 IKEV2_NOTIFY_MSG_NAT_DETECTION_DESTINATION_IP,
2366 nat_detection_sha1);
2384 IKEV2_NOTIFY_MSG_AUTHENTICATION_FAILED,
2398 IKEV2_NOTIFY_MSG_NO_PROPOSAL_CHOSEN, 0);
2410 IKEV2_NOTIFY_MSG_UNSUPPORTED_CRITICAL_PAYLOAD,
2467 IKEV2_NOTIFY_MSG_UNSUPPORTED_CRITICAL_PAYLOAD,
2478 stats->n_keepalives++;
2494 *(
u32 *)
data = clib_host_to_net_u32 (notify.
spi);
2529 chain, IKEV2_NOTIFY_MSG_UNSUPPORTED_CRITICAL_PAYLOAD,
data);
2543 tlen =
sizeof (*ike);
2552 ike->length = clib_host_to_net_u32 (tlen);
2572 plen =
sizeof (*ph);
2573 ph = (ike_payload_header_t *) &
ike->payload[0];
2577 tr_encr->
encr_type == IKEV2_TRANSFORM_ENCR_TYPE_AES_GCM_16;
2595 ph->length = clib_host_to_net_u16 (plen);
2596 ike->length = clib_host_to_net_u32 (tlen);
2602 sizeof (*
ike) +
sizeof (*
ph),
2603 ph->payload + plen - sizeof (*
ph) -
2613 (ptd, sa, tr_encr, chain->
data,
ph->payload))
2644 u8 payload =
ike->nextpayload;
2646 if (sa->
ispi != clib_net_to_host_u64 (
ike->ispi) ||
2655 ike_payload_header_t *ikep = (ike_payload_header_t *) &
ike->payload[p];
2656 u32 plen = clib_net_to_host_u16 (ikep->length);
2658 if (plen <
sizeof (ike_payload_header_t))
2669 u32 slen = clib_net_to_host_u32 (
tmp->length);
2672 ike->nextpayload =
tmp->nextpayload;
2673 ike->version =
tmp->version;
2674 ike->exchange =
tmp->exchange;
2677 ike->length =
tmp->length;
2679 slen - sizeof (*
ike));
2681 "ispi %lx IKE_SA_INIT retransmit "
2682 "from %d.%d.%d.%d to %d.%d.%d.%d",
2692 "ispi %lx IKE_SA_INIT ignore "
2693 "from %d.%d.%d.%d to %d.%d.%d.%d",
2700 payload = ikep->nextpayload;
2733 u32 msg_id = clib_net_to_host_u32 (
ike->msgid);
2747 u32 slen = clib_net_to_host_u32 (
tmp->length);
2750 ike->nextpayload =
tmp->nextpayload;
2751 ike->version =
tmp->version;
2752 ike->exchange =
tmp->exchange;
2755 ike->length =
tmp->length;
2844 src =
ip4->src_address.as_u32;
2845 dst =
ip4->dst_address.as_u32;
2849 src =
ip6->src_address.as_u32[3];
2850 dst =
ip6->dst_address.as_u32[3];
2853 "received from %d.%d.%d.%d to %d.%d.%d.%d",
2897 ASSERT (!natt || is_ip4);
2923 ptr +=
sizeof (*ip40);
2925 ptr +=
sizeof (*udp0);
2926 ike0 = (ike_header_t *) ptr;
2927 ip_hdr_sz =
sizeof (*ip40);
2939 ip_hdr_sz =
sizeof (*ip40);
2944 ip_hdr_sz =
sizeof (*ip60);
2960 if (clib_net_to_host_u32 (ike0->length) != rlen)
2963 IKEV2_ERROR_BAD_LENGTH, 1);
2970 IKEV2_ERROR_NOT_IKEV2, 1);
2982 stats->n_sa_init_req++;
2983 if (ike0->rspi == 0)
3002 IKEV2_ERROR_IKE_SA_INIT_IGNORE
3004 IKEV2_ERROR_IKE_SA_INIT_RETRANSMIT,
3010 vm, sa0, ike0, udp0, rlen,
3014 IKEV2_ERROR_MALFORMED_PACKET,
3034 IKEV2_ERROR_NO_BUFF_SPACE,
3087 IKEV2_ERROR_NO_BUFF_SPACE,
3124 IKEV2_ERROR_IKE_REQ_IGNORE
3126 IKEV2_ERROR_IKE_REQ_RETRANSMIT,
3137 IKEV2_ERROR_MALFORMED_PACKET, 1);
3156 stats->n_sa_auth_req++;
3162 IKEV2_ERROR_NO_BUFF_SPACE,
3180 IKEV2_ERROR_IKE_REQ_IGNORE
3182 IKEV2_ERROR_IKE_REQ_RETRANSMIT,
3191 IKEV2_ERROR_MALFORMED_PACKET,
3235 IKEV2_ERROR_NO_BUFF_SPACE,
3253 IKEV2_ERROR_IKE_REQ_IGNORE
3255 IKEV2_ERROR_IKE_REQ_RETRANSMIT,
3264 IKEV2_ERROR_MALFORMED_PACKET,
3292 stats->n_rekey_req++;
3299 IKEV2_ERROR_NO_BUFF_SPACE,
3307 memset (
c, 0,
sizeof (*
c));
3321 vm,
node->node_index, IKEV2_ERROR_NO_BUFF_SPACE, 1);
3333 if (slen && ~0 != slen)
3394 && (b0->
flags & VLIB_BUFFER_IS_TRACED)))
3408 IKEV2_ERROR_PROCESSED,
frame->n_vectors);
3410 return frame->n_vectors;
3435 .name =
"ikev2-ip4",
3436 .vector_size =
sizeof (
u32),
3440 .n_errors = IKEV2_N_ERROR,
3441 .error_counters = ikev2_error_counters,
3452 .name =
"ikev2-ip4-natt",
3453 .vector_size =
sizeof (
u32),
3457 .n_errors = IKEV2_N_ERROR,
3458 .error_counters = ikev2_error_counters,
3469 .name =
"ikev2-ip6",
3470 .vector_size =
sizeof (
u32),
3474 .n_errors = IKEV2_N_ERROR,
3475 .error_counters = ikev2_error_counters,
3494 vec_add2 (*proposals, proposal, 1);
3502 if (td->
type == IKEV2_TRANSFORM_TYPE_ENCR
3507 attr[0] = clib_host_to_net_u16 (14 | (1 << 15));
3508 attr[1] = clib_host_to_net_u16 (td->
key_len << 3);
3523 if (IKEV2_TRANSFORM_INTEG_TYPE_NONE != ts->
integ_alg)
3529 if (td->
type == IKEV2_TRANSFORM_TYPE_INTEG
3540 (
"Didn't find any supported algorithm for IKEV2_TRANSFORM_TYPE_INTEG");
3552 if (td->
type == IKEV2_TRANSFORM_TYPE_PRF
3553 && td->
prf_type == IKEV2_TRANSFORM_PRF_TYPE_PRF_HMAC_SHA2_256)
3596 if (td->
type == IKEV2_TRANSFORM_TYPE_ESN)
3670 clib_host_to_net_u32 (0x6 << 28);
3681 b0->
flags |= VNET_BUFFER_F_LOCALLY_ORIGINATED;
3716 EVP_PKEY_free (km->
pkey);
3718 if (km->
pkey == NULL)
3761 if (send_notification)
3772 ike0->ispi = clib_host_to_net_u64 (sa->
ispi);
3773 ike0->rspi = clib_host_to_net_u64 (sa->
rspi);
3919 u8 * auth_data,
u8 data_hex_format)
3938 p->
auth.
hex = data_hex_format;
3940 if (auth_method == IKEV2_AUTH_METHOD_RSA_SIG)
3954 return (id_type == IKEV2_ID_TYPE_ID_IPV4_ADDR ||
3955 id_type == IKEV2_ID_TYPE_ID_IPV6_ADDR ||
3956 id_type == IKEV2_ID_TYPE_ID_RFC822_ADDR ||
3957 id_type == IKEV2_ID_TYPE_ID_FQDN);
4111 if ((IKEV2_TRANSFORM_INTEG_TYPE_NONE !=
integ_alg) +
4112 (IKEV2_TRANSFORM_ENCR_TYPE_AES_GCM_16 == crypto_alg) !=
4174 return VNET_API_ERROR_INVALID_VALUE;
4179 return VNET_API_ERROR_VALUE_EXIST;
4186 return VNET_API_ERROR_INVALID_VALUE;
4237 ip6_address_t *if_ip6;
4300 int len =
sizeof (ike_header_t), valid_ip = 0;
4385 clib_host_to_net_u64 (sa.
ispi), clib_host_to_net_u64 (sa.
rspi), &src_if_ip,
4389 nat_detection_sha1);
4391 nat_detection_sha1 =
4393 clib_host_to_net_u64 (sa.
rspi),
4395 clib_host_to_net_u16 (sa.
dst_port));
4397 IKEV2_NOTIFY_MSG_NAT_DETECTION_DESTINATION_IP,
4398 nat_detection_sha1);
4402 u64 tmpsig = clib_host_to_net_u64 (0x0001000200030004);
4405 IKEV2_NOTIFY_MSG_SIGNATURE_HASH_ALGORITHMS,
4414 char *errmsg =
"buffer alloc failure";
4423 ike0->length = clib_host_to_net_u32 (
len);
4430 ike0->ispi = clib_host_to_net_u64 (sa.
ispi);
4467 (
"ispi %lx rspi %lx IKEV2_EXCHANGE_SA_INIT sent to ",
4468 clib_host_to_net_u64 (sa0->
ispi), 0,
4496 ike0->ispi = clib_host_to_net_u64 (sa->
ispi);
4497 ike0->rspi = clib_host_to_net_u64 (sa->
rspi);
4545 if (!fchild || !fsa)
4616 ike0->ispi = clib_host_to_net_u64 (sa->
ispi);
4617 ike0->rspi = clib_host_to_net_u64 (sa->
rspi);
4627 RAND_bytes ((
u8 *) & proposals[0].
spi,
sizeof (proposals[0].
spi));
4628 rekey->
spi = proposals[0].
spi;
4668 if (!fchild || !fsa)
4768 for (thread_id = 0; thread_id < tm->
n_vlib_mains; thread_id++)
4775 #if OPENSSL_VERSION_NUMBER >= 0x10100000L
4776 ptd->
evp_ctx = EVP_CIPHER_CTX_new ();
4779 EVP_CIPHER_CTX_init (&ptd->_evp_ctx);
4780 ptd->
evp_ctx = &ptd->_evp_ctx;
4781 HMAC_CTX_init (&(ptd->_hmac_ctx));
4811 .runs_after =
VLIB_INITS (
"ipsec_init",
"ipsec_punt_init",
"dns_init"),
4867 ip46_address_t local_ip;
4868 ip46_address_t remote_ip;
4885 ipip_tunnel_key_t
key = {
4935 if (period == 0 || max_retries == 0)
5024 IKEV2_NOTIFY_MSG_NAT_DETECTION_DESTINATION_IP);
5028 clib_host_to_net_u64 (sa->
ispi), clib_host_to_net_u64 (sa->
rspi),
5046 IKEV2_NOTIFY_MSG_NAT_DETECTION_SOURCE_IP);
5051 clib_host_to_net_u64 (sa->
rspi),
5082 sa = pool_elt_at_index (km->sais, sai);
5083 if (sa->init_response_received)
5086 ikev2_process_pending_sa_init_one (vm, km, sa);
5111 ike0->ispi = clib_host_to_net_u64 (sa->
ispi);
5112 ike0->rspi = clib_host_to_net_u64 (sa->
rspi);
5188 u32 *to_be_deleted = 0;
5264 "ikev2-manager-process",
5268 .version = VPP_BUILD_VER,
5269 .description =
"Internet Key Exchange (IKEv2) Protocol",
static void ikev2_del_tunnel_from_main(ikev2_del_ipsec_tunnel_args_t *a)
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
static void ikev2_rewrite_v4_addrs(ikev2_sa_t *sa, ip4_header_t *ih)
@ IKEV2_STATE_AUTHENTICATED
static int ikev2_sa_sw_if_match(ikev2_sa_t *sa, u32 sw_if_index)
vlib_node_registration_t ip4_lookup_node
(constructor) VLIB_REGISTER_NODE (ip4_lookup_node)
void ikev2_payload_add_nonce(ikev2_payload_chain_t *c, u8 *nonce)
ikev2_sa_proposal_t * i_proposals
#define ikev2_log_error(...)
static void ikev2_send_informational_request(ikev2_sa_t *sa)
static int ikev2_ts_cmp(ikev2_ts_t *ts1, ikev2_ts_t *ts2)
clib_error_t * ikev2_set_profile_sa_lifetime(vlib_main_t *vm, u8 *name, u64 lifetime, u32 jitter, u32 handover, u64 maxdata)
static vlib_node_registration_t ikev2_mngr_process_node
(constructor) VLIB_REGISTER_NODE (ikev2_mngr_process_node)
static void ikev2_process_pending_sa_init_one(vlib_main_t *vm, ikev2_main_t *km, ikev2_sa_t *sa)
void vlib_worker_thread_barrier_release(vlib_main_t *vm)
#define vec_add(V, E, N)
Add N elements to end of vector V (no header, unspecified alignment)
static void ikev2_initiate_delete_ike_sa_internal(vlib_main_t *vm, ikev2_main_per_thread_data_t *tkm, ikev2_sa_t *sa, u8 send_notification)
#define IKEV2_PAYLOAD_NONE
#define hash_set(h, key, value)
#define ikev2_set_state(sa, v,...)
clib_error_t * ikev2_set_profile_tunnel_interface(vlib_main_t *vm, u8 *name, u32 sw_if_index)
ikev2_profile_t * profiles
static uword ikev2_mngr_process_fn(vlib_main_t *vm, vlib_node_runtime_t *rt, vlib_frame_t *f)
clib_error_t * ikev2_initiate_rekey_child_sa(vlib_main_t *vm, u32 ispi)
vl_api_address_t end_addr
static void ikev2_init_sa(vlib_main_t *vm, ikev2_sa_t *sa)
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
vl_api_ip_port_and_mask_t dst_port
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
static void ikev2_del_sa_init_from_main(u64 *ispi)
@ IKEV2_NEXT_IP6_ERROR_DROP
static int ikev2_get_if_address(u32 sw_if_index, ip_address_family_t af, ip_address_t *out_addr)
ikev2_generate_sa_error_t
#define IKEV2_PAYLOAD_TSR
static void ikev2_set_ts_addrs(ikev2_ts_t *ts, const ip_address_t *start, const ip_address_t *end)
static void ikev2_delete_child_sa_internal(vlib_main_t *vm, ikev2_sa_t *sa, ikev2_child_sa_t *csa)
#define vec_new(T, N)
Create new vector of given type and length (unspecified alignment, no header).
#define clib_memcpy(d, s, n)
static void ikev2_update_stats(vlib_main_t *vm, u32 node_index, ikev2_stats_t *s)
nat44_ei_hairpin_src_next_t next_index
ip4_main_t ip4_main
Global ip4 main structure.
ikev2_ts_t * ikev2_parse_ts_payload(ike_payload_header_t *ikep, u32 rlen)
ikev2_traffic_selector_type_t ts_type
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
static void ikev2_sa_free_all_vec(ikev2_sa_t *sa)
#define IKEV2_GCM_ICV_SIZE
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
#define clib_memcmp(s1, s2, m1)
A representation of an IP tunnel config.
u8 * ikev2_calc_sign(EVP_PKEY *pkey, u8 *data)
static_always_inline void clib_memset_u16(void *p, u16 val, uword count)
#define ike_hdr_is_initiator(_h)
void ikev2_generate_dh(ikev2_sa_t *sa, ikev2_sa_transform_t *t)
u8 * format_ikev2_id_type(u8 *s, va_list *args)
#define ike_hdr_is_response(_h)
vlib_get_buffers(vm, from, b, n_left_from)
static void ikev2_del_sa_init(u64 ispi)
ipsec_crypto_alg_t encr_type
@ VLIB_NODE_TYPE_INTERNAL
vlib_log_class_t vlib_log_register_class(char *class, char *subclass)
#define IKEV2_GCM_IV_SIZE
EVP_PKEY * ikev2_load_cert_file(u8 *file)
void ikev2_payload_add_ke(ikev2_payload_chain_t *c, u16 dh_group, u8 *dh_data)
vlib_main_t vlib_node_runtime_t * node
u32 current_remote_id_mask
@ IKEV2_GENERATE_SA_INIT_OK
EVP_PKEY * ikev2_load_key_file(u8 *file)
static void ikev2_calc_keys(ikev2_sa_t *sa)
ikev2_sa_proposal_t * r_proposal
static int ikev2_process_sa_init_req(vlib_main_t *vm, ikev2_sa_t *sa, ike_header_t *ike, udp_header_t *udp, u32 len, u32 sw_if_index)
static vlib_punt_hdl_t punt_hdl
u8 * ikev2_calc_prfplus(ikev2_sa_transform_t *tr, u8 *key, u8 *seed, int len)
#define clib_error_return(e, args...)
#define IKEV2_PAYLOAD_NONCE
static vnet_api_error_t ikev2_register_udp_port(ikev2_profile_t *p, u16 port)
@ DNS_API_PENDING_NAME_TO_IP
#define hash_foreach(key_var, value_var, h, body)
#define vec_append(v1, v2)
Append v2 after v1.
ikev2_sa_proposal_t * i_proposals
static ikev2_profile_t * ikev2_select_profile(ikev2_main_t *km, ikev2_sa_t *sa, ikev2_sa_transform_t *tr_prf, u8 *key_pad)
u8 * last_res_packet_data
ikev2_sa_proposal_t * i_proposal
ikev2_rekey_t * new_child
mhash_t profile_index_by_name
@ IKEV2_STATE_NO_PROPOSAL_CHOSEN
ikev2_auth_method_t method
u8 init_response_received
ikev2_transforms_set ike_ts
static void ikev2_complete_sa_data(ikev2_sa_t *sa, ikev2_sa_t *sai)
#define pool_put(P, E)
Free an object E in pool P.
static uword * mhash_get(mhash_t *h, const void *key)
#define IKEV2_EXCHANGE_SA_INIT
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
@ IKEV2_STATE_AUTH_FAILED
static int ikev2_delete_tunnel_interface(vnet_main_t *vnm, ikev2_sa_t *sa, ikev2_child_sa_t *child)
static void ikev2_sa_auth(ikev2_sa_t *sa)
static ikev2_profile_t * ikev2_profile_index_by_name(u8 *name)
int ikev2_encrypt_data(ikev2_main_per_thread_data_t *ptd, ikev2_sa_t *sa, ikev2_sa_transform_t *tr_encr, v8 *src, u8 *dst)
ikev2_child_sa_t * childs
int(* dns_resolve_name)()
vl_api_ikev2_sa_stats_t stats
static u32 ikev2_mk_local_sa_id(u32 sai, u32 ci, u32 ti)
clib_error_t * ikev2_set_profile_esp_transforms(vlib_main_t *vm, u8 *name, ikev2_transform_encr_type_t crypto_alg, ikev2_transform_integ_type_t integ_alg, u32 crypto_key_size)
vlib_buffer_enqueue_to_next(vm, node, from,(u16 *) nexts, frame->n_vectors)
#define IKEV2_PAYLOAD_TSI
int vlib_punt_register(vlib_punt_hdl_t client, vlib_punt_reason_t reason, const char *node_name)
Register a node to receive particular punted buffers.
vl_api_address_t start_addr
clib_error_t * ikev2_set_local_key(vlib_main_t *vm, u8 *file)
static u32 ikev2_flip_alternate_sa_bit(u32 id)
vnet_hw_if_output_node_runtime_t * r
static ikev2_sa_proposal_t * ikev2_select_proposal(ikev2_sa_proposal_t *proposals, ikev2_protocol_id_t prot_id)
vlib_frame_t * vlib_get_frame_to_node(vlib_main_t *vm, u32 to_node_index)
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
vlib_node_registration_t ip6_lookup_node
(constructor) VLIB_REGISTER_NODE (ip6_lookup_node)
#define IKEV2_PAYLOAD_NOTIFY
clib_error_t * ikev2_set_profile_id(vlib_main_t *vm, u8 *name, u8 id_type, u8 *data, int is_local)
#define ikev2_elog_uint_peers(_level, _format, _val, _ip1, _ip2)
A representation of a IPIP tunnel.
vlib_log_class_t log_class
ikev2_log_level_t log_level
#define hash_unset(h, key)
vnet_api_error_t ikev2_set_profile_ipsec_udp_port(vlib_main_t *vm, u8 *name, u16 port, u8 is_set)
pool_header_t * ph(void *p)
GDB callable function: ph - call pool_header - get pool header.
__clib_export u8 * format_clib_error(u8 *s, va_list *va)
u16 ip_address_size(const ip_address_t *a)
#define ikev2_payload_new_chain(V)
void ikev2_payload_add_delete(ikev2_payload_chain_t *c, ikev2_delete_t *d)
void ipsec_unregister_udp_port(u16 port)
ikev2_main_per_thread_data_t * per_thread_data
static void ikev2_delete_sa(ikev2_main_per_thread_data_t *ptd, ikev2_sa_t *sa)
static u32 random_u32(u32 *seed)
32-bit random number generator
#define IKEV2_PAYLOAD_IDI
static int ikev2_is_id_equal(ikev2_id_t *i1, ikev2_id_t *i2)
int ikev2_verify_sign(EVP_PKEY *pkey, u8 *sigbuf, u8 *data)
ikev2_delete_t * ikev2_parse_delete_payload(ike_payload_header_t *ikep, u32 rlen)
void ikev2_payload_add_notify(ikev2_payload_chain_t *c, u16 msg_type, u8 *data)
#define ikev2_elog_uint(_level, _format, _val)
int ikev2_decrypt_data(ikev2_main_per_thread_data_t *ptd, ikev2_sa_t *sa, ikev2_sa_transform_t *tr_encr, u8 *data, int len, u32 *out_len)
static vlib_node_registration_t ikev2_node_ip6
(constructor) VLIB_REGISTER_NODE (ikev2_node_ip6)
static void ikev2_generate_sa_init_data_and_log(ikev2_sa_t *sa)
static uword vlib_process_get_events(vlib_main_t *vm, uword **data_vector)
Return the first event type which has occurred and a vector of per-event data of that type,...
static clib_error_t * ikev2_set_initiator_proposals(vlib_main_t *vm, ikev2_sa_t *sa, ikev2_transforms_set *ts, ikev2_sa_proposal_t **proposals, int is_ike)
i16 current_data
signed offset in data[], pre_data[] that we are currently processing.
void vlib_put_frame_to_node(vlib_main_t *vm, u32 to_node_index, vlib_frame_t *f)
#define IPSEC_UDP_PORT_NONE
#define ip_addr_version(_a)
#define pool_foreach(VAR, POOL)
Iterate through pool.
void ip_address_copy_addr(void *dst, const ip_address_t *src)
enum ipsec_sad_flags_t_ ipsec_sa_flags_t
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
#define IKEV2_PAYLOAD_IDR
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
static void ikev2_set_ip_address(ikev2_sa_t *sa, const void *iaddr, const void *raddr, const ip_address_family_t af)
clib_error_t * ikev2_init(vlib_main_t *vm)
void vnet_sw_interface_admin_up(vnet_main_t *vnm, u32 sw_if_index)
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
static int ikev2_parse_auth_payload(const void *p, u32 rlen, ikev2_auth_t *a)
static uword ikev2_ip4_natt(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
static void ikev2_profile_free(ikev2_profile_t *p)
static __clib_warn_unused_result u32 vlib_buffer_alloc(vlib_main_t *vm, u32 *buffers, u32 n_buffers)
Allocate buffers into supplied array.
clib_error_t * ikev2_initiate_delete_child_sa(vlib_main_t *vm, u32 ispi)
v8 * ikev2_calc_prf(ikev2_sa_transform_t *tr, v8 *key, v8 *data)
#define vec_dup(V)
Return copy of vector (no header, no alignment)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
vnet_main_t * vnet_get_main(void)
#define IKEV2_GENERATE_SA_INIT_OK_ERR_NO_DH_STR
#define VLIB_NODE_FLAG_TRACE
Combined counter to hold both packets and byte differences.
vlib_combined_counter_main_t ipsec_sa_counters
SA packet & bytes counters.
#define IKEV2_HDR_FLAG_INITIATOR
#define vlib_worker_thread_barrier_sync(X)
static_always_inline uword vlib_get_thread_index(void)
static void ikev2_mngr_process_ipsec_sa(ipsec_sa_t *ipsec_sa)
static int ikev2_insert_non_esp_marker(ike_header_t *ike, int len)
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
ikev2_sa_transform_t * transforms
f64 end
end of the time range
#define vec_validate_aligned(V, I, A)
Make sure vector is long enough for given index (no header, specified alignment)
ikev2_sa_transform_t * supported_transforms
static void ikev2_elog_uint_peers_addr(u32 exchange, ip4_header_t *ip4, ip6_header_t *ip6, u8 is_ip4)
ikev2_notify_t * ikev2_parse_notify_payload(ike_payload_header_t *ikep, u32 rlen)
static vlib_node_registration_t ikev2_node_ip4
(constructor) VLIB_REGISTER_NODE (ikev2_node_ip4)
@ IKEV2_NEXT_IP4_ERROR_DROP
#define ikev2_elog_exchange(_fmt, _ispi, _rspi, _addr, _v4)
static int ikev2_is_id_supported(u8 id_type)
static void mhash_init_vec_string(mhash_t *h, uword n_value_bytes)
@ IKEV2_STATE_NOTIFY_AND_DELETE
int ipsec_tun_protect_update(u32 sw_if_index, const ip_address_t *nh, u32 sa_out, u32 *sas_in)
u8 * last_sa_init_res_packet_data
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
int ikev2_encrypt_aead_data(ikev2_main_per_thread_data_t *ptd, ikev2_sa_t *sa, ikev2_sa_transform_t *tr_encr, v8 *src, u8 *dst, u8 *aad, u32 aad_len, u8 *tag)
static void ikev2_send_ike(vlib_main_t *vm, ip_address_t *src, ip_address_t *dst, u32 bi0, u32 len, u16 src_port, u16 dst_port, u32 sw_if_index)
static void ikev2_unregister_udp_port(ikev2_profile_t *p)
static u8 * format_ikev2_gen_sa_error(u8 *s, va_list *args)
static int ikev2_process_auth_req(vlib_main_t *vm, ikev2_sa_t *sa, ike_header_t *ike, u32 len)
static void ikev2_add_tunnel_from_main(ikev2_add_ipsec_tunnel_args_t *a)
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
void udp_register_dst_port(vlib_main_t *vm, udp_dst_port_t dst_port, u32 node_index, u8 is_ip4)
vl_api_ip_port_and_mask_t src_port
clib_error_t * ikev2_set_profile_responder(vlib_main_t *vm, u8 *name, u32 sw_if_index, ip_address_t addr)
static vlib_node_registration_t ikev2_node_ip4_natt
(constructor) VLIB_REGISTER_NODE (ikev2_node_ip4_natt)
#define ip_address_initializer
ikev2_sa_proposal_t * r_proposals
static void ikev2_sa_match_ts(ikev2_sa_t *sa)
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
u8 * ikev2_find_ike_notify_payload(ike_header_t *ike, u32 msg_type)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
ipsec_integ_alg_t integ_type
void ipsec_register_udp_port(u16 port)
static u32 ikev2_generate_message(vlib_buffer_t *b, ikev2_sa_t *sa, ike_header_t *ike, void *user, udp_header_t *udp, ikev2_stats_t *stats)
static u8 * ikev2_decrypt_sk_payload(ikev2_sa_t *sa, ike_header_t *ike, u8 *payload, u32 rlen, u32 *out_len)
#define IKEV2_HDR_FLAG_RESPONSE
fib_protocol_t ip_address_to_46(const ip_address_t *addr, ip46_address_t *a)
#define IKEV2_EXCHANGE_IKE_AUTH
void ip_address_set(ip_address_t *dst, const void *src, ip_address_family_t af)
#define ikev2_payload_destroy_chain(V)
void ikev2_payload_add_notify_2(ikev2_payload_chain_t *c, u16 msg_type, u8 *data, ikev2_notify_t *notify)
int ipip_del_tunnel(u32 sw_if_index)
#define CLIB_CACHE_LINE_BYTES
struct _vlib_node_registration vlib_node_registration_t
clib_error_t * ikev2_initiate_sa_init(vlib_main_t *vm, u8 *name)
#define IKEV2_LIVENESS_PERIOD_CHECK
u16 current_length
Nbytes between current data and the end of this buffer.
clib_error_t * ikev2_set_profile_auth(vlib_main_t *vm, u8 *name, u8 auth_method, u8 *auth_data, u8 data_hex_format)
enum ikev2_log_level_t_ ikev2_log_level_t
VNET_SW_INTERFACE_ADD_DEL_FUNCTION(ikev2_sw_interface_add_del)
static u8 * ikev2_compute_nat_sha1(u64 ispi, u64 rspi, ip_address_t *ia, u16 port)
void ikev2_payload_add_ts(ikev2_payload_chain_t *c, ikev2_ts_t *ts, u8 type)
ikev2_protocol_id_t protocol_id
bool ip_address_is_zero(const ip_address_t *ip)
@ IKEV2_GENERATE_SA_INIT_ERR_UNSUPPORTED_DH
static u32 ikev2_mk_remote_sa_id(u32 sai, u32 ci, u32 ti)
#define ike_hdr_is_request(_h)
static void vlib_get_combined_counter(const vlib_combined_counter_main_t *cm, u32 index, vlib_counter_t *result)
Get the value of a combined counter, never called in the speed path Scrapes the entire set of per-thr...
#define vec_free(V)
Free vector's memory (no header).
void ipsec_mk_key(ipsec_key_t *key, const u8 *data, u8 len)
static void ikev2_rekey_child_sa_internal(vlib_main_t *vm, ikev2_sa_t *sa, ikev2_child_sa_t *csa)
static void ikev2_sa_del(ikev2_profile_t *p, u32 sw_if_index)
clib_error_t * ikev2_profile_natt_disable(u8 *name)
clib_error_t * ikev2_set_profile_ike_transforms(vlib_main_t *vm, u8 *name, ikev2_transform_encr_type_t crypto_alg, ikev2_transform_integ_type_t integ_alg, ikev2_transform_dh_type_t dh_type, u32 crypto_key_size)
static void ikev2_set_ts_type(ikev2_ts_t *ts, const ip_address_t *addr)
#define IKEV2_PAYLOAD_FLAG_CRITICAL
static u32 ikev2_get_new_ike_header_buff(vlib_main_t *vm, vlib_buffer_t **b)
#define ikev2_natt_active(_sa)
static u8 * format_ikev2_trace(u8 *s, va_list *args)
static int ikev2_parse_nonce_payload(const void *p, u32 rlen, u8 *nonce)
#define IKEV2_PAYLOAD_VENDOR
ip6_address_t * ip6_interface_first_address(ip6_main_t *im, u32 sw_if_index)
get first IPv6 interface address
#define ikev2_log_debug(...)
static f64 vlib_process_wait_for_event_or_clock(vlib_main_t *vm, f64 dt)
Suspend a cooperative multi-tasking thread Waits for an event, or for the indicated number of seconds...
description fragment has unexpected format
ikev2_natt_state_t natt_state
static ikev2_sa_transform_t * ikev2_find_transform_data(ikev2_sa_transform_t *t)
ikev2_responder_t responder
ikev2_sa_proposal_t * r_proposals
void ikev2_parse_vendor_payload(ike_payload_header_t *ikep)
static clib_error_t * ikev2_sw_interface_add_del(vnet_main_t *vnm, u32 sw_if_index, u32 is_add)
@ IKEV2_GENERATE_SA_INIT_ERR_NO_DH
void ikev2_cli_reference(void)
static_always_inline u32 vlib_buffer_get_default_data_size(vlib_main_t *vm)
static int ikev2_process_informational_req(vlib_main_t *vm, ikev2_sa_t *sa, ike_header_t *ike, u32 len)
static void ikev2_rewrite_v6_addrs(ikev2_sa_t *sa, ip6_header_t *ih)
#define VLIB_INIT_FUNCTION(x)
vl_api_address_family_t af
static int ikev2_create_tunnel_interface(vlib_main_t *vm, ikev2_sa_t *sa, ikev2_child_sa_t *child, u32 sa_index, u32 child_index, u8 is_rekey)
ipip_tunnel_t * ipip_tunnel_db_find(const ipip_tunnel_key_t *key)
counter_t bytes
byte counter
int ipsec_tun_protect_del(u32 sw_if_index, const ip_address_t *nh)
static uword ikev2_node_internal(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, u8 is_ip4, u8 natt)
static int ikev2_process_create_child_sa_req(vlib_main_t *vm, ikev2_sa_t *sa, ike_header_t *ike, u32 len)
#define IKEV2_LIVENESS_RETRIES
void ikev2_payload_add_sa(ikev2_payload_chain_t *c, ikev2_sa_proposal_t *proposals)
#define vec_foreach(var, vec)
Vector iterator.
clib_error_t * ikev2_set_profile_udp_encap(vlib_main_t *vm, u8 *name)
static void ikev2_sa_free_all_child_sa(ikev2_child_sa_t **childs)
void vl_api_rpc_call_main_thread(void *fp, u8 *data, u32 data_length)
static ip46_address_t to_ip46(u32 is_ipv6, u8 *buf)
int vlib_punt_hdl_t
Typedef for a client handle.
static int ikev2_parse_ke_payload(const void *p, u32 rlen, ikev2_sa_t *sa, u8 **ke_data)
static clib_error_t * ikev2_resolve_responder_hostname(vlib_main_t *vm, ikev2_responder_t *r)
void ikev2_payload_add_auth(ikev2_payload_chain_t *c, ikev2_auth_t *auth)
#define vec_resize(V, N)
Resize a vector (no header, unspecified alignment) Add N elements to end of given vector V,...
static uword ikev2_ip4(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
ikev2_transforms_set esp_ts
static u32 ikev2_retransmit_sa_init(ike_header_t *ike, ip_address_t iaddr, ip_address_t raddr, u32 rlen)
static void ikev2_initial_contact_cleanup(ikev2_main_per_thread_data_t *ptd, ikev2_sa_t *sa)
void ikev2_crypto_init(ikev2_main_t *km)
static uword ikev2_ip6(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
static void ikev2_sa_free_child_sa(ikev2_child_sa_t *c)
ikev2_transform_dh_type_t
static u8 * ikev2_sa_generate_authmsg(ikev2_sa_t *sa, int is_responder)
static void ikev2_calc_child_keys(ikev2_sa_t *sa, ikev2_child_sa_t *child)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
ikev2_child_sa_t * ikev2_sa_get_child(ikev2_sa_t *sa, u32 spi, ikev2_protocol_id_t prot_id, int by_initiator)
static void ikev2_process_sa_init_resp(vlib_main_t *vm, ikev2_sa_t *sa, ike_header_t *ike, udp_header_t *udp, u32 len)
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
void ikev2_payload_chain_add_padding(ikev2_payload_chain_t *c, int bs)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
vnet_interface_output_runtime_t * rt
v8 * ikev2_calc_integr(ikev2_sa_transform_t *tr, v8 *key, u8 *data, int len)
static u16 ip4_header_checksum(ip4_header_t *i)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
u8 * last_sa_init_req_packet_data
clib_error_t * ikev2_initiate_delete_ike_sa(vlib_main_t *vm, u64 ispi)
clib_error_t * ikev2_add_del_profile(vlib_main_t *vm, u8 *name, int is_add)
clib_error_t * ikev2_set_profile_ts(vlib_main_t *vm, u8 *name, u8 protocol_id, u16 start_port, u16 end_port, ip_address_t start_addr, ip_address_t end_addr, int is_local)
#define vec_terminate_c_string(V)
(If necessary) NULL terminate a vector containing a c-string.
#define IKEV2_PAYLOAD_AUTH
int ikev2_decrypt_aead_data(ikev2_main_per_thread_data_t *ptd, ikev2_sa_t *sa, ikev2_sa_transform_t *tr_encr, u8 *data, int data_len, u8 *aad, u32 aad_len, u8 *tag, u32 *out_len)
static void ikev2_profile_responder_free(ikev2_responder_t *r)
f64 liveness_period_check
ikev2_transform_encr_type_t
static u32 ikev2_retransmit_sa_init_one(ikev2_sa_t *sa, ike_header_t *ike, ip_address_t iaddr, ip_address_t raddr, u32 rlen)
void ikev2_disable_dpd(void)
static void ikev2_sa_auth_init(ikev2_sa_t *sa)
static int ikev2_parse_id_payload(const void *p, u16 rlen, ikev2_id_t *sa_id)
ikev2_sa_proposal_t * ikev2_parse_sa_payload(ike_payload_header_t *ikep, u32 rlen)
#define clib_error_free(e)
u16 nexts[VLIB_FRAME_SIZE]
void ikev2_sa_free_proposal_vector(ikev2_sa_proposal_t **v)
#define ikev2_elog_error(_msg)
static u16 ikev2_get_port(ikev2_sa_t *sa)
int ip_address_cmp(const ip_address_t *ip1, const ip_address_t *ip2)
static f64 vlib_time_now(vlib_main_t *vm)
int ipsec_sa_add_and_lock(u32 id, u32 spi, ipsec_protocol_t proto, ipsec_crypto_alg_t crypto_alg, const ipsec_key_t *ck, ipsec_integ_alg_t integ_alg, const ipsec_key_t *ik, ipsec_sa_flags_t flags, u32 salt, u16 src_port, u16 dst_port, const tunnel_t *tun, u32 *sa_out_index)
ip4_address_t * ip4_interface_first_address(ip4_main_t *im, u32 sw_if_index, ip_interface_address_t **result_ia)
void ikev2_complete_dh(ikev2_sa_t *sa, ikev2_sa_transform_t *t)
#define IKEV2_EXCHANGE_CREATE_CHILD_SA
static int ikev2_check_payload_length(const ike_payload_header_t *ikep, int rlen, u16 *plen)
#define IKEV2_EXCHANGE_INFORMATIONAL
int ikev2_set_log_level(ikev2_log_level_t log_level)
clib_error_t * ikev2_set_liveness_params(u32 period, u32 max_retries)
#define IKEV2_GENERATE_SA_INIT_OK_ERR_UNSUPP_STR
vlib_punt_hdl_t vlib_punt_client_register(const char *who)
Register a new clinet.
static void ikev2_initial_contact_cleanup_internal(ikev2_main_per_thread_data_t *ptd, ikev2_sa_t *sa)
static vlib_thread_main_t * vlib_get_thread_main()
void ip_address_copy(ip_address_t *dst, const ip_address_t *src)
static void ikev2_process_pending_sa_init(vlib_main_t *vm, ikev2_main_t *km)
clib_error_t * ikev2_set_profile_responder_hostname(vlib_main_t *vm, u8 *name, u8 *hostname, u32 sw_if_index)
vl_api_interface_index_t sw_if_index
#define hash_create(elts, value_bytes)
static void ikev2_sa_del_child_sa(ikev2_sa_t *sa, ikev2_child_sa_t *child)
static_always_inline ikev2_main_per_thread_data_t * ikev2_get_per_thread_data()
#define clib_atomic_bool_cmp_and_swap(addr, old, new)
#define IKEV2_PAYLOAD_DELETE
static u8 ikev2_mngr_process_child_sa(ikev2_sa_t *sa, ikev2_child_sa_t *csa, u8 del_old_ids)
ikev2_transform_integ_type_t
__clib_export uword mhash_set_mem(mhash_t *h, void *key, uword *new_value, uword *old_value)
int ipsec_sa_unlock_id(u32 id)
static void ikev2_cleanup_profile_sessions(ikev2_main_t *km, ikev2_profile_t *p)
ikev2_sa_transform_t * ikev2_sa_get_td_for_type(ikev2_sa_proposal_t *p, ikev2_transform_type_t type)
__clib_export uword mhash_unset(mhash_t *h, void *key, uword *old_value)
void vnet_sw_interface_admin_down(vnet_main_t *vnm, u32 sw_if_index)
vlib_punt_reason_t ipsec_punt_reason[IPSEC_PUNT_N_REASONS]
ipsec_sa_t * ipsec_sa_pool
Pool of IPSec SAs.
static ikev2_generate_sa_error_t ikev2_generate_sa_init_data(ikev2_sa_t *sa)
@ IKEV2_STATE_TS_UNACCEPTABLE
vl_api_fib_path_type_t type
static int ikev2_mngr_process_responder_sas(ikev2_sa_t *sa)
#define hash_set1(h, key)
int ipip_add_tunnel(ipip_transport_t transport, u32 instance, ip46_address_t *src, ip46_address_t *dst, u32 fib_index, tunnel_encap_decap_flags_t flags, ip_dscp_t dscp, tunnel_mode_t tmode, u32 *sw_if_indexp)
#define vec_del1(v, i)
Delete the element at index I.
u8 * ip_addr_bytes(ip_address_t *ip)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
static u32 ikev2_retransmit_resp(ikev2_sa_t *sa, ike_header_t *ike)
enum ip_address_family_t_ ip_address_family_t
void ikev2_payload_add_id(ikev2_payload_chain_t *c, ikev2_id_t *id, u8 type)
VLIB buffer representation.
void * vlib_get_plugin_symbol(char *plugin_name, char *symbol_name)
#define VLIB_REGISTER_NODE(x,...)