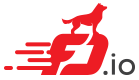 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
30 #define foreach_ipsec_tun_protect_input_error \
31 _(RX, "good packets received") \
32 _(DISABLED, "ipsec packets received on disabled interface") \
33 _(NO_TUNNEL, "no matching tunnel") \
34 _(TUNNEL_MISMATCH, "SPI-tunnel mismatch") \
35 _(NAT_KEEPALIVE, "NAT Keepalive") \
36 _(TOO_SHORT, "Too Short") \
40 #define _(sym,string) string,
47 #define _(sym,str) IPSEC_TUN_PROTECT_INPUT_ERROR_##sym,
55 #define _(v, s) IPSEC_TUN_PROTECT_NEXT_##v,
81 s =
format (s,
"IPSec: %U seq %u",
84 s =
format (s,
"IPSec: %U seq %u sa %d",
96 b->
error =
node->errors[IPSEC_TUN_PROTECT_INPUT_ERROR_SPI_0];
98 IPSEC_PUNT_IP4_SPI_UDP_0 :
99 IPSEC_PUNT_IP4_NO_SUCH_TUNNEL)];
103 b->
error =
node->errors[IPSEC_TUN_PROTECT_INPUT_ERROR_NO_TUNNEL];
113 b->
error =
node->errors[IPSEC_TUN_PROTECT_INPUT_ERROR_NO_TUNNEL];
142 nexts,
is_ip6 ?
im->esp6_decrypt_next_index :
im->esp4_decrypt_next_index,
146 u32 n_disabled = 0, n_no_tunnel = 0;
148 u32 last_sw_if_index = ~0;
160 clib_memset (&last_key6, 0xff,
sizeof (last_key6));
169 u32 sw_if_index0, len0, hdr_sz0;
194 if (ip40->
protocol == IP_PROTOCOL_UDP)
218 node->errors[IPSEC_TUN_PROTECT_INPUT_ERROR_NAT_KEEPALIVE];
221 node->errors[IPSEC_TUN_PROTECT_INPUT_ERROR_TOO_SHORT];
232 key60->
key.__pad = 0;
234 if (memcmp (key60, &last_key6,
sizeof (last_key6)) == 0)
241 clib_bihash_search_inline_24_16 (&
im->tun6_protect_by_key,
247 sizeof (last_result));
262 if (key40->
key == last_key4.
key)
269 clib_bihash_search_inline_8_16 (&
im->tun4_protect_by_key,
275 sizeof (last_result));
276 last_key4.
key = key40->
key;
299 b[0]->
error =
node->errors[IPSEC_TUN_PROTECT_INPUT_ERROR_DISABLED];
313 if (n_packets && !(itr0.
flags & IPSEC_PROTECT_ENCAPED))
320 last_sw_if_index = sw_if_index0;
327 im->esp4_decrypt_tun_next_index;
329 if (itr0.
flags & IPSEC_PROTECT_FEAT)
342 if (
b[0]->
flags & VLIB_BUFFER_IS_TRACED)
351 tr->
seq = (len0 >=
sizeof (*esp0) ?
352 clib_host_to_net_u32 (esp0->
seq) : ~0);
362 if (n_packets && !(itr0.
flags & IPSEC_PROTECT_ENCAPED))
365 last_sw_if_index, n_packets,
n_bytes);
368 IPSEC_TUN_PROTECT_INPUT_ERROR_RX,
372 IPSEC_TUN_PROTECT_INPUT_ERROR_NO_TUNNEL,
389 .name =
"ipsec4-tun-input",
390 .vector_size =
sizeof (
u32),
395 .sibling_of =
"device-input",
408 .name =
"ipsec6-tun-input",
409 .vector_size =
sizeof (
u32),
414 .sibling_of =
"device-input",
vnet_interface_main_t * im
static void ipsec4_tunnel_mk_key(ipsec4_tunnel_kv_t *k, const ip4_address_t *ip, u32 spi)
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
ipsec_tun_protect_input_error_t
#define clib_memcpy(d, s, n)
@ IPSEC_TUN_PROTECT_INPUT_N_ERROR
@ IPSEC_TUN_PROTECT_N_NEXT
static_always_inline void clib_memset_u16(void *p, u16 val, uword count)
static uword ipsec_tun_protect_input_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *from_frame, int is_ip6)
vlib_get_buffers(vm, from, b, n_left_from)
static char * ipsec_tun_protect_input_error_strings[]
@ VLIB_NODE_TYPE_INTERNAL
vlib_main_t vlib_node_runtime_t * node
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
vlib_main_t vlib_node_runtime_t vlib_frame_t * from_frame
vlib_buffer_enqueue_to_next(vm, node, from,(u16 *) nexts, frame->n_vectors)
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
static u16 ipsec_ip4_if_no_tunnel(vlib_node_runtime_t *node, vlib_buffer_t *b, const esp_header_t *esp, const ip4_header_t *ip4)
static u8 * format_ipsec_tun_protect_input_trace(u8 *s, va_list *args)
@ VNET_DEVICE_INPUT_NEXT_IP6_DROP
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
vlib_error_t error
Error code for buffers to be enqueued to error handler.
@ VNET_DEVICE_INPUT_NEXT_IP4_DROP
#define VLIB_NODE_FN(node)
#define VLIB_NODE_FLAG_TRACE
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
#define foreach_ipsec_tun_protect_input_error
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
result of a lookup in the protection bihash
@ VNET_DEVICE_INPUT_NEXT_PUNT
#define foreach_ipsec_input_next
vnet_feature_main_t feature_main
@ VNET_INTERFACE_COUNTER_RX
struct _vlib_node_registration vlib_node_registration_t
vlib_combined_counter_main_t * combined_sw_if_counters
static uword vnet_sw_interface_is_admin_up(vnet_main_t *vnm, u32 sw_if_index)
enum ipsec_tun_next_t_ ipsec_tun_next_t
static u16 ipsec_ip6_if_no_tunnel(vlib_node_runtime_t *node, vlib_buffer_t *b, const esp_header_t *esp)
8 octet key, 8 octet key value pair
description fragment has unexpected format
A collection of combined counters.
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
struct ipsec6_tunnel_kv_t_::@461 key
vlib_node_registration_t ipsec6_tun_input_node
(constructor) VLIB_REGISTER_NODE (ipsec6_tun_input_node)
static_always_inline void vnet_feature_arc_start(u8 arc, u32 sw_if_index, u32 *next0, vlib_buffer_t *b0)
u16 nexts[VLIB_FRAME_SIZE]
static int ip4_header_bytes(const ip4_header_t *i)
vlib_node_registration_t ipsec4_tun_input_node
(constructor) VLIB_REGISTER_NODE (ipsec4_tun_input_node)
u8 device_input_feature_arc_index
Feature arc index for device-input.
ipsec_protect_flags_t flags
vlib_punt_reason_t ipsec_punt_reason[IPSEC_PUNT_N_REASONS]
vl_api_fib_path_type_t type
@ VNET_INTERFACE_COUNTER_DROP
vnet_interface_main_t interface_main
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)
vl_api_wireguard_peer_flags_t flags