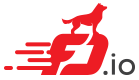 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
39 #include <interface.api_enum.h>
40 #include <interface.api_types.h>
42 #define REPLY_MSG_ID_BASE msg_id_base
49 #define foreach_vpe_api_msg \
50 _ (SW_INTERFACE_SET_FLAGS, sw_interface_set_flags) \
51 _ (SW_INTERFACE_SET_PROMISC, sw_interface_set_promisc) \
52 _ (HW_INTERFACE_SET_MTU, hw_interface_set_mtu) \
53 _ (SW_INTERFACE_SET_MTU, sw_interface_set_mtu) \
54 _ (WANT_INTERFACE_EVENTS, want_interface_events) \
55 _ (SW_INTERFACE_DUMP, sw_interface_dump) \
56 _ (SW_INTERFACE_ADD_DEL_ADDRESS, sw_interface_add_del_address) \
57 _ (SW_INTERFACE_SET_RX_MODE, sw_interface_set_rx_mode) \
58 _ (SW_INTERFACE_RX_PLACEMENT_DUMP, sw_interface_rx_placement_dump) \
59 _ (SW_INTERFACE_SET_RX_PLACEMENT, sw_interface_set_rx_placement) \
60 _ (SW_INTERFACE_SET_TABLE, sw_interface_set_table) \
61 _ (SW_INTERFACE_GET_TABLE, sw_interface_get_table) \
62 _ (SW_INTERFACE_SET_UNNUMBERED, sw_interface_set_unnumbered) \
63 _ (SW_INTERFACE_CLEAR_STATS, sw_interface_clear_stats) \
64 _ (SW_INTERFACE_TAG_ADD_DEL, sw_interface_tag_add_del) \
65 _ (SW_INTERFACE_ADD_DEL_MAC_ADDRESS, sw_interface_add_del_mac_address) \
66 _ (SW_INTERFACE_SET_MAC_ADDRESS, sw_interface_set_mac_address) \
67 _ (SW_INTERFACE_GET_MAC_ADDRESS, sw_interface_get_mac_address) \
68 _ (CREATE_VLAN_SUBIF, create_vlan_subif) \
69 _ (CREATE_SUBIF, create_subif) \
70 _ (DELETE_SUBIF, delete_subif) \
71 _ (CREATE_LOOPBACK, create_loopback) \
72 _ (CREATE_LOOPBACK_INSTANCE, create_loopback_instance) \
73 _ (DELETE_LOOPBACK, delete_loopback) \
74 _ (INTERFACE_NAME_RENUMBER, interface_name_renumber) \
75 _ (COLLECT_DETAILED_INTERFACE_STATS, collect_detailed_interface_stats) \
76 _ (SW_INTERFACE_SET_IP_DIRECTED_BROADCAST, \
77 sw_interface_set_ip_directed_broadcast) \
78 _ (SW_INTERFACE_ADDRESS_REPLACE_BEGIN, sw_interface_address_replace_begin) \
79 _ (SW_INTERFACE_ADDRESS_REPLACE_END, sw_interface_address_replace_end)
84 vl_api_sw_interface_set_flags_reply_t *rmp;
111 vl_api_sw_interface_set_promisc_reply_t *rmp;
126 rv = VNET_API_ERROR_INVALID_VALUE;
135 REPLY_MACRO (VL_API_SW_INTERFACE_SET_PROMISC_REPLY);
141 vl_api_hw_interface_set_mtu_reply_t *rmp;
153 rv = VNET_API_ERROR_INVALID_VALUE;
154 goto bad_sw_if_index;
162 rv = VNET_API_ERROR_FEATURE_DISABLED;
163 goto bad_sw_if_index;
166 if (mtu < hi->min_supported_packet_bytes)
168 rv = VNET_API_ERROR_INVALID_VALUE;
169 goto bad_sw_if_index;
172 if (mtu >
hi->max_supported_packet_bytes)
174 rv = VNET_API_ERROR_INVALID_VALUE;
175 goto bad_sw_if_index;
187 vl_api_sw_interface_set_mtu_reply_t *rmp;
198 per_protocol_mtu[
i] = ntohl (mp->
mtu[
i]);
210 vl_api_sw_interface_set_ip_directed_broadcast_reply_t *rmp;
220 REPLY_MACRO (VL_API_SW_INTERFACE_SET_IP_DIRECTED_BROADCAST_REPLY);
276 if (dev_class && dev_class->name)
281 if (
swif->sup_sw_if_index ==
swif->sw_if_index
291 else if (
swif->sup_sw_if_index !=
swif->sw_if_index)
305 u32 vtr_push_dot1q = 0, vtr_tag1 = 0, vtr_tag2 = 0;
308 &vtr_op, &vtr_push_dot1q, &vtr_tag1, &vtr_tag2) != 0)
312 clib_warning (
"cannot get vlan tag rewrite for sw_if_index %d",
317 mp->
vtr_op = ntohl (vtr_op);
332 &pbb_vtr_op, &outer_tag, ð_hdr, &b_vlanid, &i_sid))
365 u8 *filter = 0, *
name = 0;
391 char *strcasestr (
char *,
char *);
401 if (filter && !strcasestr((
char *)
name, (
char *) filter))
418 vl_api_sw_interface_add_del_address_reply_t *rmp;
450 REPLY_MACRO (VL_API_SW_INTERFACE_ADD_DEL_ADDRESS_REPLY);
456 vl_api_sw_interface_set_table_reply_t *rmp;
478 u32 fib_index, mfib_index;
499 if (~0 == fib_index || ~0 == mfib_index)
501 return (VNET_API_ERROR_NO_SUCH_FIB);
517 return (VNET_API_ERROR_ADDRESS_FOUND_FOR_INTERFACE);
562 return (VNET_API_ERROR_ADDRESS_FOUND_FOR_INTERFACE);
612 mp->
retval = htonl (retval);
655 vl_api_sw_interface_set_unnumbered_reply_t *rmp;
668 rv = VNET_API_ERROR_INVALID_SW_IF_INDEX;
675 rv = VNET_API_ERROR_INVALID_SW_IF_INDEX_2;
682 REPLY_MACRO (VL_API_SW_INTERFACE_SET_UNNUMBERED_REPLY);
689 vl_api_sw_interface_clear_stats_reply_t *rmp;
703 for (j = 0; j < n_counters; j++)
715 for (j = 0; j < n_counters; j++)
727 REPLY_MACRO (VL_API_SW_INTERFACE_CLEAR_STATS_REPLY);
772 uword *event_by_sw_if_index = 0;
777 uword *event_data = 0;
798 for (
i = 0;
i <
vec_len (event_by_sw_if_index);
i++)
800 if (event_by_sw_if_index[
i] == 0)
830 .name =
"vpe-link-state-process",
897 vl_api_sw_interface_tag_add_del_reply_t *rmp;
908 rv = VNET_API_ERROR_INVALID_VALUE;
921 REPLY_MACRO (VL_API_SW_INTERFACE_TAG_ADD_DEL_REPLY);
927 vl_api_sw_interface_add_del_mac_address_reply_t *rmp;
942 rv = VNET_API_ERROR_UNIMPLEMENTED;
949 REPLY_MACRO (VL_API_SW_INTERFACE_ADD_DEL_MAC_ADDRESS_REPLY);
955 vl_api_sw_interface_set_mac_address_reply_t *rmp;
971 rv = VNET_API_ERROR_UNIMPLEMENTED;
978 REPLY_MACRO (VL_API_SW_INTERFACE_SET_MAC_ADDRESS_REPLY);
1017 vl_api_sw_interface_set_interface_name_reply_t *rmp;
1024 if (mp->
name[0] == 0)
1026 rv = VNET_API_ERROR_INVALID_VALUE;
1031 rv = VNET_API_ERROR_INVALID_SW_IF_INDEX;
1039 rv = VNET_API_ERROR_INVALID_SW_IF_INDEX;
1043 REPLY_MACRO (VL_API_SW_INTERFACE_SET_INTERFACE_NAME_REPLY);
1049 vl_api_sw_interface_set_rx_mode_reply_t *rmp;
1062 rv = VNET_API_ERROR_INVALID_VALUE;
1063 goto bad_sw_if_index;
1074 rv = VNET_API_ERROR_UNIMPLEMENTED;
1081 REPLY_MACRO (VL_API_SW_INTERFACE_SET_RX_MODE_REPLY);
1146 goto bad_sw_if_index;
1152 clib_warning (
"interface type is not HARDWARE! P2P, PIPE and SUB"
1153 " interfaces are not supported");
1154 goto bad_sw_if_index;
1175 vl_api_sw_interface_set_rx_placement_reply_t *rmp;
1187 rv = VNET_API_ERROR_INVALID_VALUE;
1188 goto bad_sw_if_index;
1196 rv = VNET_API_ERROR_UNIMPLEMENTED;
1203 REPLY_MACRO (VL_API_SW_INTERFACE_SET_RX_PLACEMENT_REPLY);
1218 u64 sup_and_sub_key;
1228 rv = VNET_API_ERROR_BOND_SLAVE_NOT_ALLOWED;
1233 if (
id == 0 ||
id > 4095)
1235 rv = VNET_API_ERROR_INVALID_VLAN;
1239 sup_and_sub_key = ((
u64) (
hi->sw_if_index) << 32) | (
u64)
id;
1244 rv = VNET_API_ERROR_VLAN_ALREADY_EXISTS;
1251 template.sup_sw_if_index =
hi->sw_if_index;
1252 template.sub.id =
id;
1253 template.sub.eth.raw_flags = 0;
1254 template.sub.eth.flags.one_tag = 1;
1255 template.sub.eth.outer_vlan_id =
id;
1256 template.sub.eth.flags.exact_match = 1;
1262 rv = VNET_API_ERROR_INVALID_REGISTRATION;
1267 *kp = sup_and_sub_key;
1292 u32 sub_sw_if_index = ~0;
1301 rv = VNET_API_ERROR_BOND_SLAVE_NOT_ALLOWED;
1323 vl_api_delete_subif_reply_t *rmp;
1335 vl_api_interface_name_renumber_reply_t *rmp;
1345 REPLY_MACRO (VL_API_INTERFACE_NAME_RENUMBER_REPLY);
1379 is_specified, user_instance);
1392 vl_api_delete_loopback_reply_t *rmp;
1406 vl_api_collect_detailed_interface_stats_reply_t *rmp;
1413 REPLY_MACRO (VL_API_COLLECT_DETAILED_INTERFACE_STATS_REPLY);
1420 vl_api_sw_interface_address_replace_begin_reply_t *rmp;
1425 REPLY_MACRO (VL_API_SW_INTERFACE_ADDRESS_REPLACE_BEGIN_REPLY);
1432 vl_api_sw_interface_address_replace_end_reply_t *rmp;
1437 REPLY_MACRO (VL_API_SW_INTERFACE_ADDRESS_REPLACE_END_REPLY);
1450 #include <vnet/interface.api.c>
1457 am->is_mp_safe[VL_API_SW_INTERFACE_DUMP] = 1;
1458 am->is_mp_safe[VL_API_SW_INTERFACE_DETAILS] = 1;
1459 am->is_mp_safe[VL_API_SW_INTERFACE_TAG_ADD_DEL] = 1;
1460 am->is_mp_safe[VL_API_SW_INTERFACE_SET_INTERFACE_NAME] = 1;
1463 am->api_trace_cfg[VL_API_SW_INTERFACE_DUMP].replay_enable = 0;
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
@ SUB_IF_API_FLAG_MASK_VNET
VNET_SW_INTERFACE_ADMIN_UP_DOWN_FUNCTION(admin_up_down_function)
vl_api_interface_index_t sw_if_index
vnet_interface_main_t * im
Reply to get_sw_interface_vrf.
#define VALIDATE_SW_IF_INDEX(mp)
static vl_api_registration_t * vl_api_client_index_to_registration(u32 index)
clib_error_t * vnet_sw_interface_set_flags(vnet_main_t *vnm, u32 sw_if_index, vnet_sw_interface_flags_t flags)
VNET_HW_INTERFACE_LINK_UP_DOWN_FUNCTION(link_up_down_function)
static void vl_api_sw_interface_set_mac_address_t_handler(vl_api_sw_interface_set_mac_address_t *mp)
IP interface address replace begin.
struct vnet_sub_interface_t::@374 eth
@ VNET_SW_INTERFACE_TYPE_PIPE
#define hash_set(h, key, value)
vl_api_interface_index_t sw_if_index
vnet_sw_interface_type_t type
dump the rx queue placement of interface(s)
static void vl_api_collect_detailed_interface_stats_t_handler(vl_api_collect_detailed_interface_stats_t *mp)
static void vl_api_sw_interface_set_ip_directed_broadcast_t_handler(vl_api_sw_interface_set_ip_directed_broadcast_t *mp)
#define foreach_ip_interface_address(lm, a, sw_if_index, loop, body)
void vnet_hw_interface_set_mtu(vnet_main_t *vnm, u32 hw_if_index, u32 mtu)
struct _vnet_device_class vnet_device_class_t
struct vnet_sub_interface_t::@374::@375::@377 flags
#define REPLY_MACRO2(t, body)
ip_lookup_main_t lookup_main
static void vl_api_sw_interface_dump_t_handler(vl_api_sw_interface_dump_t *mp)
static void send_interface_rx_placement_details(vpe_api_main_t *am, vl_api_registration_t *rp, u32 sw_if_index, u32 worker_id, u32 queue_id, u8 mode, u32 context)
static_always_inline int vnet_hw_if_rxq_cmp_cli_api(vnet_hw_if_rx_queue_t **a, vnet_hw_if_rx_queue_t **b)
VNET_SW_INTERFACE_ADD_DEL_FUNCTION(sw_interface_add_del_function)
vl_api_if_status_flags_t flags
static vlib_node_registration_t link_state_process_node
(constructor) VLIB_REGISTER_NODE (link_state_process_node)
int vnet_interface_name_renumber(u32 sw_if_index, u32 new_show_dev_instance)
ip4_table_bind_callback_t * table_bind_callbacks
Functions to call when interface to table biding changes.
ip4_main_t ip4_main
Global ip4 main structure.
static void vl_api_send_msg(vl_api_registration_t *rp, u8 *elem)
@ IF_STATUS_API_FLAG_LINK_UP
u32 ethernet_set_flags(vnet_main_t *vnm, u32 hw_if_index, u32 flags)
static uword * vlib_process_wait_for_event(vlib_main_t *vm)
static_always_inline vnet_hw_if_rx_queue_t * vnet_hw_if_get_rx_queue(vnet_main_t *vnm, u32 queue_index)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
u32 l2pbb_get(vlib_main_t *vlib_main, vnet_main_t *vnet_main, u32 sw_if_index, u32 *vtr_op, u16 *outer_tag, ethernet_header_t *eth_hdr, u16 *b_vlanid, u32 *i_sid)
Get pbb tag rewrite on the given interface.
vl_api_interface_index_t sw_if_index
int vnet_create_sub_interface(u32 sw_if_index, u32 id, u32 flags, u16 inner_vlan_id, u16 outer_vlan_id, u32 *sub_sw_if_index)
void vlib_clear_combined_counters(vlib_combined_counter_main_t *cm)
Clear a collection of combined counters.
vl_api_interface_index_t sw_if_index
vl_api_interface_index_t sw_if_index
static void vl_api_sw_interface_address_replace_begin_t_handler(vl_api_sw_interface_address_replace_begin_t *mp)
static clib_error_t * admin_up_down_function(vnet_main_t *vm, u32 hw_if_index, u32 flags)
clib_error_t * set_hw_interface_rx_placement(u32 hw_if_index, u32 queue_id, u32 thread_index, u8 is_main)
Reply for get interface's MAC address request.
Create loopback interface response.
static void send_sw_interface_event(vpe_api_main_t *am, vpe_client_registration_t *reg, vl_api_registration_t *vl_reg, u32 sw_if_index, enum api_events events)
@ VNET_SW_INTERFACE_TYPE_SUB
u32 mfib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, mfib_source_t src)
Get the index of the FIB for a Table-ID.
IP interface address replace end.
static uword vnet_swif_is_api_visible(vnet_sw_interface_t *si)
static void send_sw_interface_get_table_reply(vl_api_registration_t *reg, u32 context, int retval, u32 vrf_id)
static void vl_api_sw_interface_address_replace_end_t_handler(vl_api_sw_interface_address_replace_end_t *mp)
Reply for the vlan subinterface create request.
@ VNET_FLOOD_CLASS_NORMAL
Set IP4 directed broadcast The directed broadcast enabled a packet sent to the interface's subnet add...
vl_api_interface_index_t sw_if_index
#define hash_set_mem(h, key, value)
vl_api_tunnel_mode_t mode
vl_api_if_status_flags_t flags
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
vnet_hw_interface_class_t ethernet_hw_interface_class
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static vnet_sw_interface_t * vnet_get_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
vl_api_interface_index_t sw_if_index
@ VNET_HW_INTERFACE_FLAG_LINK_UP
#define VNET_HW_INTERFACE_FLAG_DUPLEX_MASK
Set interface physical MTU.
static void vl_api_sw_interface_set_rx_mode_t_handler(vl_api_sw_interface_set_rx_mode_t *mp)
vlib_simple_counter_main_t * sw_if_counters
Set custom interface name Set custom interface name for the interface.
static vnet_device_class_t * vnet_get_device_class(vnet_main_t *vnm, u32 dev_class_index)
static void vl_api_sw_interface_add_del_mac_address_t_handler(vl_api_sw_interface_add_del_mac_address_t *mp)
#define clib_error_report(e)
vpe_api_main_t vpe_api_main
vnet_hw_if_rx_queue_t * hw_if_rx_queues
Set interface promiscuous mode.
static void vlib_process_signal_event(vlib_main_t *vm, uword node_index, uword type_opaque, uword data)
VLIB_API_INIT_FUNCTION(interface_api_hookup)
void vnet_sw_interface_ip_directed_broadcast(vnet_main_t *vnm, u32 sw_if_index, u8 enable)
clib_error_t * set_hw_interface_change_rx_mode(vnet_main_t *vnm, u32 hw_if_index, u8 queue_id_valid, u32 queue_id, vnet_hw_if_rx_mode mode)
ip6_table_bind_function_t * function
vl_api_interface_index_t sw_if_index
Get VRF id assigned to interface.
Create loopback interface instance response.
Interface details structure (fix this)
vl_api_mac_address_t b_dmac
static uword vnet_sw_interface_is_api_valid(vnet_main_t *vnm, u32 sw_if_index)
ip46_type_t ip_address_decode(const vl_api_address_t *in, ip46_address_t *out)
Decode/Encode for struct/union types.
static u8 * vnet_get_sw_interface_tag(vnet_main_t *vnm, u32 sw_if_index)
u32 * fib_index_by_sw_if_index
Table index indexed by software interface.
ethernet_interface_t * interfaces
static uword vlib_process_get_events(vlib_main_t *vm, uword **data_vector)
Return the first event type which has occurred and a vector of per-event data of that type,...
Request all or filtered subset of sw_interface_details.
vl_api_interface_index_t sw_if_index[default=0xFFFFFFFF]
A protocol Independent FIB table.
void ip_interface_address_mark(void)
void mfib_table_lock(u32 fib_index, fib_protocol_t proto, mfib_source_t source)
Release a reference counting lock on the table.
#define pool_foreach(VAR, POOL)
Iterate through pool.
Set or delete one or all ip addresses on a specified interface.
static void vl_api_sw_interface_set_unnumbered_t_handler(vl_api_sw_interface_set_unnumbered_t *mp)
enum mfib_source_t_ mfib_source_t
Possible [control plane] sources of MFIB entries.
clib_error_t * ip6_add_del_interface_address(vlib_main_t *vm, u32 sw_if_index, ip6_address_t *address, u32 address_length, u32 is_del)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
Clear interface statistics.
Create loopback interface instance request.
vl_api_interface_index_t sw_if_index
vl_api_interface_index_t sw_if_index
clib_error_t * ip4_add_del_interface_address(vlib_main_t *vm, u32 sw_if_index, ip4_address_t *address, u32 address_length, u32 is_del)
string interface_dev_type[64]
static void vl_api_sw_interface_rx_placement_dump_t_handler(vl_api_sw_interface_rx_placement_dump_t *mp)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
int vnet_sw_interface_update_unnumbered(u32 unnumbered_sw_if_index, u32 ip_sw_if_index, u8 enable)
clib_error_t * vnet_hw_interface_change_mac_address(vnet_main_t *vnm, u32 hw_if_index, const u8 *mac_address)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
vl_api_sub_if_flags_t sub_if_flags
static clib_error_t * link_up_down_function(vnet_main_t *vm, u32 hw_if_index, u32 flags)
@ IF_STATUS_API_FLAG_ADMIN_UP
vnet_main_t * vnet_get_main(void)
u32 ft_table_id
Table ID (hash key) for this FIB.
string interface_name[64]
Delete sub interface request.
#define VNET_HW_INTERFACE_FLAG_DUPLEX_SHIFT
u32 fib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, fib_source_t src)
Get the index of the FIB for a Table-ID.
Set an interface's rx-placement Rx-Queue placement on specific thread is operational for only hardwar...
An API client registration, only in vpp/vlib.
static void setup_message_id_table(api_main_t *am)
int vnet_create_loopback_interface(u32 *sw_if_indexp, u8 *mac_address, u8 is_specified, u32 user_instance)
vl_api_interface_index_t sw_if_index
vl_api_interface_index_t sw_if_index
vl_api_interface_index_t sw_if_index
clib_error_t * vnet_create_sw_interface(vnet_main_t *vnm, vnet_sw_interface_t *template, u32 *sw_if_index)
Create loopback interface request.
static void vlib_zero_combined_counter(vlib_combined_counter_main_t *cm, u32 index)
Clear a combined counter Clears the set of per-thread counters.
vl_api_interface_index_t sw_if_index
ip6_table_bind_callback_t * table_bind_callbacks
Functions to call when interface to table biding changes.
Set an interface's rx-mode.
#define REPLY_MSG_ID_BASE
static void vl_api_create_loopback_t_handler(vl_api_create_loopback_t *mp)
static api_main_t * vlibapi_get_main(void)
static void vnet_set_sw_interface_tag(vnet_main_t *vnm, u8 *tag, u32 sw_if_index)
static void vl_api_sw_interface_set_mtu_t_handler(vl_api_sw_interface_set_mtu_t *mp)
static perfmon_event_t events[]
static uword link_state_process(vlib_main_t *vm, vlib_node_runtime_t *rt, vlib_frame_t *f)
static void vl_api_sw_interface_set_table_t_handler(vl_api_sw_interface_set_table_t *mp)
vnet_feature_config_main_t * cm
vl_api_mac_address_t l2_address
static void vl_api_delete_loopback_t_handler(vl_api_delete_loopback_t *mp)
u32 * fib_index_by_sw_if_index
manual_print typedef address
@ API_SW_INTERFACE_ADD_EVENT
Set unnumbered interface add / del request.
#define VNET_HW_INTERFACE_BOND_INFO_SLAVE
enum fib_protocol_t_ fib_protocol_t
Protocol Type.
clib_error_t * vnet_rename_interface(vnet_main_t *vnm, u32 hw_if_index, char *new_name)
static void vl_api_create_vlan_subif_t_handler(vl_api_create_vlan_subif_t *mp)
vl_api_mac_address_t b_smac
vl_api_interface_index_t sw_if_index
Set / clear software interface tag.
Set flags on the interface.
vl_api_link_duplex_t link_duplex
struct _vlib_node_registration vlib_node_registration_t
vlib_combined_counter_main_t * combined_sw_if_counters
vnet_sw_interface_t * sw_interfaces
vl_api_interface_index_t sw_if_index
static void vl_api_sw_interface_set_promisc_t_handler(vl_api_sw_interface_set_promisc_t *mp)
void mfib_table_unlock(u32 fib_index, fib_protocol_t proto, mfib_source_t source)
Take a reference counting lock on the table.
ethernet_interface_t * ethernet_get_interface(ethernet_main_t *em, u32 hw_if_index)
static void vl_api_sw_interface_set_interface_name_t_handler(vl_api_sw_interface_set_interface_name_t *mp)
static void vl_api_sw_interface_add_del_address_t_handler(vl_api_sw_interface_add_del_address_t *mp)
vl_api_interface_index_t sw_if_index
#define BAD_SW_IF_INDEX_LABEL
vnet_api_error_t api_errno
static void vl_api_interface_name_renumber_t_handler(vl_api_interface_name_renumber_t *mp)
API main structure, used by both vpp and binary API clients.
static uword vnet_sw_if_index_is_api_valid(u32 sw_if_index)
@ API_SW_INTERFACE_DEL_EVENT
Delete loopback interface request.
#define hash_get_mem(h, key)
vl_api_interface_index_t sw_if_index
static void vl_api_create_loopback_instance_t_handler(vl_api_create_loopback_instance_t *mp)
#define vec_free(V)
Free vector's memory (no header).
int vnet_delete_loopback_interface(u32 sw_if_index)
vl_api_interface_index_t sw_if_index
u32 * mfib_index_by_sw_if_index
Table index indexed by software interface.
vl_api_sub_if_flags_t sub_if_flags
ethernet_main_t ethernet_main
vl_api_interface_index_t sw_if_index
void mac_address_encode(const mac_address_t *in, u8 *out)
u32 mfib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
description fragment has unexpected format
A collection of combined counters.
static void vlib_zero_simple_counter(vlib_simple_counter_main_t *cm, u32 index)
Clear a simple counter Clears the set of per-thread u16 counters, and the u64 counter.
int ip_table_bind(fib_protocol_t fproto, u32 sw_if_index, u32 table_id, u8 is_api)
#define vec_validate_init_empty(V, I, INIT)
Make sure vector is long enough for given index and initialize empty space (no header,...
fib_table_t * fib_table_get(fib_node_index_t index, fib_protocol_t proto)
Get a pointer to a FIB table.
u32 fib_table_get_index_for_sw_if_index(fib_protocol_t proto, u32 sw_if_index)
Get the index of the FIB bound to the interface.
static void vl_api_delete_subif_t_handler(vl_api_delete_subif_t *mp)
void fib_table_lock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Release a reference counting lock on the table.
u32 * mfib_index_by_sw_if_index
Table index indexed by software interface.
static vnet_hw_interface_t * vnet_get_sup_hw_interface(vnet_main_t *vnm, u32 sw_if_index)
Set an interface's MAC address.
void ip_interface_address_sweep(void)
u32 l2vtr_get(vlib_main_t *vlib_main, vnet_main_t *vnet_main, u32 sw_if_index, u32 *vtr_op, u32 *push_dot1q, u32 *vtr_tag1, u32 *vtr_tag2)
Get vtag tag rewrite on the given interface.
static void vnet_clear_sw_interface_tag(vnet_main_t *vnm, u32 sw_if_index)
pub_sub_handler(interface_events, INTERFACE_EVENTS)
Interface Event generated by want_interface_events.
#define vec_foreach(var, vec)
Vector iterator.
static clib_error_t * interface_api_hookup(vlib_main_t *vm)
vl_api_interface_index_t sw_if_index
@ FIB_SOURCE_CLI
From the CLI.
void mac_address_decode(const u8 *in, mac_address_t *out)
Conversion functions to/from (decode/encode) API types to VPP internal types.
ip4_table_bind_function_t * function
@ FIB_SOURCE_API
From the control plane API.
ip_lookup_main_t lookup_main
foreach_registration_hash u8 link_state_process_up
static void vl_api_sw_interface_set_rx_placement_t_handler(vl_api_sw_interface_set_rx_placement_t *mp)
int vnet_sw_interface_stats_collect_enable_disable(u32 sw_if_index, u8 enable)
u8 * vl_api_from_api_to_new_vec(void *mp, vl_api_string_t *astr)
vl_api_interface_index_t sw_if_index
#define vec_sort_with_function(vec, f)
Sort a vector using the supplied element comparison function.
vl_api_interface_index_t sw_if_index
static void send_sw_interface_details(vpe_api_main_t *am, vl_api_registration_t *rp, vnet_sw_interface_t *swif, u8 *interface_name, u32 context)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
clib_error_t * vnet_hw_interface_add_del_mac_address(vnet_main_t *vnm, u32 hw_if_index, const u8 *mac_address, u8 is_add)
vl_api_mac_address_t mac_address
A collection of simple counters.
Enable or disable detailed interface stats.
vl_api_interface_index_t sw_if_index
static vlib_main_t * vlib_get_main(void)
Associate the specified interface with a fib table.
vl_api_interface_index_t sw_if_index
vnet_interface_output_runtime_t * rt
#define ETHERNET_INTERFACE_FLAG_ACCEPT_ALL
static void vl_api_sw_interface_tag_add_del_t_handler(vl_api_sw_interface_tag_add_del_t *mp)
static void vl_api_sw_interface_set_flags_t_handler(vl_api_sw_interface_set_flags_t *mp)
uword * sw_if_index_by_sup_and_sub
void fib_table_unlock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Take a reference counting lock on the table.
@ VNET_SW_INTERFACE_TYPE_P2P
static void vl_api_sw_interface_clear_stats_t_handler(vl_api_sw_interface_clear_stats_t *mp)
@ API_LINK_STATE_UP_EVENT
#define clib_warning(format, args...)
vl_api_mac_address_t mac_address
enum fib_source_t_ fib_source_t
The different sources that can create a route.
#define clib_error_free(e)
vl_api_if_status_flags_t flags
vl_api_interface_index_t sw_if_index
vl_api_interface_index_t sw_if_index
Add or delete a secondary MAC address on an interface.
@ API_LINK_STATE_DOWN_EVENT
vl_api_mac_address_t mac_address
static void vl_api_create_subif_t_handler(vl_api_create_subif_t *mp)
static void vl_api_sw_interface_get_mac_address_t_handler(vl_api_sw_interface_get_mac_address_t *mp)
@ VNET_SW_INTERFACE_TYPE_HARDWARE
Get interface's MAC address.
static void vl_api_sw_interface_get_table_t_handler(vl_api_sw_interface_get_table_t *mp)
vl_api_interface_index_t sw_if_index
static vnet_sw_interface_t * vnet_get_sup_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
vl_api_address_with_prefix_t prefix
u32 new_show_dev_instance
u32 fib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
vl_api_interface_index_t sw_if_index
show the interface's queue - thread placement This api is used to display the interface and queue wor...
void vlib_clear_simple_counters(vlib_simple_counter_main_t *cm)
Clear a collection of simple counters.
ethernet_interface_address_t address
void ip_del_all_interface_addresses(vlib_main_t *vm, u32 sw_if_index)
vl_api_mac_address_t addr
static clib_error_t * sw_interface_add_del_function(vnet_main_t *vm, u32 sw_if_index, u32 flags)
static void * clib_mem_alloc(uword size)
int vnet_delete_sub_interface(u32 sw_if_index)
vnet_interface_main_t interface_main
Create a new subinterface with the given vlan id.
ethernet_main_t * ethernet_get_main(vlib_main_t *vm)
vl_api_interface_index_t sw_if_index
void vnet_sw_interface_set_protocol_mtu(vnet_main_t *vnm, u32 sw_if_index, u32 mtu[])
static void vl_api_hw_interface_set_mtu_t_handler(vl_api_hw_interface_set_mtu_t *mp)
format_function_t format_vnet_sw_interface_name
vl_api_interface_index_t sw_if_index
void * vl_msg_api_alloc(int nbytes)
#define VLIB_REGISTER_NODE(x,...)
vl_api_mac_address_t mac_address
vl_api_interface_index_t unnumbered_sw_if_index
vl_api_wireguard_peer_flags_t flags