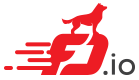 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
74 u32 hw_if_index, *hw_if_indices = 0;
82 vec_add1 (hw_if_indices, hw_if_index);
85 else if (
unformat (input,
"%u", &hw_if_index))
86 vec_add1 (hw_if_indices, hw_if_index);
88 else if (
unformat (input,
"verbose"))
113 if (
vec_len (hw_if_indices) == 0)
132 else if ((
hi->bond_info) &&
160 if (dc->clear_counters)
161 dc->clear_counters (
hi->dev_instance);
243 .path =
"show hardware-interfaces",
244 .short_help =
"show hardware-interfaces [brief|verbose|detail] [bond] "
245 "[<interface> [<interface> [..]]] [<sw_idx> [<sw_idx> [..]]]",
264 .path =
"clear hardware-interfaces",
265 .short_help =
"clear hardware-interfaces "
266 "[<interface> [<interface> [..]]] [<sw_idx> [<sw_idx> [..]]]",
291 u8 show_addresses = 0;
292 u8 show_features = 0;
320 else if (
unformat (linput,
"verbose"))
347 if (show_features || show_tag || show_vtr)
376 tag ? (
char *) tag :
"(none)");
387 u32 push_dot1q = 0, tag1 = 0, tag2 = 0;
390 &vtr_op, &push_dot1q, &tag1, &tag2) != 0)
410 _vec_len (sorted_sis) = 0;
432 u32 fib_index4 = 0, fib_index6 = 0;
447 (
vm,
"%U (%s): \n unnumbered, use %U",
465 ip4_address_t *r4 = ip_interface_address_get_address (lm4, ia);
466 if (fib4->hash.table_id)
468 vm,
" L3 %U/%d ip4 table-id %d fib-idx %d", format_ip4_address,
469 r4, ia->address_length, fib4->hash.table_id,
470 ip4_fib_index_from_table_id (fib4->hash.table_id));
472 vlib_cli_output (vm,
" L3 %U/%d",
473 format_ip4_address, r4, ia->address_length);
482 ip6_address_t *r6 = ip_interface_address_get_address (lm6, ia);
484 vlib_cli_output (vm,
" L3 %U/%d ip6 table-id %d fib-idx %d",
485 format_ip6_address, r6, ia->address_length,
487 ip6_fib_index_from_table_id (fib6->table_id));
489 vlib_cli_output (vm,
" L3 %U/%d",
490 format_ip6_address, r6, ia->address_length);
510 .path =
"show interface",
511 .short_help =
"show interface [address|addr|features|feat|vtr] [<interface> [<interface> [..]]] [verbose]",
521 .short_help =
"Interface commands",
527 .path =
"set interface",
528 .short_help =
"Interface commands",
544 for (j = 0; j < n_counters; j++)
553 for (j = 0; j < n_counters; j++)
573 .path =
"clear interfaces",
574 .short_help =
"clear interfaces",
646 u32 inner_vlan, outer_vlan;
648 if (
unformat (input,
"any inner-dot1q any"))
650 template->sub.eth.flags.two_tags = 1;
651 template->sub.eth.flags.outer_vlan_id_any = 1;
652 template->sub.eth.flags.inner_vlan_id_any = 1;
656 template->sub.eth.flags.one_tag = 1;
657 template->sub.eth.flags.outer_vlan_id_any = 1;
659 else if (
unformat (input,
"%d inner-dot1q any", &outer_vlan))
661 template->sub.eth.flags.two_tags = 1;
662 template->sub.eth.flags.inner_vlan_id_any = 1;
663 template->sub.eth.outer_vlan_id = outer_vlan;
665 else if (
unformat (input,
"%d inner-dot1q %d", &outer_vlan, &inner_vlan))
667 template->sub.eth.flags.two_tags = 1;
668 template->sub.eth.outer_vlan_id = outer_vlan;
669 template->sub.eth.inner_vlan_id = inner_vlan;
671 else if (
unformat (input,
"%d", &outer_vlan))
673 template->sub.eth.flags.one_tag = 1;
674 template->sub.eth.outer_vlan_id = outer_vlan;
685 if (
unformat (input,
"exact-match"))
687 template->sub.eth.flags.exact_match = 1;
703 u32 id, id_min, id_max;
715 template.sub.eth.raw_flags = 0;
717 if (
unformat (input,
"%d default", &id_min))
720 template.sub.eth.flags.default_sub = 1;
722 else if (
unformat (input,
"%d untagged", &id_min))
725 template.sub.eth.flags.no_tags = 1;
726 template.sub.eth.flags.exact_match = 1;
728 else if (
unformat (input,
"%d dot1q", &id_min))
736 else if (
unformat (input,
"%d dot1ad", &id_min))
740 template.sub.eth.flags.dot1ad = 1;
745 else if (
unformat (input,
"%d-%d", &id_min, &id_max))
747 template.sub.eth.flags.one_tag = 1;
748 template.sub.eth.flags.exact_match = 1;
752 else if (
unformat (input,
"%d", &id_min))
755 template.sub.eth.flags.one_tag = 1;
756 template.sub.eth.outer_vlan_id = id_min;
757 template.sub.eth.flags.exact_match = 1;
773 "not allowed as %v belong to a BondEthernet interface",
778 for (
id = id_min;
id <= id_max;
id++)
782 u64 sup_and_sub_key = ((
u64) (
hi->sw_if_index) << 32) | (
u64)
id;
789 clib_warning (
"sup sw_if_index %d, sub id %d already exists\n",
790 hi->sw_if_index,
id);
796 template.sup_sw_if_index =
hi->sw_if_index;
797 template.sub.id =
id;
799 template.sub.eth.outer_vlan_id =
id;
806 *kp = sup_and_sub_key;
904 .path =
"create sub-interfaces",
905 .short_help =
"create sub-interfaces <interface> "
906 "{<subId> [default|untagged]} | "
907 "{<subId>-<subId>} | "
908 "{<subId> dot1q|dot1ad <vlanId>|any [inner-dot1q <vlanId>|any] [exact-match]}",
961 .path =
"set interface state",
962 .short_help =
"set interface state <interface> [up|down|punt|enable]",
972 u32 unnumbered_sw_if_index = ~0;
973 u32 inherit_from_sw_if_index = ~0;
982 &unnumbered_sw_if_index))
988 if (~0 == unnumbered_sw_if_index)
990 if (enable && ~0 == inherit_from_sw_if_index)
992 " IP enabled interface that it uses");
995 unnumbered_sw_if_index, inherit_from_sw_if_index, enable);
1002 case VNET_API_ERROR_UNEXPECTED_INTF_STATE:
1005 "When enabling unnumbered both interfaces must be in the same tables");
1009 0,
"vnet_sw_interface_update_unnumbered returned %d",
rv);
1017 .path =
"set interface unnumbered",
1018 .short_help =
"set interface unnumbered [<interface> use <interface> | del <interface>]",
1032 u32 hw_if_index, hw_class_index;
1060 .path =
"set interface hw-class",
1061 .short_help =
"Set interface hardware class",
1080 u32 new_dev_instance;
1088 if (!
unformat (input,
"%d", &new_dev_instance))
1110 .path =
"renumber interface",
1111 .short_help =
"renumber interface <interface> <new-dev-instance>",
1129 else if (
unformat (input,
"off %U",
1146 .path =
"set interface promiscuous",
1147 .short_help =
"set interface promiscuous [on|off] <interface>",
1160 if (
unformat (input,
"%d %U", &mtu,
1173 if (mtu < hi->min_supported_packet_bytes)
1175 "must be >= min pkt bytes (%d)", mtu,
1176 hi->min_supported_packet_bytes);
1178 if (mtu >
hi->max_supported_packet_bytes)
1180 hi->max_supported_packet_bytes);
1185 else if (
unformat (input,
"packet %d %U", &mtu,
1189 else if (
unformat (input,
"ip4 %d %U", &mtu,
1192 else if (
unformat (input,
"ip6 %d %U", &mtu,
1195 else if (
unformat (input,
"mpls %d %U", &mtu,
1210 .path =
"set interface mtu",
1211 .short_help =
"set interface mtu [packet|ip4|ip6|mpls] <value> <interface>",
1233 if (
vec_len (sorted_sis) == 0)
1237 _vec_len (sorted_sis) = 0;
1288 .path =
"show interface secondary-mac-address",
1289 .short_help =
"show interface secondary-mac-address [<interface>]",
1305 is_add = is_del = 0;
1330 if (is_add == is_del)
1362 .path =
"set interface secondary-mac-address",
1363 .short_help =
"set interface secondary-mac-address <interface> <mac-address> [(add|del)]",
1412 .path =
"set interface mac address",
1413 .short_help =
"set interface mac address <interface> <mac-address>",
1437 .path =
"set interface tag",
1438 .short_help =
"set interface tag <interface> <tag>",
1461 .path =
"clear interface tag",
1462 .short_help =
"clear interface tag <interface>",
1476 else if (
unformat (input,
"enable"))
1478 else if (
unformat (input,
"disable"))
1501 .path =
"set interface ip directed-broadcast",
1502 .short_help =
"set interface enable <interface> <enable|disable>",
1509 u8 queue_id_valid,
u32 queue_id,
1514 u32 *queue_indices = 0;
1523 if (queue_index == ~0)
1525 queue_id, hw->
name);
1526 vec_add1 (queue_indices, queue_index);
1531 for (
int i = 0;
i <
vec_len (queue_indices);
i++)
1552 u32 hw_if_index = (
u32) ~ 0;
1553 u32 queue_id = (
u32) ~ 0;
1555 u8 queue_id_valid = 0;
1565 else if (
unformat (line_input,
"queue %d", &queue_id))
1567 else if (
unformat (line_input,
"polling"))
1569 else if (
unformat (line_input,
"interrupt"))
1571 else if (
unformat (line_input,
"adaptive"))
1584 if (hw_if_index == (
u32) ~ 0)
1631 .path =
"set interface rx-mode",
1632 .short_help =
"set interface rx-mode <interface> [queue <n>] [polling | interrupt | adaptive]",
1657 if (current_node != prev_node)
1662 if (qptr == all_queues +
vec_len (all_queues) - 1 ||
1669 prev_node = current_node;
1703 .path =
"show interface rx-placement",
1704 .short_help =
"show interface rx-placement",
1724 "please specify valid worker thread or main");
1730 if (queue_index == ~0)
1745 u32 hw_if_index = (
u32) ~ 0;
1758 else if (
unformat (line_input,
"queue %d", &queue_id))
1775 if (hw_if_index == (
u32) ~ 0)
1834 .path =
"set interface rx-placement",
1835 .short_help =
"set interface rx-placement <interface> [queue <n>] "
1836 "[worker <n> | main]",
1861 if (queue_index == ~0)
1888 u32 hw_if_index = (
u32) ~0;
1900 else if (
unformat (line_input,
"queue %d", &queue_id))
1916 if (hw_if_index == (
u32) ~0)
1930 .path =
"set interface tx-queue",
1931 .short_help =
"set interface tx-queue <interface> queue <n> "
1955 u32 hw_if_index = (
u32) ~ 0;
1980 if (hw_if_index == (
u32) ~ 0)
2014 .path =
"set interface rss queues",
2015 .short_help =
"set interface rss queues <interface> <list <queue-list>>",
2024 int type = va_arg (*args,
int);
2069 (
vm,
"pcap %U dispatch capture enabled: %d of %d pkts...",
2084 && (
a->rx_enable +
a->tx_enable +
a->drop_enable))
2085 return VNET_API_ERROR_INVALID_VALUE;
2090 ((
a->rx_enable +
a->tx_enable +
a->drop_enable == 0)))
2091 return VNET_API_ERROR_VALUE_EXIST;
2095 && (
a->rx_enable +
a->tx_enable +
a->drop_enable)
2097 return VNET_API_ERROR_INVALID_VALUE_2;
2100 if ((
a->rx_enable +
a->tx_enable +
a->drop_enable) &&
a->filter &&
2101 (!
cm->classify_table_index_by_sw_if_index ||
2102 cm->classify_table_index_by_sw_if_index[0] == ~0))
2103 return VNET_API_ERROR_NO_SUCH_LABEL;
2105 if (
a->rx_enable +
a->tx_enable +
a->drop_enable)
2107 void *save_pcap_data;
2110 if (
a->max_bytes_per_pkt < 32 ||
a->max_bytes_per_pkt > 9000)
2111 return VNET_API_ERROR_INVALID_MEMORY_SIZE;
2122 memset (pm, 0,
sizeof (*pm));
2131 if (
a->filename == 0)
2136 stem =
format (stem,
"rx");
2138 stem =
format (stem,
"tx");
2140 stem =
format (stem,
"drop");
2141 a->filename =
format (0,
"/tmp/%v.pcap%c", stem, 0);
2149 if (
a->preallocate_data)
2160 cm->classify_table_index_by_sw_if_index[0];
2189 return VNET_API_ERROR_SYSCALL_ERROR_1;
2197 return VNET_API_ERROR_NO_SUCH_ENTRY;
2212 u32 max_bytes_per_pkt = 512;
2216 int preallocate_data = 0;
2217 int drop_enable = 0;
2232 else if (
unformat (line_input,
"tx"))
2234 else if (
unformat (line_input,
"drop"))
2236 else if (
unformat (line_input,
"off"))
2237 rx_enable = tx_enable = drop_enable = 0;
2238 else if (
unformat (line_input,
"max-bytes-per-pkt %u",
2239 &max_bytes_per_pkt))
2241 else if (
unformat (line_input,
"max %d", &max))
2243 else if (
unformat (line_input,
"packets-to-capture %d", &max))
2248 else if (
unformat (line_input,
"status %=", &status, 1))
2250 else if (
unformat (line_input,
"intfc %U",
2253 else if (
unformat (line_input,
"interface %U",
2259 else if (
unformat (line_input,
"preallocate-data %=",
2260 &preallocate_data, 1))
2262 else if (
unformat (line_input,
"free-data %=", &free_data, 1))
2264 else if (
unformat (line_input,
"intfc any")
2265 ||
unformat (line_input,
"interface any"))
2267 else if (
unformat (line_input,
"filter"))
2279 a->filename = filename;
2280 a->rx_enable = rx_enable;
2281 a->tx_enable = tx_enable;
2282 a->preallocate_data = preallocate_data;
2283 a->free_data = free_data;
2284 a->drop_enable = drop_enable;
2286 a->packets_to_capture = max;
2289 a->max_bytes_per_pkt = max_bytes_per_pkt;
2290 a->drop_err = drop_err;
2299 case VNET_API_ERROR_INVALID_VALUE:
2302 case VNET_API_ERROR_VALUE_EXIST:
2305 case VNET_API_ERROR_INVALID_VALUE_2:
2307 (0,
"can't change number of records to capture while tracing...");
2309 case VNET_API_ERROR_SYSCALL_ERROR_1:
2312 case VNET_API_ERROR_NO_SUCH_ENTRY:
2315 case VNET_API_ERROR_INVALID_MEMORY_SIZE:
2317 "Max bytes per pkt must be > 32, < 9000...");
2319 case VNET_API_ERROR_NO_SUCH_LABEL:
2321 (0,
"No classify filter configured, see 'classify filter...'");
2411 .path =
"pcap trace",
2413 "pcap trace [rx] [tx] [drop] [off] [max <nn>] [intfc <interface>|any]\n"
2414 " [file <name>] [status] [max-bytes-per-pkt <nnnn>][filter]\n"
2415 " [preallocate-data][free-data]",
2427 u32 hw_if_index = ~0;
2436 &hw_if_index, &
name))
2450 if (hw_if_index == (
u32) ~0 ||
name == 0)
2464 .path =
"set interface name",
2465 .short_help =
"set interface name <interface-name> <new-interface-name>",
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
static void clib_spinlock_init(clib_spinlock_t *p)
static vlib_cli_command_t clear_interface_counters_command
(constructor) VLIB_CLI_COMMAND (clear_interface_counters_command)
uword last_worker_thread_index
vnet_device_main_t vnet_device_main
vnet_interface_main_t * im
static clib_error_t * show_interface_sec_mac_addr_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
uword unformat_ethernet_address(unformat_input_t *input, va_list *args)
clib_error_t * vnet_sw_interface_set_flags(vnet_main_t *vnm, u32 sw_if_index, vnet_sw_interface_flags_t flags)
static clib_error_t * set_interface_tx_queue(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
uword * hw_interface_class_by_name
#define hash_set(h, key, value)
static vlib_cli_command_t set_hw_class_command
(constructor) VLIB_CLI_COMMAND (set_hw_class_command)
#define foreach_ip_interface_address(lm, a, sw_if_index, loop, body)
void vnet_hw_interface_set_mtu(vnet_main_t *vnm, u32 hw_if_index, u32 mtu)
@ VNET_HW_IF_RX_MODE_ADAPTIVE
static clib_error_t * show_interface_rx_placement_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
struct _vnet_device_class vnet_device_class_t
static void show_bond(vlib_main_t *vm)
ip_lookup_main_t lookup_main
clib_error_t * vnet_hw_interface_set_class(vnet_main_t *vnm, u32 hw_if_index, u32 hw_class_index)
static vlib_cli_command_t clear_tag_command
(constructor) VLIB_CLI_COMMAND (clear_tag_command)
static clib_error_t * renumber_interface_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static_always_inline int vnet_hw_if_rxq_cmp_cli_api(vnet_hw_if_rx_queue_t **a, vnet_hw_if_rx_queue_t **b)
#define vec_new(T, N)
Create new vector of given type and length (unspecified alignment, no header).
clib_error_t * vnet_hw_interface_set_rss_queues(vnet_main_t *vnm, vnet_hw_interface_t *hi, clib_bitmap_t *bitmap)
static clib_error_t * clear_tag(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u32 vnet_hw_if_get_tx_queue_index_by_id(vnet_main_t *vnm, u32 hw_if_index, u32 queue_id)
int vnet_interface_name_renumber(u32 sw_if_index, u32 new_show_dev_instance)
static clib_error_t * set_interface_name(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
ip4_main_t ip4_main
Global ip4 main structure.
static clib_error_t * clear_interface_counters(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u32 ethernet_set_flags(vnet_main_t *vnm, u32 hw_if_index, u32 flags)
int vnet_hw_if_set_rx_queue_mode(vnet_main_t *vnm, u32 queue_index, vnet_hw_if_rx_mode mode)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
void vlib_clear_combined_counters(vlib_combined_counter_main_t *cm)
Clear a collection of combined counters.
u8 * format_l2_output_features(u8 *s, va_list *args)
__clib_export clib_error_t * pcap_close(pcap_main_t *pm)
Close PCAP file.
clib_error_t * set_hw_interface_rx_placement(u32 hw_if_index, u32 queue_id, u32 thread_index, u8 is_main)
vnet_classify_main_t vnet_classify_main
static clib_error_t * clear_hw_interfaces(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static clib_error_t * set_interface_rx_mode(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
@ VNET_SW_INTERFACE_TYPE_SUB
static vlib_cli_command_t set_interface_promiscuous_cmd
(constructor) VLIB_CLI_COMMAND (set_interface_promiscuous_cmd)
#define clib_error_return(e, args...)
static uword vnet_swif_is_api_visible(vnet_sw_interface_t *si)
@ VNET_FLOOD_CLASS_NORMAL
void vnet_hw_if_tx_queue_assign_thread(vnet_main_t *vnm, u32 queue_index, u32 thread_index)
static clib_error_t * promiscuous_cmd(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static int compare_interface_names(void *a1, void *a2)
#define hash_set_mem(h, key, value)
vl_api_tunnel_mode_t mode
static vlib_cli_command_t show_interface_rx_placement
(constructor) VLIB_CLI_COMMAND (show_interface_rx_placement)
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
@ VNET_HW_IF_RX_MODE_POLLING
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static vlib_cli_command_t set_interface_mac_address_cmd
(constructor) VLIB_CLI_COMMAND (set_interface_mac_address_cmd)
static vnet_sw_interface_t * vnet_get_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
vlib_simple_counter_main_t * sw_if_counters
pcap_packet_type_t packet_type
Packet type.
vnet_hw_interface_t * hw_interfaces
static vlib_cli_command_t show_hw_interfaces_command
(constructor) VLIB_CLI_COMMAND (show_hw_interfaces_command)
#define clib_error_report(e)
PCAP main state data structure.
static vlib_cli_command_t cmd_set_if_name
(constructor) VLIB_CLI_COMMAND (cmd_set_if_name)
vnet_hw_if_rx_queue_t * hw_if_rx_queues
vlib_worker_thread_t * vlib_worker_threads
static vlib_cli_command_t pcap_tx_trace_command
(constructor) VLIB_CLI_COMMAND (pcap_tx_trace_command)
static clib_error_t * vnet_interface_cli_init(vlib_main_t *vm)
static clib_error_t * set_tag(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static ip6_fib_t * ip6_fib_get(fib_node_index_t index)
static u8 * format_vnet_pcap(u8 *s, va_list *args)
uword unformat_ethernet_interface(unformat_input_t *input, va_list *args)
void vnet_sw_interface_ip_directed_broadcast(vnet_main_t *vnm, u32 sw_if_index, u8 enable)
clib_error_t * set_hw_interface_change_rx_mode(vnet_main_t *vnm, u32 hw_if_index, u8 queue_id_valid, u32 queue_id, vnet_hw_if_rx_mode mode)
word vnet_sw_interface_compare(vnet_main_t *vnm, uword sw_if_index0, uword sw_if_index1)
static vlib_cli_command_t set_ip_directed_broadcast_command
(constructor) VLIB_CLI_COMMAND (set_ip_directed_broadcast_command)
vnet_sw_interface_flags_t flags
clib_spinlock_t lock
spinlock to protect e.g.
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
clib_error_t * set_hw_interface_tx_queue(u32 hw_if_index, u32 queue_id, uword *bitmap)
#define vec_elt(v, i)
Get vector value at index i.
static u8 * vnet_get_sw_interface_tag(vnet_main_t *vnm, u32 sw_if_index)
static clib_error_t * set_ip_directed_broadcast(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u32 * fib_index_by_sw_if_index
Table index indexed by software interface.
#define pool_foreach(VAR, POOL)
Iterate through pool.
static clib_error_t * set_hw_class(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
unformat_function_t unformat_vlib_error
format_function_t format_vnet_hw_if_rx_mode
static vlib_cli_command_t vnet_cli_set_interface_command
(constructor) VLIB_CLI_COMMAND (vnet_cli_set_interface_command)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
u32 vnet_hw_if_get_rx_queue_index_by_id(vnet_main_t *vnm, u32 hw_if_index, u32 queue_id)
int vnet_sw_interface_update_unnumbered(u32 unnumbered_sw_if_index, u32 ip_sw_if_index, u8 enable)
clib_error_t * vnet_hw_interface_change_mac_address(vnet_main_t *vnm, u32 hw_if_index, const u8 *mac_address)
static clib_error_t * interface_add_del_mac_address(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
vnet_main_t * vnet_get_main(void)
static uword clib_bitmap_count_set_bits(uword *ai)
Return the number of set bits in a bitmap.
static clib_error_t * set_unnumbered(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u8 * format_mac_address_t(u8 *s, va_list *args)
#define vec_validate_aligned(V, I, A)
Make sure vector is long enough for given index (no header, specified alignment)
static vlib_cli_command_t cmd_set_if_rx_mode
(constructor) VLIB_CLI_COMMAND (cmd_set_if_rx_mode)
static clib_error_t * mtu_cmd(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static vlib_cli_command_t cmd_set_if_rx_placement
(constructor) VLIB_CLI_COMMAND (cmd_set_if_rx_placement)
static clib_error_t * set_interface_rss_queues_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
clib_error_t * vnet_create_sw_interface(vnet_main_t *vnm, vnet_sw_interface_t *template, u32 *sw_if_index)
static vlib_cli_command_t interface_add_del_mac_address_cmd
(constructor) VLIB_CLI_COMMAND (interface_add_del_mac_address_cmd)
#define clib_bitmap_free(v)
Free a bitmap.
static vlib_cli_command_t cmd_set_if_tx_queue
(constructor) VLIB_CLI_COMMAND (cmd_set_if_tx_queue)
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
void vnet_hw_if_update_runtime_data(vnet_main_t *vnm, u32 hw_if_index)
static vlib_cli_command_t show_sw_interfaces_command
(constructor) VLIB_CLI_COMMAND (show_sw_interfaces_command)
@ VNET_HW_IF_RX_MODE_INTERRUPT
static void vnet_set_sw_interface_tag(vnet_main_t *vnm, u8 *tag, u32 sw_if_index)
unformat_function_t unformat_hash_string
static_always_inline vnet_hw_if_tx_queue_t * vnet_hw_if_get_tx_queue(vnet_main_t *vnm, u32 queue_index)
static ip4_fib_t * ip4_fib_get(u32 index)
Get the FIB at the given index.
vnet_feature_config_main_t * cm
u8 * pcap_data
Vector of pcap data.
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
u32 * fib_index_by_sw_if_index
@ VNET_SW_INTERFACE_FLAG_UNNUMBERED
#define VLIB_CLI_COMMAND(x,...)
vnet_device_class_t * device_classes
u32 filter_classify_table_index
#define VNET_HW_INTERFACE_BOND_INFO_SLAVE
clib_error_t * vnet_rename_interface(vnet_main_t *vnm, u32 hw_if_index, char *new_name)
static vlib_cli_command_t set_interface_mtu_cmd
(constructor) VLIB_CLI_COMMAND (set_interface_mtu_cmd)
clib_error_t * set_interface_rss_queues(vlib_main_t *vm, u32 hw_if_index, clib_bitmap_t *bitmap)
format_function_t format_vnet_hw_interface
unformat_function_t unformat_vnet_hw_interface
static clib_error_t * set_interface_rx_placement(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define CLIB_CACHE_LINE_BYTES
vlib_combined_counter_main_t * combined_sw_if_counters
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
vnet_sw_interface_t * sw_interfaces
static int sw_interface_name_compare(void *a1, void *a2)
ethernet_interface_t * ethernet_get_interface(ethernet_main_t *em, u32 hw_if_index)
u32 unnumbered_sw_if_index
static clib_error_t * show_sw_interfaces(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static vlib_cli_command_t create_sub_interfaces_command
(constructor) VLIB_CLI_COMMAND (create_sub_interfaces_command)
static vlib_cli_command_t cmd_set_interface_rss_queues
(constructor) VLIB_CLI_COMMAND (cmd_set_interface_rss_queues)
l2_output_config_t * l2output_intf_config(u32 sw_if_index)
Get a pointer to the config for the given interface.
static clib_error_t * set_interface_mac_address(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define hash_get_mem(h, key)
uword first_worker_thread_index
void vnet_interface_features_show(vlib_main_t *vm, u32 sw_if_index, int verbose)
Display the set of driver features configured on a specific interface Called by "show interface" hand...
#define vec_free(V)
Free vector's memory (no header).
format_function_t format_vlib_node_name
static vlib_cli_command_t set_tag_command
(constructor) VLIB_CLI_COMMAND (set_tag_command)
ethernet_main_t ethernet_main
format_function_t format_vnet_sw_if_index_name
u8 * vnet_trace_placeholder
unformat_function_t unformat_vnet_sw_interface
description fragment has unexpected format
A collection of combined counters.
#define VLIB_INIT_FUNCTION(x)
format_function_t format_vtr
u32 l2vtr_get(vlib_main_t *vlib_main, vnet_main_t *vnet_main, u32 sw_if_index, u32 *vtr_op, u32 *push_dot1q, u32 *vtr_tag1, u32 *vtr_tag2)
Get vtag tag rewrite on the given interface.
static clib_error_t * show_or_clear_hw_interfaces(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd, int is_show)
static void vnet_clear_sw_interface_tag(vnet_main_t *vnm, u32 sw_if_index)
#define vec_foreach(var, vec)
Vector iterator.
unformat_function_t unformat_vnet_sw_interface_flags
struct _vnet_classify_main vnet_classify_main_t
static uword pool_elts(void *v)
Number of active elements in a pool.
ip_lookup_main_t lookup_main
static vlib_cli_command_t set_unnumbered_command
(constructor) VLIB_CLI_COMMAND (set_unnumbered_command)
__clib_export clib_error_t * pcap_write(pcap_main_t *pm)
Write PCAP file.
#define vec_sort_with_function(vec, f)
Sort a vector using the supplied element comparison function.
word vnet_hw_interface_compare(vnet_main_t *vnm, uword hw_if_index0, uword hw_if_index1)
static uword clib_bitmap_last_set(uword *ai)
Return the higest numbered set bit in a bitmap.
u32 n_packets_to_capture
Number of packets to capture.
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
clib_error_t * vnet_hw_interface_add_del_mac_address(vnet_main_t *vnm, u32 hw_if_index, const u8 *mac_address, u8 is_add)
A collection of simple counters.
static vlib_main_t * vlib_get_main(void)
format_function_t format_vnet_sw_interface
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
#define ETHERNET_INTERFACE_FLAG_ACCEPT_ALL
uword * sw_if_index_by_sup_and_sub
#define PCAP_MAIN_INIT_DONE
char * file_name
File name of pcap output.
#define clib_warning(format, args...)
static clib_error_t * parse_vlan_sub_interfaces(unformat_input_t *input, vnet_sw_interface_t *template)
Parse subinterface names.
void vnet_hw_if_set_rx_queue_thread_index(vnet_main_t *vnm, u32 queue_index, u32 thread_index)
static vlib_cli_command_t show_interface_sec_mac_addr
(constructor) VLIB_CLI_COMMAND (show_interface_sec_mac_addr)
#define clib_bitmap_foreach(i, ai)
Macro to iterate across set bits in a bitmap.
static vnet_sw_interface_t * vnet_get_sup_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
static clib_error_t * pcap_trace_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
vl_api_interface_index_t sw_if_index
ethernet_interface_address_t * secondary_addrs
static clib_error_t * create_sub_interfaces(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
void vlib_clear_simple_counters(vlib_simple_counter_main_t *cm)
Clear a collection of simple counters.
static vlib_cli_command_t renumber_interface_command
(constructor) VLIB_CLI_COMMAND (renumber_interface_command)
u32 n_packets_captured
Number of packets currently captured.
vlib_error_t pcap_error_index
static vlib_cli_command_t vnet_cli_interface_command
(constructor) VLIB_CLI_COMMAND (vnet_cli_interface_command)
static void * clib_mem_alloc(uword size)
__clib_export uword unformat_bitmap_list(unformat_input_t *input, va_list *va)
unformat a list of bit ranges into a bitmap (eg "0-3,5-7,11" )
vl_api_fib_path_type_t type
static clib_error_t * set_state(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
vnet_interface_main_t interface_main
int vnet_pcap_dispatch_trace_configure(vnet_pcap_dispatch_trace_args_t *a)
static vlib_cli_command_t set_state_command
(constructor) VLIB_CLI_COMMAND (set_state_command)
void vnet_sw_interface_set_protocol_mtu(vnet_main_t *vnm, u32 sw_if_index, u32 mtu[])
@ VNET_HW_IF_RX_MODE_UNKNOWN
static vlib_cli_command_t clear_hw_interface_counters_command
(constructor) VLIB_CLI_COMMAND (clear_hw_interface_counters_command)
static clib_error_t * show_hw_interfaces(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
vl_api_wireguard_peer_flags_t flags