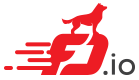 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
51 #define TEIB_NOTIFY(_te, _fn) { \
53 vec_foreach(_vft, teib_vfts) { \
60 #define TEIB_DBG(...) \
61 vlib_log_debug (teib_logger, __VA_ARGS__);
63 #define TEIB_INFO(...) \
64 vlib_log_notice (teib_logger, __VA_ARGS__);
66 #define TEIB_TE_DBG(_te, _fmt, _args...) \
67 vlib_log_debug (teib_logger, "[%U]: " _fmt, format_teib_entry, _te - teib_pool, ##_args)
68 #define TEIB_TE_INFO(_te, _fmt, _args...) \
69 vlib_log_notice (teib_logger, "[%U]: " _fmt, format_teib_entry, _te - teib_pool, ##_args)
216 return (VNET_API_ERROR_NO_SUCH_FIB);
252 return (VNET_API_ERROR_ENTRY_ALREADY_EXISTS);
288 return (VNET_API_ERROR_NO_SUCH_ENTRY);
302 s =
format (s,
"[%d] ", tei);
307 s =
format (s,
" via [%d]:%U",
387 .old_fib_index = old_fib_index,
388 .new_fib_index = new_fib_index,
400 .old_fib_index = old_fib_index,
401 .new_fib_index = new_fib_index,
static uword ip6_address_is_link_local_unicast(const ip6_address_t *a)
fib_node_index_t fib_table_entry_path_add(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, dpo_proto_t next_hop_proto, const ip46_address_t *next_hop, u32 next_hop_sw_if_index, u32 next_hop_fib_index, u32 next_hop_weight, fib_mpls_label_t *next_hop_labels, fib_route_path_flags_t path_flags)
Add one path to an entry (aka route) in the FIB.
vnet_interface_main_t * im
teib_entry_t * teib_entry_find_46(u32 sw_if_index, fib_protocol_t fproto, const ip46_address_t *peer)
static walk_rc_t teib_walk_table_bind(index_t tei, void *arg)
#define clib_memcpy(d, s, n)
u32 fib_table_get_table_id(u32 fib_index, fib_protocol_t proto)
Get the Table-ID of the FIB from protocol and index.
ip4_table_bind_callback_t * table_bind_callbacks
Functions to call when interface to table biding changes.
ip4_main_t ip4_main
Global ip4 main structure.
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
vlib_log_class_t vlib_log_register_class(char *class, char *subclass)
static void clib_mem_free(void *p)
@ FIB_ENTRY_FLAG_ATTACHED
void adj_midchain_delegate_stack(adj_index_t ai, u32 fib_index, const fib_prefix_t *pfx)
create/attach a midchain delegate and stack it on the prefix passed
#define hash_set_mem(h, key, value)
static void teib_walk_itf_proto(u32 sw_if_index, ip_address_family_t af, teib_walk_cb_t fn, void *ctx)
void ip_address_to_fib_prefix(const ip_address_t *addr, fib_prefix_t *prefix)
convert from a IP address to a FIB prefix
#define pool_put(P, E)
Free an object E in pool P.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
void ip6_ll_table_entry_delete(const ip6_ll_prefix_t *ilp)
Delete a IP6 link-local entry.
fib_node_index_t ip6_ll_table_entry_update(const ip6_ll_prefix_t *ilp, fib_route_path_flags_t flags)
Update an entry in the table.
int teib_entry_add(u32 sw_if_index, const ip_address_t *peer, u32 nh_table_id, const ip_address_t *nh)
Create a new TEIB entry.
const fib_prefix_t * teib_entry_get_nh(const teib_entry_t *te)
void ip_address_from_46(const ip46_address_t *nh, fib_protocol_t fproto, ip_address_t *ip)
ip6_table_bind_function_t * function
teib_entry_t * teib_entry_get(index_t tei)
@ FIB_SOURCE_ADJ
Adjacency source.
#define ip_addr_version(_a)
#define hash_create_mem(elts, key_bytes, value_bytes)
struct teib_key_t_ teib_key_t
static ip_address_family_t teib_entry_get_af(const teib_entry_t *te)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
void fib_table_entry_path_remove(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, dpo_proto_t next_hop_proto, const ip46_address_t *next_hop, u32 next_hop_sw_if_index, u32 next_hop_fib_index, u32 next_hop_weight, fib_route_path_flags_t path_flags)
remove one path to an entry (aka route) in the FIB.
#define hash_unset_mem(h, key)
static void teib_adj_fib_add(const ip_address_t *ip, u32 sw_if_index, u32 fib_index)
struct teib_table_bind_ctx_t_ teib_table_bind_ctx_t
vnet_main_t * vnet_get_main(void)
static void teib_adj_fib_remove(ip_address_t *ip, u32 sw_if_index, u32 fib_index)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
#define TEIB_TE_INFO(_te, _fmt, _args...)
u8 * format_ip_address(u8 *s, va_list *args)
ip6_table_bind_callback_t * table_bind_callbacks
Functions to call when interface to table biding changes.
u8 * format_teib_entry(u8 *s, va_list *args)
enum fib_protocol_t_ fib_protocol_t
Protocol Type.
Aggregate type for a prefix in the IPv6 Link-local table.
u32 teib_entry_get_fib_index(const teib_entry_t *te)
dpo_proto_t fib_proto_to_dpo(fib_protocol_t fib_proto)
ip46_address_t fp_addr
The address type is not deriveable from the fp_addr member.
fib_protocol_t ip_address_family_to_fib_proto(ip_address_family_t af)
walk_rc_t(* teib_walk_cb_t)(index_t nei, void *ctx)
#define hash_get_mem(h, key)
void teib_walk_itf(u32 sw_if_index, teib_walk_cb_t fn, void *ctx)
void teib_register(const teib_vft_t *vft)
static vlib_log_class_t teib_logger
#define pool_foreach_index(i, v)
format_function_t format_vnet_sw_if_index_name
description fragment has unexpected format
u32 fib_table_get_index_for_sw_if_index(fib_protocol_t proto, u32 sw_if_index)
Get the index of the FIB bound to the interface.
@ FIB_ROUTE_PATH_FLAG_NONE
void fib_table_lock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Release a reference counting lock on the table.
u32 teib_entry_get_sw_if_index(const teib_entry_t *te)
accessors for the opaque struct
#define VLIB_INIT_FUNCTION(x)
vl_api_address_family_t af
u8 * format_fib_prefix(u8 *s, va_list *args)
const ip_address_t * teib_entry_get_peer(const teib_entry_t *te)
ip4_table_bind_function_t * function
fib_protocol_t fp_proto
protocol type
u32 adj_index_t
An index for adjacencies.
int teib_entry_del(u32 sw_if_index, const ip_address_t *peer)
static teib_entry_t * teib_pool
#define pool_get_zero(P, E)
Allocate an object E from a pool P and zero it.
void teib_walk(teib_walk_cb_t fn, void *ctx)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
teib_entry_t * teib_entry_find(u32 sw_if_index, const ip_address_t *peer)
struct teib_db_t_ teib_db_t
void fib_table_unlock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Take a reference counting lock on the table.
void teib_entry_adj_stack(const teib_entry_t *te, adj_index_t ai)
static void teib_table_bind_v6(ip6_main_t *im, uword opaque, u32 sw_if_index, u32 new_fib_index, u32 old_fib_index)
ip6_address_t ilp_addr
the IP6 address
static void teib_table_bind_v4(ip4_main_t *im, uword opaque, u32 sw_if_index, u32 new_fib_index, u32 old_fib_index)
static clib_error_t * teib_init(vlib_main_t *vm)
u32 fib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
#define TEIB_NOTIFY(_te, _fn)
vl_api_interface_index_t sw_if_index
STATIC_ASSERT_SIZEOF(teib_key_t, 24)
enum walk_rc_t_ walk_rc_t
Walk return code.
static void * clib_mem_alloc(uword size)
Aggregate type for a prefix.
static teib_vft_t * teib_vfts
enum ip_address_family_t_ ip_address_family_t