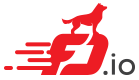 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
28 #define foreach_ipsec_input_error \
29 _(RX_PKTS, "IPSec pkts received") \
30 _(RX_POLICY_MATCH, "IPSec policy match") \
31 _(RX_POLICY_NO_MATCH, "IPSec policy not matched") \
32 _(RX_POLICY_BYPASS, "IPSec policy bypass") \
33 _(RX_POLICY_DISCARD, "IPSec policy discard")
37 #define _(sym,str) IPSEC_INPUT_ERROR_##sym,
44 #define _(sym,string) string,
67 s =
format (s,
"%U: sa_id %u spd %u policy %d spi %u (0x%08x) seq %u",
86 if (da < clib_net_to_host_u32 (p->
laddr.
start.ip4.as_u32))
89 if (da > clib_net_to_host_u32 (p->
laddr.
stop.ip4.as_u32))
92 if (sa < clib_net_to_host_u32 (p->
raddr.
start.ip4.as_u32))
95 if (sa > clib_net_to_host_u32 (p->
raddr.
stop.ip4.as_u32))
119 if (ipsec_sa_is_set_IS_TUNNEL (s))
121 if (da != clib_net_to_host_u32 (s->
tunnel.
t_dst.
ip.ip4.as_u32))
124 if (sa != clib_net_to_host_u32 (s->
tunnel.
t_src.
ip.ip4.as_u32))
130 if (da < clib_net_to_host_u32 (p->
laddr.
start.ip4.as_u32))
133 if (da > clib_net_to_host_u32 (p->
laddr.
stop.ip4.as_u32))
136 if (sa < clib_net_to_host_u32 (p->
raddr.
start.ip4.as_u32))
139 if (sa > clib_net_to_host_u32 (p->
raddr.
stop.ip4.as_u32))
151 if ((memcmp (
a->as_u64, la->as_u64, 2 * sizeof (
u64)) >= 0) &&
152 (memcmp (
a->as_u64, ua->as_u64, 2 * sizeof (
u64)) <= 0))
160 ip6_address_t * da,
u32 spi)
175 if (ipsec_sa_is_set_IS_TUNNEL (s))
205 u64 ipsec_unprocessed = 0, ipsec_matched = 0;
206 u64 ipsec_dropped = 0, ipsec_bypassed = 0;
234 b[0]->
flags |= VNET_BUFFER_F_IS_IP4;
235 b[0]->
flags &= ~VNET_BUFFER_F_IS_IP6;
244 (ip0->
protocol == IP_PROTOCOL_IPSEC_ESP
245 || ip0->
protocol == IP_PROTOCOL_UDP))
272 pi0 = p0 -
im->policies;
275 thread_index, pi0, 1, clib_net_to_host_u16 (ip0->length));
278 next[0] =
im->esp4_decrypt_next_index;
290 (ip0->src_address.as_u32),
292 (ip0->dst_address.as_u32),
293 IPSEC_SPD_POLICY_IP4_INBOUND_BYPASS);
298 pi0 = p0 -
im->policies;
301 clib_net_to_host_u16 (ip0->length));
313 (ip0->src_address.as_u32),
315 (ip0->dst_address.as_u32),
316 IPSEC_SPD_POLICY_IP4_INBOUND_DISCARD);
321 pi0 = p0 -
im->policies;
324 clib_net_to_host_u16 (ip0->length));
326 next[0] = IPSEC_INPUT_NEXT_DROP;
341 tr->
proto = ip0->protocol;
343 tr->
spi = has_space0 ? clib_net_to_host_u32 (esp0->
spi) : ~0;
344 tr->
seq = has_space0 ? clib_net_to_host_u32 (esp0->
seq) : ~0;
349 else if (ip0->
protocol == IP_PROTOCOL_IPSEC_AH)
369 pi0 = p0 -
im->policies;
372 thread_index, pi0, 1, clib_net_to_host_u16 (ip0->length));
375 next[0] =
im->ah4_decrypt_next_index;
386 (ip0->src_address.as_u32),
388 (ip0->dst_address.as_u32),
389 IPSEC_SPD_POLICY_IP4_INBOUND_BYPASS);
394 pi0 = p0 -
im->policies;
397 clib_net_to_host_u16 (ip0->length));
409 (ip0->src_address.as_u32),
411 (ip0->dst_address.as_u32),
412 IPSEC_SPD_POLICY_IP4_INBOUND_DISCARD);
417 pi0 = p0 -
im->policies;
420 clib_net_to_host_u16 (ip0->length));
422 next[0] = IPSEC_INPUT_NEXT_DROP;
437 tr->
proto = ip0->protocol;
439 tr->
spi = has_space0 ? clib_net_to_host_u32 (ah0->
spi) : ~0;
440 tr->
seq = has_space0 ? clib_net_to_host_u32 (ah0->
seq_no) : ~0;
447 ipsec_unprocessed += 1;
457 IPSEC_INPUT_ERROR_RX_PKTS,
frame->n_vectors);
460 IPSEC_INPUT_ERROR_RX_POLICY_MATCH,
464 IPSEC_INPUT_ERROR_RX_POLICY_NO_MATCH,
468 IPSEC_INPUT_ERROR_RX_POLICY_DISCARD,
472 IPSEC_INPUT_ERROR_RX_POLICY_BYPASS,
475 return frame->n_vectors;
481 .name =
"ipsec4-input-feature",
482 .vector_size =
sizeof (
u32),
489 #define _(s,n) [IPSEC_INPUT_NEXT_##s] = n,
505 u32 ipsec_unprocessed = 0;
506 u32 ipsec_matched = 0;
530 u32 header_size =
sizeof (ip0[0]);
532 bi0 = to_next[0] =
from[0];
539 b0->
flags |= VNET_BUFFER_F_IS_IP6;
540 b0->
flags &= ~VNET_BUFFER_F_IS_IP4;
553 (
"packet received from %U to %U spi %u size %u spd_id %u",
569 pi0 = p0 -
im->policies;
577 next0 =
im->esp6_decrypt_next_index;
586 else if (ip0->
protocol == IP_PROTOCOL_IPSEC_AH)
597 pi0 = p0 -
im->policies;
605 next0 =
im->ah6_decrypt_next_index;
615 ipsec_unprocessed += 1;
628 tr->
spi = clib_net_to_host_u32 (esp0->
spi);
629 tr->
seq = clib_net_to_host_u32 (esp0->
seq);
634 n_left_to_next, bi0, next0);
640 IPSEC_INPUT_ERROR_RX_PKTS,
644 IPSEC_INPUT_ERROR_RX_POLICY_MATCH,
653 .name =
"ipsec6-input-feature",
654 .vector_size =
sizeof (
u32),
661 #define _(s,n) [IPSEC_INPUT_NEXT_##s] = n,
static uword ip6_address_is_equal(const ip6_address_t *a, const ip6_address_t *b)
vnet_interface_main_t * im
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
nat44_ei_hairpin_src_next_t next_index
ip46_address_range_t raddr
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
vlib_get_buffers(vm, from, b, n_left_from)
@ VLIB_NODE_TYPE_INTERNAL
vlib_main_t vlib_node_runtime_t * node
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
vlib_main_t vlib_node_runtime_t vlib_frame_t * from_frame
vlib_buffer_enqueue_to_next(vm, node, from,(u16 *) nexts, frame->n_vectors)
static_always_inline void * vnet_feature_next_with_data(u32 *next0, vlib_buffer_t *b0, u32 n_data_bytes)
u32 id
the User's ID for this policy
static u8 vlib_buffer_has_space(vlib_buffer_t *b, word l)
Check if there is enough space in buffer to advance.
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
#define VLIB_NODE_FN(node)
static ipsec_sa_t * ipsec_sa_get(u32 sa_index)
#define VLIB_NODE_FLAG_TRACE
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
#define foreach_ipsec_input_next
struct _vlib_node_registration vlib_node_registration_t
#define vlib_validate_buffer_enqueue_x1(vm, node, next_index, to_next, n_left_to_next, bi0, next0)
Finish enqueueing one buffer forward in the graph.
description fragment has unexpected format
vlib_combined_counter_main_t ipsec_spd_policy_counters
Policy packet & bytes counters.
vlib_put_next_frame(vm, node, next_index, 0)
#define vec_foreach(var, vec)
Vector iterator.
A Secruity Policy Database.
enum ip_protocol ip_protocol_t
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
ip46_address_range_t laddr
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
#define clib_warning(format, args...)
u32 * policies[IPSEC_SPD_POLICY_N_TYPES]
vectors for each of the policy types
u16 nexts[VLIB_FRAME_SIZE]
static int ip4_header_bytes(const ip4_header_t *i)
enum ipsec_spd_policy_t_ ipsec_spd_policy_type_t
#define vlib_get_next_frame(vm, node, next_index, vectors, n_vectors_left)
Get pointer to next frame vector data by (vlib_node_runtime_t, next_index).
vl_api_fib_path_type_t type
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
#define vlib_prefetch_buffer_data(b, type)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)
vl_api_wireguard_peer_flags_t flags