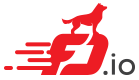 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
38 u64 fa = clib_net_to_host_u64 (a1->as_u64[0]) ^
39 clib_net_to_host_u64 (a2->as_u64[0]);
42 u64 la = clib_net_to_host_u64 (a1->as_u64[1]) ^
43 clib_net_to_host_u64 (a2->as_u64[1]);
46 return 64 + __builtin_clzll (la);
50 return __builtin_clzll (fa);
58 clib_net_to_host_u32 (a1->
as_u32) ^ clib_net_to_host_u32 (a2->
as_u32);
61 return __builtin_clz (
a);
77 ip6_address_t *
tmp, *bestsrc = 0;
81 dst->as_u32[0] == clib_host_to_net_u32 (0xff020000))
93 if (l > bestlen || bestsrc == 0)
126 if (l > bestlen || bestsrc == 0)
163 if (fib_index == (
u32) ~0)
171 if (output_sw_if_index == ~0)
173 if_index = output_sw_if_index;
201 if (fib_index == (
u32) ~0)
209 if (output_sw_if_index == ~0)
211 if_index = output_sw_if_index;
@ IP_INTERFACE_ADDRESS_FLAG_STALE
static uword ip6_address_is_link_local_unicast(const ip6_address_t *a)
static void * ip_interface_address_get_address(ip_lookup_main_t *lm, ip_interface_address_t *a)
#define foreach_ip_interface_address(lm, a, sw_if_index, loop, body)
ip_lookup_main_t lookup_main
#define clib_memcpy(d, s, n)
ip4_main_t ip4_main
Global ip4 main structure.
#define FIB_NODE_INDEX_INVALID
fib_node_index_t fib_table_lookup(u32 fib_index, const fib_prefix_t *prefix)
Perfom a longest prefix match in the non-forwarding table.
bool ip4_sas(u32 table_id, u32 sw_if_index, const ip4_address_t *dst, ip4_address_t *src)
bool ip6_sas(u32 table_id, u32 sw_if_index, const ip6_address_t *dst, ip6_address_t *src)
bool ip4_sas_by_sw_if_index(u32 sw_if_index, const ip4_address_t *dst, ip4_address_t *src)
const ip6_address_t * ip6_get_link_local_address(u32 sw_if_index)
u32 fib_node_index_t
A typedef of a node index.
static int ip4_sas_commonlen(const ip4_address_t *a1, const ip4_address_t *a2)
static void ip6_address_copy(ip6_address_t *dst, const ip6_address_t *src)
u32 fib_entry_get_resolving_interface(fib_node_index_t entry_index)
ip_lookup_main_t lookup_main
IP prefix management on interfaces.
bool ip6_sas_by_sw_if_index(u32 sw_if_index, const ip6_address_t *dst, ip6_address_t *src)
u32 fib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
vl_api_interface_index_t sw_if_index
ip_interface_address_flags_t flags
Aggregate type for a prefix.
static int ip6_sas_commonlen(const ip6_address_t *a1, const ip6_address_t *a2)