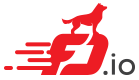 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
62 iproto =
ip4->protocol;
70 iproto =
ip6->protocol;
79 if (!session_not_found)
190 .name =
"cnat-input-ip4",
191 .vector_size =
sizeof (
u32),
196 .sibling_of =
"ip4-lookup",
200 .arc_name =
"ip4-unicast",
201 .node_name =
"cnat-input-ip4",
216 .name =
"cnat-input-ip6",
217 .vector_size =
sizeof (
u32),
222 .sibling_of =
"ip6-lookup",
226 .arc_name =
"ip6-unicast",
227 .node_name =
"cnat-input-ip6",
257 iproto =
ip4->protocol;
263 iproto =
ip6->protocol;
271 if (!session_not_found)
309 CNAT_ERROR_EXHAUSTED_PORTS, 1);
315 if (iproto == IP_PROTOCOL_TCP || iproto == IP_PROTOCOL_UDP)
353 .name =
"cnat-output-ip4",
354 .vector_size =
sizeof (
u32),
366 .arc_name =
"ip4-output",
367 .node_name =
"cnat-output-ip4",
383 .name =
"cnat-output-ip6",
384 .vector_size =
sizeof (
u32),
396 .arc_name =
"ip6-output",
397 .node_name =
"cnat-output-ip6",
static_always_inline cnat_client_t * cnat_client_ip6_find(const ip6_address_t *ip)
Find a client from an IP6 address.
u16 dpoi_next_node
The next VLIB node to follow.
static vlib_cli_command_t trace
(constructor) VLIB_CLI_COMMAND (trace)
u16 lb_n_buckets
number of buckets in the load-balance.
static_always_inline void cnat_add_trace(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_buffer_t *b, cnat_session_t *session, const cnat_translation_t *ct, u8 flags)
index_t dpoi_index
the index of objects of that type
static_always_inline cnat_ep_trk_t * cnat_load_balance(const cnat_translation_t *ct, ip_address_family_t af, ip4_header_t *ip4, ip6_header_t *ip6, u32 *dpoi_index)
ip46_address_t cs_ip[VLIB_N_DIR]
IP 4/6 address in the rx/tx direction.
vlib_node_registration_t cnat_output_feature_ip6_node
(constructor) VLIB_REGISTER_NODE (cnat_output_feature_ip6_node)
return cnat_node_inline(vm, node, frame, cnat_input_feature_fn, AF_IP4, CNAT_LOCATION_INPUT, 0)
cnat_endpoint_t ct_ep[VLIB_N_DIR]
The EP being tracked.
static void ip46_address_set_ip4(ip46_address_t *ip46, const ip4_address_t *ip)
index_t cs_lbi
The load balance object to use to forward.
static_always_inline u32 cnat_client_cnt_session(cnat_client_t *cc)
Add a session refcnt to this client.
@ CNAT_SESSION_FLAG_NO_CLIENT
This session doesn't have a client, do not attempt to free it.
@ VLIB_NODE_TYPE_INTERNAL
static_always_inline void ip46_address_set_ip6(ip46_address_t *dst, const ip6_address_t *src)
char * cnat_error_strings[]
static_always_inline cnat_client_t * cnat_client_ip4_find(const ip4_address_t *ip)
Find a client from an IP4 address.
static_always_inline void cnat_session_create(cnat_session_t *session, cnat_node_ctx_t *ctx, cnat_session_location_t rsession_location, u8 rsession_flags)
Create NAT sessions rsession_location is the location the (return) session will be matched at.
u32 dpoi_next_node
Persist translation->ct_lb.dpoi_next_node.
A session represents the memory of a translation.
VNET_FEATURE_INIT(cnat_in_ip4_feature, static)
struct cnat_session_t_::@645 key
this key sits in the same memory location a 'key' in the bihash kvp
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
dpo_id_t cc_parent
How to send packets to this client post translation.
@ CNAT_TRACE_SESSION_FOUND
#define VLIB_NODE_FN(node)
u8 ct_flags
Allows to disable if not resolved yet.
index_t parent_cci
Parent cnat_client index if cloned via interpose or own index if vanilla client.
#define VLIB_NODE_FLAG_TRACE
static u8 * format_cnat_trace(u8 *s, va_list *args)
static_always_inline void vnet_feature_next(u32 *next0, vlib_buffer_t *b0)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
vlib_node_registration_t cnat_input_feature_ip6_node
(constructor) VLIB_REGISTER_NODE (cnat_input_feature_ip6_node)
vlib_main_t vlib_node_runtime_t * node
static uword cnat_output_feature_fn(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_buffer_t *b, cnat_node_ctx_t *ctx, int session_not_found, cnat_session_t *session)
static_always_inline void cnat_translation_ip6(const cnat_session_t *session, ip6_header_t *ip6, udp_header_t *udp)
static void cnat_timestamp_update(u32 index, f64 t)
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
static const dpo_id_t * load_balance_get_bucket_i(const load_balance_t *lb, u32 bucket)
static_always_inline void cnat_translation_ip4(const cnat_session_t *session, ip4_header_t *ip4, udp_header_t *udp)
int cnat_allocate_port(u16 *port, ip_protocol_t iproto)
struct _vlib_node_registration vlib_node_registration_t
static_always_inline void ip46_address_copy(ip46_address_t *dst, const ip46_address_t *src)
u32 cs_ts_index
Timestamp index this session was last used.
ip_protocol_t cs_proto
The IP protocol TCP or UDP only supported.
bool ip_address_is_zero(const ip_address_t *ip)
vlib_node_registration_t cnat_input_feature_ip4_node
(constructor) VLIB_REGISTER_NODE (cnat_input_feature_ip4_node)
A collection of combined counters.
@ CNAT_SESSION_FLAG_NO_NAT
@ CNAT_SESSION_FLAG_ALLOC_PORT
This session source port was allocated, free it on cleanup.
cnat_snat_policy_t snat_policy
static load_balance_t * load_balance_get(index_t lbi)
enum ip_protocol ip_protocol_t
static uword cnat_input_feature_fn(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_buffer_t *b, cnat_node_ctx_t *ctx, int session_not_found, cnat_session_t *session)
vlib_combined_counter_main_t cnat_translation_counters
Counters for each translation.
#define VNET_FEATURES(...)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
dpo_id_t ct_lb
The LB used to forward to the backends.
cnat_translation_t * cnat_translation_pool
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
struct cnat_session_t_::@646 value
this value sits in the same memory location a 'value' in the bihash kvp
cnat_snat_policy_main_t cnat_snat_policy_main
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
enum cnat_feature_next_ cnat_feature_next_t
A client is a representation of an IP address behind the NAT.
vlib_node_registration_t cnat_output_feature_ip4_node
(constructor) VLIB_REGISTER_NODE (cnat_output_feature_ip4_node)
dpo_id_t ct_dpo
The forwarding contributed by the entry.
vl_api_fib_path_type_t type
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
@ CNAT_TRACE_SESSION_CREATED
A Translation represents the translation of a VEP to one of a set of real server addresses.
Data used to track an EP in the FIB.
VLIB buffer representation.
u16 cs_port[VLIB_N_DIR]
ports in rx/tx
static_always_inline cnat_translation_t * cnat_find_translation(index_t cti, u16 port, ip_protocol_t proto)
#define VLIB_REGISTER_NODE(x,...)