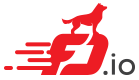 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
23 #include <sys/types.h>
26 #include <netinet/in.h>
57 #undef __included_bihash_template_h__
63 #include <vnet/classify/classify.api_enum.h>
64 #include <vnet/ip/ip.api_enum.h>
73 #define vl_print(handle, ...) vlib_cli_output (handle, __VA_ARGS__)
79 #define foreach_vpe_api_msg \
80 _(CONTROL_PING, control_ping) \
82 _(CLI_INBAND, cli_inband) \
83 _(GET_NODE_INDEX, get_node_index) \
84 _(ADD_NODE_NEXT, add_node_next) \
85 _(SHOW_VERSION, show_version) \
86 _(SHOW_THREADS, show_threads) \
87 _(GET_NODE_GRAPH, get_node_graph) \
88 _(GET_NEXT_INDEX, get_next_index) \
89 _(LOG_DUMP, log_dump) \
90 _(SHOW_VPE_SYSTEM_TIME, show_vpe_system_time) \
91 _(GET_F64_ENDIAN_VALUE, get_f64_endian_value) \
92 _(GET_F64_INCREMENT_BY_ONE, get_f64_increment_by_one) \
95 #define QUOTE(x) QUOTE_(x)
114 p = hash_get (vam->a##_registration_hash, client_index); \
116 rp = pool_elt_at_index (vam->a##_registrations, p[0]); \
117 pool_put (vam->a##_registrations, rp); \
118 hash_unset (vam->a##_registration_hash, client_index); \
136 rmp->
vpe_pid = ntohl (getpid());
144 u8 **shmem_vecp = (
u8 **) arg;
149 shmem_vec = *shmem_vecp;
161 *shmem_vecp = shmem_vec;
180 rp->_vl_msg_id =
ntohs (VL_API_CLI_REPLY);
199 u8 **mem_vecp = (
u8 **) arg;
200 u8 *mem_vec = *mem_vecp;
270 td->id = htonl (
index);
272 strncpy ((
char *) td->name, (
char *) w->
name,
ARRAY_LEN (td->name) - 1);
276 td->pid = htonl (w->
lwp);
277 td->cpu_id = htonl (w->
cpu_id);
279 td->cpu_socket = htonl (w->
numa_id);
287 #if !defined(__powerpc64__)
290 vl_api_thread_data_t *td;
303 rmp->_vl_msg_id = htons (VL_API_SHOW_THREADS_REPLY);
318 clib_warning (
"power pc does not support show threads api");
322 rmp->count = htonl(
count);
340 rv = VNET_API_ERROR_NO_SUCH_NODE;
366 rv = VNET_API_ERROR_NO_SUCH_NODE;
374 rv = VNET_API_ERROR_NO_SUCH_NODE2;
378 next_node_index = next_node->
index;
380 p =
hash_get (
node->next_slot_by_node, next_node_index);
384 rv = VNET_API_ERROR_NO_SUCH_ENTRY;
412 rv = VNET_API_ERROR_NO_SUCH_NODE;
419 rv = VNET_API_ERROR_NO_SUCH_NODE2;
454 &node_dups, &stat_vms);
471 vl_api_log_level_t * level,
u8 * msg_class,
u8 * message)
480 msg_size =
sizeof (*rmp) + class_len + message_len;
484 rmp->_vl_msg_id =
ntohs (VL_API_LOG_DETAILS);
488 rmp->
level = htonl (*level);
490 memcpy (rmp->
msg_class, msg_class, class_len - 1);
491 memcpy (rmp->
message, message, message_len - 1);
508 f64 time_offset, start_time;
523 if (start_time <= e->
timestamp + time_offset)
525 (vl_api_log_level_t *) & e->
level,
553 rv = VNET_API_ERROR_API_ENDIAN_FAILED;
578 #define BOUNCE_HANDLER(nn) \
579 static void vl_api_##nn##_t_handler ( \
580 vl_api_##nn##_t *mp) \
582 vpe_client_registration_t *reg; \
583 vpe_api_main_t * vam = &vpe_api_main; \
587 pool_foreach (reg, vam->nn##_registrations) \
589 q = vl_api_client_index_to_input_queue (reg->client_index); \
596 if (q->cursize == q->maxsize) { \
597 clib_warning ("ERROR: receiver queue full, drop msg"); \
598 vl_msg_api_free (mp); \
601 vl_msg_api_send_shmem (q, (u8 *)&mp); \
605 vl_msg_api_free (mp); \
623 vl_msg_api_set_handlers(VL_API_##N, #n, \
624 vl_api_##n##_t_handler, \
626 vl_api_##n##_t_endian, \
627 vl_api_##n##_t_print, \
628 sizeof(vl_api_##n##_t), 1);
635 am->api_trace_cfg[VL_API_CLASSIFY_ADD_DEL_TABLE].size += 5 *
sizeof (
u32x4);
636 am->api_trace_cfg[VL_API_CLASSIFY_ADD_DEL_SESSION].size +=
642 am->is_mp_safe[VL_API_CONTROL_PING] = 1;
643 am->is_mp_safe[VL_API_CONTROL_PING_REPLY] = 1;
644 am->is_mp_safe[VL_API_IP_ROUTE_ADD_DEL] = 1;
645 am->is_mp_safe[VL_API_GET_NODE_GRAPH] = 1;
665 am->a##_registration_hash = hash_create (0, sizeof (uword));
679 u64 baseva,
size, pvt_heap_size;
681 const int max_buf_size = 4096;
683 struct passwd _pw, *pw;
684 struct group _grp, *grp;
689 if (
unformat (input,
"prefix %s", &chroot_path))
694 else if (
unformat (input,
"uid %d", &uid))
696 else if (
unformat (input,
"gid %d", &gid))
698 else if (
unformat (input,
"baseva %llx", &baseva))
706 else if (
unformat (input,
"global-pvt-heap-size %lldM", &pvt_heap_size))
708 else if (
unformat (input,
"global-pvt-heap-size size %lld",
711 else if (
unformat (input,
"api-pvt-heap-size %lldM", &pvt_heap_size))
713 else if (
unformat (input,
"api-pvt-heap-size size %lld",
722 else if (
unformat (input,
"uid %s", &s))
737 "cannot fetch username %s", s);
753 else if (
unformat (input,
"gid %s", &s))
769 "cannot fetch group %s", s);
800 #define vl_msg_name_crc_list
802 #undef vl_msg_name_crc_list
807 #define _(id,n,crc) vl_msg_api_add_msg_name_crc (am, #n "_" #crc, id);
808 foreach_vl_msg_name_crc_memclnt;
809 foreach_vl_msg_name_crc_vpe;
812 #define vl_api_version_tuple(n,mj, mi, p) \
813 vl_msg_api_add_version (am, #n, mj, mi, p);
815 #undef vl_api_version_tuple
static void vl_api_control_ping_t_handler(vl_api_control_ping_t *mp)
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
static void vl_api_cli_t_handler(vl_api_cli_t *mp)
void vlib_node_get_nodes(vlib_main_t *vm, u32 max_threads, int include_stats, int barrier_sync, vlib_node_t ****node_dupsp, vlib_main_t ***stat_vmsp)
Get list of nodes.
vpe_api_main_t vpe_api_main
show_threads display the information about vpp threads running on system along with their process id,...
#define foreach_registration_hash
static vl_api_registration_t * vl_api_client_index_to_registration(u32 index)
vlib_log_entry_t * entries
char * vpe_api_get_build_date(void)
return the build date
static f64 unix_time_now(void)
f64 f64_value[default=1.0]
#define REPLY_MACRO2(t, body)
static uword vlib_node_add_next(vlib_main_t *vm, uword node, uword next_node)
#define vec_new(T, N)
Create new vector of given type and length (unspecified alignment, no header).
Reply for show vpe system time.
#define clib_memcpy(d, s, n)
nat44_ei_hairpin_src_next_t next_index
vl_api_timestamp_t start_timestamp
static void vl_api_send_msg(vl_api_registration_t *rp, u8 *elem)
Show the current system timestamp.
static clib_error_t * vpe_api_hookup(vlib_main_t *vm)
static f64 clib_host_to_net_f64(f64 x)
vlib_main_t vlib_node_runtime_t * node
static void vl_api_get_node_graph_t_handler(vl_api_get_node_graph_t *mp)
#define clib_error_return(e, args...)
static clib_error_t * api_segment_config(vlib_main_t *vm, unformat_input_t *input)
static clib_error_t * memclnt_delete_callback(u32 client_index)
#define foreach_vpe_api_msg
Query relative index via node names.
void vl_set_memory_uid(int uid)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static void shmem_cli_output(uword arg, u8 *buffer, uword buffer_bytes)
vlib_worker_thread_t * vlib_worker_threads
@ RESOLVE_IP4_ADD_DEL_ROUTE
#define VLIB_EARLY_CONFIG_FUNCTION(x, n,...)
char * vpe_api_get_version(void)
Return the image version string.
Set the next node for a given node request.
void vl_set_global_pvt_heap_size(u64 size)
void vl_set_api_pvt_heap_size(u64 size)
char * vpe_api_get_build_directory(void)
Return the image build directory name.
Control ping from the client to the server response.
static void vl_api_get_next_index_t_handler(vl_api_get_next_index_t *mp)
#define clib_error_return_code(e, code, flags, args...)
void vl_set_memory_region_name(const char *name)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
u32 vl_msg_api_get_msg_length(void *msg_arg)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
vnet_main_t * vnet_get_main(void)
Get node index using name request.
struct clib_bihash_value offset
template key/value backing page structure
static void vl_api_get_f64_increment_by_one_t_handler(vl_api_get_f64_increment_by_one_t *mp)
An API client registration, only in vpp/vlib.
static void vl_api_show_version_t_handler(vl_api_show_version_t *mp)
f64 types are not standardized across the wire.
@ RESOLVE_IP6_ADD_DEL_ROUTE
int vlib_cli_input(vlib_main_t *vm, unformat_input_t *input, vlib_cli_output_function_t *function, uword function_arg)
vpe parser cli string response
static void vl_api_get_f64_endian_value_t_handler(vl_api_get_f64_endian_value_t *mp)
void vl_set_memory_root_path(const char *name)
Fixed length block allocator. Pools are built from clib vectors and bitmaps. Use pools when repeatedl...
VLIB_API_INIT_FUNCTION(af_xdp_plugin_api_hookup)
static api_main_t * vlibapi_get_main(void)
Reply for get next node index.
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
get_f64_endian_value reply message
Verify f64 wire format by sending a value and receiving the value + 1.0.
static void setup_message_id_table(api_main_t *am)
static void vl_api_log_dump_t_handler(vl_api_log_dump_t *mp)
static void vl_api_cli_inband_t_handler(vl_api_cli_inband_t *mp)
#define REPLY_MACRO3(t, n, body)
static void vl_api_get_node_index_t_handler(vl_api_get_node_index_t *mp)
static void vl_api_add_node_next_t_handler(vl_api_add_node_next_t *mp)
static void vl_api_show_threads_t_handler(vl_api_show_threads_t *mp)
u32 vl_api_string_len(vl_api_string_t *astr)
API main structure, used by both vpp and binary API clients.
#define vec_free(V)
Free vector's memory (no header).
vlib_thread_registration_t * registration
template key/value backing page structure
vlib_node_t * vlib_get_node_by_name(vlib_main_t *vm, u8 *name)
static void inband_cli_output(uword arg, u8 *buffer, uword buffer_bytes)
get_f64_increment_by_one reply
Control ping from client to api server request.
vl_api_timestamp_t timestamp
string build_directory[256]
int vl_api_vec_to_api_string(const u8 *vec, vl_api_string_t *str)
vl_api_thread_data_t thread_data[count]
void vl_set_api_memory_size(u64 size)
Process a vpe parser cli string request.
static void get_thread_data(vl_api_thread_data_t *td, int index)
unformat_function_t unformat_vnet_sw_interface
description fragment has unexpected format
static void show_log_details(vl_api_registration_t *reg, u32 context, f64 timestamp, vl_api_log_level_t *level, u8 *msg_class, u8 *message)
void * vl_msg_push_heap(void)
VL_MSG_API_REAPER_FUNCTION(memclnt_delete_callback)
vl_api_timestamp_t vpe_system_time
void vl_set_global_memory_size(u64 size)
struct timeval time_zero_timeval
clib_error_t * vpe_api_init(vlib_main_t *vm)
void * get_unformat_vnet_sw_interface(void)
#define vec_resize(V, N)
Resize a vector (no header, unspecified alignment) Add N elements to end of given vector V,...
static void vl_api_show_vpe_system_time_t_handler(vl_api_show_vpe_system_time_t *mp)
u8 * vl_api_from_api_to_new_vec(void *mp, vl_api_string_t *astr)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
#define clib_error_return_fatal(e, args...)
void vl_set_global_memory_baseva(u64 baseva)
static vlib_main_t * vlib_get_main(void)
static f64 clib_net_to_host_f64(f64 x)
IP Set the next node for a given node response.
#define clib_warning(format, args...)
void vl_msg_pop_heap(void *oldheap)
Get node index using name request.
u8 * format_vlib_log_class(u8 *s, va_list *args)
u8 * vlib_node_serialize(vlib_main_t *vm, vlib_node_t ***node_dups, u8 *vector, int include_nexts, int include_stats)
void vl_set_memory_gid(int gid)
void * vl_msg_api_alloc(int nbytes)
void vl_mem_api_enable_disable(vlib_main_t *vm, int yesno)