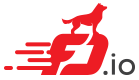 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
33 #include <vnet/l2/l2.api_enum.h>
34 #include <vnet/l2/l2.api_types.h>
37 #include <vnet/l2/l2.api.h>
64 if (flags & L2FIB_ENTRY_RESULT_FLAG_##a) \
65 s = format (s, "%s ", t); \
77 tmp = clib_net_to_host_u64 (
tmp);
79 tmp = clib_host_to_net_u64 (
tmp);
96 return format (s,
"Stale");
117 result.
raw = kvp->value;
119 if ((
ctx->bd_index == ~0) || (
ctx->bd_index ==
key.fields.bd_index))
125 return (BIHASH_WALK_CONTINUE);
135 .bd_index = bd_index,
141 *l2fe_key =
ctx.l2fe_key;
142 *l2fe_res =
ctx.l2fe_res;
160 s =
format (s,
"%3d/%-3d", bd_sn, if_sn);
186 if (
ctx->verbose &&
ctx->first_entry)
188 ctx->first_entry = 0;
190 "%=19s%=7s%=7s%=8s%=9s%=7s%=7s%=5s%=30s",
191 "Mac-Address",
"BD-Idx",
"If-Idx",
192 "BSN-ISN",
"Age(min)",
"static",
"filter",
193 "bvi",
"Interface-Name");
197 result.
raw = kvp->value;
198 ctx->total_entries++;
201 ((
ctx->bd_index >> 31) || (
ctx->bd_index ==
key.fields.bd_index)))
205 if (
ctx->learn && l2fib_entry_result_is_set_AGE_NOT (&result))
206 return (BIHASH_WALK_CONTINUE);
208 if (
ctx->add && !l2fib_entry_result_is_set_AGE_NOT (&result))
209 return (BIHASH_WALK_CONTINUE);
213 if (l2fib_entry_result_is_set_AGE_NOT (&result))
215 else if (bd_config->
mac_age == 0)
220 delta += delta < 0 ? 256 : 0;
221 s =
format (s,
"%d", delta);
225 "%=19U%=7d%=7d %U%=9v%=7s%=7s%=5s%=30U",
228 result.
fields.sw_if_index == ~0
229 ? -1 : result.
fields.sw_if_index,
231 l2fib_entry_result_is_set_STATIC (&result) ?
"*" :
"-",
232 l2fib_entry_result_is_set_FILTER (&result) ?
"*" :
"-",
233 l2fib_entry_result_is_set_BVI (&result) ?
"*" :
"-",
239 return (BIHASH_WALK_CONTINUE);
267 else if (
unformat (input,
"verbose"))
295 "bridge domain id %d doesn't exist\n",
311 if (
ctx.total_entries == 0)
317 "Last scan time: %.4esec Learn limit: %d ",
322 "Last e-scan time: %.4esec Delay: %.2esec "
323 "Max macs in event: %d",
330 BV (format_bihash), &msm->mac_table, 1 );
358 .path =
"show l2fib",
359 .short_help =
"show l2fib [all] | [bd_id <nn> | bd_index <nn>] [learn | add] | [raw]",
421 .path =
"clear l2fib",
422 .short_help =
"clear l2fib",
446 __attribute__ ((unused))
u32 bucket_contents;
449 BVT (clib_bihash_kv) kv;
451 if (
fm->mac_table_initialized == 0)
459 if (BV (clib_bihash_search) (&
fm->mac_table, &kv, &kv))
462 result.
raw = kv.value;
463 if (!l2fib_entry_result_is_set_AGE_NOT (&result))
486 l2fib_entry_result_set_AGE_NOT (&result);
488 kv.value = result.
raw;
552 flags |= L2FIB_ENTRY_RESULT_FLAG_STATIC;
554 flags |= (L2FIB_ENTRY_RESULT_FLAG_STATIC | L2FIB_ENTRY_RESULT_FLAG_BVI);
599 .short_help =
"l2fib add <mac> <bridge-domain-id> filter | <intf> [static | bvi]",
609 u8 mac[6], save_mac[6];
638 if (is_add == 0 && is_del == 0 && is_check == 0)
640 "noop: pick at least one of (add,del,check)");
656 BVT (clib_bihash_kv) kv;
667 if (BV (clib_bihash_search) (&mp->mac_table, &kv, &kv))
729 .path =
"test l2fib",
730 .short_help =
"test l2fib [add|del|check] mac <base-addr> count <nn>",
746 BVT (clib_bihash_kv) kv;
754 if (BV (clib_bihash_search) (&mp->mac_table, &kv, &kv))
757 result.
raw = kv.value;
764 if (!l2fib_entry_result_is_set_AGE_NOT (&result))
839 .short_help =
"l2fib del <mac> <bridge-domain-id> []",
852 if (!
unformat (input,
"%d", &scan_delay))
858 fm->event_scan_delay = (
f64) (scan_delay) *10e-3;
869 .path =
"set l2fib scan-delay",
870 .short_help =
"set l2fib scan-delay <delay>",
982 .path =
"l2fib flush-mac all",
983 .short_help =
"l2fib flush-mac all",
998 .path =
"l2fib flush-mac interface",
999 .short_help =
"l2fib flush-mac interface <if-name>",
1047 .path =
"l2fib flush-mac bridge-domain",
1048 .short_help =
"l2fib flush-mac bridge-domain <bd-id>",
1065 BVT (clib_bihash) * get_mac_table (
void)
1068 return &mp->mac_table;
1076 (
sizeof (*mp) + (
fm->max_macs_in_event * sizeof (vl_api_mac_entry_t)));
1078 mp->
pid = htonl (client);
1089 BVT (clib_bihash) *
h = &
fm->mac_table;
1091 f64 last_start = start_time;
1095 u32 learn_count = 0;
1101 static u32 *bd_learn_counts = 0;
1104 if (alloc_arena (
h) == 0)
1116 for (
i = 0;
i <
h->nbuckets;
i++)
1120 if (delta_t > 20e-6)
1130 if (
i < (
h->nbuckets - 3))
1132 BVT (clib_bihash_bucket) *
b =
1133 BV (clib_bihash_get_bucket) (
h,
i + 3);
1135 b = BV (clib_bihash_get_bucket) (
h,
i + 1);
1136 if (!BV (clib_bihash_bucket_is_empty) (
b))
1144 BVT (clib_bihash_bucket) *
b = BV (clib_bihash_get_bucket) (
h,
i);
1145 if (BV (clib_bihash_bucket_is_empty) (
b))
1148 for (j = 0; j < (1 <<
b->log2_pages); j++)
1152 if (v->kvp[k].key == ~0ULL && v->kvp[k].value == ~0ULL)
1158 if (!l2fib_entry_result_is_set_AGE_NOT (&result))
1161 vec_elt (bd_learn_counts,
key.fields.bd_index)++;
1171 mp->
n_macs = htonl (evt_idx);
1179 " %d MAC entries lost", client,
1185 if (l2fib_entry_result_is_set_LRN_EVT (&result))
1190 mp->
mac[evt_idx].action =
1191 l2fib_entry_result_is_set_LRN_MOV (&result) ?
1194 mp->
mac[evt_idx].action =
1195 htonl (mp->
mac[evt_idx].action);
1196 mp->
mac[evt_idx].sw_if_index =
1197 htonl (result.
fields.sw_if_index);
1199 l2fib_entry_result_clear_LRN_EVT (&result);
1200 l2fib_entry_result_clear_LRN_MOV (&result);
1201 BVT (clib_bihash_kv) kv;
1203 kv.value = result.
raw;
1210 if (event_only || l2fib_entry_result_is_set_AGE_NOT (&result))
1214 u32 bd_index =
key.fields.bd_index;
1217 if (result.
fields.sn != sn)
1226 i16 delta = (
u8) (start_time / 60) - result.
fields.timestamp;
1227 delta += delta < 0 ? 256 : 0;
1229 if (delta < bd_config->mac_age)
1238 mp->
mac[evt_idx].action =
1240 mp->
mac[evt_idx].action = htonl (mp->
mac[evt_idx].action);
1241 mp->
mac[evt_idx].sw_if_index =
1242 htonl (result.
fields.sw_if_index);
1246 BVT (clib_bihash_kv) kv;
1250 vec_elt (bd_learn_counts,
key.fields.bd_index)--;
1255 if (BV (clib_bihash_bucket_is_empty) (
b))
1269 vec_elt (bd_learn_counts, bd_index);
1279 mp->
n_macs = htonl (evt_idx);
1286 " %d MAC entries lost", client, evt_idx);
1293 return delta_t + accum_t;
1300 uword event_type, *event_data = 0;
1323 { SCAN_MAC_AGE, SCAN_MAC_EVENT, SCAN_DISABLE } scan = SCAN_MAC_AGE;
1328 if (lm->
client_pid != 0 && start_time < next_age_scan_time)
1329 scan = SCAN_MAC_EVENT;
1338 scan = SCAN_DISABLE;
1348 if (scan == SCAN_MAC_EVENT)
1352 if (scan == SCAN_MAC_AGE)
1354 if (scan == SCAN_DISABLE)
1373 .name =
"l2fib-mac-age-scanner-process",
1409 uword table_size = ~0;
1417 else if (
unformat (input,
"num-buckets %u", &n_buckets))
1424 if (n_buckets != ~0)
1431 if (table_size != ~0)
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
static void l2fib_add_filter_entry(const u8 *mac, u32 bd_index)
void l2fib_flush_int_mac(vlib_main_t *vm, u32 sw_if_index)
Flush all non static MACs from an interface.
L2 MAC event for a list of learned or aged MACs.
static vl_api_registration_t * vl_api_client_index_to_registration(u32 index)
uword unformat_ethernet_address(unformat_input_t *input, va_list *args)
static int l2fib_show_walk_cb(BVT(clib_bihash_kv) *kvp, void *arg)
static vlib_cli_command_t l2fib_set_scan_delay_cli
(constructor) VLIB_CLI_COMMAND (l2fib_set_scan_delay_cli)
static_always_inline void * allocate_mac_evt_buf(u32 client, u32 client_index)
@ L2_MAC_AGE_PROCESS_EVENT_START
const char *const const char *const raw
static vlib_cli_command_t l2fib_test_command
(constructor) VLIB_CLI_COMMAND (l2fib_test_command)
u8 * format_l2fib_entry_result_flags(u8 *s, va_list *args)
static void vl_api_send_msg(vl_api_registration_t *rp, u8 *elem)
#define BIHASH_KVP_PER_PAGE
struct l2fib_entry_result_t_::@469::@471 fields
static uword * vlib_process_wait_for_event(vlib_main_t *vm)
static vnet_sw_interface_t * vnet_get_sw_interface_or_null(vnet_main_t *vnm, u32 sw_if_index)
#define L2FIB_AGE_SCAN_INTERVAL
@ L2_MAC_AGE_PROCESS_EVENT_STOP
u8 * format_ethernet_address(u8 *s, va_list *args)
#define clib_error_return(e, args...)
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
struct l2fib_show_walk_ctx_t_ l2fib_show_walk_ctx_t
#define L2FIB_MEMORY_SIZE
void l2fib_start_ager_scan(vlib_main_t *vm)
Kick off ager to scan MACs to age/delete MAC entries.
static vlib_cli_command_t clear_l2fib_cli
(constructor) VLIB_CLI_COMMAND (clear_l2fib_cli)
static void * clib_bihash_get_value(clib_bihash *h, uword offset)
Get pointer to value page given its clib mheap offset.
static void vlib_process_signal_event(vlib_main_t *vm, uword node_index, uword type_opaque, uword data)
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
static clib_error_t * l2fib_del(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
Delete an entry from the L2FIB.
void l2fib_add_entry(const u8 *mac, u32 bd_index, u32 sw_if_index, l2fib_entry_result_flags_t flags)
Add an entry to the l2fib.
static clib_error_t * lfib_config(vlib_main_t *vm, unformat_input_t *input)
clib_error_t * l2fib_init(vlib_main_t *vm)
#define VLIB_CONFIG_FUNCTION(x, n,...)
#define vec_elt(v, i)
Get vector value at index i.
struct l2fib_dump_walk_ctx_t_ l2fib_dump_walk_ctx_t
static uword vlib_process_get_events(vlib_main_t *vm, uword **data_vector)
Return the first event type which has occurred and a vector of per-event data of that type,...
static vlib_cli_command_t show_l2fib_cli
(constructor) VLIB_CLI_COMMAND (show_l2fib_cli)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
static clib_error_t * clear_l2fib(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
Clear all entries in L2FIB.
void clib_bihash_init(clib_bihash *h, char *name, u32 nbuckets, uword memory_size)
initialize a bounded index extensible hash table
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
static clib_error_t * l2fib_flush_mac_all(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
Flush all MACs, except static ones The CLI format is: l2fib flush-mac all.
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
vnet_main_t * vnet_get_main(void)
static void incr_mac_address(u8 *mac)
An API client registration, only in vpp/vlib.
l2fib_entry_result_t * l2fe_res
l2fib_entry_key_t * l2fe_key
static int l2fib_dump_walk_cb(BVT(clib_bihash_kv) *kvp, void *arg)
void l2fib_flush_all_mac(vlib_main_t *vm)
Flush all non static MACs - flushes all valid BDs.
#define static_always_inline
#define L2FIB_NUM_BUCKETS
#define vec_foreach_index(var, v)
Iterate over vector indices.
void l2fib_clear_table(void)
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
void clib_bihash_free(clib_bihash *h)
Destroy a bounded index extensible hash table.
BVT(clib_bihash)
The table of adjacencies indexed by the rewrite string.
clib_error_t * l2fib_sw_interface_up_down(vnet_main_t *vnm, u32 sw_if_index, u32 flags)
static clib_error_t * l2fib_set_scan_delay(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static vlib_cli_command_t l2fib_flush_mac_all_cli
(constructor) VLIB_CLI_COMMAND (l2fib_flush_mac_all_cli)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
#define VLIB_CLI_COMMAND(x,...)
@ MAC_EVENT_ACTION_DELETE
uword mac_table_memory_size
static uword l2fib_mac_age_scanner_process(vlib_main_t *vm, vlib_node_runtime_t *rt, vlib_frame_t *f)
struct _vlib_node_registration vlib_node_registration_t
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static clib_error_t * show_l2fib(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
Display the contents of the l2fib.
l2learn_main_t l2learn_main
void l2fib_flush_bd_mac(vlib_main_t *vm, u32 bd_index)
Flush all non static MACs in a bridge domain.
static vlib_cli_command_t l2fib_flush_mac_int_cli
(constructor) VLIB_CLI_COMMAND (l2fib_flush_mac_int_cli)
#define vec_free(V)
Free vector's memory (no header).
static vlib_cli_command_t l2fib_flush_mac_bd_cli
(constructor) VLIB_CLI_COMMAND (l2fib_flush_mac_bd_cli)
template key/value backing page structure
static u32 bd_is_valid(l2_bridge_domain_t *bd_config)
@ foreach_l2fib_entry_result_attr
static u64 l2fib_make_key(const u8 *mac_address, u16 bd_index)
void vl_msg_api_free(void *)
static_always_inline f64 l2fib_scan(vlib_main_t *vm, f64 start_time, u8 event_only)
unformat_function_t unformat_vnet_sw_interface
static f64 vlib_process_wait_for_event_or_clock(vlib_main_t *vm, f64 dt)
Suspend a cooperative multi-tasking thread Waits for an event, or for the indicated number of seconds...
description fragment has unexpected format
static int vl_api_can_send_msg(vl_api_registration_t *rp)
static l2fib_seq_num_t l2fib_cur_seq_num(u32 bd_index, u32 sw_if_index)
static uword vlib_process_suspend(vlib_main_t *vm, f64 dt)
Suspend a vlib cooperative multi-tasking thread for a period of time.
#define VLIB_INIT_FUNCTION(x)
static_always_inline l2fib_seq_num_t l2_fib_mk_seq_num(u8 bd_sn, u8 if_sn)
static_always_inline void clib_prefetch_load(void *p)
u32 l2fib_del_entry(const u8 *mac, u32 bd_index, u32 sw_if_index)
Delete an entry from the l2fib.
#define vec_foreach(var, vec)
Vector iterator.
int clib_bihash_add_del(clib_bihash *h, clib_bihash_kv *add_v, int is_add)
Add or delete a (key,value) pair from a bi-hash table.
static clib_error_t * l2fib_flush_mac_int(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
Flush MACs, except static ones, associated with an interface The CLI format is: l2fib flush-mac inter...
u8 * format_l2_fib_seq_num(u8 *s, va_list *a)
vl_api_mac_entry_t mac[n_macs]
vlib_node_registration_t l2fib_mac_age_scanner_process_node
(constructor) VLIB_REGISTER_NODE (l2fib_mac_age_scanner_process_node)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
uword mac_table_n_buckets
void clib_bihash_foreach_key_value_pair(clib_bihash *h, clib_bihash_foreach_key_value_pair_cb *callback, void *arg)
Visit active (key,value) pairs in a bi-hash table.
static vlib_main_t * vlib_get_main(void)
vnet_interface_output_runtime_t * rt
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
static vlib_cli_command_t l2fib_del_cli
(constructor) VLIB_CLI_COMMAND (l2fib_del_cli)
static vlib_cli_command_t l2fib_add_cli
(constructor) VLIB_CLI_COMMAND (l2fib_add_cli)
static clib_error_t * l2fib_flush_mac_bd(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
Flush bridge-domain MACs except static ones.
enum l2fib_entry_result_flags_t_ l2fib_entry_result_flags_t
#define clib_warning(format, args...)
u16 l2fib_seq_num_t
A combined representation of the sequence number associated with the interface and the BD.
static unsigned char test_key[46]
static f64 vlib_time_now(vlib_main_t *vm)
@ L2_MAC_AGE_PROCESS_EVENT_ONE_PASS
VNET_SW_INTERFACE_ADMIN_UP_DOWN_FUNCTION(l2fib_sw_interface_up_down)
u8 * format_vnet_sw_if_index_name_with_NA(u8 *s, va_list *args)
Format sw_if_index.
l2_input_config_t * configs
void l2fib_table_dump(u32 bd_index, l2fib_entry_key_t **l2fe_key, l2fib_entry_result_t **l2fe_res)
@ L2FIB_ENTRY_RESULT_FLAG_NONE
static uword is_pow2(uword x)
vl_api_interface_index_t sw_if_index
void l2_fib_extract_seq_num(l2fib_seq_num_t sn, u8 *bd_sn, u8 *if_sn)
uword * bd_index_by_bd_id
void l2fib_table_init(void)
l2_bridge_domain_t * bd_configs
format_function_t format_vnet_sw_interface_name
static clib_error_t * l2fib_add(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
Add an entry to the L2FIB.
static clib_error_t * l2fib_test_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
void * vl_msg_api_alloc(int nbytes)
#define VLIB_REGISTER_NODE(x,...)
vl_api_wireguard_peer_flags_t flags