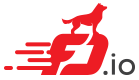 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
19 #include <sys/socket.h>
23 #include <netlink/route/link/vlan.h>
24 #include <linux/if_ether.h>
76 #define LCP_ITF_PAIR_DBG(...) \
77 vlib_log_notice (lcp_itf_pair_logger, __VA_ARGS__);
79 #define LCP_ITF_PAIR_INFO(...) \
80 vlib_log_notice (lcp_itf_pair_logger, __VA_ARGS__);
82 #define LCP_ITF_PAIR_ERR(...) vlib_log_err (lcp_itf_pair_logger, __VA_ARGS__);
96 s =
format (s,
" <no-phy-if>");
102 s =
format (s,
" <no-host-if>");
141 ns ? (
char *) ns :
"<unset>");
143 if (phy_sw_if_index == ~0)
179 u32 phy_sw_if_index,
u8 *ns)
189 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
198 [
AF_IP4] =
"linux-cp-xc-ip4",
199 [
AF_IP6] =
"linux-cp-xc-ip6",
202 [
AF_IP4] =
"linux-cp-xc-l3-ip4",
203 [
AF_IP6] =
"linux-cp-xc-l3-ip6",
260 return VNET_API_ERROR_VALUE_EXIST;
354 struct rtnl_link *link;
358 sk = nl_socket_alloc ();
359 if ((err = nl_connect (sk, NETLINK_ROUTE)) < 0)
366 link = rtnl_link_vlan_alloc ();
368 rtnl_link_set_link (link, parent);
369 rtnl_link_set_name (link,
name);
370 rtnl_link_vlan_set_id (link, vlan);
371 rtnl_link_vlan_set_protocol (link, htons (
proto));
373 if ((err = rtnl_link_add (sk, link, NLM_F_CREATE)) < 0)
380 rtnl_link_put (link);
389 struct rtnl_link *link;
393 sk = nl_socket_alloc ();
394 if ((err = nl_connect (sk, NETLINK_ROUTE)) < 0)
397 link = rtnl_link_alloc ();
398 rtnl_link_set_name (link,
name);
400 if ((err = rtnl_link_delete (sk, link)) < 0)
403 rtnl_link_put (link);
420 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
476 u32 host_sw_if_index;
506 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
542 host = phy = ns = default_ns = NULL;
548 if (
unformat (input,
"pair %s %s %s", &phy, &
host, &ns))
555 "linux-cp namespace must"
556 " be less than %d characters",
576 else if (
unformat (input,
"default netns %v", &default_ns))
582 "linux-cp default namespace must"
583 " be less than %d characters",
587 else if (
unformat (input,
"interface-auto-create"))
642 int curr_ns_fd, vif_ns_fd;
647 curr_ns_fd = vif_ns_fd = -1;
662 if (curr_ns_fd != -1)
691 "inner-dot1q %d: interface %U",
729 u32 *host_sw_if_indexp)
733 u32 vif_index = 0, host_sw_if_index;
741 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
748 return VNET_API_ERROR_INVALID_ARGUMENT;
757 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
764 if (ns == 0 || ns[0] == 0)
771 int orig_ns_fd, ns_fd;
773 u16 outer_vlan, inner_vlan;
774 u16 outer_proto, inner_proto;
776 u32 parent_vif_index;
783 "sub-interface without exact-match set");
784 return VNET_API_ERROR_INVALID_ARGUMENT;
789 outer_proto = inner_proto = ETH_P_8021Q;
791 outer_proto = ETH_P_8021AD;
804 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
810 "sub-interface without an LCP on the parent");
811 return VNET_API_ERROR_INVALID_ARGUMENT;
819 orig_ns_fd = ns_fd = -1;
822 if (ns && ns[0] != 0)
826 if (orig_ns_fd == -1 || ns_fd == -1)
860 "pair_create: can't find LCP for outer vlan %d "
863 outer_proto == ETH_P_8021AD ?
"dot1ad" :
"dot1q",
880 (
const char *) host_if_name);
884 "outer(proto:0x%04x,vlan:%u).inner(proto:0x%"
885 "04x,vlan:%u) name:'%s'",
886 outer_proto, outer_vlan, inner_proto,
887 inner_vlan, host_if_name);
900 outer_vlan, &host_sw_if_index))
903 "pair_create: failed to create tap subint: %d.%d on %U",
907 0,
"failed to create tap subinti: %d.%d. on %U", outer_vlan,
913 if (orig_ns_fd != -1)
922 return VNET_API_ERROR_INVALID_ARGUMENT;
932 .host_if_name = host_if_name,
951 if (ns && ns[0] != 0)
1014 host_sw_if_index, host_if_name);
1015 lcp_itf_pair_add (host_sw_if_index, phy_sw_if_index, host_if_name, vif_index,
1030 if (host_sw_if_indexp)
1031 *host_sw_if_indexp = host_sw_if_index;
1096 uword *event_data = 0;
1123 .name =
"linux-cp-itf-process",
1214 .runs_after =
VLIB_INITS (
"vnet_interface_init",
"tcp_init",
"udp_init"),
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
#define LCP_ITF_PAIR_INFO(...)
void udp_punt_unknown(vlib_main_t *vm, u8 is_ip4, u8 is_add)
void ip_feature_enable_disable(ip_address_family_t af, ip_sub_address_family_t safi, ip_feature_location_t loc, const char *feature_name, u32 sw_if_index, int enable, void *feature_config, u32 n_feature_config_bytes)
struct lcp_itf_pair_names_t_ lcp_itf_pair_names_t
static u32 vlib_num_workers()
static clib_error_t * lcp_itf_pair_init(vlib_main_t *vm)
const char * lcp_itf_l3_feat_names[N_LCP_ITF_HOST][N_AF]
struct vnet_sub_interface_t::@374 eth
#define LCP_ITF_PAIR_DBG(...)
#define hash_set(h, key, value)
static int lcp_validate_if_name(u8 *name)
vnet_sw_interface_type_t type
u8 * format_lcp_itf_pair(u8 *s, va_list *args)
static uword lcp_itf_pair_process(vlib_main_t *vm, vlib_node_runtime_t *rt, vlib_frame_t *f)
void vnet_sw_interface_set_mtu(vnet_main_t *vnm, u32 sw_if_index, u32 mtu)
static walk_rc_t lcp_itf_pair_walk_sweep(index_t lipi, void *arg)
void ip6_punt_redirect_del(u32 rx_sw_if_index)
index_t lcp_itf_pair_find_by_vif(u32 vif_index)
Find a interface-pair object from the host interface.
struct vnet_sub_interface_t::@374::@375::@377 flags
static clib_error_t * lcp_netlink_add_link_vlan(int parent, u32 vlan, u16 proto, const char *name)
static index_t lcp_itf_pair_find_by_outer_vlan(u32 sup_if_index, u16 vlan, bool dot1ad)
const ip46_address_t zero_addr
#include <vnet/feature/feature.h>
void lcp_set_auto_intf(u8 is_auto)
manage interface auto creation
adj_index_t adj_nbr_add_or_lock(fib_protocol_t nh_proto, vnet_link_t link_type, const ip46_address_t *nh_addr, u32 sw_if_index)
Neighbour Adjacency sub-type.
void tap_create_if(vlib_main_t *vm, tap_create_if_args_t *args)
static uword * vlib_process_wait_for_event(vlib_main_t *vm)
u8 * lcp_get_default_ns(void)
static clib_error_t * lcp_itf_pair_config(vlib_main_t *vm, unformat_input_t *input)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
static vnet_sw_interface_t * vnet_get_sw_interface_or_null(vnet_main_t *vnm, u32 sw_if_index)
int vnet_create_sub_interface(u32 sw_if_index, u32 id, u32 flags, u16 inner_vlan_id, u16 outer_vlan_id, u32 *sub_sw_if_index)
#define ETHERNET_INTERFACE_FLAG_STATUS_L3
vlib_log_class_t vlib_log_register_class(char *class, char *subclass)
adj_index_t adj_mcast_add_or_lock(fib_protocol_t proto, vnet_link_t link_type, u32 sw_if_index)
Mcast Adjacency.
u32 lcp_itf_num_pairs(void)
vnet_hw_interface_flags_t flags
static vlib_punt_hdl_t punt_hdl
@ VNET_SW_INTERFACE_TYPE_SUB
#define clib_error_return(e, args...)
void adj_unlock(adj_index_t adj_index)
Release a reference counting lock on the adjacency.
unsigned int if_nametoindex(const char *ifname)
#define FOR_EACH_IP_ADDRESS_FAMILY(_af)
static vlib_node_registration_t lcp_itf_pair_process_node
(constructor) VLIB_REGISTER_NODE (lcp_itf_pair_process_node)
static void lcp_itf_pair_delete_by_index(index_t lipi)
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
#define pool_put(P, E)
Free an object E in pool P.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static vnet_sw_interface_t * vnet_get_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
@ VNET_HW_INTERFACE_FLAG_LINK_UP
vl_api_dhcp_client_state_t state
VNET_HW_INTERFACE_LINK_UP_DOWN_FUNCTION(lcp_itf_pair_link_up_down)
int lcp_itf_pair_add(u32 host_sw_if_index, u32 phy_sw_if_index, u8 *host_name, u32 host_index, lip_host_type_t host_type, u8 *ns)
Create an interface-pair.
int vlib_punt_register(vlib_punt_hdl_t client, vlib_punt_reason_t reason, const char *node_name)
Register a node to receive particular punted buffers.
dpo_proto_t frp_proto
The protocol of the address below.
int lcp_itf_pair_add_sub(u32 vif, u8 *host_if_name, u32 sub_sw_if_index, u32 phy_sw_if_index, u8 *ns)
lcp_itf_phy_adj_t lip_phy_adjs
static void vlib_process_signal_event(vlib_main_t *vm, uword node_index, uword type_opaque, uword data)
__clib_export int clib_netns_open(u8 *netns_u8)
#define vec_cmp(v1, v2)
Compare two vectors (only applicable to vectors of signed numbers).
static clib_error_t * lcp_itf_phy_add(vnet_main_t *vnm, u32 sw_if_index, u32 is_create)
adj_index_t adj_index[N_AF]
@ FIB_ROUTE_PATH_DVR
A path that resolves via a DVR DPO.
#define VLIB_EARLY_CONFIG_FUNCTION(x, n,...)
#define hash_unset(h, key)
import vnet interface_types api
vnet_sw_interface_flags_t flags
index_t * lip_db_by_phy
Retreive the pair in the DP.
lcp_itf_pair_t * lcp_itf_pair_get(u32 index)
Get an interface-pair object from its VPP index.
virtio_main_t virtio_main
ethernet_interface_t * interfaces
static uword vlib_process_get_events(vlib_main_t *vm, uword **data_vector)
Return the first event type which has occurred and a vector of per-event data of that type,...
static_always_inline void mac_address_copy(mac_address_t *dst, const mac_address_t *src)
walk_rc_t(* lcp_itf_pair_walk_cb_t)(index_t index, void *ctx)
Callback function invoked during a walk of all interface-pairs.
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
void vnet_sw_interface_admin_up(vnet_main_t *vnm, u32 sw_if_index)
int lcp_itf_pair_create(u32 phy_sw_if_index, u8 *host_if_name, lip_host_type_t host_if_type, u8 *ns, u32 *host_sw_if_indexp)
Create an interface-pair from PHY sw_if_index and tap name.
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
static vlib_log_class_t lcp_itf_pair_logger
#define vec_dup(V)
Return copy of vector (no header, no alignment)
clib_error_t * vnet_netlink_set_link_state(int ifindex, int up)
vnet_main_t * vnet_get_main(void)
int lcp_itf_pair_del(u32 phy_sw_if_index)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
static uword vnet_sw_interface_is_sub(vnet_main_t *vnm, u32 sw_if_index)
void lcp_itf_pair_register_vft(lcp_itf_pair_vft_t *lcp_itf_vft)
int tap_delete_if(vlib_main_t *vm, u32 sw_if_index)
lcp_itf_pair_add_cb_t pair_add_fn
void ip6_punt_redirect_add_paths(u32 rx_sw_if_index, const fib_route_path_t *paths)
int lcp_itf_pair_replace_begin(void)
Begin and End the replace process.
__clib_export int clib_setns(int nfd)
lcp_itf_pair_del_cb_t pair_del_fn
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
static lcp_itf_pair_vft_t * lcp_itf_vfts
vector of virtual function table
void tcp_punt_unknown(vlib_main_t *vm, u8 is_ip4, u8 is_add)
VNET_SW_INTERFACE_ADD_DEL_FUNCTION(lcp_itf_phy_add)
struct _vlib_node_registration vlib_node_registration_t
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static lcp_itf_pair_names_t * lipn_names
static walk_rc_t lcp_itf_pair_walk_mark(index_t lipi, void *ctx)
const fib_route_path_flags_t lcp_itf_route_path_flags[N_LCP_ITF_HOST]
static clib_error_t * lcp_itf_pair_link_up_down(vnet_main_t *vnm, u32 hw_if_index, u32 flags)
lip_host_type_t lip_host_type
static void lcp_itf_set_adjs(lcp_itf_pair_t *lip)
static uword vnet_sw_if_index_is_api_valid(u32 sw_if_index)
int lcp_itf_pair_delete(u32 phy_sw_if_index)
Delete a LCP_ITF_PAIR.
description ARP replies copied to host
#define clib_strnlen(s, m)
#define vec_free(V)
Free vector's memory (no header).
fib_route_path_flags_t frp_flags
flags on the path
static walk_rc_t lcp_itf_pair_find_walk(vnet_main_t *vnm, u32 sw_if_index, void *arg)
#define pool_foreach_index(i, v)
ethernet_main_t ethernet_main
format_function_t format_vnet_sw_if_index_name
description fragment has unexpected format
#define vec_validate_init_empty(V, I, INIT)
Make sure vector is long enough for given index and initialize empty space (no header,...
@ FIB_ROUTE_PATH_FLAG_NONE
#define VLIB_INIT_FUNCTION(x)
static vnet_hw_interface_t * vnet_get_sup_hw_interface(vnet_main_t *vnm, u32 sw_if_index)
vl_api_address_family_t af
int tap_set_carrier(u32 hw_if_index, u32 carrier_up)
A representation of a path as described by a route producer.
static vnet_hw_interface_t * vnet_get_hw_interface_or_null(vnet_main_t *vnm, u32 hw_if_index)
#define vec_foreach(var, vec)
Vector iterator.
static uword pool_elts(void *v)
Number of active elements in a pool.
struct lcp_itf_pair_sweep_ctx_t_ lcp_itf_pair_sweep_ctx_t
int vlib_punt_hdl_t
Typedef for a client handle.
static void lcp_itf_set_link_state(const lcp_itf_pair_t *lip, u8 state)
int vnet_feature_enable_disable(const char *arc_name, const char *node_name, u32 sw_if_index, int enable_disable, void *feature_config, u32 n_feature_config_bytes)
static vlib_main_t * vlib_get_main(void)
static uword * lip_db_by_vif
DBs of interface-pair objects:
void ip4_punt_redirect_del(u32 rx_sw_if_index)
vnet_interface_output_runtime_t * rt
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
static index_t lcp_itf_pair_find_by_phy(u32 phy_sw_if_index)
#define ETHERNET_MAX_PACKET_BYTES
void lcp_itf_pair_walk(lcp_itf_pair_walk_cb_t cb, void *ctx)
Walk/visit each of the interface pairs.
#define LCP_ITF_PAIR_ERR(...)
enum fib_route_path_flags_t_ fib_route_path_flags_t
Path flags from the control plane.
lcp_itf_pair_t * lcp_itf_pair_pool
Pool of LIP objects.
void vnet_hw_interface_walk_sw(vnet_main_t *vnm, u32 hw_if_index, vnet_hw_sw_interface_walk_t fn, void *ctx)
Walk the SW interfaces on a HW interface - this is the super interface and any sub-interfaces.
static f64 vlib_time_now(vlib_main_t *vm)
vlib_punt_hdl_t vlib_punt_client_register(const char *who)
Register a new clinet.
void ip4_punt_redirect_add_paths(u32 rx_sw_if_index, const fib_route_path_t *paths)
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
void lcp_itf_pair_show(u32 phy_sw_if_index)
vl_api_interface_index_t sw_if_index
ethernet_interface_address_t address
int lcp_itf_pair_replace_end(void)
enum walk_rc_t_ walk_rc_t
Walk return code.
static ip_adjacency_t * adj_get(adj_index_t adj_index)
Get a pointer to an adjacency object from its index.
vlib_punt_reason_t ipsec_punt_reason[IPSEC_PUNT_N_REASONS]
int lcp_set_default_ns(u8 *ns)
Get/Set the default namespace for LCP host taps.
static walk_rc_t lcp_itf_pair_walk_show_cb(index_t api, void *ctx)
int vnet_delete_sub_interface(u32 sw_if_index)
mac_address_t host_mac_addr
format_function_t format_vnet_sw_interface_name
enum ip_address_family_t_ ip_address_family_t
int tap_set_speed(u32 hw_if_index, u32 speed)
static void lcp_itf_unset_adjs(lcp_itf_pair_t *lip)
#define VLIB_REGISTER_NODE(x,...)
vl_api_wireguard_peer_flags_t flags
static clib_error_t * lcp_netlink_del_link(const char *name)