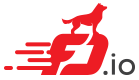 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
49 u8 code = va_arg (*args,
int);
52 #define _(n,f) case n: t = #f; break;
70 switch ((
type << 8) | code)
72 #define _(a,n,f) case (ICMP6_##a << 8) | (n): t = #f; break;
88 icmp46_header_t *
icmp = va_arg (*args, icmp46_header_t *);
89 u32 max_header_bytes = va_arg (*args,
u32);
92 if (max_header_bytes <
sizeof (
icmp[0]))
93 return format (s,
"ICMP header truncated");
95 s =
format (s,
"ICMP %U checksum 0x%x",
97 clib_net_to_host_u16 (
icmp->checksum));
99 if (max_header_bytes >=
100 sizeof (icmp6_neighbor_solicitation_or_advertisement_header_t) &&
101 (
icmp->type == ICMP6_neighbor_solicitation ||
102 icmp->type == ICMP6_neighbor_advertisement))
104 icmp6_neighbor_solicitation_or_advertisement_header_t *icmp6_nd =
105 (icmp6_neighbor_solicitation_or_advertisement_header_t *)
icmp;
106 s =
format (s,
"\n target address %U",
145 u8 input_next_index_by_type[256];
148 u8 max_valid_code_by_type[256];
151 u8 min_valid_hop_limit_by_type[256];
153 u8 min_valid_length_by_type[256];
183 icmp46_header_t *icmp0;
185 u32 bi0, next0, error0, len0;
187 bi0 = to_next[0] =
from[0];
199 error0 = ICMP6_ERROR_NONE;
201 next0 =
im->input_next_index_by_type[type0];
208 im->max_valid_code_by_type[type0] ?
209 ICMP6_ERROR_INVALID_CODE_FOR_TYPE : error0;
216 im->min_valid_hop_limit_by_type[type0] ?
217 ICMP6_ERROR_INVALID_HOP_LIMIT_FOR_TYPE : error0;
222 im->min_valid_length_by_type[type0] ?
223 ICMP6_ERROR_LENGTH_TOO_SMALL_FOR_TYPE : error0;
230 to_next, n_left_to_next,
237 return frame->n_vectors;
243 .name =
"ip6-icmp-input",
245 .vector_size =
sizeof (
u32),
291 icmp46_header_t *icmp0, *icmp1;
292 ip6_address_t tmp0, tmp1;
295 u32 fib_index0, fib_index1;
299 bi0 = to_next[0] =
from[0];
300 bi1 = to_next[1] =
from[1];
315 sum0 = icmp0->checksum;
316 sum1 = icmp1->checksum;
318 ASSERT (icmp0->type == ICMP6_echo_request);
319 ASSERT (icmp1->type == ICMP6_echo_request);
320 sum0 =
ip_csum_update (sum0, ICMP6_echo_request, ICMP6_echo_reply,
321 icmp46_header_t,
type);
322 sum1 =
ip_csum_update (sum1, ICMP6_echo_request, ICMP6_echo_reply,
323 icmp46_header_t,
type);
328 icmp0->type = ICMP6_echo_reply;
329 icmp1->type = ICMP6_echo_reply;
346 fib_index0 =
vec_elt (
im->fib_index_by_sw_if_index,
350 fib_index1 =
vec_elt (
im->fib_index_by_sw_if_index,
354 p0->
flags |= VNET_BUFFER_F_LOCALLY_ORIGINATED;
355 p1->
flags |= VNET_BUFFER_F_LOCALLY_ORIGINATED;
360 to_next, n_left_to_next,
361 bi0, bi1, next0, next1);
368 icmp46_header_t *icmp0;
375 bi0 = to_next[0] =
from[0];
387 sum0 = icmp0->checksum;
389 ASSERT (icmp0->type == ICMP6_echo_request);
390 sum0 =
ip_csum_update (sum0, ICMP6_echo_request, ICMP6_echo_reply,
391 icmp46_header_t,
type);
395 icmp0->type = ICMP6_echo_reply;
406 fib_index0 =
vec_elt (
im->fib_index_by_sw_if_index,
410 p0->
flags |= VNET_BUFFER_F_LOCALLY_ORIGINATED;
413 to_next, n_left_to_next,
421 ICMP6_ERROR_ECHO_REPLIES_SENT,
frame->n_vectors);
423 return frame->n_vectors;
429 .name =
"ip6-icmp-echo-request",
431 .vector_size =
sizeof (
u32),
463 case ICMP6_destination_unreachable:
464 return ICMP6_ERROR_DEST_UNREACH_SENT;
465 case ICMP6_packet_too_big:
466 return ICMP6_ERROR_PACKET_TOO_BIG_SENT;
467 case ICMP6_time_exceeded:
468 return ICMP6_ERROR_TTL_EXPIRE_SENT;
469 case ICMP6_parameter_problem:
470 return ICMP6_ERROR_PARAM_PROBLEM_SENT;
472 return ICMP6_ERROR_DROP;
509 u8 error0 = ICMP6_ERROR_NONE;
512 icmp46_header_t *icmp0;
518 if (!p0 || pi0 == ~0)
534 sizeof (icmp46_header_t) + 4));
537 p0->
flags |= VNET_BUFFER_F_LOCALLY_ORIGINATED;
542 icmp0 = (icmp46_header_t *) & out_ip0[1];
546 clib_host_to_net_u32 (0x6 << 28);
550 out_ip0->
protocol = IP_PROTOCOL_ICMP6;
558 error0 = ICMP6_ERROR_DROP;
564 *((
u32 *) (icmp0 + 1)) =
565 clib_host_to_net_u32 (
vnet_buffer (p0)->ip.icmp.data);
572 if (error0 == ICMP6_ERROR_NONE)
579 to_next, n_left_to_next,
594 return frame->n_vectors;
600 .name =
"ip6-icmp-error",
601 .vector_size =
sizeof (
u32),
620 icmp46_header_t *
h = va_arg (*args, icmp46_header_t *);
625 cm->type_and_code_by_name, &
i))
627 h->type = (
i >> 8) & 0xff;
628 h->code = (
i >> 0) & 0xff;
631 cm->type_by_name, &
i))
648 u32 ip_offset, icmp_offset;
652 ip_offset = (g - 1)->start_byte_offset;
654 while (n_packets >= 1)
658 icmp46_header_t *icmp0;
665 ip0 = (
void *) (p0->
data + ip_offset);
666 icmp0 = (
void *) (p0->
data + icmp_offset);
670 ASSERT (bogus_length == 0);
684 #define _(f) pg_edit_init (&p->f, icmp46_header_t, f);
750 im->input_next_index_by_type[
type]
772 #define _(n,t) hash_set_mem (cm->type_by_name, #t, (n));
777 #define _(a,n,t) hash_set_mem (cm->type_by_name, #t, (n) | (ICMP6_##a << 8));
784 sizeof (
cm->max_valid_code_by_type));
786 #define _(a,n,t) cm->max_valid_code_by_type[ICMP6_##a] = clib_max (cm->max_valid_code_by_type[ICMP6_##a], n);
791 sizeof (
cm->min_valid_hop_limit_by_type));
792 cm->min_valid_hop_limit_by_type[ICMP6_router_solicitation] = 255;
793 cm->min_valid_hop_limit_by_type[ICMP6_router_advertisement] = 255;
794 cm->min_valid_hop_limit_by_type[ICMP6_neighbor_solicitation] = 255;
795 cm->min_valid_hop_limit_by_type[ICMP6_neighbor_advertisement] = 255;
796 cm->min_valid_hop_limit_by_type[ICMP6_redirect] = 255;
798 clib_memset (
cm->min_valid_length_by_type, sizeof (icmp46_header_t),
799 sizeof (
cm->min_valid_length_by_type));
800 cm->min_valid_length_by_type[ICMP6_router_solicitation] =
801 sizeof (icmp6_neighbor_discovery_header_t);
802 cm->min_valid_length_by_type[ICMP6_router_advertisement] =
803 sizeof (icmp6_router_advertisement_header_t);
804 cm->min_valid_length_by_type[ICMP6_neighbor_solicitation] =
805 sizeof (icmp6_neighbor_solicitation_or_advertisement_header_t);
806 cm->min_valid_length_by_type[ICMP6_neighbor_advertisement] =
807 sizeof (icmp6_neighbor_solicitation_or_advertisement_header_t);
808 cm->min_valid_length_by_type[ICMP6_redirect] =
809 sizeof (icmp6_redirect_header_t);
static char * icmp_error_strings[]
vnet_interface_main_t * im
static void pg_edit_set_fixed(pg_edit_t *e, u64 value)
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
void icmp6_error_set_vnet_buffer(vlib_buffer_t *b, u8 type, u8 code, u32 data)
static uword vlib_node_add_next(vlib_main_t *vm, uword node, uword next_node)
u16 ip6_tcp_udp_icmp_compute_checksum(vlib_main_t *vm, vlib_buffer_t *p0, ip6_header_t *ip0, int *bogus_lengthp)
nat44_ei_hairpin_src_next_t next_index
static void pg_icmp_header_init(pg_icmp46_header_t *p)
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
static uword ip6_icmp_echo_request(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
void vlib_trace_frame_buffers_only(vlib_main_t *vm, vlib_node_runtime_t *node, u32 *buffers, uword n_buffers, uword next_buffer_stride, uword n_buffer_data_bytes_in_trace)
vlib_main_t vlib_node_runtime_t * node
static ip_protocol_info_t * ip_get_protocol_info(ip_main_t *im, u32 protocol)
#define hash_create_string(elts, value_bytes)
format_function_t * format_header
static uword ip6_icmp_error(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
#define vlib_call_init_function(vm, x)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
uword unformat_pg_number(unformat_input_t *input, va_list *args)
icmp6_echo_request_next_t
static void vlib_error_count(vlib_main_t *vm, uword node_index, uword counter, uword increment)
static void * ip6_next_header(ip6_header_t *i)
static vlib_node_registration_t ip6_icmp_echo_request_node
(constructor) VLIB_REGISTER_NODE (ip6_icmp_echo_request_node)
#define vec_elt(v, i)
Get vector value at index i.
void icmp6_register_type(vlib_main_t *vm, icmp6_type_t type, u32 node_index)
i16 current_data
signed offset in data[], pre_data[] that we are currently processing.
static_always_inline void vlib_buffer_enqueue_to_single_next(vlib_main_t *vm, vlib_node_runtime_t *node, u32 *buffers, u16 next_index, u32 count)
uword edit_function_opaque
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
vlib_error_t error
Error code for buffers to be enqueued to error handler.
static uword ip6_icmp_input(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
#define VLIB_NODE_FLAG_TRACE
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
@ ICMP6_ECHO_REQUEST_NEXT_LOOKUP
static clib_error_t * icmp6_init(vlib_main_t *vm)
vnet_feature_config_main_t * cm
@ ICMP6_ECHO_REQUEST_NEXT_OUTPUT
static pg_edit_group_t * pg_stream_get_group(pg_stream_t *s, u32 group_index)
static void pg_free_edit_group(pg_stream_t *s)
clib_error_t * ip_main_init(vlib_main_t *vm)
static vlib_buffer_t * vlib_buffer_copy_no_chain(vlib_main_t *vm, vlib_buffer_t *b, u32 *di)
struct _vlib_node_registration vlib_node_registration_t
uword unformat_pg_edit(unformat_input_t *input, va_list *args)
@ IP6_ICMP_ERROR_NEXT_DROP
u16 current_length
Nbytes between current data and the end of this buffer.
static uword unformat_icmp_type_and_code(unformat_input_t *input, va_list *args)
u8 * format_icmp6_input_trace(u8 *s, va_list *va)
uword unformat_pg_payload(unformat_input_t *input, va_list *args)
#define vlib_validate_buffer_enqueue_x1(vm, node, next_index, to_next, n_left_to_next, bi0, next0)
Finish enqueueing one buffer forward in the graph.
vlib_node_registration_t ip6_icmp_error_node
(constructor) VLIB_REGISTER_NODE (ip6_icmp_error_node)
description fragment has unexpected format
static void * pg_create_edit_group(pg_stream_t *s, int n_edit_bytes, int n_packet_bytes, u32 *group_index)
vlib_put_next_frame(vm, node, next_index, 0)
#define VLIB_INIT_FUNCTION(x)
static uword unformat_pg_icmp_header(unformat_input_t *input, va_list *args)
static u8 icmp6_icmp_type_to_error(u8 type)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
#define ip_csum_update(sum, old, new, type, field)
static vlib_main_t * vlib_get_main(void)
uword * type_and_code_by_name
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
@ ICMP6_ECHO_REQUEST_N_NEXT
bool ip6_sas_by_sw_if_index(u32 sw_if_index, const ip6_address_t *dst, ip6_address_t *src)
#define vlib_validate_buffer_enqueue_x2(vm, node, next_index, to_next, n_left_to_next, bi0, bi1, next0, next1)
Finish enqueueing two buffers forward in the graph.
vlib_node_registration_t ip6_icmp_input_node
(constructor) VLIB_REGISTER_NODE (ip6_icmp_input_node)
static u8 * format_ip6_icmp_type_and_code(u8 *s, va_list *args)
void(* edit_function)(struct pg_main_t *pg, struct pg_stream_t *s, struct pg_edit_group_t *g, u32 *buffers, u32 n_buffers)
vl_api_interface_index_t sw_if_index
#define vlib_get_next_frame(vm, node, next_index, vectors, n_vectors_left)
Get pointer to next frame vector data by (vlib_node_runtime_t, next_index).
static u16 ip_csum_fold(ip_csum_t c)
unformat_function_t * unformat_pg_edit
static void icmp6_pg_edit_function(pg_main_t *pg, pg_stream_t *s, pg_edit_group_t *g, u32 *packets, u32 n_packets)
static u8 * format_icmp6_header(u8 *s, va_list *args)
vl_api_fib_path_type_t type
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
@ IP6_ICMP_ERROR_NEXT_LOOKUP
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)