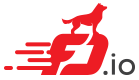 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
49 #define foreach_ethernet_input_next \
50 _ (PUNT, "error-punt") \
51 _ (DROP, "error-drop") \
52 _ (LLC, "llc-input") \
53 _ (IP4_INPUT, "ip4-input") \
54 _ (IP4_INPUT_NCS, "ip4-input-no-checksum")
58 #define _(s,n) ETHERNET_INPUT_NEXT_##s,
83 s =
format (s,
", hw-if-index %u, sw-if-index %u",
108 u16 * outer_id,
u16 * inner_id,
u32 * match_flags)
120 b0->
flags |= VNET_BUFFER_F_L2_HDR_OFFSET_VALID;
124 *
type = clib_net_to_host_u16 (e0->
type);
135 *
type = clib_net_to_host_u16 (e0[0]);
160 *outer_id = tag & 0xfff;
164 *
type = clib_net_to_host_u16 (h0->
type);
169 if (*
type == ETHERNET_TYPE_VLAN)
178 *inner_id = tag & 0xfff;
180 *
type = clib_net_to_host_u16 (h0->
type);
184 if (*
type == ETHERNET_TYPE_VLAN)
199 u64 * dmacs,
u8 * dmacs_bad,
214 u32 * new_sw_if_index,
u8 * error0,
u32 * is_l2)
220 qinq_intf, new_sw_if_index, error0, is_l2);
236 dmacs[0] = *(
u64 *) e0;
248 *error0 = ETHERNET_ERROR_L3_MAC_MISMATCH;
252 *error0 = (*new_sw_if_index) != ~0 ? (*error0) : ETHERNET_ERROR_DOWN;
263 b0->
flags |= VNET_BUFFER_F_L3_HDR_OFFSET_VALID;
268 *next0 = ETHERNET_INPUT_NEXT_DROP;
282 else if (type0 == ETHERNET_TYPE_IP4)
286 else if (type0 == ETHERNET_TYPE_IP6)
290 else if (type0 == ETHERNET_TYPE_MPLS)
316 *next0 = ETHERNET_INPUT_NEXT_LLC;
328 "l3_hdr_offset must follow l2_hdr_offset");
334 u32 flags = VNET_BUFFER_F_L2_HDR_OFFSET_VALID |
335 VNET_BUFFER_F_L3_HDR_OFFSET_VALID;
337 #ifdef CLIB_HAVE_VEC256
348 adv, -adv, 0, 0, adv, -adv, 0, 0,
349 adv, -adv, 0, 0, adv, -adv, 0, 0
436 u32 flags = VNET_BUFFER_F_L2_HDR_OFFSET_VALID |
437 VNET_BUFFER_F_L3_HDR_OFFSET_VALID;
456 #ifdef CLIB_HAVE_VEC128
457 u64x2 r = u64x2_load_unaligned (((
u8 *) & e->
type) - 6);
474 return (etype < 0x600) ? ETHERNET_INPUT_NEXT_LLC :
510 int main_is_l3,
int check_dmac)
522 u16 vlan1 = clib_net_to_host_u16 (t[0]) & 0xFFF;
523 u16 vlan2 = clib_net_to_host_u16 (t[2]) & 0xFFF;
524 u32 matched, is_l2, new_sw_if_index;
528 vif = &vlan_table->
vlans[vlan1];
530 qif = &qinq_table->
vlans[vlan2];
531 l->
err = ETHERNET_ERROR_NONE;
532 l->
type = clib_net_to_host_u16 (t[1]);
534 if (l->
type == ETHERNET_TYPE_VLAN)
536 l->
type = clib_net_to_host_u16 (t[3]);
540 qif, &new_sw_if_index, &l->
err,
548 new_sw_if_index =
hi->sw_if_index;
549 l->
err = ETHERNET_ERROR_NONE;
551 is_l2 = main_is_l3 == 0;
556 vif, qif, &new_sw_if_index,
569 clib_net_to_host_u64 (0xffffffffffffffff) :
570 clib_net_to_host_u64 (0xffffffff00000000);
573 l->
err = ETHERNET_ERROR_DOWN;
581 l->
adv = is_l2 ? 0 : l->
len;
584 l->
next = ETHERNET_INPUT_NEXT_DROP;
587 else if (l->
type == ETHERNET_TYPE_IP4)
589 else if (l->
type == ETHERNET_TYPE_IP6)
591 else if (l->
type == ETHERNET_TYPE_MPLS)
598 if (l->
next == ETHERNET_INPUT_NEXT_PUNT)
599 l->
err = ETHERNET_ERROR_UNKNOWN_TYPE;
603 if (check_dmac && l->
adv > 0 && dmac_bad)
605 l->
err = ETHERNET_ERROR_L3_MAC_MISMATCH;
606 next[0] = ETHERNET_INPUT_NEXT_PUNT;
615 if (l->
err == ETHERNET_ERROR_NONE)
628 #define DMAC_MASK clib_net_to_host_u64 (0xFFFFFFFFFFFF0000)
629 #define DMAC_IGBIT clib_net_to_host_u64 (0x0100000000000000)
631 #ifdef CLIB_HAVE_VEC256
633 is_dmac_bad_x4 (
u64 * dmacs,
u64 hwaddr)
635 u64x4 r0 = u64x4_load_unaligned (dmacs) & u64x4_splat (
DMAC_MASK);
636 r0 = (r0 != u64x4_splat (hwaddr)) & ((r0 & u64x4_splat (
DMAC_IGBIT)) == 0);
645 return (r0 != hwaddr) && ((r0 &
DMAC_IGBIT) == 0);
654 #ifdef CLIB_HAVE_VEC256
656 is_sec_dmac_bad_x4 (
u64 * dmacs,
u64 hwaddr)
658 u64x4 r0 = u64x4_load_unaligned (dmacs) & u64x4_splat (
DMAC_MASK);
659 r0 = (r0 != u64x4_splat (hwaddr));
674 #ifdef CLIB_HAVE_VEC256
675 *(
u32 *) (dmac_bad + 0) &= is_sec_dmac_bad_x4 (dmac + 0, hwaddr);
682 return *(
u32 *) dmac_bad;
693 u64 * dmacs,
u8 * dmacs_bad,
703 dmacs_bad[1] = ((n_packets > 1) &
is_dmac_bad (dmacs[1], hwaddr));
705 bad = dmacs_bad[0] | dmacs_bad[1];
714 hwaddr = sec_addr->
as_u64;
728 u64 * dmacs,
u8 * dmacs_bad,
734 u8 *dmac_bad = dmacs_bad;
740 #ifdef CLIB_HAVE_VEC256
743 bad |= *(
u32 *) (dmac_bad + 0) = is_dmac_bad_x4 (dmac + 0, hwaddr);
744 bad |= *(
u32 *) (dmac_bad + 4) = is_dmac_bad_x4 (dmac + 4, hwaddr);
754 bad |= dmac_bad[0] =
is_dmac_bad (dmac[0], hwaddr);
755 bad |= dmac_bad[1] =
is_dmac_bad (dmac[1], hwaddr);
756 bad |= dmac_bad[2] =
is_dmac_bad (dmac[2], hwaddr);
757 bad |= dmac_bad[3] =
is_dmac_bad (dmac[3], hwaddr);
766 if (have_sec_dmac && bad)
775 u8 *dmac_bad = dmacs_bad;
832 "VLIB_FRAME_SIZE must be power of 8");
836 u32 * buffer_indices,
u32 n_packets,
int main_is_l3,
837 int ip4_cksum_ok,
int dmac_check)
847 u16 next_ip4, next_ip6, next_mpls, next_l2;
848 u16 et_ip4 = clib_host_to_net_u16 (ETHERNET_TYPE_IP4);
849 u16 et_ip6 = clib_host_to_net_u16 (ETHERNET_TYPE_IP6);
850 u16 et_mpls = clib_host_to_net_u16 (ETHERNET_TYPE_MPLS);
851 u16 et_vlan = clib_host_to_net_u16 (ETHERNET_TYPE_VLAN);
852 u16 et_dot1ad = clib_host_to_net_u16 (ETHERNET_TYPE_DOT1AD);
932 if (next_ip4 == ETHERNET_INPUT_NEXT_IP4_INPUT && ip4_cksum_ok)
933 next_ip4 = ETHERNET_INPUT_NEXT_IP4_INPUT_NCS;
935 #ifdef CLIB_HAVE_VEC256
936 u16x16 et16_ip4 = u16x16_splat (et_ip4);
937 u16x16 et16_ip6 = u16x16_splat (et_ip6);
938 u16x16 et16_mpls = u16x16_splat (et_mpls);
939 u16x16 et16_vlan = u16x16_splat (et_vlan);
940 u16x16 et16_dot1ad = u16x16_splat (et_dot1ad);
941 u16x16 next16_ip4 = u16x16_splat (next_ip4);
942 u16x16 next16_ip6 = u16x16_splat (next_ip6);
943 u16x16 next16_mpls = u16x16_splat (next_mpls);
944 u16x16 next16_l2 = u16x16_splat (next_l2);
946 u16x16 stairs = { 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15 };
960 #ifdef CLIB_HAVE_VEC256
964 u16x16 e16 = u16x16_load_unaligned (etype);
967 r += (e16 == et16_ip4) & next16_ip4;
968 r += (e16 == et16_ip6) & next16_ip6;
969 r += (e16 == et16_mpls) & next16_mpls;
972 r = ((e16 != et16_vlan) & (e16 != et16_dot1ad)) & next16_l2;
973 u16x16_store_unaligned (
r,
next);
975 if (!u16x16_is_all_zero (
r == zero))
977 if (u16x16_is_all_zero (
r))
979 u16x16_store_unaligned (u16x16_splat (
i) + stairs,
980 slowpath_indices + n_slowpath);
985 for (
int j = 0; j < 16; j++)
987 slowpath_indices[n_slowpath++] =
i + j;
998 if (main_is_l3 && etype[0] == et_ip4)
1000 else if (main_is_l3 && etype[0] == et_ip6)
1002 else if (main_is_l3 && etype[0] == et_mpls)
1003 next[0] = next_mpls;
1004 else if (main_is_l3 == 0 &&
1005 etype[0] != et_vlan && etype[0] != et_dot1ad)
1010 slowpath_indices[n_slowpath++] =
i;
1023 u16 *
si = slowpath_indices;
1024 u32 last_unknown_etype = ~0;
1025 u32 last_unknown_next = ~0;
1028 .tag = tags[
si[0]] ^ -1LL,
1037 u16 etype = etypes[
i];
1039 if (etype == et_vlan)
1043 &dot1q_lookup, dmacs_bad[
i], 0,
1044 main_is_l3, dmac_check);
1047 else if (etype == et_dot1ad)
1051 &dot1ad_lookup, dmacs_bad[
i], 1,
1052 main_is_l3, dmac_check);
1057 if (last_unknown_etype != etype)
1059 last_unknown_etype = etype;
1060 etype = clib_host_to_net_u16 (etype);
1063 if (dmac_check && main_is_l3 && dmacs_bad[
i])
1066 b->
error =
node->errors[ETHERNET_ERROR_L3_MAC_MISMATCH];
1067 nexts[
i] = ETHERNET_INPUT_NEXT_PUNT;
1070 nexts[
i] = last_unknown_next;
1102 ((
hi->l2_if_count != 0) && (
hi->l3_if_count == 0)))
1105 1, ip4_cksum_ok, 0);
1110 1, ip4_cksum_ok, 1);
1116 if (
hi->l3_if_count == 0)
1119 0, ip4_cksum_ok, 0);
1124 0, ip4_cksum_ok, 1);
1146 if (b0->
flags & VLIB_BUFFER_IS_TRACED)
1193 u32 stats_sw_if_index, stats_n_packets, stats_n_bytes;
1195 u32 cached_sw_if_index = ~0;
1196 u32 cached_is_l2 = 0;
1210 stats_sw_if_index =
node->runtime_data[0];
1211 stats_n_packets = stats_n_bytes = 0;
1224 u8 next0, next1, error0, error1;
1225 u16 type0, orig_type0, type1, orig_type1;
1226 u16 outer_id0, inner_id0, outer_id1, inner_id1;
1227 u32 match_flags0, match_flags1;
1228 u32 old_sw_if_index0, new_sw_if_index0, len0, old_sw_if_index1,
1229 new_sw_if_index1, len1;
1254 n_left_to_next -= 2;
1261 error0 = error1 = ETHERNET_ERROR_NONE;
1263 type0 = clib_net_to_host_u16 (e0->
type);
1265 type1 = clib_net_to_host_u16 (e1->
type);
1270 b0->
flags |= VNET_BUFFER_F_L2_HDR_OFFSET_VALID;
1271 b1->
flags |= VNET_BUFFER_F_L2_HDR_OFFSET_VALID;
1280 u32 sw_if_index0, sw_if_index1;
1284 is_l20 = cached_is_l2;
1293 cached_sw_if_index = sw_if_index0;
1309 b0->
flags |= VNET_BUFFER_F_L3_HDR_OFFSET_VALID;
1310 b1->
flags |= VNET_BUFFER_F_L3_HDR_OFFSET_VALID;
1319 goto skip_dmac_check01;
1321 dmacs[0] = *(
u64 *) e0;
1322 dmacs[1] = *(
u64 *) e1;
1338 error0 = ETHERNET_ERROR_L3_MAC_MISMATCH;
1340 error1 = ETHERNET_ERROR_L3_MAC_MISMATCH;
1358 &orig_type0, &outer_id0, &inner_id0, &match_flags0);
1363 &orig_type1, &outer_id1, &inner_id1, &match_flags1);
1375 &main_intf0, &vlan_intf0, &qinq_intf0);
1384 &main_intf1, &vlan_intf1, &qinq_intf1);
1392 qinq_intf0, &new_sw_if_index0, &error0, &is_l20);
1400 qinq_intf1, &new_sw_if_index1, &error1, &is_l21);
1405 ETHERNET_ERROR_NONE ? old_sw_if_index0 : new_sw_if_index0;
1408 ETHERNET_ERROR_NONE ? old_sw_if_index1 : new_sw_if_index1;
1411 if (((new_sw_if_index0 != ~0)
1412 && (new_sw_if_index0 != old_sw_if_index0))
1413 || ((new_sw_if_index1 != ~0)
1414 && (new_sw_if_index1 != old_sw_if_index1)))
1422 stats_n_packets += 2;
1423 stats_n_bytes += len0 + len1;
1426 (!(new_sw_if_index0 == stats_sw_if_index
1427 && new_sw_if_index1 == stats_sw_if_index)))
1429 stats_n_packets -= 2;
1430 stats_n_bytes -= len0 + len1;
1432 if (new_sw_if_index0 != old_sw_if_index0
1433 && new_sw_if_index0 != ~0)
1435 interface_main.combined_sw_if_counters
1439 new_sw_if_index0, 1,
1441 if (new_sw_if_index1 != old_sw_if_index1
1442 && new_sw_if_index1 != ~0)
1444 interface_main.combined_sw_if_counters
1448 new_sw_if_index1, 1,
1451 if (new_sw_if_index0 == new_sw_if_index1)
1453 if (stats_n_packets > 0)
1460 stats_n_packets, stats_n_bytes);
1461 stats_n_packets = stats_n_bytes = 0;
1463 stats_sw_if_index = new_sw_if_index0;
1469 is_l20 = is_l21 = 0;
1482 n_left_to_next, bi0, bi1, next0,
1491 u16 type0, orig_type0;
1492 u16 outer_id0, inner_id0;
1494 u32 old_sw_if_index0, new_sw_if_index0, len0;
1516 n_left_to_next -= 1;
1521 error0 = ETHERNET_ERROR_NONE;
1523 type0 = clib_net_to_host_u16 (e0->
type);
1527 b0->
flags |= VNET_BUFFER_F_L2_HDR_OFFSET_VALID;
1538 is_l20 = cached_is_l2;
1542 cached_sw_if_index = sw_if_index0;
1556 b0->
flags |= VNET_BUFFER_F_L3_HDR_OFFSET_VALID;
1563 goto skip_dmac_check0;
1565 dmacs[0] = *(
u64 *) e0;
1581 error0 = ETHERNET_ERROR_L3_MAC_MISMATCH;
1595 &orig_type0, &outer_id0, &inner_id0, &match_flags0);
1606 &main_intf0, &vlan_intf0, &qinq_intf0);
1614 qinq_intf0, &new_sw_if_index0, &error0, &is_l20);
1619 ETHERNET_ERROR_NONE ? old_sw_if_index0 : new_sw_if_index0;
1630 if ((new_sw_if_index0 != ~0)
1631 && (new_sw_if_index0 != old_sw_if_index0))
1637 stats_n_packets += 1;
1638 stats_n_bytes += len0;
1644 stats_n_packets -= 1;
1645 stats_n_bytes -= len0;
1647 if (new_sw_if_index0 != ~0)
1652 if (stats_n_packets > 0)
1658 stats_sw_if_index, stats_n_packets, stats_n_bytes);
1659 stats_n_packets = stats_n_bytes = 0;
1661 stats_sw_if_index = new_sw_if_index0;
1676 to_next, n_left_to_next,
1684 if (stats_n_packets > 0)
1689 thread_index, stats_sw_if_index, stats_n_packets, stats_n_bytes);
1690 node->runtime_data[0] = stats_sw_if_index;
1777 u32 p2pe_sw_if_index =
1779 if (p2pe_sw_if_index == ~0)
1932 u32 placeholder_flags;
1933 u32 placeholder_unsup;
1939 &placeholder_unsup);
1957 #ifndef CLIB_MARCH_VARIANT
1963 u32 placeholder_flags;
1964 u32 placeholder_unsup;
1973 &placeholder_unsup);
2013 u32 placeholder_flags;
2014 u32 placeholder_unsup;
2019 &placeholder_unsup);
2054 u32 unsupported = 0;
2098 #define ethernet_error(n,c,s) s,
2100 #undef ethernet_error
2105 .name =
"ethernet-input",
2107 .vector_size =
sizeof (
u32),
2113 #define _(s,n) [ETHERNET_INPUT_NEXT_##s] = n,
2123 .name =
"ethernet-input-type",
2125 .vector_size =
sizeof (
u32),
2128 #define _(s,n) [ETHERNET_INPUT_NEXT_##s] = n,
2135 .name =
"ethernet-input-not-l2",
2137 .vector_size =
sizeof (
u32),
2140 #define _(s,n) [ETHERNET_INPUT_NEXT_##s] = n,
2147 #ifndef CLIB_MARCH_VARIANT
2171 ETHERNET_INPUT_NEXT_DROP);
2173 ETHERNET_INPUT_NEXT_PUNT);
2223 if (ethertype == ETHERNET_TYPE_IP4)
2227 else if (ethertype == ETHERNET_TYPE_IP6)
2231 else if (ethertype == ETHERNET_TYPE_MPLS)
2253 __attribute__ ((unused))
vlan_table_t *invalid_vlan_table;
2254 __attribute__ ((unused))
qinq_table_t *invalid_qinq_table;
void ethernet_sw_interface_set_l2_mode_noport(vnet_main_t *vnm, u32 sw_if_index, u32 l2)
vlan_intf_t vlans[ETHERNET_N_VLAN]
p2p_ethernet_main_t p2p_main
static_always_inline void eth_input_adv_and_flags_x1(vlib_buffer_t **b, int is_l3)
struct vnet_sub_interface_t::@374 eth
@ VNET_SW_INTERFACE_TYPE_PIPE
static u32 eth_identify_subint(vnet_hw_interface_t *hi, u32 match_flags, main_intf_t *main_intf, vlan_intf_t *vlan_intf, qinq_intf_t *qinq_intf, u32 *new_sw_if_index, u8 *error0, u32 *is_l2)
vnet_sw_interface_type_t type
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
subint_config_t * p2p_subif_pool
u8 next_by_ethertype_register_called
static_always_inline u8 is_dmac_bad(u64 dmac, u64 hwaddr)
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
#define vlib_prefetch_buffer_header(b, type)
Prefetch buffer metadata.
#define SUBINT_CONFIG_MATCH_3_TAG
struct vnet_sub_interface_t::@374::@375::@377 flags
static uword vlib_node_add_next(vlib_main_t *vm, uword node, uword next_node)
static_always_inline u8 eth_input_sec_dmac_check_x1(u64 hwaddr, u64 *dmac, u8 *dmac_bad)
void ethernet_register_l2_input(vlib_main_t *vm, u32 node_index)
nat44_ei_hairpin_src_next_t next_index
@ ETHERNET_INPUT_VARIANT_ETHERNET_TYPE
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
static clib_error_t * ethernet_sw_interface_add_del(vnet_main_t *vnm, u32 sw_if_index, u32 is_create)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
representation of a pipe interface
static_always_inline int ethernet_frame_is_tagged(u16 type)
void ethernet_register_l3_redirect(vlib_main_t *vm, u32 node_index)
static_always_inline void eth_input_get_etype_and_tags(vlib_buffer_t **b, u16 *etype, u64 *tags, u64 *dmacs, int offset, int dmac_check)
static_always_inline void identify_subint(ethernet_main_t *em, vnet_hw_interface_t *hi, vlib_buffer_t *b0, u32 match_flags, main_intf_t *main_intf, vlan_intf_t *vlan_intf, qinq_intf_t *qinq_intf, u32 *new_sw_if_index, u8 *error0, u32 *is_l2)
static_always_inline void determine_next_node(ethernet_main_t *em, ethernet_input_variant_t variant, u32 is_l20, u32 type0, vlib_buffer_t *b0, u8 *error0, u8 *next0)
static_always_inline int ethernet_frame_is_any_tagged_x2(u16 type0, u16 type1)
vlib_get_buffers(vm, from, b, n_left_from)
#define ETHERNET_INTERFACE_FLAG_STATUS_L3
static_always_inline void eth_input_adv_and_flags_x4(vlib_buffer_t **b, int is_l3)
static_always_inline void eth_input_single_int(vlib_main_t *vm, vlib_node_runtime_t *node, vnet_hw_interface_t *hi, u32 *from, u32 n_pkts, int ip4_cksum_ok)
vlib_main_t vlib_node_runtime_t * node
u8 * format_ethernet_header(u8 *s, va_list *args)
vnet_p2p_sub_interface_t p2p
void ethernet_setup_node(vlib_main_t *vm, u32 node_index)
@ VNET_SW_INTERFACE_TYPE_SUB
#define clib_error_return(e, args...)
subint_config_t subint
Sub-interface config.
#define vlib_call_init_function(vm, x)
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
vlib_node_registration_t ethernet_input_node
(constructor) VLIB_REGISTER_NODE (ethernet_input_node)
epu8_epi32 epu16_epi32 u64x2
vnet_hw_interface_class_t ethernet_hw_interface_class
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static_always_inline u8 is_sec_dmac_bad(u64 dmac, u64 hwaddr)
VNET_SW_INTERFACE_ADD_DEL_FUNCTION(ethernet_sw_interface_add_del)
qinq_intf_t vlans[ETHERNET_N_VLAN]
static vnet_sw_interface_t * vnet_get_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
vlib_main_t vlib_node_runtime_t vlib_frame_t * from_frame
vlib_buffer_enqueue_to_next(vm, node, from,(u16 *) nexts, frame->n_vectors)
#define SUBINT_CONFIG_P2P
#define clib_error_report(e)
static void pcap_add_buffer(pcap_main_t *pm, struct vlib_main_t *vm, u32 buffer_index, u32 n_bytes_in_trace)
Add buffer (vlib_buffer_t) to the trace.
#define u16x16_blend(v1, v2, mask)
vnet_hw_if_output_node_runtime_t * r
static clib_error_t * ethernet_init(vlib_main_t *vm)
unformat_function_t * unformat_buffer
#define SPARSE_VEC_INVALID_INDEX
#define SUBINT_CONFIG_MATCH_2_TAG
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
subint_config_t untagged_subint
pool_header_t * ph(void *p)
GDB callable function: ph - call pool_header - get pool header.
static_always_inline void eth_input_process_frame(vlib_main_t *vm, vlib_node_runtime_t *node, vnet_hw_interface_t *hi, u32 *buffer_indices, u32 n_packets, int main_is_l3, int ip4_cksum_ok, int dmac_check)
vlib_node_registration_t ethernet_input_not_l2_node
(constructor) VLIB_REGISTER_NODE (ethernet_input_not_l2_node)
clib_error_t * next_by_ethertype_init(next_by_ethertype_t *l3_next)
#define vec_elt(v, i)
Get vector value at index i.
subint_config_t inner_any_subint
subint_config_t single_tag_subint
#define CLIB_PREFETCH(addr, size, type)
static_always_inline void ethernet_input_trace(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *from_frame)
ethernet_interface_t * interfaces
#define STRUCT_OFFSET_OF(t, f)
i16 current_data
signed offset in data[], pre_data[] that we are currently processing.
vlib_error_t * errors
Vector of errors for this node.
STATIC_ASSERT_OFFSET_OF(vlib_buffer_t, current_data, 0)
void ethernet_set_rx_redirect(vnet_main_t *vnm, vnet_hw_interface_t *hi, u32 enable)
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
format_function_t * format_buffer
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
next_by_ethertype_t l3_next
vlib_error_t error
Error code for buffers to be enqueued to error handler.
static_always_inline u32 eth_input_sec_dmac_check_x4(u64 hwaddr, u64 *dmac, u8 *dmac_bad)
#define sparse_vec_validate(v, i)
#define VLIB_NODE_FN(node)
unformat_function_t * unformat_edit
static_always_inline void ethernet_input_inline_dmac_check(vnet_hw_interface_t *hi, u64 *dmacs, u8 *dmacs_bad, u32 n_packets, ethernet_interface_t *ei, u8 have_sec_dmac)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
vnet_main_t * vnet_get_main(void)
#define VLIB_NODE_FLAG_TRACE
struct clib_bihash_value offset
template key/value backing page structure
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
#define static_always_inline
#define ethernet_buffer_header_size(b)
Determine the size of the Ethernet headers of the current frame in the buffer.
static clib_error_t * ethernet_sw_interface_up_down(vnet_main_t *vnm, u32 sw_if_index, u32 flags)
static pg_node_t * pg_get_node(uword node_index)
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
static_always_inline void parse_header(ethernet_input_variant_t variant, vlib_buffer_t *b0, u16 *type, u16 *orig_type, u16 *outer_id, u16 *inner_id, u32 *match_flags)
static uword sparse_vec_index(void *v, uword sparse_index)
static vlib_node_t * vlib_get_node(vlib_main_t *vm, u32 i)
Get vlib node by index.
static u8 * format_ethernet_input_trace(u8 *s, va_list *va)
_mm256_packus_epi16 _mm256_packus_epi32 static_always_inline u32 u8x32_msb_mask(u8x32 v)
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
STATIC_ASSERT(STRUCT_OFFSET_OF(vnet_buffer_opaque_t, l2_hdr_offset)==STRUCT_OFFSET_OF(vnet_buffer_opaque_t, l3_hdr_offset) - 2, "l3_hdr_offset must follow l2_hdr_offset")
subint_config_t default_subint
@ VNET_INTERFACE_COUNTER_RX
struct _vlib_node_registration vlib_node_registration_t
vlib_combined_counter_main_t * combined_sw_if_counters
static_always_inline u64x4 u64x4_gather(void *p0, void *p1, void *p2, void *p3)
static void * vlib_frame_scalar_args(vlib_frame_t *f)
Get pointer to frame scalar data.
ethernet_interface_t * ethernet_get_interface(ethernet_main_t *em, u32 hw_if_index)
clib_error_t * next_by_ethertype_register(next_by_ethertype_t *l3_next, u32 ethertype, u32 next_index)
u32 * sparse_index_by_input_next_index
void ethernet_sw_interface_set_l2_mode(vnet_main_t *vnm, u32 sw_if_index, u32 l2)
#define vlib_validate_buffer_enqueue_x1(vm, node, next_index, to_next, n_left_to_next, bi0, next0)
Finish enqueueing one buffer forward in the graph.
template key/value backing page structure
void l2bvi_register_input_type(vlib_main_t *vm, ethernet_type_t type, u32 node_index)
@ ETHERNET_INPUT_VARIANT_NOT_L2
uword unformat_ethernet_header(unformat_input_t *input, va_list *args)
@ ETHERNET_INPUT_VARIANT_ETHERNET
ethernet_main_t ethernet_main
description fragment has unexpected format
u32 p2p_ethernet_lookup(u32 parent_if_index, u8 *client_mac)
static_always_inline void eth_input_process_frame_dmac_check(vnet_hw_interface_t *hi, u64 *dmacs, u8 *dmacs_bad, u32 n_packets, ethernet_interface_t *ei, u8 have_sec_dmac)
vlib_put_next_frame(vm, node, next_index, 0)
epu16_epi64 epu8_epi16 epu8_epi64 epi16_epi64 i16x16
static_always_inline int vnet_is_packet_pcaped(vnet_pcap_t *pp, vlib_buffer_t *b, u32 sw_if_index)
vnet_is_packet_pcaped
static vnet_hw_interface_t * vnet_get_sup_hw_interface(vnet_main_t *vnm, u32 sw_if_index)
static subint_config_t * ethernet_sw_interface_get_config(vnet_main_t *vnm, u32 sw_if_index, u32 *flags, u32 *unsupported)
u8 * format_ethernet_header_with_length(u8 *s, va_list *args)
#define SUBINT_CONFIG_VALID
static_always_inline void clib_prefetch_load(void *p)
void ethernet_register_input_type(vlib_main_t *vm, ethernet_type_t type, u32 node_index)
#define foreach_ethernet_input_next
#define vec_foreach(var, vec)
Vector iterator.
#define ETH_INPUT_FRAME_F_IP4_CKSUM_OK
#define ethernet_buffer_set_vlan_count(b, v)
Sets the number of VLAN headers in the current Ethernet frame in the buffer.
uword unformat_pg_ethernet_header(unformat_input_t *input, va_list *args)
#define ETH_INPUT_FRAME_F_SINGLE_SW_IF_IDX
static vlib_node_runtime_t * vlib_node_get_runtime(vlib_main_t *vm, u32 node_index)
Get node runtime by node index.
int vnet_hw_interface_rx_redirect_to_node(vnet_main_t *vnm, u32 hw_if_index, u32 node_index)
static ethernet_type_info_t * ethernet_get_type_info(ethernet_main_t *em, ethernet_type_t type)
static_always_inline void ethernet_input_inline(vlib_main_t *vm, vlib_node_runtime_t *node, u32 *from, u32 n_packets, ethernet_input_variant_t variant)
static_always_inline void eth_input_update_if_counters(vlib_main_t *vm, vnet_main_t *vnm, eth_input_tag_lookup_t *l)
static_always_inline void u32x8_scatter_one(u32x8 r, int index, void *p)
static_always_inline u16 eth_input_next_by_type(u16 etype)
VNET_SW_INTERFACE_ADMIN_UP_DOWN_FUNCTION(bond_sw_interface_up_down)
#define SUBINT_CONFIG_MATCH_1_TAG
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
pipe_t * pipe_get(u32 sw_if_index)
Get the pipe instnace based on one end.
#define SUBINT_CONFIG_MATCH_0_TAG
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
@ VNET_SW_INTERFACE_TYPE_P2P
static char * ethernet_error_strings[]
#define clib_warning(format, args...)
#define pool_alloc(P, N)
Allocate N more free elements to pool (unspecified alignment).
u16 nexts[VLIB_FRAME_SIZE]
#define vlib_validate_buffer_enqueue_x2(vm, node, next_index, to_next, n_left_to_next, bi0, bi1, next0, next1)
Finish enqueueing two buffers forward in the graph.
vl_api_interface_index_t sw_if_index
ethernet_interface_address_t * secondary_addrs
#define vlib_get_next_frame(vm, node, next_index, vectors, n_vectors_left)
Get pointer to next frame vector data by (vlib_node_runtime_t, next_index).
ethernet_interface_address_t address
void ethernet_input_init(vlib_main_t *vm, ethernet_main_t *em)
vl_api_fib_path_type_t type
vnet_interface_main_t interface_main
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
#define vlib_prefetch_buffer_data(b, type)
_mm256_packus_epi16 u16x16
static void eth_vlan_table_lookups(ethernet_main_t *em, vnet_main_t *vnm, u32 port_sw_if_index0, u16 first_ethertype, u16 outer_id, u16 inner_id, vnet_hw_interface_t **hi, main_intf_t **main_intf, vlan_intf_t **vlan_intf, qinq_intf_t **qinq_intf)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
static_always_inline void u64x4_scatter(u64x4 r, void *p0, void *p1, void *p2, void *p3)
static void * sparse_vec_new(uword elt_bytes, uword sparse_index_bits)
vlib_node_registration_t ethernet_input_type_node
(constructor) VLIB_REGISTER_NODE (ethernet_input_type_node)
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)
vl_api_wireguard_peer_flags_t flags
static_always_inline void eth_input_tag_lookup(vlib_main_t *vm, vnet_main_t *vnm, vlib_node_runtime_t *node, vnet_hw_interface_t *hi, u64 tag, u16 *next, vlib_buffer_t *b, eth_input_tag_lookup_t *l, u8 dmac_bad, int is_dot1ad, int main_is_l3, int check_dmac)