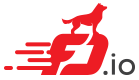 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
21 #include <sys/ioctl.h>
22 #include <sys/socket.h>
25 #include <sys/types.h>
27 #include <netinet/in.h>
30 #include <linux/if_arp.h>
31 #include <linux/if_tun.h>
86 ssize_t map_sz = (vui->
regions[
i].memory_size +
88 page_sz - 1) & ~(page_sz - 1);
94 vu_log_debug (vui,
"unmap memory region %d addr 0x%lx len 0x%lx "
102 vu_log_err (vui,
"failed to unmap memory region (errno %d)",
124 u32 q = qid >> 1, rxvq_count;
130 rxvq_count = (qid >> 1) + 1;
197 vu_log_warn (vui,
"unable to set rx mode for interface %d, "
206 int i, found[2] = { };
212 return found[0] && found[1];
223 is_ready ?
"ready" :
"down");
235 __attribute__ ((unused))
int n;
258 __attribute__ ((unused)) ssize_t n;
264 u32 is_txq = qid & 1;
319 if (qid == 0 || qid == 1)
342 if (vring->
errfd != -1)
344 close (vring->
errfd);
393 txvq->
used_event->flags = VRING_EVENT_F_DISABLE;
414 int fd, number_of_fds = 0;
416 vhost_user_msg_t msg;
421 struct cmsghdr *cmsg;
438 iov[0].iov_base = (
void *) &msg;
443 mh.msg_control = control;
444 mh.msg_controllen =
sizeof (control);
452 vu_log_debug (vui,
"recvmsg returned error %d %s", errno,
457 vu_log_debug (vui,
"n (%d) != VHOST_USER_MSG_HDR_SZ (%d)",
463 if (mh.msg_flags & MSG_CTRUNC)
469 cmsg = CMSG_FIRSTHDR (&mh);
471 if (cmsg && (cmsg->cmsg_len > 0) && (cmsg->cmsg_level == SOL_SOCKET) &&
472 (cmsg->cmsg_type == SCM_RIGHTS) &&
473 (cmsg->cmsg_len - CMSG_LEN (0) <=
476 number_of_fds = (cmsg->cmsg_len - CMSG_LEN (0)) /
sizeof (
int);
481 if ((msg.flags & 7) != 1)
483 vu_log_debug (vui,
"malformed message received. closing socket");
497 else if (
rv != msg.size)
499 vu_log_debug (vui,
"message too short (read %dB should be %dB)",
rv,
527 msg.size =
sizeof (msg.u64);
528 vu_log_debug (vui,
"if %d msg VHOST_USER_GET_FEATURES - reply "
540 vu_log_debug (vui,
"if %d msg VHOST_USER_SET_FEATURES features "
576 vu_log_debug (vui,
"if %d msg VHOST_USER_SET_MEM_TABLE nregions %d",
579 if ((msg.memory.nregions < 1) ||
582 vu_log_debug (vui,
"number of mem regions must be between 1 and %i",
587 if (msg.memory.nregions != number_of_fds)
595 for (
i = 0;
i < msg.memory.nregions;
i++)
600 ssize_t map_sz = (msg.memory.regions[
i].memory_size +
601 msg.memory.regions[
i].mmap_offset +
602 page_sz - 1) & ~(page_sz - 1);
604 region_mmap_addr[
i] = mmap (0, map_sz, PROT_READ | PROT_WRITE,
605 MAP_SHARED, fds[
i], 0);
606 if (region_mmap_addr[
i] == MAP_FAILED)
608 vu_log_err (vui,
"failed to map memory. errno is %d", errno);
609 for (j = 0; j <
i; j++)
610 munmap (region_mmap_addr[j], map_sz);
613 vu_log_debug (vui,
"map memory region %d addr 0 len 0x%lx fd %d "
614 "mapped 0x%lx page_sz 0x%x",
i, map_sz, fds[
i],
615 region_mmap_addr[
i], page_sz);
620 for (
i = 0;
i < msg.memory.nregions;
i++)
623 sizeof (vhost_user_memory_region_t));
657 vu_log_debug (vui,
"if %d msg VHOST_USER_SET_VRING_NUM idx %d num %d",
660 if ((msg.state.num > 32768) ||
661 (msg.state.num == 0) ||
662 ((msg.state.num - 1) & msg.state.num) ||
663 (msg.state.index >= vui->
num_qid))
665 vu_log_debug (vui,
"invalid VHOST_USER_SET_VRING_NUM: msg.state.num"
666 " %d, msg.state.index %d, curruent max q %d",
667 msg.state.num, msg.state.index, vui->
num_qid);
674 vu_log_debug (vui,
"if %d msg VHOST_USER_SET_VRING_ADDR idx %d",
677 if (msg.state.index >= vui->
num_qid)
679 vu_log_debug (vui,
"invalid vring index VHOST_USER_SET_VRING_ADDR:"
680 " %u >= %u", msg.state.index, vui->
num_qid);
684 if (msg.size < sizeof (msg.addr))
686 vu_log_debug (vui,
"vhost message is too short (%d < %d)",
687 msg.size, sizeof (msg.addr));
695 if ((desc == NULL) || (used == NULL) || (avail == NULL))
697 vu_log_debug (vui,
"failed to map user memory for hw_if_index %d",
742 vu_log_debug (vui,
"if %d msg VHOST_USER_SET_VRING_CALL %d",
745 q = (
u8) (msg.u64 & 0xFF);
760 vui->
num_qid = (q & 1) ? (q + 1) : (q + 2);
788 vui->
num_qid = (q & 1) ? (q + 1) : (q + 2);
793 if (number_of_fds != 1)
800 template.file_descriptor = fds[0];
801 template.private_data =
803 template.description =
format (0,
"vhost user");
811 vu_log_debug (vui,
"if %d msg VHOST_USER_SET_VRING_KICK %d",
814 q = (
u8) (msg.u64 & 0xFF);
817 vu_log_debug (vui,
"invalid vring index VHOST_USER_SET_VRING_KICK:"
832 if (number_of_fds != 1)
839 template.file_descriptor = fds[0];
840 template.private_data =
856 vu_log_debug (vui,
"if %d msg VHOST_USER_SET_VRING_ERR %d",
859 q = (
u8) (msg.u64 & 0xFF);
862 vu_log_debug (vui,
"invalid vring index VHOST_USER_SET_VRING_ERR:"
872 if (number_of_fds != 1)
883 "if %d msg VHOST_USER_SET_VRING_BASE idx %d num 0x%x",
885 if (msg.state.index >= vui->
num_qid)
887 vu_log_debug (vui,
"invalid vring index VHOST_USER_SET_VRING_ADDR:"
888 " %u >= %u", msg.state.index, vui->
num_qid);
907 msg.state.num & 0x7fff;
910 ! !(msg.state.num & (1 << 15));
935 if (msg.state.index >= vui->
num_qid)
937 vu_log_debug (vui,
"invalid vring index VHOST_USER_GET_VRING_BASE:"
938 " %u >= %u", msg.state.index, vui->
num_qid);
959 msg.size =
sizeof (msg.state);
968 "if %d msg VHOST_USER_GET_VRING_BASE idx %d num 0x%x",
988 if (msg.size != sizeof (msg.log))
990 vu_log_debug (vui,
"invalid msg size for VHOST_USER_SET_LOG_BASE:"
991 " %d instead of %d", msg.size, sizeof (msg.log));
997 vu_log_debug (vui,
"VHOST_USER_PROTOCOL_F_LOG_SHMFD not set but "
998 "VHOST_USER_SET_LOG_BASE received");
1006 (msg.log.size + msg.log.offset + page_sz - 1) & ~(page_sz - 1);
1008 void *log_base_addr = mmap (0, map_sz, PROT_READ | PROT_WRITE,
1011 vu_log_debug (vui,
"map log region addr 0 len 0x%lx off 0x%lx fd %d "
1012 "mapped 0x%lx", map_sz, msg.log.offset, fd,
1015 if (log_base_addr == MAP_FAILED)
1017 vu_log_err (vui,
"failed to map memory. errno is %d", errno);
1028 msg.size =
sizeof (msg.u64);
1046 msg.size =
sizeof (msg.u64);
1047 vu_log_debug (vui,
"if %d msg VHOST_USER_GET_PROTOCOL_FEATURES - "
1059 vu_log_debug (vui,
"if %d msg VHOST_USER_SET_PROTOCOL_FEATURES "
1067 msg.size =
sizeof (msg.u64);
1068 vu_log_debug (vui,
"if %d msg VHOST_USER_GET_QUEUE_NUM - reply %d",
1080 vu_log_debug (vui,
"if %d VHOST_USER_SET_VRING_ENABLE: %s queue %d",
1081 vui->
hw_if_index, msg.state.num ?
"enable" :
"disable",
1083 if (msg.state.index >= vui->
num_qid)
1085 vu_log_debug (vui,
"invalid vring idx VHOST_USER_SET_VRING_ENABLE:"
1086 " %u >= %u", msg.state.index, vui->
num_qid);
1096 vu_log_debug (vui,
"unknown vhost-user message %d received. "
1097 "closing socket", msg.request);
1129 int client_fd, client_len;
1130 struct sockaddr_un client;
1137 client_len =
sizeof (client);
1139 (
struct sockaddr *) &client,
1140 (socklen_t *) & client_len);
1147 vu_log_debug (vui,
"Close client socket for vhost interface %d, fd %d",
1152 vu_log_debug (vui,
"New client socket for vhost interface %d, fd %d",
1156 template.file_descriptor = client_fd;
1204 f64 timeout = 3153600000.0 ;
1205 uword event_type, *event_data = 0;
1208 f64 now, poll_time_remaining;
1214 poll_time_remaining =
1223 timeout = poll_time_remaining;
1245 next_timeout = timeout;
1260 if ((next_timeout < timeout) && (next_timeout > 0.0))
1261 timeout = next_timeout;
1268 clib_warning (
"BUG: unhandled event type %d", event_type);
1275 timeout = 3153600000.0;
1284 .name =
"vhost-user-send-interrupt-process",
1294 struct sockaddr_un sun;
1297 f64 timeout = 3153600000.0 ;
1298 uword *event_data = 0;
1301 sun.sun_family = AF_UNIX;
1320 ((sockfd = socket (AF_UNIX, SOCK_STREAM, 0)) < 0))
1329 (
"Error: Could not open unix socket for %s",
1338 sizeof (sun.sun_path) - 1);
1339 sun.sun_path[
sizeof (sun.sun_path) - 1] = 0;
1342 if (fcntl(sockfd, F_SETFL, O_NONBLOCK) < 0)
1345 if (connect (sockfd, (
struct sockaddr *) &sun,
1346 sizeof (
struct sockaddr_un)) == 0)
1349 if (fcntl(sockfd, F_SETFL, 0) < 0)
1353 template.file_descriptor = sockfd;
1354 template.private_data =
1356 template.description =
format (0,
"vhost user process");
1375 getsockopt (fd, SOL_SOCKET, SO_ERROR, &
error, &
len);
1394 .name =
"vhost-user-process",
1445 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
1449 vu_log_debug (vui,
"Deleting vhost-user interface %s (instance %d)",
1526 struct sockaddr_un
un = { };
1529 if ((fd = socket (AF_UNIX, SOCK_STREAM, 0)) < 0)
1530 return VNET_API_ERROR_SYSCALL_ERROR_1;
1532 un.sun_family = AF_UNIX;
1533 strncpy ((
char *)
un.sun_path, (
char *) sock_filename,
1534 sizeof (
un.sun_path) - 1);
1537 unlink ((
char *) sock_filename);
1539 if (bind (fd, (
struct sockaddr *) &
un,
sizeof (
un)) == -1)
1541 rv = VNET_API_ERROR_SYSCALL_ERROR_2;
1545 if (listen (fd, 1) == -1)
1547 rv = VNET_API_ERROR_SYSCALL_ERROR_3;
1610 if (server_sock_fd != -1)
1614 template.file_descriptor = server_sock_fd;
1616 template.description =
format (0,
"vhost user %d", sw);
1675 int server_sock_fd = -1;
1681 return VNET_API_ERROR_INVALID_ARGUMENT;
1690 return VNET_API_ERROR_IF_ALREADY_EXISTS;
1697 &server_sock_fd)) != 0)
1731 int server_sock_fd = -1;
1739 return VNET_API_ERROR_INVALID_SW_IF_INDEX;
1742 return VNET_API_ERROR_INVALID_ARGUMENT;
1752 if (if_index && (*if_index != vui->
if_index))
1753 return VNET_API_ERROR_IF_ALREADY_EXISTS;
1758 &server_sock_fd)) != 0)
1801 else if (
unformat (line_input,
"server"))
1803 else if (
unformat (line_input,
"gso"))
1805 else if (
unformat (line_input,
"packed"))
1807 else if (
unformat (line_input,
"event-idx"))
1809 else if (
unformat (line_input,
"feature-mask 0x%llx",
1815 else if (
unformat (line_input,
"renumber %d",
1898 u32 *hw_if_indices = 0;
1908 for (
i = 0;
i <
vec_len (hw_if_indices);
i++)
1929 *out_vuids = r_vuids;
1937 char *
fmt = va_arg (*args,
char *);
1940 int idx = va_arg (*args,
int);
1941 u32 *mem_hint = va_arg (*args,
u32 *);
1944 desc_table[idx].
flags, desc_table[idx].
next,
1962 int show_descr,
int show_verbose)
1973 " avail.flags %x avail event idx %u avail.idx %d "
1974 "used.flags %x used event idx %u used.idx %d\n",
1985 " slot addr len flags next "
1988 " ===== ================== ===== ====== ===== "
1989 "==================\n");
1990 for (j = 0; j < vq->
qsz_mask + 1; j++)
1992 desc_table = vq->
desc;
1994 " %-5d 0x%016lx %-5d 0x%04x %-5d 0x%016lx\n", vui,
1995 desc_table, j, &mem_hint);
2002 for (idx = 0; idx <
clib_min (20, n_entries); idx++)
2006 "> %-4u 0x%016lx %-5u 0x%04x %-5u 0x%016lx\n", vui,
2007 desc_table, idx, &mem_hint);
2009 if (n_entries >= 20)
2021 char *
fmt = va_arg (*args,
char *);
2023 vring_packed_desc_t *desc_table = va_arg (*args, vring_packed_desc_t *);
2024 int idx = va_arg (*args,
int);
2025 u32 *mem_hint = va_arg (*args,
u32 *);
2028 desc_table[idx].
flags, desc_table[idx].
id,
2043 static event_idx_flags event_idx_array[] = {
2044 #define _(s,v) { .str = #s, .value = v, },
2048 u32 num_entries =
sizeof (event_idx_array) /
sizeof (event_idx_flags);
2050 if (
flags < num_entries)
2059 int show_descr,
int show_verbose)
2065 vring_packed_desc_t *desc_table;
2090 " slot addr len flags id "
2093 " ===== ================== ===== ====== ===== "
2094 "==================\n");
2095 for (j = 0; j < vq->
qsz_mask + 1; j++)
2099 " %-5u 0x%016lx %-5u 0x%04x %-5u 0x%016lx\n", vui,
2100 desc_table, j, &mem_hint);
2103 n_entries = desc_table[j].len >> 4;
2107 for (idx = 0; idx <
clib_min (20, n_entries); idx++)
2111 "> %-4u 0x%016lx %-5u 0x%04x %-5u 0x%016lx\n", vui,
2112 desc_table, idx, &mem_hint);
2114 if (n_entries >= 20)
2132 u32 hw_if_index, *hw_if_indices = 0;
2137 int show_verbose = 0;
2143 struct feat_struct *feat_entry;
2145 static struct feat_struct feat_array[] = {
2146 #define _(s,b) { .str = #s, .bit = b, },
2152 #define foreach_protocol_feature \
2153 _(VHOST_USER_PROTOCOL_F_MQ) \
2154 _(VHOST_USER_PROTOCOL_F_LOG_SHMFD)
2156 static struct feat_struct proto_feat_array[] = {
2157 #define _(s) { .str = #s, .bit = s},
2175 vec_add1 (hw_if_indices, hw_if_index);
2179 else if (
unformat (input,
"verbose"))
2188 if (
vec_len (hw_if_indices) == 0)
2206 for (
i = 0;
i <
vec_len (hw_if_indices);
i++)
2222 " features mask (0x%llx): \n"
2223 " features (0x%llx): \n",
2227 feat_entry = (
struct feat_struct *) &feat_array;
2228 while (feat_entry->str)
2230 if (vui->
features & (1ULL << feat_entry->bit))
2238 feat_entry = (
struct feat_struct *) &proto_feat_array;
2239 while (feat_entry->str)
2260 if (txvq->
qid == -1)
2290 " region fd guest_phys_addr memory_size userspace_addr mmap_offset mmap_addr\n");
2292 " ====== ===== ================== ================== ================== ================== ==================\n");
2294 for (j = 0; j < vui->
nregions; j++)
2297 " %d %-5d 0x%016lx 0x%016lx 0x%016lx 0x%016lx 0x%016lx\n",
2299 vui->
regions[j].guest_phys_addr,
2301 vui->
regions[j].userspace_addr,
2311 (q & 1) ?
"RX" :
"TX",
2318 " qsz %d last_avail_idx %d last_used_idx %d"
2395 .path =
"create vhost-user",
2396 .short_help =
"create vhost-user socket <socket-filename> [server] "
2397 "[feature-mask <hex>] [hwaddr <mac-addr>] [renumber <dev_instance>] [gso] "
2398 "[packed] [event-idx]",
2418 .path =
"delete vhost-user",
2419 .short_help =
"delete vhost-user {<interface> | sw_if_index <sw_idx>}",
2558 .path =
"show vhost-user",
2559 .short_help =
"show vhost-user [<interface> [<interface> [..]]] "
2560 "[[descriptors] [verbose]]",
2577 else if (
unformat (input,
"dont-dump-memory"))
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
static void clib_spinlock_init(clib_spinlock_t *p)
static_always_inline void vhost_user_update_gso_interface_count(vhost_user_intf_t *vui, u8 add)
#define VNET_HW_IF_RXQ_THREAD_ANY
static void vhost_user_term_if(vhost_user_intf_t *vui)
Disables and reset interface structure.
#define VHOST_USER_MSG_HDR_SZ
static_always_inline void vhost_user_send_call(vlib_main_t *vm, vhost_user_intf_t *vui, vhost_user_vring_t *vq)
static u32 vlib_num_workers()
uword unformat_ethernet_address(unformat_input_t *input, va_list *args)
clib_file_main_t file_main
void vlib_worker_thread_barrier_release(vlib_main_t *vm)
u64 region_guest_addr_lo[VHOST_MEMORY_MAX_NREGIONS]
static u8 * format_vhost_user_packed_desc(u8 *s, va_list *args)
@ VNET_HW_IF_RX_MODE_ADAPTIVE
void vnet_sw_interface_set_mtu(vnet_main_t *vnm, u32 sw_if_index, u32 mtu)
static void vhost_user_vui_init(vnet_main_t *vnm, vhost_user_intf_t *vui, int server_sock_fd, vhost_user_create_if_args_t *args, u32 *sw_if_index)
@ VHOST_USER_SET_VRING_KICK
#define VHOST_VRING_F_LOG
#define VNET_HW_INTERFACE_CAP_SUPPORTS_L4_TX_CKSUM
vnet_hw_interface_capabilities_t caps
#define clib_memcpy(d, s, n)
@ foreach_virtio_net_features
int vnet_interface_name_renumber(u32 sw_if_index, u32 new_show_dev_instance)
static_always_inline void vhost_user_thread_placement(vhost_user_intf_t *vui, u32 qid)
int vhost_user_dump_ifs(vnet_main_t *vnm, vlib_main_t *vm, vhost_user_intf_details_t **out_vuids)
#define VHOST_MEMORY_MAX_NREGIONS
int vnet_hw_if_set_rx_queue_mode(vnet_main_t *vnm, u32 queue_index, vnet_hw_if_rx_mode mode)
static uword pointer_to_uword(const void *p)
static void vhost_user_show_fds(vlib_main_t *vm, vhost_user_vring_t *vq)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
static void vhost_user_show_desc_packed(vlib_main_t *vm, vhost_user_intf_t *vui, int q, int show_descr, int show_verbose)
static_always_inline u16 vhost_user_avail_event_idx(vhost_user_vring_t *vq)
vnet_device_class_t vhost_user_device_class
static_always_inline void vhost_user_tx_thread_placement(vhost_user_intf_t *vui, u32 qid)
static clib_error_t * vhost_user_socket_error(clib_file_t *uf)
__clib_export errno_t memcpy_s(void *__restrict__ dest, rsize_t dmax, const void *__restrict__ src, rsize_t n)
copy src to dest, at most n bytes, up to dmax
vlib_log_class_t vlib_log_register_class(char *class, char *subclass)
static void stop_timer(wg_peer_t *peer, u32 timer_id)
static vlib_node_registration_t vhost_user_process_node
(constructor) VLIB_REGISTER_NODE (vhost_user_process_node)
#define clib_error_return(e, args...)
clib_file_function_t * read_function
void vnet_hw_if_tx_queue_assign_thread(vnet_main_t *vnm, u32 queue_index, u32 thread_index)
vl_api_tunnel_mode_t mode
static clib_error_t * vhost_user_callfd_read_ready(clib_file_t *uf)
#define pool_put(P, E)
Free an object E in pool P.
static uword * mhash_get(mhash_t *h, const void *key)
@ VNET_HW_IF_RX_MODE_POLLING
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static clib_error_t * vhost_user_config(vlib_main_t *vm, unformat_input_t *input)
#define VIRTIO_FEATURE(X)
@ VNET_HW_INTERFACE_FLAG_LINK_UP
VNET_HW_INTERFACE_CLASS(vhost_interface_class, static)
#define clib_error_report(e)
vnet_hw_if_output_node_runtime_t * r
vring_desc_event_t * avail_event
void vnet_hw_if_tx_queue_unassign_thread(vnet_main_t *vnm, u32 queue_index, u32 thread_index)
static void vlib_process_signal_event(vlib_main_t *vm, uword node_index, uword type_opaque, uword data)
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
static void mhash_init_c_string(mhash_t *h, uword n_value_bytes)
static clib_error_t * vhost_user_socksvr_accept_ready(clib_file_t *uf)
vlib_node_registration_t vhost_user_input_node
(constructor) VLIB_REGISTER_NODE (vhost_user_input_node)
#define clib_unix_warning(format, args...)
@ VHOST_USER_SET_VRING_NUM
__clib_export u8 * format_bitmap_list(u8 *s, va_list *args)
Format a bitmap as a list.
@ VHOST_USER_GET_PROTOCOL_FEATURES
@ VHOST_USER_SET_LOG_BASE
#define FOR_ALL_VHOST_TXQ(qid, vui)
static u32 random_u32(u32 *seed)
32-bit random number generator
#define VHOST_VRING_IDX_RX(qid)
#define VHOST_USER_VRING_NOFD_MASK
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_TCP_CKSUM
#define VLIB_CONFIG_FUNCTION(x, n,...)
#define FOR_ALL_VHOST_RX_TXQ(qid, vui)
static void vhost_user_show_desc(vlib_main_t *vm, vhost_user_intf_t *vui, int q, int show_descr, int show_verbose)
vhost_user_memory_region_t regions[VHOST_MEMORY_MAX_NREGIONS]
@ VHOST_USER_SET_PROTOCOL_FEATURES
static uword vlib_process_get_events(vlib_main_t *vm, uword **data_vector)
Return the first event type which has occurred and a vector of per-event data of that type,...
static_always_inline u64 vhost_user_is_packed_ring_supported(vhost_user_intf_t *vui)
void vhost_user_unmap_all(void)
#define pool_foreach(VAR, POOL)
Iterate through pool.
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
format_function_t format_vnet_hw_if_rx_mode
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
#define VHOST_USER_EVENT_STOP_TIMER
#define VRING_DESC_F_AVAIL
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
vnet_main_t * vnet_get_main(void)
#define FEATURE_VIRTIO_NET_F_HOST_GUEST_TSO_FEATURE_BITS
#define FOR_ALL_VHOST_RXQ(qid, vui)
#define vlib_worker_thread_barrier_sync(X)
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TCP_GSO
#define vec_validate_aligned(V, I, A)
Make sure vector is long enough for given index (no header, specified alignment)
static vnet_hw_interface_t * vnet_get_sup_hw_interface_api_visible_or_null(vnet_main_t *vnm, u32 sw_if_index)
#define foreach_protocol_feature
#define static_always_inline
clib_error_t * vhost_user_connect_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
clib_error_t * show_vhost_user_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define VRING_DESC_F_INDIRECT
#define VHOST_USER_PROTOCOL_F_LOG_SHMFD
static vlib_cli_command_t vhost_user_connect_command
(constructor) VLIB_CLI_COMMAND (vhost_user_connect_command)
#define vu_log_err(dev, f,...)
#define vu_log_warn(dev, f,...)
format_function_t format_vnet_hw_if_index_name
static_always_inline void * map_user_mem(vhost_user_intf_t *vui, uword addr)
void vnet_hw_if_update_runtime_data(vnet_main_t *vnm, u32 hw_if_index)
static vlib_cli_command_t show_vhost_user_command
(constructor) VLIB_CLI_COMMAND (show_vhost_user_command)
static_always_inline u16 vhost_user_used_event_idx(vhost_user_vring_t *vq)
static_always_inline void vhost_user_update_iface_state(vhost_user_intf_t *vui)
@ VNET_HW_IF_RX_MODE_INTERRUPT
vring_packed_desc_t * packed_desc
static_always_inline vnet_hw_if_tx_queue_t * vnet_hw_if_get_tx_queue(vnet_main_t *vnm, u32 queue_index)
static void clib_spinlock_free(clib_spinlock_t *p)
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
vhost_user_vring_t * vrings
#define VLIB_CLI_COMMAND(x,...)
static_always_inline void vnet_hw_if_rx_queue_set_int_pending(vnet_main_t *vnm, u32 queue_index)
@ VHOST_USER_SET_VRING_BASE
@ VHOST_USER_SET_FEATURES
unformat_function_t unformat_vnet_hw_interface
#define VRING_USED_F_NO_NOTIFY
#define CLIB_CACHE_LINE_BYTES
struct _vlib_node_registration vlib_node_registration_t
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
vhost_cpu_t * cpus
Per-CPU data for vhost-user.
#define VHOST_USER_PROTOCOL_F_MQ
void vnet_hw_if_set_rx_queue_file_index(vnet_main_t *vnm, u32 queue_index, u32 file_index)
static_always_inline int vhost_user_intf_ready(vhost_user_intf_t *vui)
Returns whether at least one TX and one RX vring are enabled.
void * region_mmap_addr[VHOST_MEMORY_MAX_NREGIONS]
@ VHOST_USER_GET_VRING_BASE
vring_desc_event_t * used_event
#define VHOST_VRING_INIT_MQ_PAIR_SZ
#define vec_free(V)
Free vector's memory (no header).
static uword vhost_user_process(vlib_main_t *vm, vlib_node_runtime_t *rt, vlib_frame_t *f)
#define VHOST_USER_EVENT_START_TIMER
static long get_huge_page_size(int fd)
format_function_t format_vnet_sw_if_index_name
unformat_function_t unformat_vnet_sw_interface
static f64 vlib_process_wait_for_event_or_clock(vlib_main_t *vm, f64 dt)
Suspend a cooperative multi-tasking thread Waits for an event, or for the indicated number of seconds...
description fragment has unexpected format
u32 * show_dev_instance_by_real_dev_instance
static void clib_file_del(clib_file_main_t *um, clib_file_t *f)
vhost_user_main_t vhost_user_main
static vnet_sw_interface_t * vnet_get_hw_sw_interface(vnet_main_t *vnm, u32 hw_if_index)
#define VLIB_BUFFER_PRE_DATA_SIZE
#define VLIB_INIT_FUNCTION(x)
void ethernet_delete_interface(vnet_main_t *vnm, u32 hw_if_index)
static vlib_cli_command_t vhost_user_delete_command
(constructor) VLIB_CLI_COMMAND (vhost_user_delete_command)
static clib_error_t * vhost_user_kickfd_read_ready(clib_file_t *uf)
static_always_inline void vhost_user_vring_init(vhost_user_intf_t *vui, u32 qid)
#define vec_foreach(var, vec)
Vector iterator.
u64 region_guest_addr_hi[VHOST_MEMORY_MAX_NREGIONS]
#define clib_error_return_unix(e, args...)
static uword clib_file_add(clib_file_main_t *um, clib_file_t *template)
static clib_error_t * vhost_user_init(vlib_main_t *vm)
static uword vhost_user_send_interrupt_process(vlib_main_t *vm, vlib_node_runtime_t *rt, vlib_frame_t *f)
int vhost_user_modify_if(vnet_main_t *vnm, vlib_main_t *vm, vhost_user_create_if_args_t *args)
static_always_inline void vhost_user_vring_close(vhost_user_intf_t *vui, u32 qid)
@ foreach_virtio_event_idx_flags
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
static u32 vlib_get_n_threads()
u32 vnet_hw_if_register_rx_queue(vnet_main_t *vnm, u32 hw_if_index, u32 queue_id, u32 thread_index)
#define VHOST_VRING_MAX_MQ_PAIR_SZ
static vlib_main_t * vlib_get_main(void)
clib_error_t * vnet_hw_interface_set_flags(vnet_main_t *vnm, u32 hw_if_index, vnet_hw_interface_flags_t flags)
vnet_interface_output_runtime_t * rt
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
static u8 * format_vhost_user_desc(u8 *s, va_list *args)
static void unmap_all_mem_regions(vhost_user_intf_t *vui)
static_always_inline void * map_guest_mem(vhost_user_intf_t *vui, uword addr, u32 *hint)
static int vhost_user_init_server_sock(const char *sock_filename, int *sock_fd)
Open server unix socket on specified sock_filename.
@ VHOST_USER_SET_VRING_ENABLE
clib_spinlock_t vring_lock
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_UDP_CKSUM
static u8 * format_vhost_user_event_idx_flags(u8 *s, va_list *args)
#define UNIX_GET_FD(unixfd_idx)
static_always_inline void vhost_user_rx_thread_placement(vhost_user_intf_t *vui, u32 qid)
Unassign existing interface/queue to thread mappings and re-assign new interface/queue to thread mapp...
#define clib_warning(format, args...)
static uword vlib_process_suspend_time_is_zero(f64 dt)
Returns TRUE if a process suspend time is less than 10us.
#define vu_log_debug(dev, f,...)
@ VHOST_USER_GET_FEATURES
@ VNET_HW_INTERFACE_CAP_SUPPORTS_INT_MODE
static void vhost_user_create_ethernet(vnet_main_t *vnm, vlib_main_t *vm, vhost_user_intf_t *vui, vhost_user_create_if_args_t *args)
Create ethernet interface for vhost user interface.
vhost_user_intf_t * vhost_user_interfaces
int vhost_user_delete_if(vnet_main_t *vnm, vlib_main_t *vm, u32 sw_if_index)
u32 region_mmap_fd[VHOST_MEMORY_MAX_NREGIONS]
static_always_inline void vhost_user_if_disconnect(vhost_user_intf_t *vui)
vlib_log_class_t log_default
static f64 vlib_time_now(vlib_main_t *vm)
#define VLIB_MAIN_LOOP_EXIT_FUNCTION(x)
@ VHOST_USER_GET_QUEUE_NUM
@ VHOST_USER_SET_VRING_ERR
static_always_inline u32 vnet_hw_if_get_rx_queue_thread_index(vnet_main_t *vnm, u32 queue_index)
u32 vnet_hw_if_register_tx_queue(vnet_main_t *vnm, u32 hw_if_index, u32 queue_id)
static vlib_thread_main_t * vlib_get_thread_main()
@ VHOST_USER_SET_VRING_CALL
int dont_dump_vhost_user_memory
vl_api_interface_index_t sw_if_index
void vhost_user_set_operation_mode(vhost_user_intf_t *vui, vhost_user_vring_t *txvq)
u32 random
Pseudo random iterator.
static clib_error_t * vhost_user_socket_read(clib_file_t *uf)
__clib_export uword mhash_set_mem(mhash_t *h, void *key, uword *new_value, uword *old_value)
__clib_export uword mhash_unset(mhash_t *h, void *key, uword *old_value)
vlib_node_registration_t vhost_user_send_interrupt_node
(constructor) VLIB_REGISTER_NODE (vhost_user_send_interrupt_node)
static clib_error_t * vhost_user_exit(vlib_main_t *vm)
@ VHOST_USER_SET_MEM_TABLE
clib_error_t * ethernet_register_interface(vnet_main_t *vnm, u32 dev_class_index, u32 dev_instance, const u8 *address, u32 *hw_if_index_return, ethernet_flag_change_function_t flag_change)
void vnet_hw_if_set_input_node(vnet_main_t *vnm, u32 hw_if_index, u32 node_index)
@ VNET_HW_IF_RX_MODE_UNKNOWN
int vhost_user_create_if(vnet_main_t *vnm, vlib_main_t *vm, vhost_user_create_if_args_t *args)
mhash_t if_index_by_sock_name
@ VHOST_USER_SET_VRING_ADDR
#define VLIB_REGISTER_NODE(x,...)
static uword random_default_seed(void)
Default random seed (unix/linux user-mode)
vl_api_address_union_t un
vl_api_wireguard_peer_flags_t flags
clib_error_t * vhost_user_delete_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)