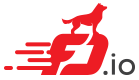 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
25 #include <vpp/app/version.h>
77 #define VRRP4_MCAST_ADDR_AS_U8 { 224, 0, 0, 18 }
78 #define VRRP6_MCAST_ADDR_AS_U8 \
79 { 0xff, 0x2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0x12 }
100 const ip46_address_t *
dst)
108 ip4->ip_version_and_header_length = 0x45;
110 ip4->protocol = IP_PROTOCOL_VRRP;
119 ip4->length = clib_host_to_net_u16 (
sizeof (*
ip4) +
126 return sizeof (*ip4);
133 ip6->ip_version_traffic_class_and_flow_label = 0x00000060;
134 ip6->hop_limit = 255;
135 ip6->protocol = IP_PROTOCOL_VRRP;
144 return sizeof (*ip6);
155 int word_size =
sizeof (
uword);
170 for (
i = 0;
i < (2 * addr_len);
i += word_size)
172 if (word_size ==
sizeof (
u64))
181 clib_host_to_net_u32 (
len + (
proto << 16)));
196 ip46_address_t *vr_addr;
209 len =
sizeof (*vrrp) + n_addrs *
sizeof (ip6_address_t);;
212 ip6->payload_length = clib_host_to_net_u16 (
len);
220 vrrp->vrrp_version_and_type = 0x31;
227 hdr_addr = (
void *) (vrrp + 1);
297 clib_warning (
"Unicast VR configuration corrupted for %U",
323 b->
flags |= VNET_BUFFER_F_LOCALLY_ORIGINATED;
359 icmp6_neighbor_solicitation_or_advertisement_header_t *na;
360 icmp6_neighbor_discovery_ethernet_link_layer_address_option_t *ll_opt;
361 int payload_length, bogus_length;
362 int rewrite_bytes = 0;
386 ip6->ip_version_traffic_class_and_flow_label = 0x00000060;
387 ip6->protocol = IP_PROTOCOL_ICMP6;
388 ip6->hop_limit = 255;
390 IP6_MULTICAST_SCOPE_link_local,
391 IP6_MULTICAST_GROUP_ID_all_hosts);
397 na = (icmp6_neighbor_solicitation_or_advertisement_header_t *) (
ip6 + 1);
399 (icmp6_neighbor_discovery_ethernet_link_layer_address_option_t *) (na +
402 payload_length =
sizeof (*na) +
sizeof (*ll_opt);
406 na->icmp.type = ICMP6_neighbor_advertisement;
407 na->target_address = *addr6;
408 na->advertisement_flags = clib_host_to_net_u32
412 ll_opt->header.type =
413 ICMP6_NEIGHBOR_DISCOVERY_OPTION_target_link_layer_address;
414 ll_opt->header.n_data_u64s = 1;
418 ip6->payload_length = clib_host_to_net_u16 (payload_length);
424 .
bytes = {0xff, 0xff, 0xff, 0xff, 0xff, 0xff,},
453 arp->
l2_type = clib_host_to_net_u16 (ETHERNET_ARP_HARDWARE_TYPE_ethernet);
454 arp->
l3_type = clib_host_to_net_u16 (ETHERNET_TYPE_IP4);
457 arp->
opcode = clib_host_to_net_u16 (ETHERNET_ARP_OPCODE_request);
481 clib_warning (
"Unable to send gratuitous ARP for VR %U - no addresses",
504 ip46_address_t *
addr;
509 b->
flags |= VNET_BUFFER_F_LOCALLY_ORIGINATED;
529 #define IGMP4_MCAST_ADDR_AS_U8 { 224, 0, 0, 22 }
534 .protocol = IP_PROTOCOL_IGMP,
558 ip4_options = (
u8 *) (
ip4 + 1);
559 ip4_options[0] = 0x94;
560 ip4_options[1] = 0x04;
561 ip4_options[2] = 0x0;
562 ip4_options[3] = 0x0;
569 report->
header.
type = IGMP_TYPE_membership_report_v3;
573 report->
n_groups = clib_host_to_net_u16 (1);
579 group->
type = IGMP_MEMBERSHIP_GROUP_change_to_exclude;
600 ip6_hop_by_hop_ext_t ext_hdr;
601 ip6_router_alert_option_t alert;
602 ip6_padN_option_t
pad;
603 icmp46_header_t
icmp;
605 u16 num_addr_records;
606 icmp6_multicast_address_record_t records[0];
607 }) icmp6_multicast_listener_report_header_t;
614 icmp6_multicast_listener_report_header_t *rh;
615 icmp6_multicast_address_record_t *rr;
616 ip46_address_t *vr_addr;
617 int bogus_length, n_addrs;
621 payload_length =
sizeof (*rh) + (n_addrs *
sizeof (*rr));
623 b->
error = ICMP6_ERROR_NONE;
626 rh = (icmp6_multicast_listener_report_header_t *) (
ip6 + 1);
627 rr = (icmp6_multicast_address_record_t *) (rh + 1);
631 ip6->ip_version_traffic_class_and_flow_label =
632 clib_host_to_net_u32 (0x60000000);
634 ip6->protocol = IP_PROTOCOL_IP6_HOP_BY_HOP_OPTIONS;
636 IP6_MULTICAST_SCOPE_link_local,
637 IP6_MULTICAST_GROUP_ID_mldv2_routers);
644 rh->ext_hdr.next_hdr = IP_PROTOCOL_ICMP6;
645 rh->ext_hdr.n_data_u64s = 0;
655 rh->icmp.type = ICMP6_multicast_listener_report_v2;
656 rh->icmp.checksum = 0;
659 rh->num_addr_records = clib_host_to_net_u16 (n_addrs);
665 rr->aux_data_len_u32s = 0;
677 rr->aux_data_len_u32s = 0;
680 id = clib_net_to_host_u32 (vr_addr->ip6.as_u32[3]) & 0x00ffffff;
684 ip6->payload_length = clib_host_to_net_u16 (payload_length);
697 u32 bi = 0, *to_next;
719 b->
flags |= VNET_BUFFER_F_LOCALLY_ORIGINATED;
729 vrrp_icmp6_mlr_pkt_build (vr,
b);
vlib_node_registration_t ip4_lookup_node
(constructor) VLIB_REGISTER_NODE (ip4_lookup_node)
#define IGMP4_MCAST_ADDR_AS_U8
#define ICMP6_NEIGHBOR_ADVERTISEMENT_FLAG_OVERRIDE
static void ip6_multicast_ethernet_address(u8 *ethernet_address, u32 group_id)
u8 * ethernet_build_rewrite(vnet_main_t *vnm, u32 sw_if_index, vnet_link_t link_type, const void *dst_address)
build a rewrite string to use for sending packets of type 'link_type' to 'dst_address'
igmp_membership_group_v3_type_t type
static void vlib_buffer_chain_increase_length(vlib_buffer_t *first, vlib_buffer_t *last, i32 len)
ip46_address_t * vr_addrs
static const ip4_header_t igmp_ip4_mcast
#define VRRP6_MCAST_ADDR_AS_U8
u16 ip6_tcp_udp_icmp_compute_checksum(vlib_main_t *vm, vlib_buffer_t *p0, ip6_header_t *ip0, int *bogus_lengthp)
#define clib_memcpy(d, s, n)
ip46_address_t * peer_addrs
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
u8 * format_vrrp_vr_key(u8 *s, va_list *args)
const mac_address_t broadcast_mac
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
vrrp_vr_runtime_t runtime
static u8 vrrp_vr_is_ipv6(vrrp_vr_t *vr)
static int vrrp_adv_l2_build_multicast(vrrp_vr_t *vr, vlib_buffer_t *b)
vlib_frame_t * vlib_get_frame_to_node(vlib_main_t *vm, u32 to_node_index)
const static_always_inline ip46_address_t * vrrp_adv_mcast_addr(vrrp_vr_t *vr)
u16 vrrp_adv_csum(void *l3_hdr, void *payload, u8 is_ipv6, u16 len)
vlib_node_registration_t ip4_rewrite_mcast_node
(constructor) VLIB_REGISTER_NODE (ip4_rewrite_mcast_node)
vlib_node_registration_t ip6_lookup_node
(constructor) VLIB_REGISTER_NODE (ip6_lookup_node)
static void vrrp4_garp_pkt_build(vrrp_vr_t *vr, vlib_buffer_t *b, ip4_address_t *ip4)
static u8 vrrp_vr_is_unicast(vrrp_vr_t *vr)
#define vec_elt(v, i)
Get vector value at index i.
int vrrp_adv_send(vrrp_vr_t *vr, int shutdown)
i16 current_data
signed offset in data[], pre_data[] that we are currently processing.
void vlib_put_frame_to_node(vlib_main_t *vm, u32 to_node_index, vlib_frame_t *f)
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
void * ip_interface_get_first_ip(u32 sw_if_index, u8 is_ip4)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
vlib_error_t error
Error code for buffers to be enqueued to error handler.
static __clib_warn_unused_result u32 vlib_buffer_alloc(vlib_main_t *vm, u32 *buffers, u32 n_buffers)
Allocate buffers into supplied array.
const ip6_address_t * ip6_get_link_local_address(u32 sw_if_index)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
vnet_main_t * vnet_get_main(void)
vl_api_mac_address_t src_addr
static void ip6_set_solicited_node_multicast_address(ip6_address_t *a, u32 id)
static ip_csum_t ip_incremental_checksum(ip_csum_t sum, void *_data, uword n_bytes)
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
static const u8 vrrp6_dst_mac[6]
#define static_always_inline
vlib_node_registration_t ip6_rewrite_mcast_node
(constructor) VLIB_REGISTER_NODE (ip6_rewrite_mcast_node)
static int vrrp_adv_l3_build(vrrp_vr_t *vr, vlib_buffer_t *b, const ip46_address_t *dst)
#define clib_mem_unaligned(pointer, type)
int vrrp_vr_multicast_group_join(vrrp_vr_t *vr)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
static void ip6_address_copy(ip6_address_t *dst, const ip6_address_t *src)
u16 current_length
Nbytes between current data and the end of this buffer.
#define VRRP4_MCAST_ADDR_AS_U8
#define ICMP6_NEIGHBOR_ADVERTISEMENT_FLAG_ROUTER
static void ip6_set_reserved_multicast_address(ip6_address_t *a, ip6_multicast_address_scope_t scope, u16 id)
bool ip6_link_is_enabled(u32 sw_if_index)
#define vec_free(V)
Free vector's memory (no header).
static const u8 vrrp_src_mac_prefix[4]
static void vrrp6_na_pkt_build(vrrp_vr_t *vr, vlib_buffer_t *b, ip6_address_t *addr6)
static ip_csum_t ip_csum_with_carry(ip_csum_t sum, ip_csum_t x)
static const u8 vrrp4_dst_mac[6]
static const ip46_address_t vrrp4_mcast_addr
#define vec_foreach(var, vec)
Vector iterator.
#define IP6_MLDP_ALERT_TYPE
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
enum vnet_link_t_ vnet_link_t
Link Type: A description of the protocol of packets on the link.
static void vrrp_igmp_pkt_build(vrrp_vr_t *vr, vlib_buffer_t *b)
static vlib_main_t * vlib_get_main(void)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
static u16 ip4_header_checksum(ip4_header_t *i)
#define clib_warning(format, args...)
static uword vnet_sw_interface_is_up(vnet_main_t *vnm, u32 sw_if_index)
u8 pad[3]
log2 (size of the packing page block)
static void vlib_buffer_reset(vlib_buffer_t *b)
Reset current header & length to state they were in when packet was received.
int vrrp_garp_or_na_send(vrrp_vr_t *vr)
static u16 ip_csum_fold(ip_csum_t c)
static u8 vrrp_vr_priority(vrrp_vr_t *vr)
static vrrp_intf_t * vrrp_intf_get(u32 sw_if_index)
static int vrrp_adv_payload_build(vrrp_vr_t *vr, vlib_buffer_t *b, int shutdown)
static u16 vrrp_adv_payload_len(vrrp_vr_t *vr)
static_always_inline u32 vrrp_adv_next_node(vrrp_vr_t *vr)
typedef CLIB_PACKED(struct { ip6_hop_by_hop_ext_t ext_hdr;ip6_router_alert_option_t alert;ip6_padN_option_t pad;icmp46_header_t icmp;u16 rsvd;u16 num_addr_records;icmp6_multicast_address_record_t records[0];})
adj_index_t mcast_adj_index[2]
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
static const ip46_address_t vrrp6_mcast_addr
ip4_address_t group_address
VLIB buffer representation.