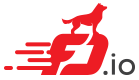 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
34 case IKEV2_ID_TYPE_ID_IPV4_ADDR:
37 case IKEV2_ID_TYPE_ID_IPV6_ADDR:
40 case IKEV2_ID_TYPE_ID_FQDN:
41 case IKEV2_ID_TYPE_ID_RFC822_ADDR:
59 s =
format (s,
"%u type %u protocol_id %u addr "
60 "%U - %U port %u - %u\n",
65 clib_net_to_host_u16 (ts->
end_port));
84 IKEV2_TRANSFORM_TYPE_ENCR);
88 IKEV2_TRANSFORM_TYPE_INTEG);
92 IKEV2_TRANSFORM_TYPE_ESN);
102 s =
format (s,
"%USK_e i:%U\n%Ur:%U\n",
109 s =
format (s,
"%USK_a i:%U\n%Ur:%U\n",
128 int details = va_arg (*va,
int);
133 s =
format (s,
"iip %U ispi %lx rip %U rspi %lx",
155 s =
format (s,
"nonce i:%U\n%Ur:%U\n",
164 s =
format (s,
"%USK_a i:%U\n%Ur:%U\n",
170 s =
format (s,
"%USK_e i:%U\n%Ur:%U\n",
175 s =
format (s,
"%USK_p i:%U\n%Ur:%U\n",
181 s =
format (s,
"%Uidentifier (i) %U\n",
184 s =
format (s,
"%Uidentifier (r) %U\n",
194 s =
format (s,
"Stats:\n");
215 int details = 0, show_one = 0;
225 else if (
unformat (line_input,
"details"))
258 .path =
"show ikev2 sa",
259 .short_help =
"show ikev2 sa [rspi <rspi>] [details]",
275 .path =
"ikev2 dpd disable",
276 .short_help =
"ikev2 dpd disable",
284 u8 **string_return = va_arg (*va,
u8 **);
285 const char *token_chars =
"a-zA-Z0-9_";
309 u32 tmp1, tmp2, tmp3;
312 u32 responder_sw_if_index = (
u32) ~ 0;
313 u32 tun_sw_if_index = (
u32) ~ 0;
333 else if (
unformat (line_input,
"set %U auth shared-key-mic string %v",
338 IKEV2_AUTH_METHOD_SHARED_KEY_MIC,
data,
342 else if (
unformat (line_input,
"set %U auth shared-key-mic hex %U",
348 IKEV2_AUTH_METHOD_SHARED_KEY_MIC,
data,
352 else if (
unformat (line_input,
"set %U auth rsa-sig cert-file %v",
360 else if (
unformat (line_input,
"set %U id local %U %U",
371 else if (
unformat (line_input,
"set %U id local %U 0x%U",
380 else if (
unformat (line_input,
"set %U id local %U %v",
388 else if (
unformat (line_input,
"set %U id remote %U %U",
399 else if (
unformat (line_input,
"set %U id remote %U 0x%U",
408 else if (
unformat (line_input,
"set %U id remote %U %v",
416 else if (
unformat (line_input,
"set %U traffic-selector local "
417 "ip-range %U - %U port-range %u - %u protocol %u",
427 else if (
unformat (line_input,
"set %U traffic-selector remote "
428 "ip-range %U - %U port-range %u - %u protocol %u",
438 else if (
unformat (line_input,
"set %U responder %U %U",
447 else if (
unformat (line_input,
"set %U responder %U %v",
450 &responder_sw_if_index, &
data))
453 responder_sw_if_index);
456 else if (
unformat (line_input,
"set %U tunnel %U",
466 "set %U ike-crypto-alg %U %u ike-integ-alg %U ike-dh %U",
480 "set %U ike-crypto-alg %U %u ike-dh %U",
487 IKEV2_TRANSFORM_INTEG_TYPE_NONE,
494 "set %U esp-crypto-alg %U %u esp-integ-alg %U",
506 "set %U esp-crypto-alg %U %u",
514 else if (
unformat (line_input,
"set %U sa-lifetime %lu %u %u %lu",
516 &tmp4, &tmp1, &tmp2, &tmp5))
522 else if (
unformat (line_input,
"set %U udp-encap",
528 else if (
unformat (line_input,
"set %U ipsec-over-udp port %u",
536 else if (
unformat (line_input,
"set %U disable natt",
558 .path =
"ikev2 profile",
560 "ikev2 profile [add|del] <id>\n"
561 "ikev2 profile set <id> auth [rsa-sig|shared-key-mic] [cert-file|string|hex]"
563 "ikev2 profile set <id> id <local|remote> <type> <data>\n"
564 "ikev2 profile set <id> tunnel <interface>\n"
565 "ikev2 profile set <id> udp-encap\n"
566 "ikev2 profile set <id> traffic-selector <local|remote> ip-range "
567 "<start-addr> - <end-addr> port-range <start-port> - <end-port> "
568 "protocol <protocol-number>\n"
569 "ikev2 profile set <id> responder <interface> <addr>\n"
570 "ikev2 profile set <id> ike-crypto-alg <crypto alg> <key size> ike-integ-alg <integ alg> ike-dh <dh type>\n"
571 "ikev2 profile set <id> esp-crypto-alg <crypto alg> <key size> "
572 "[esp-integ-alg <integ alg>]\n"
573 "ikev2 profile set <id> sa-lifetime <seconds> <jitter> <handover> <max bytes>"
574 "ikev2 profile set <id> disable natt\n",
661 .path =
"show ikev2 profile",
662 .short_help =
"show ikev2 profile",
674 u32 period = 0, max_retries = 0;
681 if (
unformat (line_input,
"%d %d", &period, &max_retries))
700 .path =
"ikev2 set liveness",
701 .short_help =
"ikev2 set liveness <period> <max-retires>",
740 .path =
"set ikev2 local key",
742 "set ikev2 local key <file>",
768 else if (
unformat (line_input,
"del-child-sa %x", &tmp1))
773 else if (
unformat (line_input,
"del-sa %lx", &tmp2))
778 else if (
unformat (line_input,
"rekey-child-sa %x", &tmp1))
798 .path =
"ikev2 initiate",
800 "ikev2 initiate sa-init <profile id>\n"
801 "ikev2 initiate del-child-sa <child sa ispi>\n"
802 "ikev2 initiate del-sa <sa ispi>\n"
803 "ikev2 initiate rekey-child-sa <child sa ispi>\n",
843 .path =
"ikev2 set logging level",
845 .short_help =
"ikev2 set logging level <0-5>",
static clib_error_t * ikev2_initiate_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
ikev2_sa_proposal_t * i_proposals
clib_error_t * ikev2_set_profile_sa_lifetime(vlib_main_t *vm, u8 *name, u64 lifetime, u32 jitter, u32 handover, u64 maxdata)
clib_error_t * ikev2_set_profile_tunnel_interface(vlib_main_t *vm, u8 *name, u32 sw_if_index)
ikev2_profile_t * profiles
clib_error_t * ikev2_initiate_rekey_child_sa(vlib_main_t *vm, u32 ispi)
vl_api_address_t end_addr
u8 * format_ikev2_sa_transform(u8 *s, va_list *args)
uword unformat_ikev2_id_type(unformat_input_t *input, va_list *args)
#define vec_new(T, N)
Create new vector of given type and length (unspecified alignment, no header).
#define clib_memcpy(d, s, n)
static clib_error_t * ikev2_disable_dpd_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
ikev2_traffic_selector_type_t ts_type
static vlib_cli_command_t ikev2_set_log_level_command
(constructor) VLIB_CLI_COMMAND (ikev2_set_log_level_command)
u8 * format_ikev2_id_type(u8 *s, va_list *args)
static vlib_cli_command_t set_ikev2_local_key_command
(constructor) VLIB_CLI_COMMAND (set_ikev2_local_key_command)
#define clib_error_return(e, args...)
ikev2_auth_method_t method
ikev2_transforms_set ike_ts
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
ikev2_child_sa_t * childs
static u8 * format_vnet_api_errno(u8 *s, va_list *args)
clib_error_t * ikev2_set_profile_esp_transforms(vlib_main_t *vm, u8 *name, ikev2_transform_encr_type_t crypto_alg, ikev2_transform_integ_type_t integ_alg, u32 crypto_key_size)
clib_error_t * ikev2_set_local_key(vlib_main_t *vm, u8 *file)
vnet_hw_if_output_node_runtime_t * r
static vlib_cli_command_t show_ikev2_sa_command
(constructor) VLIB_CLI_COMMAND (show_ikev2_sa_command)
clib_error_t * ikev2_set_profile_id(vlib_main_t *vm, u8 *name, u8 id_type, u8 *data, int is_local)
vnet_api_error_t ikev2_set_profile_ipsec_udp_port(vlib_main_t *vm, u8 *name, u16 port, u8 is_set)
u16 ip_address_size(const ip_address_t *a)
ikev2_main_per_thread_data_t * per_thread_data
static vlib_cli_command_t show_ikev2_profile_command
(constructor) VLIB_CLI_COMMAND (show_ikev2_profile_command)
#define IPSEC_UDP_PORT_NONE
static vlib_cli_command_t ikev2_profile_add_del_command
(constructor) VLIB_CLI_COMMAND (ikev2_profile_add_del_command)
#define pool_foreach(VAR, POOL)
Iterate through pool.
uword unformat_ip_address(unformat_input_t *input, va_list *args)
static clib_error_t * set_ikev2_local_key_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
uword unformat_ikev2_transform_encr_type(unformat_input_t *input, va_list *args)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
static clib_error_t * show_ikev2_profile_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
clib_error_t * ikev2_initiate_delete_child_sa(vlib_main_t *vm, u32 ispi)
vnet_main_t * vnet_get_main(void)
static uword unformat_ikev2_token(unformat_input_t *input, va_list *va)
u8 * format_ikev2_transform_dh_type(u8 *s, va_list *args)
u8 * format_ip_address(u8 *s, va_list *args)
u8 * format_ikev2_transform_integ_type(u8 *s, va_list *args)
static vlib_cli_command_t ikev2_initiate_command
(constructor) VLIB_CLI_COMMAND (ikev2_initiate_command)
uword unformat_ikev2_transform_dh_type(unformat_input_t *input, va_list *args)
clib_error_t * ikev2_set_profile_responder(vlib_main_t *vm, u8 *name, u32 sw_if_index, ip_address_t addr)
ikev2_sa_proposal_t * r_proposals
#define VLIB_CLI_COMMAND(x,...)
static vlib_cli_command_t set_ikev2_liveness_command
(constructor) VLIB_CLI_COMMAND (set_ikev2_liveness_command)
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
clib_error_t * ikev2_initiate_sa_init(vlib_main_t *vm, u8 *name)
void ikev2_cli_reference(void)
clib_error_t * ikev2_set_profile_auth(vlib_main_t *vm, u8 *name, u8 auth_method, u8 *auth_data, u8 data_hex_format)
bool ip_address_is_zero(const ip_address_t *ip)
static u8 * format_ikev2_child_sa(u8 *s, va_list *va)
#define vec_free(V)
Free vector's memory (no header).
u8 * format_ikev2_id_type_and_data(u8 *s, va_list *args)
clib_error_t * ikev2_profile_natt_disable(u8 *name)
clib_error_t * ikev2_set_profile_ike_transforms(vlib_main_t *vm, u8 *name, ikev2_transform_encr_type_t crypto_alg, ikev2_transform_integ_type_t integ_alg, ikev2_transform_dh_type_t dh_type, u32 crypto_key_size)
format_function_t format_vnet_sw_if_index_name
unformat_function_t unformat_vnet_sw_interface
description fragment has unexpected format
ikev2_responder_t responder
ikev2_sa_proposal_t * r_proposals
#define vec_foreach(var, vec)
Vector iterator.
clib_error_t * ikev2_set_profile_udp_encap(vlib_main_t *vm, u8 *name)
u8 * format_ikev2_auth_method(u8 *s, va_list *args)
static clib_error_t * set_ikev2_liveness_period_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
ikev2_transforms_set esp_ts
static u8 * format_ikev2_traffic_selector(u8 *s, va_list *va)
static clib_error_t * show_ikev2_sa_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
ikev2_transform_dh_type_t
uword unformat_ikev2_transform_integ_type(unformat_input_t *input, va_list *args)
u8 * format_ikev2_transform_encr_type(u8 *s, va_list *args)
clib_error_t * ikev2_initiate_delete_ike_sa(vlib_main_t *vm, u64 ispi)
clib_error_t * ikev2_add_del_profile(vlib_main_t *vm, u8 *name, int is_add)
clib_error_t * ikev2_set_profile_ts(vlib_main_t *vm, u8 *name, u8 protocol_id, u16 start_port, u16 end_port, ip_address_t start_addr, ip_address_t end_addr, int is_local)
static clib_error_t * ikev2_set_log_level_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
ikev2_transform_encr_type_t
void ikev2_disable_dpd(void)
static clib_error_t * ikev2_profile_add_del_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static u8 * format_ikev2_sa(u8 *s, va_list *va)
int ikev2_set_log_level(ikev2_log_level_t log_level)
clib_error_t * ikev2_set_liveness_params(u32 period, u32 max_retries)
clib_error_t * ikev2_set_profile_responder_hostname(vlib_main_t *vm, u8 *name, u8 *hostname, u32 sw_if_index)
ikev2_transform_integ_type_t
ikev2_sa_transform_t * ikev2_sa_get_td_for_type(ikev2_sa_proposal_t *p, ikev2_transform_type_t type)
static vlib_cli_command_t ikev2_cli_disable_dpd_command
(constructor) VLIB_CLI_COMMAND (ikev2_cli_disable_dpd_command)
u8 * ip_addr_bytes(ip_address_t *ip)