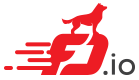 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
27 #define foreach_ah_decrypt_next \
28 _(DROP, "error-drop") \
29 _(IP4_INPUT, "ip4-input") \
30 _(IP6_INPUT, "ip6-input") \
33 #define _(v, s) AH_DECRYPT_NEXT_##v,
41 #define foreach_ah_decrypt_error \
42 _ (RX_PKTS, "AH pkts received") \
43 _ (DECRYPTION_FAILED, "AH decryption failed") \
44 _ (INTEG_ERROR, "Integrity check failed") \
45 _ (NO_TAIL_SPACE, "not enough buffer tail space (dropped)") \
46 _ (DROP_FRAGMENTS, "IP fragments drop") \
47 _ (REPLAY, "SA replayed packet")
51 #define _(sym,str) AH_DECRYPT_ERROR_##sym,
58 #define _(sym,string) string,
77 s =
format (s,
"ah: integrity %U seq-num %d",
123 ASSERT (op - ops < n_ops);
125 if (op->
status != VNET_CRYPTO_OP_STATUS_COMPLETED)
128 b[bi]->
error =
node->errors[AH_DECRYPT_ERROR_INTEG_ERROR];
129 nexts[bi] = AH_DECRYPT_NEXT_DROP;
152 u32 current_sa_index = ~0, current_sa_bytes = 0, current_sa_pkts = 0;
165 if (
vnet_buffer (
b[0])->ipsec.sad_index != current_sa_index)
167 if (current_sa_index != ~0)
175 current_sa_bytes = current_sa_pkts = 0;
192 next[0] = AH_DECRYPT_NEXT_HANDOFF;
204 ip6_ext_header_t *prev = NULL;
214 b[0]->
error =
node->errors[AH_DECRYPT_ERROR_DROP_FRAGMENTS];
215 next[0] = AH_DECRYPT_NEXT_DROP;
222 pd->
seq = clib_host_to_net_u32 (ah0->
seq_no);
228 b[0]->
error =
node->errors[AH_DECRYPT_ERROR_REPLAY];
229 next[0] = AH_DECRYPT_NEXT_DROP;
234 current_sa_pkts += 1;
242 + sizeof (
u32) > buffer_data_size))
244 b[0]->
error =
node->errors[AH_DECRYPT_ERROR_NO_TAIL_SPACE];
245 next[0] = AH_DECRYPT_NEXT_DROP;
253 op->
src = (
u8 *) ih4;
260 if (ipsec_sa_is_set_USE_ESN (sa0))
262 u32 seq_hi = clib_host_to_net_u32 (pd->
seq_hi);
264 op->
len +=
sizeof (seq_hi);
309 current_sa_index, current_sa_pkts,
330 b[0]->
error =
node->errors[AH_DECRYPT_ERROR_REPLAY];
331 next[0] = AH_DECRYPT_NEXT_DROP;
340 b[0]->
flags |= VLIB_BUFFER_TOTAL_LENGTH_VALID;
345 next[0] = AH_DECRYPT_NEXT_IP4_INPUT;
347 next[0] = AH_DECRYPT_NEXT_IP6_INPUT;
350 b[0]->
error =
node->errors[AH_DECRYPT_ERROR_DECRYPTION_FAILED];
351 next[0] = AH_DECRYPT_NEXT_DROP;
368 next[0] = AH_DECRYPT_NEXT_IP6_INPUT;
388 next[0] = AH_DECRYPT_NEXT_IP4_INPUT;
433 .name =
"ah4-decrypt",
434 .vector_size =
sizeof (
u32),
443 [AH_DECRYPT_NEXT_DROP] =
"ip4-drop",
444 [AH_DECRYPT_NEXT_IP4_INPUT] =
"ip4-input-no-checksum",
445 [AH_DECRYPT_NEXT_IP6_INPUT] =
"ip6-input",
446 [AH_DECRYPT_NEXT_HANDOFF] =
"ah4-decrypt-handoff",
460 .name =
"ah6-decrypt",
461 .vector_size =
sizeof (
u32),
470 [AH_DECRYPT_NEXT_DROP] =
"ip6-drop",
471 [AH_DECRYPT_NEXT_IP4_INPUT] =
"ip4-input-no-checksum",
472 [AH_DECRYPT_NEXT_IP6_INPUT] =
"ip6-input",
473 [AH_DECRYPT_NEXT_HANDOFF] =
"ah6-decrypt-handoff",
478 #ifndef CLIB_MARCH_VARIANT
485 im->ah4_dec_fq_index =
487 im->ah6_dec_fq_index =
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
vnet_crypto_op_t * integ_ops
static_always_inline void vnet_crypto_op_init(vnet_crypto_op_t *op, vnet_crypto_op_id_t type)
vnet_interface_main_t * im
vlib_node_registration_t ah4_decrypt_node
(constructor) VLIB_REGISTER_NODE (ah4_decrypt_node)
static vlib_cli_command_t trace
(constructor) VLIB_CLI_COMMAND (trace)
vnet_crypto_key_index_t integ_key_index
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
static int ipsec_sa_anti_replay_and_sn_advance(const ipsec_sa_t *sa, u32 seq, u32 hi_seq_used, bool post_decrypt, u32 *hi_seq_req)
#define clib_memcpy(d, s, n)
static_always_inline void clib_memset_u16(void *p, u16 val, uword count)
vnet_crypto_op_status_t status
vlib_get_buffers(vm, from, b, n_left_from)
@ VLIB_NODE_TYPE_INTERNAL
vlib_main_t vlib_node_runtime_t * node
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
vlib_main_t vlib_node_runtime_t vlib_frame_t * from_frame
vlib_buffer_enqueue_to_next(vm, node, from,(u16 *) nexts, frame->n_vectors)
static char * ah_decrypt_error_strings[]
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
u32 vlib_frame_queue_main_init(u32 node_index, u32 frame_queue_nelts)
#define VNET_CRYPTO_OP_FLAG_HMAC_CHECK
i16 current_data
signed offset in data[], pre_data[] that we are currently processing.
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
u32 vnet_crypto_process_ops(vlib_main_t *vm, vnet_crypto_op_t ops[], u32 n_ops)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
vlib_error_t error
Error code for buffers to be enqueued to error handler.
static_always_inline void ah_process_ops(vlib_main_t *vm, vlib_node_runtime_t *node, vnet_crypto_op_t *ops, vlib_buffer_t *b[], u16 *nexts)
#define VLIB_NODE_FN(node)
static clib_error_t * ah_decrypt_init(vlib_main_t *vm)
static ipsec_sa_t * ipsec_sa_get(u32 sa_index)
static int ip4_is_fragment(const ip4_header_t *i)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
vlib_combined_counter_main_t ipsec_sa_counters
SA packet & bytes counters.
static uword ah_decrypt_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *from_frame, int is_ip6)
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
static void vlib_prefetch_combined_counter(const vlib_combined_counter_main_t *cm, u32 thread_index, u32 index)
Pre-fetch a per-thread combined counter for the given object index.
static void * ip6_ext_header_find(vlib_main_t *vm, vlib_buffer_t *b, ip6_header_t *ip6_header, u8 header_type, ip6_ext_header_t **prev_ext_header)
#define static_always_inline
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
ipsec_integ_alg_t integ_alg
#define foreach_ah_decrypt_error
static u8 ah_calc_icv_padding_len(u8 icv_size, int is_ipv6)
static u32 ipsec_sa_assign_thread(u32 thread_id)
#define CLIB_CACHE_LINE_BYTES
struct _vlib_node_registration vlib_node_registration_t
u16 current_length
Nbytes between current data and the end of this buffer.
#define vec_add2_aligned(V, P, N, A)
Add N elements to end of vector V, return pointer to new elements in P.
description fragment has unexpected format
static_always_inline u32 vlib_buffer_get_default_data_size(vlib_main_t *vm)
#define VLIB_INIT_FUNCTION(x)
#define clib_atomic_cmp_and_swap(addr, old, new)
vnet_crypto_op_id_t integ_op_id
#define foreach_ah_decrypt_next
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
static u8 * format_ah_decrypt_trace(u8 *s, va_list *args)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
static u16 ip4_header_checksum(ip4_header_t *i)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
ipsec_integ_alg_t integ_alg
u32 ip_version_traffic_class_and_flow_label
u16 nexts[VLIB_FRAME_SIZE]
static int ip4_header_bytes(const ip4_header_t *i)
vl_api_fib_path_type_t type
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
vlib_node_registration_t ah6_decrypt_node
(constructor) VLIB_REGISTER_NODE (ah6_decrypt_node)
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)
static void ipsec_sa_anti_replay_advance(ipsec_sa_t *sa, u32 seq, u32 hi_seq)
vl_api_wireguard_peer_flags_t flags