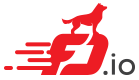 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
35 #define _(n, v) case GRE_TUNNEL_TYPE_##n: \
36 s = format (s, "%s", v); \
50 s =
format (s,
"[%d] instance %d src %U dst %U fib-idx %d sw-if-idx %d ",
59 if (t->
type == GRE_TUNNEL_TYPE_ERSPAN)
62 if (t->
type != GRE_TUNNEL_TYPE_L3)
78 a->type,
a->mode,
a->session_id, &
key->gtk_v4);
84 a->type,
a->mode,
a->session_id, &
key->gtk_v6);
227 case TUNNEL_MODE_P2P:
250 t->
type, TUNNEL_MODE_P2P, 0, &
key->gtk_v4);
255 t->
type, TUNNEL_MODE_P2P, 0, &
key->gtk_v6);
283 if (t->
mode != TUNNEL_MODE_MP)
366 u32 outer_fib_index,
u32 * sw_if_indexp)
379 return VNET_API_ERROR_IF_ALREADY_EXISTS;
385 u32 t_idx = t -
gm->tunnels;
386 u32 u_idx =
a->instance;
392 return VNET_API_ERROR_INSTANCE_IN_USE;
402 if (t->
type == GRE_TUNNEL_TYPE_ERSPAN)
405 if (t->
type == GRE_TUNNEL_TYPE_L3)
407 if (t->
mode == TUNNEL_MODE_P2P)
420 { 0xd0, 0x0b, 0xee, 0xd0, (
u8) (t_idx >> 8), (
u8) t_idx };
427 return VNET_API_ERROR_INVALID_REGISTRATION;
432 if (GRE_TUNNEL_TYPE_ERSPAN == t->
type)
452 hi->min_packet_bytes =
457 hi->min_packet_bytes =
478 if (t->
mode == TUNNEL_MODE_MP)
481 if (t->
type == GRE_TUNNEL_TYPE_ERSPAN)
503 if (t->
type != GRE_TUNNEL_TYPE_L3)
522 u32 outer_fib_index,
u32 * sw_if_indexp)
532 return VNET_API_ERROR_NO_SUCH_ENTRY;
534 if (t->
mode == TUNNEL_MODE_MP)
545 if (t->
type == GRE_TUNNEL_TYPE_L3)
585 if (~0 == outer_fib_index)
586 return VNET_API_ERROR_NO_SUCH_FIB;
589 return VNET_API_ERROR_INVALID_SESSION_ID;
592 return (VNET_API_ERROR_INVALID_DST_ADDRESS);
610 if (NULL ==
gm->tunnel_index_by_sw_if_index ||
611 hi->sw_if_index >=
vec_len (
gm->tunnel_index_by_sw_if_index))
614 ti =
gm->tunnel_index_by_sw_if_index[
hi->sw_if_index];
669 else if (
unformat (line_input,
"multipoint"))
670 t_mode = TUNNEL_MODE_MP;
671 else if (
unformat (line_input,
"teb"))
672 t_type = GRE_TUNNEL_TYPE_TEB;
674 t_type = GRE_TUNNEL_TYPE_ERSPAN;
733 case VNET_API_ERROR_IF_ALREADY_EXISTS:
736 case VNET_API_ERROR_NO_SUCH_FIB:
740 case VNET_API_ERROR_NO_SUCH_ENTRY:
743 case VNET_API_ERROR_INVALID_SESSION_ID:
747 case VNET_API_ERROR_INSTANCE_IN_USE:
764 .path =
"create gre tunnel",
765 .short_help =
"create gre tunnel src <addr> dst <addr> [instance <n>] "
766 "[outer-fib-id <fib>] [teb | erspan <session-id>] [del] "
813 .path =
"show gre tunnel",
static vlib_cli_command_t create_gre_tunnel_command
(constructor) VLIB_CLI_COMMAND (create_gre_tunnel_command)
static int vnet_gre_tunnel_add(vnet_gre_tunnel_add_del_args_t *a, u32 outer_fib_index, u32 *sw_if_indexp)
fib_protocol_t ia_nh_proto
The protocol of the neighbor/peer.
clib_error_t * vnet_sw_interface_set_flags(vnet_main_t *vnm, u32 sw_if_index, vnet_sw_interface_flags_t flags)
Used for GRE header seq number generation for ERSPAN encap.
#define hash_set(h, key, value)
teib_entry_t * teib_entry_find_46(u32 sw_if_index, fib_protocol_t fproto, const ip46_address_t *peer)
static walk_rc_t gre_tunnel_add_teib_walk(index_t nei, void *ctx)
const static teib_vft_t gre_teib_vft
enum tunnel_encap_decap_flags_t_ tunnel_encap_decap_flags_t
void vnet_sw_interface_set_mtu(vnet_main_t *vnm, u32 sw_if_index, u32 mtu)
static void gre_teib_entry_deleted(const teib_entry_t *ne)
static void gre_mk_sn_key(const gre_tunnel_t *gt, gre_sn_key_t *key)
#define clib_memcpy(d, s, n)
const ip46_address_t zero_addr
#include <vnet/feature/feature.h>
adj_index_t adj_nbr_add_or_lock(fib_protocol_t nh_proto, vnet_link_t link_type, const ip46_address_t *nh_addr, u32 sw_if_index)
Neighbour Adjacency sub-type.
#define FOR_EACH_FIB_IP_PROTOCOL(_item)
#define ip46_address_initializer
#define ADJ_INDEX_INVALID
Invalid ADJ index - used when no adj is known likewise blazoned capitals INVALID speak volumes where ...
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
#define pool_get_aligned(P, E, A)
Allocate an object E from a pool P with alignment A.
static void clib_mem_free(void *p)
enum adj_walk_rc_t_ adj_walk_rc_t
return codes from a adjacency walker callback function
static u8 ip46_address_is_ip4(const ip46_address_t *ip46)
#define clib_error_return(e, args...)
void adj_midchain_delegate_stack(adj_index_t ai, u32 fib_index, const fib_prefix_t *pfx)
create/attach a midchain delegate and stack it on the prefix passed
static void gre_mk_key6(const ip6_address_t *src, const ip6_address_t *dst, u32 fib_index, gre_tunnel_type_t ttype, tunnel_mode_t tmode, u16 session_id, gre_tunnel_key6_t *key)
static vlib_cli_command_t show_gre_tunnel_command
(constructor) VLIB_CLI_COMMAND (show_gre_tunnel_command)
static void gre_tunnel_db_add(gre_tunnel_t *t, gre_tunnel_key_t *key)
adj_walk_rc_t mgre_mk_incomplete_walk(adj_index_t ai, void *data)
clib_error_t * gre_interface_admin_up_down(vnet_main_t *vnm, u32 hw_if_index, u32 flags)
void adj_unlock(adj_index_t adj_index)
Release a reference counting lock on the adjacency.
static adj_walk_rc_t mgre_adj_walk_cb(adj_index_t ai, void *ctx)
static gre_tunnel_t * gre_tunnel_db_find(const vnet_gre_tunnel_add_del_args_t *a, u32 outer_fib_index, gre_tunnel_key_t *key)
u16 fp_len
The mask length.
tunnel_encap_decap_flags_t flags
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
#define pool_put(P, E)
Free an object E in pool P.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static void gre_teib_entry_added(const teib_entry_t *ne)
An TEIB entry has been added.
@ VNET_HW_INTERFACE_FLAG_LINK_UP
void gre_tunnel_stack(adj_index_t ai)
gre_tunnel_stack
void ip4_register_protocol(u32 protocol, u32 node_index)
#define clib_error_report(e)
const fib_prefix_t * teib_entry_get_nh(const teib_entry_t *te)
#define hash_unset(h, key)
teib_entry_t * teib_entry_get(index_t tei)
static u8 * format_gre_tunnel(u8 *s, va_list *args)
fib_prefix_t tunnel_dst
The tunnel's destination/remote address.
static void hash_set_mem_alloc(uword **h, const void *key, uword v)
void vnet_set_interface_output_node(vnet_main_t *vnm, u32 hw_if_index, u32 node_index)
Set interface output node - for interface registered without its output/tx nodes created because its ...
u8 * format_tunnel_mode(u8 *s, va_list *args)
static void mgre_tunnel_stack(adj_index_t ai)
mgre_tunnel_stack
#define ip_addr_version(_a)
#define pool_foreach(VAR, POOL)
Iterate through pool.
adj_walk_rc_t mgre_mk_complete_walk(adj_index_t ai, void *data)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
static u8 ip46_address_is_equal(const ip46_address_t *ip46_1, const ip46_address_t *ip46_2)
static int vnet_gre_tunnel_delete(vnet_gre_tunnel_add_del_args_t *a, u32 outer_fib_index, u32 *sw_if_indexp)
adj_index_t l2_adj_index
an L2 tunnel always rquires an L2 midchain.
static void gre_tunnel_db_remove(gre_tunnel_t *t, gre_tunnel_key_t *key)
enum gre_tunnel_type_t_ gre_tunnel_type_t
The GRE tunnel type.
clib_error_t * ethernet_register_interface(vnet_main_t *vnm, u32 dev_class_index, u32 dev_instance, const u8 *address, u32 *hw_if_index_return, ethernet_flag_change_function_t flag_change)
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
vnet_main_t * vnet_get_main(void)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
u8 * format_gre_tunnel_type(u8 *s, va_list *args)
void vnet_delete_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
uword unformat_tunnel_encap_decap_flags(unformat_input_t *input, va_list *args)
static void gre_teib_mk_key(const gre_tunnel_t *t, const teib_entry_t *ne, gre_tunnel_key_t *key)
A representation of a GRE tunnel.
static clib_error_t * show_gre_tunnel_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
clib_error_t * gre_interface_init(vlib_main_t *vm)
u16 session_id
ERSPAN type 2 session ID, least significant 10 bits of u16.
manual_print typedef address
#define VLIB_CLI_COMMAND(x,...)
enum fib_protocol_t_ fib_protocol_t
Protocol Type.
static void gre_tunnel_restack(gre_tunnel_t *gt)
ip46_address_t tunnel_src
The tunnel's source/local address.
vlib_node_registration_t gre_teb_encap_node
(constructor) VLIB_REGISTER_NODE (gre_teb_encap_node)
u32 teib_entry_get_fib_index(const teib_entry_t *te)
#define CLIB_CACHE_LINE_BYTES
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
ip46_address_t fp_addr
The address type is not deriveable from the fp_addr member.
void adj_midchain_delegate_unstack(adj_index_t ai)
unstack a midchain delegate (this stacks it on a drop)
void ethernet_delete_interface(vnet_main_t *vnm, u32 hw_if_index)
static u8 ip46_address_is_zero(const ip46_address_t *ip46)
static clib_error_t * create_gre_tunnel_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define hash_get_mem(h, key)
union ip_adjacency_t_::@144 sub_type
vnet_hw_interface_class_t gre_hw_interface_class
void teib_walk_itf(u32 sw_if_index, teib_walk_cb_t fn, void *ctx)
void teib_register(const teib_vft_t *vft)
@ foreach_gre_tunnel_type
format_function_t format_vnet_sw_if_index_name
description fragment has unexpected format
#define vec_validate_init_empty(V, I, INIT)
Make sure vector is long enough for given index and initialize empty space (no header,...
format_function_t format_ip46_address
u32 teib_entry_get_sw_if_index(const teib_entry_t *te)
accessors for the opaque struct
#define VLIB_INIT_FUNCTION(x)
teib_entry_added_t nv_added
const ip_address_t * teib_entry_get_peer(const teib_entry_t *te)
static uword pool_elts(void *v)
Number of active elements in a pool.
vlib_node_registration_t gre6_input_node
(constructor) VLIB_REGISTER_NODE (gre6_input_node)
#define GTK_SESSION_ID_MAX
The session ID is only a 10 bit value.
fib_protocol_t fp_proto
protocol type
static void gre_mk_key4(ip4_address_t src, ip4_address_t dst, u32 fib_index, gre_tunnel_type_t ttype, tunnel_mode_t tmode, u16 session_id, gre_tunnel_key4_t *key)
static void hash_unset_mem_free(uword **h, const void *key)
Union of the two possible key types.
u32 adj_index_t
An index for adjacencies.
enum tunnel_mode_t_ tunnel_mode_t
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
clib_error_t * vnet_hw_interface_set_flags(vnet_main_t *vnm, u32 hw_if_index, vnet_hw_interface_flags_t flags)
void adj_nbr_walk_nh(u32 sw_if_index, fib_protocol_t adj_nh_proto, const ip46_address_t *nh, adj_walk_cb_t cb, void *ctx)
Walk adjacencies on a link with a given next-hop.
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
void teib_entry_adj_stack(const teib_entry_t *te, adj_index_t ai)
gre_sn_t * gre_sn
GRE header sequence number (SN) used for ERSPAN type 2 header, must be bumped automically to be threa...
struct ip_adjacency_t_::@144::@145 nbr
IP_LOOKUP_NEXT_ARP/IP_LOOKUP_NEXT_REWRITE.
static adj_walk_rc_t gre_adj_walk_cb(adj_index_t ai, void *ctx)
Call back when restacking all adjacencies on a GRE interface.
int vnet_gre_tunnel_add_del(vnet_gre_tunnel_add_del_args_t *a, u32 *sw_if_indexp)
void adj_nbr_walk(u32 sw_if_index, fib_protocol_t adj_nh_proto, adj_walk_cb_t cb, void *ctx)
Walk all adjacencies on a link for a given next-hop protocol.
void ip6_register_protocol(u32 protocol, u32 node_index)
static uword vnet_hw_interface_get_flags(vnet_main_t *vnm, u32 hw_if_index)
vlib_node_registration_t gre4_input_node
(constructor) VLIB_REGISTER_NODE (gre4_input_node)
static walk_rc_t gre_tunnel_delete_teib_walk(index_t nei, void *ctx)
Hash key for GRE header seq number generation for ERSPAN encap.
u32 outer_fib_index
The FIB in which the src.dst address are present.
vlib_node_registration_t gre_erspan_encap_node
(constructor) VLIB_REGISTER_NODE (gre_erspan_encap_node)
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
u32 fib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
vl_api_interface_index_t sw_if_index
vnet_hw_interface_class_t mgre_hw_interface_class
enum walk_rc_t_ walk_rc_t
Walk return code.
static ip_adjacency_t * adj_get(adj_index_t adj_index)
Get a pointer to an adjacency object from its index.
static void * clib_mem_alloc(uword size)
Aggregate type for a prefix.
vl_api_fib_path_type_t type
u32 vnet_register_interface(vnet_main_t *vnm, u32 dev_class_index, u32 dev_instance, u32 hw_class_index, u32 hw_instance)
void gre_update_adj(vnet_main_t *vnm, u32 sw_if_index, adj_index_t ai)
vnet_device_class_t gre_device_class
vl_api_wireguard_peer_flags_t flags